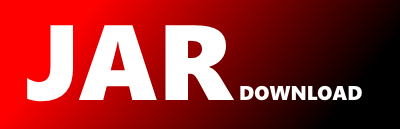
com.microsoft.bingads.v13.campaignmanagement.PriceAdExtension Maven / Gradle / Ivy
package com.microsoft.bingads.v13.campaignmanagement;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
/**
* Java class for PriceAdExtension complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
{@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "PriceAdExtension", propOrder = {
"finalUrlSuffix",
"language",
"priceExtensionType",
"tableRows",
"trackingUrlTemplate",
"urlCustomParameters"
})
public class PriceAdExtension
extends AdExtension
{
public PriceAdExtension() {
this.type = "PriceAdExtension";
}
@XmlElement(name = "FinalUrlSuffix", nillable = true)
protected String finalUrlSuffix;
@XmlElement(name = "Language", nillable = true)
protected String language;
@XmlElement(name = "PriceExtensionType", required = true)
@XmlSchemaType(name = "string")
protected PriceExtensionType priceExtensionType;
@XmlElement(name = "TableRows", nillable = true)
protected ArrayOfPriceTableRow tableRows;
@XmlElement(name = "TrackingUrlTemplate", nillable = true)
protected String trackingUrlTemplate;
@XmlElement(name = "UrlCustomParameters", nillable = true)
protected CustomParameters urlCustomParameters;
/**
* Gets the value of the finalUrlSuffix property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFinalUrlSuffix() {
return finalUrlSuffix;
}
/**
* Sets the value of the finalUrlSuffix property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFinalUrlSuffix(String value) {
this.finalUrlSuffix = value;
}
/**
* Gets the value of the language property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLanguage() {
return language;
}
/**
* Sets the value of the language property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLanguage(String value) {
this.language = value;
}
/**
* Gets the value of the priceExtensionType property.
*
* @return
* possible object is
* {@link PriceExtensionType }
*
*/
public PriceExtensionType getPriceExtensionType() {
return priceExtensionType;
}
/**
* Sets the value of the priceExtensionType property.
*
* @param value
* allowed object is
* {@link PriceExtensionType }
*
*/
public void setPriceExtensionType(PriceExtensionType value) {
this.priceExtensionType = value;
}
/**
* Gets the value of the tableRows property.
*
* @return
* possible object is
* {@link ArrayOfPriceTableRow }
*
*/
public ArrayOfPriceTableRow getTableRows() {
return tableRows;
}
/**
* Sets the value of the tableRows property.
*
* @param value
* allowed object is
* {@link ArrayOfPriceTableRow }
*
*/
public void setTableRows(ArrayOfPriceTableRow value) {
this.tableRows = value;
}
/**
* Gets the value of the trackingUrlTemplate property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTrackingUrlTemplate() {
return trackingUrlTemplate;
}
/**
* Sets the value of the trackingUrlTemplate property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTrackingUrlTemplate(String value) {
this.trackingUrlTemplate = value;
}
/**
* Gets the value of the urlCustomParameters property.
*
* @return
* possible object is
* {@link CustomParameters }
*
*/
public CustomParameters getUrlCustomParameters() {
return urlCustomParameters;
}
/**
* Sets the value of the urlCustomParameters property.
*
* @param value
* allowed object is
* {@link CustomParameters }
*
*/
public void setUrlCustomParameters(CustomParameters value) {
this.urlCustomParameters = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy