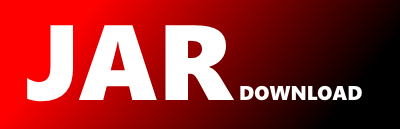
com.microsoft.bingads.v13.campaignmanagement.LocationAdExtension Maven / Gradle / Ivy
package com.microsoft.bingads.v13.campaignmanagement;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
/**
* Java class for LocationAdExtension complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
{@code
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "LocationAdExtension", propOrder = {
"address",
"companyName",
"geoCodeStatus",
"geoPoint",
"phoneNumber"
})
public class LocationAdExtension
extends AdExtension
{
public LocationAdExtension() {
this.type = "LocationAdExtension";
}
@XmlElement(name = "Address", nillable = true)
protected Address address;
@XmlElement(name = "CompanyName", nillable = true)
protected String companyName;
@XmlElement(name = "GeoCodeStatus", nillable = true)
@XmlSchemaType(name = "string")
protected BusinessGeoCodeStatus geoCodeStatus;
@XmlElement(name = "GeoPoint", nillable = true)
protected GeoPoint geoPoint;
@XmlElement(name = "PhoneNumber", nillable = true)
protected String phoneNumber;
/**
* Gets the value of the address property.
*
* @return
* possible object is
* {@link Address }
*
*/
public Address getAddress() {
return address;
}
/**
* Sets the value of the address property.
*
* @param value
* allowed object is
* {@link Address }
*
*/
public void setAddress(Address value) {
this.address = value;
}
/**
* Gets the value of the companyName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCompanyName() {
return companyName;
}
/**
* Sets the value of the companyName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCompanyName(String value) {
this.companyName = value;
}
/**
* Gets the value of the geoCodeStatus property.
*
* @return
* possible object is
* {@link BusinessGeoCodeStatus }
*
*/
public BusinessGeoCodeStatus getGeoCodeStatus() {
return geoCodeStatus;
}
/**
* Sets the value of the geoCodeStatus property.
*
* @param value
* allowed object is
* {@link BusinessGeoCodeStatus }
*
*/
public void setGeoCodeStatus(BusinessGeoCodeStatus value) {
this.geoCodeStatus = value;
}
/**
* Gets the value of the geoPoint property.
*
* @return
* possible object is
* {@link GeoPoint }
*
*/
public GeoPoint getGeoPoint() {
return geoPoint;
}
/**
* Sets the value of the geoPoint property.
*
* @param value
* allowed object is
* {@link GeoPoint }
*
*/
public void setGeoPoint(GeoPoint value) {
this.geoPoint = value;
}
/**
* Gets the value of the phoneNumber property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPhoneNumber() {
return phoneNumber;
}
/**
* Sets the value of the phoneNumber property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPhoneNumber(String value) {
this.phoneNumber = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy