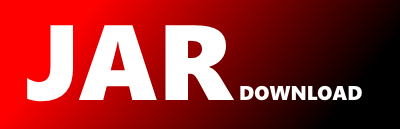
com.microsoft.bingads.v13.adinsight.IAdInsightService Maven / Gradle / Ivy
package com.microsoft.bingads.v13.adinsight;
import java.util.concurrent.Future;
import jakarta.jws.WebMethod;
import jakarta.jws.WebParam;
import jakarta.jws.WebResult;
import jakarta.jws.WebService;
import jakarta.jws.soap.SOAPBinding;
import jakarta.xml.bind.annotation.XmlSeeAlso;
import jakarta.xml.ws.AsyncHandler;
import jakarta.xml.ws.Response;
/**
* This class was generated by the XML-WS Tools.
* XML-WS Tools 4.0.1
* Generated source version: 3.0
*
*/
@WebService(name = "IAdInsightService", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13")
@SOAPBinding(parameterStyle = SOAPBinding.ParameterStyle.BARE)
@XmlSeeAlso({
ObjectFactory.class
})
public interface IAdInsightService {
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetBidOpportunities", action = "GetBidOpportunities")
public Response getBidOpportunitiesAsync(
@WebParam(name = "GetBidOpportunitiesRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetBidOpportunitiesRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetBidOpportunities", action = "GetBidOpportunities")
public Future> getBidOpportunitiesAsync(
@WebParam(name = "GetBidOpportunitiesRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetBidOpportunitiesRequest parameters,
@WebParam(name = "GetBidOpportunitiesResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.GetBidOpportunitiesResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "GetBidOpportunities", action = "GetBidOpportunities")
@WebResult(name = "GetBidOpportunitiesResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public GetBidOpportunitiesResponse getBidOpportunities(
@WebParam(name = "GetBidOpportunitiesRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetBidOpportunitiesRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetBudgetOpportunities", action = "GetBudgetOpportunities")
public Response getBudgetOpportunitiesAsync(
@WebParam(name = "GetBudgetOpportunitiesRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetBudgetOpportunitiesRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetBudgetOpportunities", action = "GetBudgetOpportunities")
public Future> getBudgetOpportunitiesAsync(
@WebParam(name = "GetBudgetOpportunitiesRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetBudgetOpportunitiesRequest parameters,
@WebParam(name = "GetBudgetOpportunitiesResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.GetBudgetOpportunitiesResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "GetBudgetOpportunities", action = "GetBudgetOpportunities")
@WebResult(name = "GetBudgetOpportunitiesResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public GetBudgetOpportunitiesResponse getBudgetOpportunities(
@WebParam(name = "GetBudgetOpportunitiesRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetBudgetOpportunitiesRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetKeywordOpportunities", action = "GetKeywordOpportunities")
public Response getKeywordOpportunitiesAsync(
@WebParam(name = "GetKeywordOpportunitiesRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetKeywordOpportunitiesRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetKeywordOpportunities", action = "GetKeywordOpportunities")
public Future> getKeywordOpportunitiesAsync(
@WebParam(name = "GetKeywordOpportunitiesRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetKeywordOpportunitiesRequest parameters,
@WebParam(name = "GetKeywordOpportunitiesResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.GetKeywordOpportunitiesResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "GetKeywordOpportunities", action = "GetKeywordOpportunities")
@WebResult(name = "GetKeywordOpportunitiesResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public GetKeywordOpportunitiesResponse getKeywordOpportunities(
@WebParam(name = "GetKeywordOpportunitiesRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetKeywordOpportunitiesRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetEstimatedBidByKeywordIds", action = "GetEstimatedBidByKeywordIds")
public Response getEstimatedBidByKeywordIdsAsync(
@WebParam(name = "GetEstimatedBidByKeywordIdsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetEstimatedBidByKeywordIdsRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetEstimatedBidByKeywordIds", action = "GetEstimatedBidByKeywordIds")
public Future> getEstimatedBidByKeywordIdsAsync(
@WebParam(name = "GetEstimatedBidByKeywordIdsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetEstimatedBidByKeywordIdsRequest parameters,
@WebParam(name = "GetEstimatedBidByKeywordIdsResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.GetEstimatedBidByKeywordIdsResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "GetEstimatedBidByKeywordIds", action = "GetEstimatedBidByKeywordIds")
@WebResult(name = "GetEstimatedBidByKeywordIdsResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public GetEstimatedBidByKeywordIdsResponse getEstimatedBidByKeywordIds(
@WebParam(name = "GetEstimatedBidByKeywordIdsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetEstimatedBidByKeywordIdsRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetEstimatedPositionByKeywordIds", action = "GetEstimatedPositionByKeywordIds")
public Response getEstimatedPositionByKeywordIdsAsync(
@WebParam(name = "GetEstimatedPositionByKeywordIdsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetEstimatedPositionByKeywordIdsRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetEstimatedPositionByKeywordIds", action = "GetEstimatedPositionByKeywordIds")
public Future> getEstimatedPositionByKeywordIdsAsync(
@WebParam(name = "GetEstimatedPositionByKeywordIdsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetEstimatedPositionByKeywordIdsRequest parameters,
@WebParam(name = "GetEstimatedPositionByKeywordIdsResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.GetEstimatedPositionByKeywordIdsResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "GetEstimatedPositionByKeywordIds", action = "GetEstimatedPositionByKeywordIds")
@WebResult(name = "GetEstimatedPositionByKeywordIdsResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public GetEstimatedPositionByKeywordIdsResponse getEstimatedPositionByKeywordIds(
@WebParam(name = "GetEstimatedPositionByKeywordIdsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetEstimatedPositionByKeywordIdsRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetEstimatedBidByKeywords", action = "GetEstimatedBidByKeywords")
public Response getEstimatedBidByKeywordsAsync(
@WebParam(name = "GetEstimatedBidByKeywordsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetEstimatedBidByKeywordsRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetEstimatedBidByKeywords", action = "GetEstimatedBidByKeywords")
public Future> getEstimatedBidByKeywordsAsync(
@WebParam(name = "GetEstimatedBidByKeywordsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetEstimatedBidByKeywordsRequest parameters,
@WebParam(name = "GetEstimatedBidByKeywordsResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.GetEstimatedBidByKeywordsResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "GetEstimatedBidByKeywords", action = "GetEstimatedBidByKeywords")
@WebResult(name = "GetEstimatedBidByKeywordsResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public GetEstimatedBidByKeywordsResponse getEstimatedBidByKeywords(
@WebParam(name = "GetEstimatedBidByKeywordsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetEstimatedBidByKeywordsRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetEstimatedPositionByKeywords", action = "GetEstimatedPositionByKeywords")
public Response getEstimatedPositionByKeywordsAsync(
@WebParam(name = "GetEstimatedPositionByKeywordsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetEstimatedPositionByKeywordsRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetEstimatedPositionByKeywords", action = "GetEstimatedPositionByKeywords")
public Future> getEstimatedPositionByKeywordsAsync(
@WebParam(name = "GetEstimatedPositionByKeywordsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetEstimatedPositionByKeywordsRequest parameters,
@WebParam(name = "GetEstimatedPositionByKeywordsResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.GetEstimatedPositionByKeywordsResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "GetEstimatedPositionByKeywords", action = "GetEstimatedPositionByKeywords")
@WebResult(name = "GetEstimatedPositionByKeywordsResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public GetEstimatedPositionByKeywordsResponse getEstimatedPositionByKeywords(
@WebParam(name = "GetEstimatedPositionByKeywordsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetEstimatedPositionByKeywordsRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetBidLandscapeByAdGroupIds", action = "GetBidLandscapeByAdGroupIds")
public Response getBidLandscapeByAdGroupIdsAsync(
@WebParam(name = "GetBidLandscapeByAdGroupIdsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetBidLandscapeByAdGroupIdsRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetBidLandscapeByAdGroupIds", action = "GetBidLandscapeByAdGroupIds")
public Future> getBidLandscapeByAdGroupIdsAsync(
@WebParam(name = "GetBidLandscapeByAdGroupIdsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetBidLandscapeByAdGroupIdsRequest parameters,
@WebParam(name = "GetBidLandscapeByAdGroupIdsResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.GetBidLandscapeByAdGroupIdsResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "GetBidLandscapeByAdGroupIds", action = "GetBidLandscapeByAdGroupIds")
@WebResult(name = "GetBidLandscapeByAdGroupIdsResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public GetBidLandscapeByAdGroupIdsResponse getBidLandscapeByAdGroupIds(
@WebParam(name = "GetBidLandscapeByAdGroupIdsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetBidLandscapeByAdGroupIdsRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetBidLandscapeByKeywordIds", action = "GetBidLandscapeByKeywordIds")
public Response getBidLandscapeByKeywordIdsAsync(
@WebParam(name = "GetBidLandscapeByKeywordIdsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetBidLandscapeByKeywordIdsRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetBidLandscapeByKeywordIds", action = "GetBidLandscapeByKeywordIds")
public Future> getBidLandscapeByKeywordIdsAsync(
@WebParam(name = "GetBidLandscapeByKeywordIdsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetBidLandscapeByKeywordIdsRequest parameters,
@WebParam(name = "GetBidLandscapeByKeywordIdsResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.GetBidLandscapeByKeywordIdsResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "GetBidLandscapeByKeywordIds", action = "GetBidLandscapeByKeywordIds")
@WebResult(name = "GetBidLandscapeByKeywordIdsResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public GetBidLandscapeByKeywordIdsResponse getBidLandscapeByKeywordIds(
@WebParam(name = "GetBidLandscapeByKeywordIdsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetBidLandscapeByKeywordIdsRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetHistoricalKeywordPerformance", action = "GetHistoricalKeywordPerformance")
public Response getHistoricalKeywordPerformanceAsync(
@WebParam(name = "GetHistoricalKeywordPerformanceRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetHistoricalKeywordPerformanceRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetHistoricalKeywordPerformance", action = "GetHistoricalKeywordPerformance")
public Future> getHistoricalKeywordPerformanceAsync(
@WebParam(name = "GetHistoricalKeywordPerformanceRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetHistoricalKeywordPerformanceRequest parameters,
@WebParam(name = "GetHistoricalKeywordPerformanceResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.GetHistoricalKeywordPerformanceResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "GetHistoricalKeywordPerformance", action = "GetHistoricalKeywordPerformance")
@WebResult(name = "GetHistoricalKeywordPerformanceResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public GetHistoricalKeywordPerformanceResponse getHistoricalKeywordPerformance(
@WebParam(name = "GetHistoricalKeywordPerformanceRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetHistoricalKeywordPerformanceRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetHistoricalSearchCount", action = "GetHistoricalSearchCount")
public Response getHistoricalSearchCountAsync(
@WebParam(name = "GetHistoricalSearchCountRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetHistoricalSearchCountRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetHistoricalSearchCount", action = "GetHistoricalSearchCount")
public Future> getHistoricalSearchCountAsync(
@WebParam(name = "GetHistoricalSearchCountRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetHistoricalSearchCountRequest parameters,
@WebParam(name = "GetHistoricalSearchCountResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.GetHistoricalSearchCountResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "GetHistoricalSearchCount", action = "GetHistoricalSearchCount")
@WebResult(name = "GetHistoricalSearchCountResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public GetHistoricalSearchCountResponse getHistoricalSearchCount(
@WebParam(name = "GetHistoricalSearchCountRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetHistoricalSearchCountRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetKeywordCategories", action = "GetKeywordCategories")
public Response getKeywordCategoriesAsync(
@WebParam(name = "GetKeywordCategoriesRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetKeywordCategoriesRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetKeywordCategories", action = "GetKeywordCategories")
public Future> getKeywordCategoriesAsync(
@WebParam(name = "GetKeywordCategoriesRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetKeywordCategoriesRequest parameters,
@WebParam(name = "GetKeywordCategoriesResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.GetKeywordCategoriesResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "GetKeywordCategories", action = "GetKeywordCategories")
@WebResult(name = "GetKeywordCategoriesResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public GetKeywordCategoriesResponse getKeywordCategories(
@WebParam(name = "GetKeywordCategoriesRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetKeywordCategoriesRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetKeywordDemographics", action = "GetKeywordDemographics")
public Response getKeywordDemographicsAsync(
@WebParam(name = "GetKeywordDemographicsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetKeywordDemographicsRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetKeywordDemographics", action = "GetKeywordDemographics")
public Future> getKeywordDemographicsAsync(
@WebParam(name = "GetKeywordDemographicsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetKeywordDemographicsRequest parameters,
@WebParam(name = "GetKeywordDemographicsResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.GetKeywordDemographicsResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "GetKeywordDemographics", action = "GetKeywordDemographics")
@WebResult(name = "GetKeywordDemographicsResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public GetKeywordDemographicsResponse getKeywordDemographics(
@WebParam(name = "GetKeywordDemographicsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetKeywordDemographicsRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetKeywordLocations", action = "GetKeywordLocations")
public Response getKeywordLocationsAsync(
@WebParam(name = "GetKeywordLocationsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetKeywordLocationsRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetKeywordLocations", action = "GetKeywordLocations")
public Future> getKeywordLocationsAsync(
@WebParam(name = "GetKeywordLocationsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetKeywordLocationsRequest parameters,
@WebParam(name = "GetKeywordLocationsResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.GetKeywordLocationsResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "GetKeywordLocations", action = "GetKeywordLocations")
@WebResult(name = "GetKeywordLocationsResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public GetKeywordLocationsResponse getKeywordLocations(
@WebParam(name = "GetKeywordLocationsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetKeywordLocationsRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "SuggestKeywordsForUrl", action = "SuggestKeywordsForUrl")
public Response suggestKeywordsForUrlAsync(
@WebParam(name = "SuggestKeywordsForUrlRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
SuggestKeywordsForUrlRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "SuggestKeywordsForUrl", action = "SuggestKeywordsForUrl")
public Future> suggestKeywordsForUrlAsync(
@WebParam(name = "SuggestKeywordsForUrlRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
SuggestKeywordsForUrlRequest parameters,
@WebParam(name = "SuggestKeywordsForUrlResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.SuggestKeywordsForUrlResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "SuggestKeywordsForUrl", action = "SuggestKeywordsForUrl")
@WebResult(name = "SuggestKeywordsForUrlResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public SuggestKeywordsForUrlResponse suggestKeywordsForUrl(
@WebParam(name = "SuggestKeywordsForUrlRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
SuggestKeywordsForUrlRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "SuggestKeywordsFromExistingKeywords", action = "SuggestKeywordsFromExistingKeywords")
public Response suggestKeywordsFromExistingKeywordsAsync(
@WebParam(name = "SuggestKeywordsFromExistingKeywordsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
SuggestKeywordsFromExistingKeywordsRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "SuggestKeywordsFromExistingKeywords", action = "SuggestKeywordsFromExistingKeywords")
public Future> suggestKeywordsFromExistingKeywordsAsync(
@WebParam(name = "SuggestKeywordsFromExistingKeywordsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
SuggestKeywordsFromExistingKeywordsRequest parameters,
@WebParam(name = "SuggestKeywordsFromExistingKeywordsResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.SuggestKeywordsFromExistingKeywordsResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "SuggestKeywordsFromExistingKeywords", action = "SuggestKeywordsFromExistingKeywords")
@WebResult(name = "SuggestKeywordsFromExistingKeywordsResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public SuggestKeywordsFromExistingKeywordsResponse suggestKeywordsFromExistingKeywords(
@WebParam(name = "SuggestKeywordsFromExistingKeywordsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
SuggestKeywordsFromExistingKeywordsRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetAuctionInsightData", action = "GetAuctionInsightData")
public Response getAuctionInsightDataAsync(
@WebParam(name = "GetAuctionInsightDataRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetAuctionInsightDataRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetAuctionInsightData", action = "GetAuctionInsightData")
public Future> getAuctionInsightDataAsync(
@WebParam(name = "GetAuctionInsightDataRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetAuctionInsightDataRequest parameters,
@WebParam(name = "GetAuctionInsightDataResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.GetAuctionInsightDataResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "GetAuctionInsightData", action = "GetAuctionInsightData")
@WebResult(name = "GetAuctionInsightDataResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public GetAuctionInsightDataResponse getAuctionInsightData(
@WebParam(name = "GetAuctionInsightDataRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetAuctionInsightDataRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetDomainCategories", action = "GetDomainCategories")
public Response getDomainCategoriesAsync(
@WebParam(name = "GetDomainCategoriesRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetDomainCategoriesRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetDomainCategories", action = "GetDomainCategories")
public Future> getDomainCategoriesAsync(
@WebParam(name = "GetDomainCategoriesRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetDomainCategoriesRequest parameters,
@WebParam(name = "GetDomainCategoriesResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.GetDomainCategoriesResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "GetDomainCategories", action = "GetDomainCategories")
@WebResult(name = "GetDomainCategoriesResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public GetDomainCategoriesResponse getDomainCategories(
@WebParam(name = "GetDomainCategoriesRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetDomainCategoriesRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "PutMetricData", action = "PutMetricData")
public Response putMetricDataAsync(
@WebParam(name = "PutMetricDataRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
PutMetricDataRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "PutMetricData", action = "PutMetricData")
public Future> putMetricDataAsync(
@WebParam(name = "PutMetricDataRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
PutMetricDataRequest parameters,
@WebParam(name = "PutMetricDataResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.PutMetricDataResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "PutMetricData", action = "PutMetricData")
@WebResult(name = "PutMetricDataResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public PutMetricDataResponse putMetricData(
@WebParam(name = "PutMetricDataRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
PutMetricDataRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetKeywordIdeaCategories", action = "GetKeywordIdeaCategories")
public Response getKeywordIdeaCategoriesAsync(
@WebParam(name = "GetKeywordIdeaCategoriesRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetKeywordIdeaCategoriesRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetKeywordIdeaCategories", action = "GetKeywordIdeaCategories")
public Future> getKeywordIdeaCategoriesAsync(
@WebParam(name = "GetKeywordIdeaCategoriesRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetKeywordIdeaCategoriesRequest parameters,
@WebParam(name = "GetKeywordIdeaCategoriesResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.GetKeywordIdeaCategoriesResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "GetKeywordIdeaCategories", action = "GetKeywordIdeaCategories")
@WebResult(name = "GetKeywordIdeaCategoriesResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public GetKeywordIdeaCategoriesResponse getKeywordIdeaCategories(
@WebParam(name = "GetKeywordIdeaCategoriesRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetKeywordIdeaCategoriesRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetKeywordIdeas", action = "GetKeywordIdeas")
public Response getKeywordIdeasAsync(
@WebParam(name = "GetKeywordIdeasRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetKeywordIdeasRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetKeywordIdeas", action = "GetKeywordIdeas")
public Future> getKeywordIdeasAsync(
@WebParam(name = "GetKeywordIdeasRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetKeywordIdeasRequest parameters,
@WebParam(name = "GetKeywordIdeasResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.GetKeywordIdeasResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "GetKeywordIdeas", action = "GetKeywordIdeas")
@WebResult(name = "GetKeywordIdeasResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public GetKeywordIdeasResponse getKeywordIdeas(
@WebParam(name = "GetKeywordIdeasRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetKeywordIdeasRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetKeywordTrafficEstimates", action = "GetKeywordTrafficEstimates")
public Response getKeywordTrafficEstimatesAsync(
@WebParam(name = "GetKeywordTrafficEstimatesRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetKeywordTrafficEstimatesRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetKeywordTrafficEstimates", action = "GetKeywordTrafficEstimates")
public Future> getKeywordTrafficEstimatesAsync(
@WebParam(name = "GetKeywordTrafficEstimatesRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetKeywordTrafficEstimatesRequest parameters,
@WebParam(name = "GetKeywordTrafficEstimatesResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.GetKeywordTrafficEstimatesResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "GetKeywordTrafficEstimates", action = "GetKeywordTrafficEstimates")
@WebResult(name = "GetKeywordTrafficEstimatesResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public GetKeywordTrafficEstimatesResponse getKeywordTrafficEstimates(
@WebParam(name = "GetKeywordTrafficEstimatesRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetKeywordTrafficEstimatesRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetAutoApplyOptInStatus", action = "GetAutoApplyOptInStatus")
public Response getAutoApplyOptInStatusAsync(
@WebParam(name = "GetAutoApplyOptInStatusRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetAutoApplyOptInStatusRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetAutoApplyOptInStatus", action = "GetAutoApplyOptInStatus")
public Future> getAutoApplyOptInStatusAsync(
@WebParam(name = "GetAutoApplyOptInStatusRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetAutoApplyOptInStatusRequest parameters,
@WebParam(name = "GetAutoApplyOptInStatusResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.GetAutoApplyOptInStatusResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "GetAutoApplyOptInStatus", action = "GetAutoApplyOptInStatus")
@WebResult(name = "GetAutoApplyOptInStatusResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public GetAutoApplyOptInStatusResponse getAutoApplyOptInStatus(
@WebParam(name = "GetAutoApplyOptInStatusRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetAutoApplyOptInStatusRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "SetAutoApplyOptInStatus", action = "SetAutoApplyOptInStatus")
public Response setAutoApplyOptInStatusAsync(
@WebParam(name = "SetAutoApplyOptInStatusRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
SetAutoApplyOptInStatusRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "SetAutoApplyOptInStatus", action = "SetAutoApplyOptInStatus")
public Future> setAutoApplyOptInStatusAsync(
@WebParam(name = "SetAutoApplyOptInStatusRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
SetAutoApplyOptInStatusRequest parameters,
@WebParam(name = "SetAutoApplyOptInStatusResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.SetAutoApplyOptInStatusResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "SetAutoApplyOptInStatus", action = "SetAutoApplyOptInStatus")
@WebResult(name = "SetAutoApplyOptInStatusResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public SetAutoApplyOptInStatusResponse setAutoApplyOptInStatus(
@WebParam(name = "SetAutoApplyOptInStatusRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
SetAutoApplyOptInStatusRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetPerformanceInsightsDetailDataByAccountId", action = "GetPerformanceInsightsDetailDataByAccountId")
public Response getPerformanceInsightsDetailDataByAccountIdAsync(
@WebParam(name = "GetPerformanceInsightsDetailDataByAccountIdRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetPerformanceInsightsDetailDataByAccountIdRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetPerformanceInsightsDetailDataByAccountId", action = "GetPerformanceInsightsDetailDataByAccountId")
public Future> getPerformanceInsightsDetailDataByAccountIdAsync(
@WebParam(name = "GetPerformanceInsightsDetailDataByAccountIdRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetPerformanceInsightsDetailDataByAccountIdRequest parameters,
@WebParam(name = "GetPerformanceInsightsDetailDataByAccountIdResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.GetPerformanceInsightsDetailDataByAccountIdResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "GetPerformanceInsightsDetailDataByAccountId", action = "GetPerformanceInsightsDetailDataByAccountId")
@WebResult(name = "GetPerformanceInsightsDetailDataByAccountIdResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public GetPerformanceInsightsDetailDataByAccountIdResponse getPerformanceInsightsDetailDataByAccountId(
@WebParam(name = "GetPerformanceInsightsDetailDataByAccountIdRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetPerformanceInsightsDetailDataByAccountIdRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetRecommendations", action = "GetRecommendations")
public Response getRecommendationsAsync(
@WebParam(name = "GetRecommendationsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetRecommendationsRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetRecommendations", action = "GetRecommendations")
public Future> getRecommendationsAsync(
@WebParam(name = "GetRecommendationsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetRecommendationsRequest parameters,
@WebParam(name = "GetRecommendationsResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.GetRecommendationsResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "GetRecommendations", action = "GetRecommendations")
@WebResult(name = "GetRecommendationsResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public GetRecommendationsResponse getRecommendations(
@WebParam(name = "GetRecommendationsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetRecommendationsRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "TagRecommendations", action = "TagRecommendations")
public Response tagRecommendationsAsync(
@WebParam(name = "TagRecommendationsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
TagRecommendationsRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "TagRecommendations", action = "TagRecommendations")
public Future> tagRecommendationsAsync(
@WebParam(name = "TagRecommendationsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
TagRecommendationsRequest parameters,
@WebParam(name = "TagRecommendationsResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.TagRecommendationsResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "TagRecommendations", action = "TagRecommendations")
@WebResult(name = "TagRecommendationsResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public TagRecommendationsResponse tagRecommendations(
@WebParam(name = "TagRecommendationsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
TagRecommendationsRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetTextAssetSuggestionsByFinalUrls", action = "GetTextAssetSuggestionsByFinalUrls")
public Response getTextAssetSuggestionsByFinalUrlsAsync(
@WebParam(name = "GetTextAssetSuggestionsByFinalUrlsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetTextAssetSuggestionsByFinalUrlsRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetTextAssetSuggestionsByFinalUrls", action = "GetTextAssetSuggestionsByFinalUrls")
public Future> getTextAssetSuggestionsByFinalUrlsAsync(
@WebParam(name = "GetTextAssetSuggestionsByFinalUrlsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetTextAssetSuggestionsByFinalUrlsRequest parameters,
@WebParam(name = "GetTextAssetSuggestionsByFinalUrlsResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.GetTextAssetSuggestionsByFinalUrlsResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "GetTextAssetSuggestionsByFinalUrls", action = "GetTextAssetSuggestionsByFinalUrls")
@WebResult(name = "GetTextAssetSuggestionsByFinalUrlsResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public GetTextAssetSuggestionsByFinalUrlsResponse getTextAssetSuggestionsByFinalUrls(
@WebParam(name = "GetTextAssetSuggestionsByFinalUrlsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetTextAssetSuggestionsByFinalUrlsRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "ApplyRecommendations", action = "ApplyRecommendations")
public Response applyRecommendationsAsync(
@WebParam(name = "ApplyRecommendationsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
ApplyRecommendationsRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "ApplyRecommendations", action = "ApplyRecommendations")
public Future> applyRecommendationsAsync(
@WebParam(name = "ApplyRecommendationsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
ApplyRecommendationsRequest parameters,
@WebParam(name = "ApplyRecommendationsResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.ApplyRecommendationsResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "ApplyRecommendations", action = "ApplyRecommendations")
@WebResult(name = "ApplyRecommendationsResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public ApplyRecommendationsResponse applyRecommendations(
@WebParam(name = "ApplyRecommendationsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
ApplyRecommendationsRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "DismissRecommendations", action = "DismissRecommendations")
public Response dismissRecommendationsAsync(
@WebParam(name = "DismissRecommendationsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
DismissRecommendationsRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "DismissRecommendations", action = "DismissRecommendations")
public Future> dismissRecommendationsAsync(
@WebParam(name = "DismissRecommendationsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
DismissRecommendationsRequest parameters,
@WebParam(name = "DismissRecommendationsResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.DismissRecommendationsResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "DismissRecommendations", action = "DismissRecommendations")
@WebResult(name = "DismissRecommendationsResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public DismissRecommendationsResponse dismissRecommendations(
@WebParam(name = "DismissRecommendationsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
DismissRecommendationsRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "RetrieveRecommendations", action = "RetrieveRecommendations")
public Response retrieveRecommendationsAsync(
@WebParam(name = "RetrieveRecommendationsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
RetrieveRecommendationsRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "RetrieveRecommendations", action = "RetrieveRecommendations")
public Future> retrieveRecommendationsAsync(
@WebParam(name = "RetrieveRecommendationsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
RetrieveRecommendationsRequest parameters,
@WebParam(name = "RetrieveRecommendationsResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.RetrieveRecommendationsResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "RetrieveRecommendations", action = "RetrieveRecommendations")
@WebResult(name = "RetrieveRecommendationsResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public RetrieveRecommendationsResponse retrieveRecommendations(
@WebParam(name = "RetrieveRecommendationsRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
RetrieveRecommendationsRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetAudienceFullEstimation", action = "GetAudienceFullEstimation")
public Response getAudienceFullEstimationAsync(
@WebParam(name = "GetAudienceFullEstimationRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetAudienceFullEstimationRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetAudienceFullEstimation", action = "GetAudienceFullEstimation")
public Future> getAudienceFullEstimationAsync(
@WebParam(name = "GetAudienceFullEstimationRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetAudienceFullEstimationRequest parameters,
@WebParam(name = "GetAudienceFullEstimationResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.adinsight.GetAudienceFullEstimationResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFaultDetail_Exception
*/
@WebMethod(operationName = "GetAudienceFullEstimation", action = "GetAudienceFullEstimation")
@WebResult(name = "GetAudienceFullEstimationResponse", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
public GetAudienceFullEstimationResponse getAudienceFullEstimation(
@WebParam(name = "GetAudienceFullEstimationRequest", targetNamespace = "https://bingads.microsoft.com/AdInsight/v13", partName = "parameters")
GetAudienceFullEstimationRequest parameters)
throws AdApiFaultDetail_Exception, ApiFaultDetail_Exception
;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy