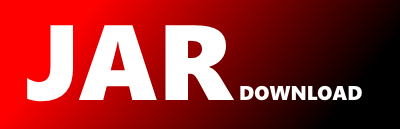
com.microsoft.bingads.v13.campaignmanagement.ConversionGoal Maven / Gradle / Ivy
package com.microsoft.bingads.v13.campaignmanagement;
import java.util.Collection;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlSeeAlso;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
/**
* Java class for ConversionGoal complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
{@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "ConversionGoal", propOrder = {
"attributionModelType",
"conversionWindowInMinutes",
"countType",
"excludeFromBidding",
"goalCategory",
"id",
"isEnhancedConversionsEnabled",
"name",
"revenue",
"scope",
"status",
"tagId",
"trackingStatus",
"type",
"viewThroughConversionWindowInMinutes"
})
@XmlSeeAlso({
UrlGoal.class,
DurationGoal.class,
PagesViewedPerVisitGoal.class,
EventGoal.class,
AppInstallGoal.class,
OfflineConversionGoal.class,
InStoreTransactionGoal.class
})
public class ConversionGoal {
@XmlElement(name = "AttributionModelType", nillable = true)
@XmlSchemaType(name = "string")
protected AttributionModelType attributionModelType;
@XmlElement(name = "ConversionWindowInMinutes", nillable = true)
protected Integer conversionWindowInMinutes;
@XmlElement(name = "CountType", nillable = true)
@XmlSchemaType(name = "string")
protected ConversionGoalCountType countType;
@XmlElement(name = "ExcludeFromBidding", nillable = true)
protected Boolean excludeFromBidding;
@XmlElement(name = "GoalCategory", nillable = true)
@XmlSchemaType(name = "string")
protected ConversionGoalCategory goalCategory;
@XmlElement(name = "Id", nillable = true)
protected Long id;
@XmlElement(name = "IsEnhancedConversionsEnabled", nillable = true)
protected Boolean isEnhancedConversionsEnabled;
@XmlElement(name = "Name", nillable = true)
protected String name;
@XmlElement(name = "Revenue", nillable = true)
protected ConversionGoalRevenue revenue;
@XmlElement(name = "Scope", nillable = true)
@XmlSchemaType(name = "string")
protected EntityScope scope;
@XmlElement(name = "Status", nillable = true)
@XmlSchemaType(name = "string")
protected ConversionGoalStatus status;
@XmlElement(name = "TagId", nillable = true)
protected Long tagId;
@XmlElement(name = "TrackingStatus", nillable = true)
@XmlSchemaType(name = "string")
protected ConversionGoalTrackingStatus trackingStatus;
@XmlElement(name = "Type", type = String.class, nillable = true)
@XmlJavaTypeAdapter(Adapter22 .class)
protected Collection type;
@XmlElement(name = "ViewThroughConversionWindowInMinutes", nillable = true)
protected Integer viewThroughConversionWindowInMinutes;
/**
* Gets the value of the attributionModelType property.
*
* @return
* possible object is
* {@link AttributionModelType }
*
*/
public AttributionModelType getAttributionModelType() {
return attributionModelType;
}
/**
* Sets the value of the attributionModelType property.
*
* @param value
* allowed object is
* {@link AttributionModelType }
*
*/
public void setAttributionModelType(AttributionModelType value) {
this.attributionModelType = value;
}
/**
* Gets the value of the conversionWindowInMinutes property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getConversionWindowInMinutes() {
return conversionWindowInMinutes;
}
/**
* Sets the value of the conversionWindowInMinutes property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setConversionWindowInMinutes(Integer value) {
this.conversionWindowInMinutes = value;
}
/**
* Gets the value of the countType property.
*
* @return
* possible object is
* {@link ConversionGoalCountType }
*
*/
public ConversionGoalCountType getCountType() {
return countType;
}
/**
* Sets the value of the countType property.
*
* @param value
* allowed object is
* {@link ConversionGoalCountType }
*
*/
public void setCountType(ConversionGoalCountType value) {
this.countType = value;
}
/**
* Gets the value of the excludeFromBidding property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean getExcludeFromBidding() {
return excludeFromBidding;
}
/**
* Sets the value of the excludeFromBidding property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setExcludeFromBidding(Boolean value) {
this.excludeFromBidding = value;
}
/**
* Gets the value of the goalCategory property.
*
* @return
* possible object is
* {@link ConversionGoalCategory }
*
*/
public ConversionGoalCategory getGoalCategory() {
return goalCategory;
}
/**
* Sets the value of the goalCategory property.
*
* @param value
* allowed object is
* {@link ConversionGoalCategory }
*
*/
public void setGoalCategory(ConversionGoalCategory value) {
this.goalCategory = value;
}
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link Long }
*
*/
public Long getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setId(Long value) {
this.id = value;
}
/**
* Gets the value of the isEnhancedConversionsEnabled property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean getIsEnhancedConversionsEnabled() {
return isEnhancedConversionsEnabled;
}
/**
* Sets the value of the isEnhancedConversionsEnabled property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setIsEnhancedConversionsEnabled(Boolean value) {
this.isEnhancedConversionsEnabled = value;
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
this.name = value;
}
/**
* Gets the value of the revenue property.
*
* @return
* possible object is
* {@link ConversionGoalRevenue }
*
*/
public ConversionGoalRevenue getRevenue() {
return revenue;
}
/**
* Sets the value of the revenue property.
*
* @param value
* allowed object is
* {@link ConversionGoalRevenue }
*
*/
public void setRevenue(ConversionGoalRevenue value) {
this.revenue = value;
}
/**
* Gets the value of the scope property.
*
* @return
* possible object is
* {@link EntityScope }
*
*/
public EntityScope getScope() {
return scope;
}
/**
* Sets the value of the scope property.
*
* @param value
* allowed object is
* {@link EntityScope }
*
*/
public void setScope(EntityScope value) {
this.scope = value;
}
/**
* Gets the value of the status property.
*
* @return
* possible object is
* {@link ConversionGoalStatus }
*
*/
public ConversionGoalStatus getStatus() {
return status;
}
/**
* Sets the value of the status property.
*
* @param value
* allowed object is
* {@link ConversionGoalStatus }
*
*/
public void setStatus(ConversionGoalStatus value) {
this.status = value;
}
/**
* Gets the value of the tagId property.
*
* @return
* possible object is
* {@link Long }
*
*/
public Long getTagId() {
return tagId;
}
/**
* Sets the value of the tagId property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setTagId(Long value) {
this.tagId = value;
}
/**
* Gets the value of the trackingStatus property.
*
* @return
* possible object is
* {@link ConversionGoalTrackingStatus }
*
*/
public ConversionGoalTrackingStatus getTrackingStatus() {
return trackingStatus;
}
/**
* Sets the value of the trackingStatus property.
*
* @param value
* allowed object is
* {@link ConversionGoalTrackingStatus }
*
*/
public void setTrackingStatus(ConversionGoalTrackingStatus value) {
this.trackingStatus = value;
}
/**
* Gets the value of the type property.
*
* @return
* possible object is
* {@link String }
*
*/
public Collection getType() {
return type;
}
/**
* Sets the value of the type property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setType(Collection value) {
}
/**
* Gets the value of the viewThroughConversionWindowInMinutes property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getViewThroughConversionWindowInMinutes() {
return viewThroughConversionWindowInMinutes;
}
/**
* Sets the value of the viewThroughConversionWindowInMinutes property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setViewThroughConversionWindowInMinutes(Integer value) {
this.viewThroughConversionWindowInMinutes = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy