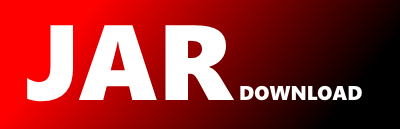
com.microsoft.bingads.v10.bulk.entities.BulkSiteLinkAdExtension Maven / Gradle / Ivy
Show all versions of microsoft.bingads Show documentation
package com.microsoft.bingads.v10.bulk.entities;
import com.microsoft.bingads.v10.bulk.BulkServiceManager;
import com.microsoft.bingads.v10.campaignmanagement.AdExtensionStatus;
import com.microsoft.bingads.v10.campaignmanagement.ArrayOfSiteLink;
import com.microsoft.bingads.v10.campaignmanagement.SiteLink;
import com.microsoft.bingads.v10.campaignmanagement.SiteLinksAdExtension;
import com.microsoft.bingads.v10.bulk.BulkOperation;
import com.microsoft.bingads.v10.bulk.BulkFileReader;
import com.microsoft.bingads.v10.bulk.BulkFileWriter;
import com.microsoft.bingads.v10.internal.bulk.StringTable;
import com.microsoft.bingads.v10.internal.bulk.BulkObjectWriter;
import com.microsoft.bingads.v10.internal.bulk.BulkStreamReader;
import com.microsoft.bingads.v10.internal.bulk.TryResult;
import com.microsoft.bingads.v10.internal.bulk.entities.MultiRecordBulkEntity;
import com.microsoft.bingads.v10.internal.bulk.entities.SiteLinkAdExtensionIdentifier;
import com.microsoft.bingads.internal.functionalinterfaces.Predicate;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.List;
/**
* Represents a sitelink ad extension that is derived from {@link BulkEntity}
* and can be read or written in a bulk file. Properties of this class and of
* classes that it is derived from, correspond to fields of the Sitelink Ad
* Extension record in a bulk file.
*
*
* For more information, see Sitelink Ad Extension at
* http://go.microsoft.com/fwlink/?LinkID=620236.
*
*
*
* The Sitelink Ad Extension record includes the distinct properties of the {@link BulkSiteLink} class,
* combined with the commmon properties of the {@link BulkSiteLinkAdExtension} class, for example
* {@link #getAccountId} and {@link #getSiteLinksAdExtension}.
*
*
*
* One {@link BulkSiteLinkAdExtension} has one or more {@link BulkSiteLink}.
* Each {@link BulkSiteLink} instance corresponds to one Sitelink Ad Extension record in the bulk file.
* If you upload a {@link BulkSiteLinkAdExtension},
* then you are effectively replacing any existing site links for the sitelink ad extension.
*
*
* @see BulkServiceManager
* @see BulkOperation
* @see BulkFileReader
* @see BulkFileWriter
*/
public class BulkSiteLinkAdExtension extends MultiRecordBulkEntity {
private Long accountId;
private SiteLinksAdExtension siteLinksAdExtension;
private final List bulkSiteLinkResults = new ArrayList();
/**
* Get The list of {@link BulkSiteLink} are represented by multiple Sitelink Ad Extension records in the file.
*
* Each item in the list corresponds to a separate Sitelink Ad Extension record that includes the distinct properties of
* the {@link BulkSiteLink} class, combined with
* the commmon properties of the {@link BulkSiteLinkAdExtension} class,
* for example {@link #getAccountId} and {@link #getSiteLinksAdExtension}.
*
*/
public List getSiteLinks() {
return Collections.unmodifiableList(this.bulkSiteLinkResults);
}
@Override
public List extends BulkEntity> getChildEntities() {
return Collections.unmodifiableList(this.bulkSiteLinkResults);
}
private SiteLinkAdExtensionIdentifier identifier;
private boolean hasDeleteAllRow;
/**
* Initializes a new instance of the BulkSiteLinkAdExtension class.
*/
public BulkSiteLinkAdExtension() {
}
public BulkSiteLinkAdExtension(SiteLinkAdExtensionIdentifier identifier) {
this();
this.identifier = identifier;
this.hasDeleteAllRow = AdExtensionStatus.DELETED.equals(identifier.getStatus());
this.siteLinksAdExtension = new SiteLinksAdExtension();
this.siteLinksAdExtension.setType(StringTable.SITE_LINKS_AD_EXTENSION);
this.siteLinksAdExtension.setId(identifier.getAdExtensionId());
this.siteLinksAdExtension.setStatus(identifier.getStatus());
this.siteLinksAdExtension.setVersion(identifier.getVersion());
this.accountId = identifier.getAccountId();
}
BulkSiteLinkAdExtension(BulkSiteLink firstSiteLink) {
this(firstSiteLink.getIdentifier());
bulkSiteLinkResults.add(firstSiteLink);
}
@Override
public void writeToStream(BulkObjectWriter rowWriter, boolean excludeReadonlyData) throws IOException {
validatePropertyNotNull(siteLinksAdExtension, StringTable.SITE_LINKS_AD_EXTENSION);
validatePropertyNotNull(siteLinksAdExtension.getId(), StringTable.SITE_LINKS_AD_EXTENSION + ".getId()");
if (!AdExtensionStatus.DELETED.equals(siteLinksAdExtension.getStatus())) {
validateListNotNullOrEmpty(
siteLinksAdExtension.getSiteLinks(),
siteLinksAdExtension.getSiteLinks().getSiteLinks(),
StringTable.SITE_LINKS_AD_EXTENSION_SITE_LINKS
);
}
SiteLinkAdExtensionIdentifier identifier = new SiteLinkAdExtensionIdentifier();
identifier.setStatus(AdExtensionStatus.DELETED);
identifier.setAccountId(accountId);
identifier.setAdExtensionId(this.siteLinksAdExtension.getId());
rowWriter.writeObjectRow(identifier, excludeReadonlyData);
if (AdExtensionStatus.DELETED.equals(siteLinksAdExtension.getStatus())) {
return;
}
for (BulkSiteLink bulkSiteLink : convertV9ToBulkSiteLinks()) {
bulkSiteLink.writeToStream(rowWriter, excludeReadonlyData);
}
}
private List convertV9ToBulkSiteLinks() {
int order = 1;
List siteLinks = siteLinksAdExtension.getSiteLinks().getSiteLinks();
List bulkSiteLinks = new ArrayList();
for (SiteLink siteLink : siteLinks) {
BulkSiteLink bulkSiteLink = new BulkSiteLink();
bulkSiteLink.setSiteLink(siteLink);
bulkSiteLink.setAccountId(accountId);
bulkSiteLink.setAdExtensionId(siteLinksAdExtension.getId());
bulkSiteLink.setOrder(order++);
bulkSiteLinks.add(bulkSiteLink);
}
return bulkSiteLinks;
}
@Override
public void readRelatedDataFromStream(BulkStreamReader reader) {
boolean hasMoreRows = true;
while (hasMoreRows) {
TryResult nextSiteLinkResult = reader.tryRead(new Predicate() {
@Override
public boolean test(BulkSiteLink x) {
return x.getIdentifier().equals(identifier);
}
}, BulkSiteLink.class);
if (nextSiteLinkResult.isSuccessful()) {
bulkSiteLinkResults.add(nextSiteLinkResult.getResult());
continue;
}
TryResult identifierResult = reader.tryRead(new Predicate() {
@Override
public boolean test(SiteLinkAdExtensionIdentifier x) {
return x.equals(identifier);
}
}, SiteLinkAdExtensionIdentifier.class);
if (identifierResult.isSuccessful()) {
if (AdExtensionStatus.DELETED.equals(identifierResult.getResult().getStatus())) {
this.hasDeleteAllRow = true;
}
} else {
hasMoreRows = false;
}
}
if (bulkSiteLinkResults.size() > 0) {
siteLinksAdExtension.setSiteLinks(this.getSortedSiteLinks());
siteLinksAdExtension.setStatus(AdExtensionStatus.ACTIVE);
} else {
siteLinksAdExtension.setStatus(AdExtensionStatus.DELETED);
}
}
private ArrayOfSiteLink getSortedSiteLinks() {
List sortedBulkSiteLinks = new ArrayList(bulkSiteLinkResults);
Collections.sort(sortedBulkSiteLinks, ORDER_BY_ORDER);
ArrayOfSiteLink apiSiteLinks = new ArrayOfSiteLink();
for (BulkSiteLink bulkSiteLink : sortedBulkSiteLinks) {
apiSiteLinks.getSiteLinks().add(bulkSiteLink.getSiteLink());
}
return apiSiteLinks;
}
static final Comparator ORDER_BY_ORDER = new Comparator() {
@Override
public int compare(BulkSiteLink o1, BulkSiteLink o2) {
return ((Integer)o1.getOrder()).compareTo(o2.getOrder());
}
};
@Override
public boolean allChildrenPresent() {
return hasDeleteAllRow;
}
/**
* Gets the ad extension's parent account identifier.
*
*
* Corresponds to the 'Parent Id' field in the bulk file.
*
*/
public Long getAccountId() {
return accountId;
}
/**
* Sets the ad extension's parent account identifier.
*
*
* Corresponds to the 'Parent Id' field in the bulk file.
*
*/
public void setAccountId(Long accountId) {
this.accountId = accountId;
}
/**
* Gets the SiteLinksAdExtension Data Object of the Campaign Management Service.
*
*
* A subset of SiteLinksAdExtension properties are available
* in the Sitelink Ad Extension record. For more information, see Sitelink Ad Extension at
* http://go.microsoft.com/fwlink/?LinkID=620236.
*
*/
public SiteLinksAdExtension getSiteLinksAdExtension() {
return siteLinksAdExtension;
}
/**
* Sets the SiteLinksAdExtension Data Object of the Campaign Management Service.
*
*
* A subset of SiteLinksAdExtension properties are available
* in the Sitelink Ad Extension record. For more information, see Sitelink Ad Extension at
* http://go.microsoft.com/fwlink/?LinkID=620236.
*
*/
public void setSiteLinksAdExtension(SiteLinksAdExtension siteLinksAdExtension) {
this.siteLinksAdExtension = siteLinksAdExtension;
}
}