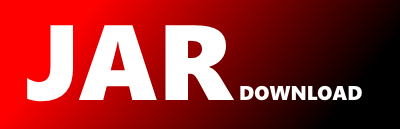
com.microsoft.bingads.v13.campaignmanagement.ImportResult Maven / Gradle / Ivy
package com.microsoft.bingads.v13.campaignmanagement;
import java.util.Calendar;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
/**
* Java class for ImportResult complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
{@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "ImportResult", propOrder = {
"entityStatistics",
"errorLogUrl",
"forwardCompatibilityMap",
"id",
"importJob",
"startTimeInUTC",
"status"
})
public class ImportResult {
@XmlElement(name = "EntityStatistics", nillable = true)
protected ArrayOfImportEntityStatistics entityStatistics;
@XmlElement(name = "ErrorLogUrl", nillable = true)
protected String errorLogUrl;
@XmlElement(name = "ForwardCompatibilityMap", nillable = true)
protected ArrayOfKeyValuePairOfstringstring forwardCompatibilityMap;
@XmlElement(name = "Id", nillable = true)
protected String id;
@XmlElement(name = "ImportJob", nillable = true)
protected ImportJob importJob;
@XmlElement(name = "StartTimeInUTC", type = String.class)
@XmlJavaTypeAdapter(Adapter1 .class)
@XmlSchemaType(name = "dateTime")
protected Calendar startTimeInUTC;
@XmlElement(name = "Status", nillable = true)
protected String status;
/**
* Gets the value of the entityStatistics property.
*
* @return
* possible object is
* {@link ArrayOfImportEntityStatistics }
*
*/
public ArrayOfImportEntityStatistics getEntityStatistics() {
return entityStatistics;
}
/**
* Sets the value of the entityStatistics property.
*
* @param value
* allowed object is
* {@link ArrayOfImportEntityStatistics }
*
*/
public void setEntityStatistics(ArrayOfImportEntityStatistics value) {
this.entityStatistics = value;
}
/**
* Gets the value of the errorLogUrl property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getErrorLogUrl() {
return errorLogUrl;
}
/**
* Sets the value of the errorLogUrl property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setErrorLogUrl(String value) {
this.errorLogUrl = value;
}
/**
* Gets the value of the forwardCompatibilityMap property.
*
* @return
* possible object is
* {@link ArrayOfKeyValuePairOfstringstring }
*
*/
public ArrayOfKeyValuePairOfstringstring getForwardCompatibilityMap() {
return forwardCompatibilityMap;
}
/**
* Sets the value of the forwardCompatibilityMap property.
*
* @param value
* allowed object is
* {@link ArrayOfKeyValuePairOfstringstring }
*
*/
public void setForwardCompatibilityMap(ArrayOfKeyValuePairOfstringstring value) {
this.forwardCompatibilityMap = value;
}
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setId(String value) {
this.id = value;
}
/**
* Gets the value of the importJob property.
*
* @return
* possible object is
* {@link ImportJob }
*
*/
public ImportJob getImportJob() {
return importJob;
}
/**
* Sets the value of the importJob property.
*
* @param value
* allowed object is
* {@link ImportJob }
*
*/
public void setImportJob(ImportJob value) {
this.importJob = value;
}
/**
* Gets the value of the startTimeInUTC property.
*
* @return
* possible object is
* {@link String }
*
*/
public Calendar getStartTimeInUTC() {
return startTimeInUTC;
}
/**
* Sets the value of the startTimeInUTC property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setStartTimeInUTC(Calendar value) {
this.startTimeInUTC = value;
}
/**
* Gets the value of the status property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getStatus() {
return status;
}
/**
* Sets the value of the status property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setStatus(String value) {
this.status = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy