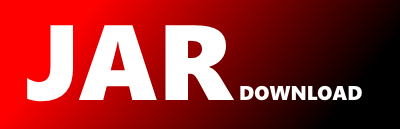
com.microsoft.bingads.v10.campaignmanagement.PlacementDetail Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of microsoft.bingads Show documentation
Show all versions of microsoft.bingads Show documentation
The Bing Ads Java SDK is a library improving developer experience when working with the Bing Ads services by providing high-level access to features such as Bulk API, OAuth Authorization and SOAP API.
package com.microsoft.bingads.v10.campaignmanagement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for PlacementDetail complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="PlacementDetail">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="ImpressionsRangePerDay" type="{https://bingads.microsoft.com/CampaignManagement/v10}ImpressionsPerDayRange" minOccurs="0"/>
* <element name="PathName" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="PlacementId" type="{http://www.w3.org/2001/XMLSchema}long" minOccurs="0"/>
* <element name="SupportedMediaTypes" type="{https://bingads.microsoft.com/CampaignManagement/v10}ArrayOfMediaType" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "PlacementDetail", propOrder = {
"impressionsRangePerDay",
"pathName",
"placementId",
"supportedMediaTypes"
})
public class PlacementDetail {
@XmlElement(name = "ImpressionsRangePerDay", nillable = true)
protected ImpressionsPerDayRange impressionsRangePerDay;
@XmlElement(name = "PathName", nillable = true)
protected String pathName;
@XmlElement(name = "PlacementId")
protected Long placementId;
@XmlElement(name = "SupportedMediaTypes", nillable = true)
protected ArrayOfMediaType supportedMediaTypes;
/**
* Gets the value of the impressionsRangePerDay property.
*
* @return
* possible object is
* {@link ImpressionsPerDayRange }
*
*/
public ImpressionsPerDayRange getImpressionsRangePerDay() {
return impressionsRangePerDay;
}
/**
* Sets the value of the impressionsRangePerDay property.
*
* @param value
* allowed object is
* {@link ImpressionsPerDayRange }
*
*/
public void setImpressionsRangePerDay(ImpressionsPerDayRange value) {
this.impressionsRangePerDay = value;
}
/**
* Gets the value of the pathName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPathName() {
return pathName;
}
/**
* Sets the value of the pathName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPathName(String value) {
this.pathName = value;
}
/**
* Gets the value of the placementId property.
*
* @return
* possible object is
* {@link Long }
*
*/
public Long getPlacementId() {
return placementId;
}
/**
* Sets the value of the placementId property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setPlacementId(Long value) {
this.placementId = value;
}
/**
* Gets the value of the supportedMediaTypes property.
*
* @return
* possible object is
* {@link ArrayOfMediaType }
*
*/
public ArrayOfMediaType getSupportedMediaTypes() {
return supportedMediaTypes;
}
/**
* Sets the value of the supportedMediaTypes property.
*
* @param value
* allowed object is
* {@link ArrayOfMediaType }
*
*/
public void setSupportedMediaTypes(ArrayOfMediaType value) {
this.supportedMediaTypes = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy