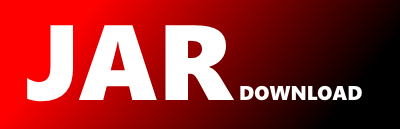
com.microsoft.bingads.v13.customermanagement.ICustomerManagementService Maven / Gradle / Ivy
package com.microsoft.bingads.v13.customermanagement;
import java.util.concurrent.Future;
import jakarta.jws.WebMethod;
import jakarta.jws.WebParam;
import jakarta.jws.WebResult;
import jakarta.jws.WebService;
import jakarta.jws.soap.SOAPBinding;
import jakarta.xml.bind.annotation.XmlSeeAlso;
import jakarta.xml.ws.AsyncHandler;
import jakarta.xml.ws.Response;
/**
* This class was generated by the XML-WS Tools.
* XML-WS Tools 4.0.1
* Generated source version: 3.0
*
*/
@WebService(name = "ICustomerManagementService", targetNamespace = "https://bingads.microsoft.com/Customer/v13")
@SOAPBinding(parameterStyle = SOAPBinding.ParameterStyle.BARE)
@XmlSeeAlso({
ObjectFactory.class
})
public interface ICustomerManagementService {
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetAccountsInfo", action = "GetAccountsInfo")
public Response getAccountsInfoAsync(
@WebParam(name = "GetAccountsInfoRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetAccountsInfoRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetAccountsInfo", action = "GetAccountsInfo")
public Future> getAccountsInfoAsync(
@WebParam(name = "GetAccountsInfoRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetAccountsInfoRequest parameters,
@WebParam(name = "GetAccountsInfoResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.GetAccountsInfoResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "GetAccountsInfo", action = "GetAccountsInfo")
@WebResult(name = "GetAccountsInfoResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public GetAccountsInfoResponse getAccountsInfo(
@WebParam(name = "GetAccountsInfoRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetAccountsInfoRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "FindAccounts", action = "FindAccounts")
public Response findAccountsAsync(
@WebParam(name = "FindAccountsRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
FindAccountsRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "FindAccounts", action = "FindAccounts")
public Future> findAccountsAsync(
@WebParam(name = "FindAccountsRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
FindAccountsRequest parameters,
@WebParam(name = "FindAccountsResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.FindAccountsResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "FindAccounts", action = "FindAccounts")
@WebResult(name = "FindAccountsResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public FindAccountsResponse findAccounts(
@WebParam(name = "FindAccountsRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
FindAccountsRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "AddAccount", action = "AddAccount")
public Response addAccountAsync(
@WebParam(name = "AddAccountRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
AddAccountRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "AddAccount", action = "AddAccount")
public Future> addAccountAsync(
@WebParam(name = "AddAccountRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
AddAccountRequest parameters,
@WebParam(name = "AddAccountResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.AddAccountResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "AddAccount", action = "AddAccount")
@WebResult(name = "AddAccountResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public AddAccountResponse addAccount(
@WebParam(name = "AddAccountRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
AddAccountRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "UpdateAccount", action = "UpdateAccount")
public Response updateAccountAsync(
@WebParam(name = "UpdateAccountRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
UpdateAccountRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "UpdateAccount", action = "UpdateAccount")
public Future> updateAccountAsync(
@WebParam(name = "UpdateAccountRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
UpdateAccountRequest parameters,
@WebParam(name = "UpdateAccountResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.UpdateAccountResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "UpdateAccount", action = "UpdateAccount")
@WebResult(name = "UpdateAccountResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public UpdateAccountResponse updateAccount(
@WebParam(name = "UpdateAccountRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
UpdateAccountRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetCustomer", action = "GetCustomer")
public Response getCustomerAsync(
@WebParam(name = "GetCustomerRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetCustomerRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetCustomer", action = "GetCustomer")
public Future> getCustomerAsync(
@WebParam(name = "GetCustomerRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetCustomerRequest parameters,
@WebParam(name = "GetCustomerResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.GetCustomerResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "GetCustomer", action = "GetCustomer")
@WebResult(name = "GetCustomerResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public GetCustomerResponse getCustomer(
@WebParam(name = "GetCustomerRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetCustomerRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "UpdateCustomer", action = "UpdateCustomer")
public Response updateCustomerAsync(
@WebParam(name = "UpdateCustomerRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
UpdateCustomerRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "UpdateCustomer", action = "UpdateCustomer")
public Future> updateCustomerAsync(
@WebParam(name = "UpdateCustomerRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
UpdateCustomerRequest parameters,
@WebParam(name = "UpdateCustomerResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.UpdateCustomerResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "UpdateCustomer", action = "UpdateCustomer")
@WebResult(name = "UpdateCustomerResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public UpdateCustomerResponse updateCustomer(
@WebParam(name = "UpdateCustomerRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
UpdateCustomerRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "SignupCustomer", action = "SignupCustomer")
public Response signupCustomerAsync(
@WebParam(name = "SignupCustomerRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
SignupCustomerRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "SignupCustomer", action = "SignupCustomer")
public Future> signupCustomerAsync(
@WebParam(name = "SignupCustomerRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
SignupCustomerRequest parameters,
@WebParam(name = "SignupCustomerResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.SignupCustomerResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "SignupCustomer", action = "SignupCustomer")
@WebResult(name = "SignupCustomerResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public SignupCustomerResponse signupCustomer(
@WebParam(name = "SignupCustomerRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
SignupCustomerRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetAccount", action = "GetAccount")
public Response getAccountAsync(
@WebParam(name = "GetAccountRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetAccountRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetAccount", action = "GetAccount")
public Future> getAccountAsync(
@WebParam(name = "GetAccountRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetAccountRequest parameters,
@WebParam(name = "GetAccountResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.GetAccountResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "GetAccount", action = "GetAccount")
@WebResult(name = "GetAccountResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public GetAccountResponse getAccount(
@WebParam(name = "GetAccountRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetAccountRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetCustomersInfo", action = "GetCustomersInfo")
public Response getCustomersInfoAsync(
@WebParam(name = "GetCustomersInfoRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetCustomersInfoRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetCustomersInfo", action = "GetCustomersInfo")
public Future> getCustomersInfoAsync(
@WebParam(name = "GetCustomersInfoRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetCustomersInfoRequest parameters,
@WebParam(name = "GetCustomersInfoResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.GetCustomersInfoResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "GetCustomersInfo", action = "GetCustomersInfo")
@WebResult(name = "GetCustomersInfoResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public GetCustomersInfoResponse getCustomersInfo(
@WebParam(name = "GetCustomersInfoRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetCustomersInfoRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "DeleteAccount", action = "DeleteAccount")
public Response deleteAccountAsync(
@WebParam(name = "DeleteAccountRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
DeleteAccountRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "DeleteAccount", action = "DeleteAccount")
public Future> deleteAccountAsync(
@WebParam(name = "DeleteAccountRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
DeleteAccountRequest parameters,
@WebParam(name = "DeleteAccountResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.DeleteAccountResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "DeleteAccount", action = "DeleteAccount")
@WebResult(name = "DeleteAccountResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public DeleteAccountResponse deleteAccount(
@WebParam(name = "DeleteAccountRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
DeleteAccountRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "DeleteCustomer", action = "DeleteCustomer")
public Response deleteCustomerAsync(
@WebParam(name = "DeleteCustomerRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
DeleteCustomerRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "DeleteCustomer", action = "DeleteCustomer")
public Future> deleteCustomerAsync(
@WebParam(name = "DeleteCustomerRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
DeleteCustomerRequest parameters,
@WebParam(name = "DeleteCustomerResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.DeleteCustomerResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "DeleteCustomer", action = "DeleteCustomer")
@WebResult(name = "DeleteCustomerResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public DeleteCustomerResponse deleteCustomer(
@WebParam(name = "DeleteCustomerRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
DeleteCustomerRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "UpdateUser", action = "UpdateUser")
public Response updateUserAsync(
@WebParam(name = "UpdateUserRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
UpdateUserRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "UpdateUser", action = "UpdateUser")
public Future> updateUserAsync(
@WebParam(name = "UpdateUserRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
UpdateUserRequest parameters,
@WebParam(name = "UpdateUserResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.UpdateUserResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "UpdateUser", action = "UpdateUser")
@WebResult(name = "UpdateUserResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public UpdateUserResponse updateUser(
@WebParam(name = "UpdateUserRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
UpdateUserRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "UpdateUserRoles", action = "UpdateUserRoles")
public Response updateUserRolesAsync(
@WebParam(name = "UpdateUserRolesRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
UpdateUserRolesRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "UpdateUserRoles", action = "UpdateUserRoles")
public Future> updateUserRolesAsync(
@WebParam(name = "UpdateUserRolesRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
UpdateUserRolesRequest parameters,
@WebParam(name = "UpdateUserRolesResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.UpdateUserRolesResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "UpdateUserRoles", action = "UpdateUserRoles")
@WebResult(name = "UpdateUserRolesResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public UpdateUserRolesResponse updateUserRoles(
@WebParam(name = "UpdateUserRolesRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
UpdateUserRolesRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetUser", action = "GetUser")
public Response getUserAsync(
@WebParam(name = "GetUserRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetUserRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetUser", action = "GetUser")
public Future> getUserAsync(
@WebParam(name = "GetUserRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetUserRequest parameters,
@WebParam(name = "GetUserResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.GetUserResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "GetUser", action = "GetUser")
@WebResult(name = "GetUserResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public GetUserResponse getUser(
@WebParam(name = "GetUserRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetUserRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetCurrentUser", action = "GetCurrentUser")
public Response getCurrentUserAsync(
@WebParam(name = "GetCurrentUserRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetCurrentUserRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetCurrentUser", action = "GetCurrentUser")
public Future> getCurrentUserAsync(
@WebParam(name = "GetCurrentUserRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetCurrentUserRequest parameters,
@WebParam(name = "GetCurrentUserResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.GetCurrentUserResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "GetCurrentUser", action = "GetCurrentUser")
@WebResult(name = "GetCurrentUserResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public GetCurrentUserResponse getCurrentUser(
@WebParam(name = "GetCurrentUserRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetCurrentUserRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "DeleteUser", action = "DeleteUser")
public Response deleteUserAsync(
@WebParam(name = "DeleteUserRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
DeleteUserRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "DeleteUser", action = "DeleteUser")
public Future> deleteUserAsync(
@WebParam(name = "DeleteUserRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
DeleteUserRequest parameters,
@WebParam(name = "DeleteUserResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.DeleteUserResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "DeleteUser", action = "DeleteUser")
@WebResult(name = "DeleteUserResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public DeleteUserResponse deleteUser(
@WebParam(name = "DeleteUserRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
DeleteUserRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetUsersInfo", action = "GetUsersInfo")
public Response getUsersInfoAsync(
@WebParam(name = "GetUsersInfoRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetUsersInfoRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetUsersInfo", action = "GetUsersInfo")
public Future> getUsersInfoAsync(
@WebParam(name = "GetUsersInfoRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetUsersInfoRequest parameters,
@WebParam(name = "GetUsersInfoResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.GetUsersInfoResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "GetUsersInfo", action = "GetUsersInfo")
@WebResult(name = "GetUsersInfoResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public GetUsersInfoResponse getUsersInfo(
@WebParam(name = "GetUsersInfoRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetUsersInfoRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetCustomerPilotFeatures", action = "GetCustomerPilotFeatures")
public Response getCustomerPilotFeaturesAsync(
@WebParam(name = "GetCustomerPilotFeaturesRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetCustomerPilotFeaturesRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetCustomerPilotFeatures", action = "GetCustomerPilotFeatures")
public Future> getCustomerPilotFeaturesAsync(
@WebParam(name = "GetCustomerPilotFeaturesRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetCustomerPilotFeaturesRequest parameters,
@WebParam(name = "GetCustomerPilotFeaturesResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.GetCustomerPilotFeaturesResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "GetCustomerPilotFeatures", action = "GetCustomerPilotFeatures")
@WebResult(name = "GetCustomerPilotFeaturesResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public GetCustomerPilotFeaturesResponse getCustomerPilotFeatures(
@WebParam(name = "GetCustomerPilotFeaturesRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetCustomerPilotFeaturesRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetAccountPilotFeatures", action = "GetAccountPilotFeatures")
public Response getAccountPilotFeaturesAsync(
@WebParam(name = "GetAccountPilotFeaturesRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetAccountPilotFeaturesRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetAccountPilotFeatures", action = "GetAccountPilotFeatures")
public Future> getAccountPilotFeaturesAsync(
@WebParam(name = "GetAccountPilotFeaturesRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetAccountPilotFeaturesRequest parameters,
@WebParam(name = "GetAccountPilotFeaturesResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.GetAccountPilotFeaturesResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "GetAccountPilotFeatures", action = "GetAccountPilotFeatures")
@WebResult(name = "GetAccountPilotFeaturesResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public GetAccountPilotFeaturesResponse getAccountPilotFeatures(
@WebParam(name = "GetAccountPilotFeaturesRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetAccountPilotFeaturesRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetPilotFeaturesCountries", action = "GetPilotFeaturesCountries")
public Response getPilotFeaturesCountriesAsync(
@WebParam(name = "GetPilotFeaturesCountriesRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetPilotFeaturesCountriesRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetPilotFeaturesCountries", action = "GetPilotFeaturesCountries")
public Future> getPilotFeaturesCountriesAsync(
@WebParam(name = "GetPilotFeaturesCountriesRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetPilotFeaturesCountriesRequest parameters,
@WebParam(name = "GetPilotFeaturesCountriesResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.GetPilotFeaturesCountriesResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "GetPilotFeaturesCountries", action = "GetPilotFeaturesCountries")
@WebResult(name = "GetPilotFeaturesCountriesResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public GetPilotFeaturesCountriesResponse getPilotFeaturesCountries(
@WebParam(name = "GetPilotFeaturesCountriesRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetPilotFeaturesCountriesRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetAccessibleCustomer", action = "GetAccessibleCustomer")
public Response getAccessibleCustomerAsync(
@WebParam(name = "GetAccessibleCustomerRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetAccessibleCustomerRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetAccessibleCustomer", action = "GetAccessibleCustomer")
public Future> getAccessibleCustomerAsync(
@WebParam(name = "GetAccessibleCustomerRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetAccessibleCustomerRequest parameters,
@WebParam(name = "GetAccessibleCustomerResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.GetAccessibleCustomerResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "GetAccessibleCustomer", action = "GetAccessibleCustomer")
@WebResult(name = "GetAccessibleCustomerResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public GetAccessibleCustomerResponse getAccessibleCustomer(
@WebParam(name = "GetAccessibleCustomerRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetAccessibleCustomerRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "FindAccountsOrCustomersInfo", action = "FindAccountsOrCustomersInfo")
public Response findAccountsOrCustomersInfoAsync(
@WebParam(name = "FindAccountsOrCustomersInfoRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
FindAccountsOrCustomersInfoRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "FindAccountsOrCustomersInfo", action = "FindAccountsOrCustomersInfo")
public Future> findAccountsOrCustomersInfoAsync(
@WebParam(name = "FindAccountsOrCustomersInfoRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
FindAccountsOrCustomersInfoRequest parameters,
@WebParam(name = "FindAccountsOrCustomersInfoResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.FindAccountsOrCustomersInfoResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "FindAccountsOrCustomersInfo", action = "FindAccountsOrCustomersInfo")
@WebResult(name = "FindAccountsOrCustomersInfoResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public FindAccountsOrCustomersInfoResponse findAccountsOrCustomersInfo(
@WebParam(name = "FindAccountsOrCustomersInfoRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
FindAccountsOrCustomersInfoRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "UpgradeCustomerToAgency", action = "UpgradeCustomerToAgency")
public Response upgradeCustomerToAgencyAsync(
@WebParam(name = "UpgradeCustomerToAgencyRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
UpgradeCustomerToAgencyRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "UpgradeCustomerToAgency", action = "UpgradeCustomerToAgency")
public Future> upgradeCustomerToAgencyAsync(
@WebParam(name = "UpgradeCustomerToAgencyRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
UpgradeCustomerToAgencyRequest parameters,
@WebParam(name = "UpgradeCustomerToAgencyResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.UpgradeCustomerToAgencyResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "UpgradeCustomerToAgency", action = "UpgradeCustomerToAgency")
@WebResult(name = "UpgradeCustomerToAgencyResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public UpgradeCustomerToAgencyResponse upgradeCustomerToAgency(
@WebParam(name = "UpgradeCustomerToAgencyRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
UpgradeCustomerToAgencyRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "AddPrepayAccount", action = "AddPrepayAccount")
public Response addPrepayAccountAsync(
@WebParam(name = "AddPrepayAccountRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
AddPrepayAccountRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "AddPrepayAccount", action = "AddPrepayAccount")
public Future> addPrepayAccountAsync(
@WebParam(name = "AddPrepayAccountRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
AddPrepayAccountRequest parameters,
@WebParam(name = "AddPrepayAccountResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.AddPrepayAccountResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "AddPrepayAccount", action = "AddPrepayAccount")
@WebResult(name = "AddPrepayAccountResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public AddPrepayAccountResponse addPrepayAccount(
@WebParam(name = "AddPrepayAccountRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
AddPrepayAccountRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "UpdatePrepayAccount", action = "UpdatePrepayAccount")
public Response updatePrepayAccountAsync(
@WebParam(name = "UpdatePrepayAccountRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
UpdatePrepayAccountRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "UpdatePrepayAccount", action = "UpdatePrepayAccount")
public Future> updatePrepayAccountAsync(
@WebParam(name = "UpdatePrepayAccountRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
UpdatePrepayAccountRequest parameters,
@WebParam(name = "UpdatePrepayAccountResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.UpdatePrepayAccountResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "UpdatePrepayAccount", action = "UpdatePrepayAccount")
@WebResult(name = "UpdatePrepayAccountResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public UpdatePrepayAccountResponse updatePrepayAccount(
@WebParam(name = "UpdatePrepayAccountRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
UpdatePrepayAccountRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "MapCustomerIdToExternalCustomerId", action = "MapCustomerIdToExternalCustomerId")
public Response mapCustomerIdToExternalCustomerIdAsync(
@WebParam(name = "MapCustomerIdToExternalCustomerIdRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
MapCustomerIdToExternalCustomerIdRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "MapCustomerIdToExternalCustomerId", action = "MapCustomerIdToExternalCustomerId")
public Future> mapCustomerIdToExternalCustomerIdAsync(
@WebParam(name = "MapCustomerIdToExternalCustomerIdRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
MapCustomerIdToExternalCustomerIdRequest parameters,
@WebParam(name = "MapCustomerIdToExternalCustomerIdResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.MapCustomerIdToExternalCustomerIdResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "MapCustomerIdToExternalCustomerId", action = "MapCustomerIdToExternalCustomerId")
@WebResult(name = "MapCustomerIdToExternalCustomerIdResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public MapCustomerIdToExternalCustomerIdResponse mapCustomerIdToExternalCustomerId(
@WebParam(name = "MapCustomerIdToExternalCustomerIdRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
MapCustomerIdToExternalCustomerIdRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "MapAccountIdToExternalAccountIds", action = "MapAccountIdToExternalAccountIds")
public Response mapAccountIdToExternalAccountIdsAsync(
@WebParam(name = "MapAccountIdToExternalAccountIdsRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
MapAccountIdToExternalAccountIdsRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "MapAccountIdToExternalAccountIds", action = "MapAccountIdToExternalAccountIds")
public Future> mapAccountIdToExternalAccountIdsAsync(
@WebParam(name = "MapAccountIdToExternalAccountIdsRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
MapAccountIdToExternalAccountIdsRequest parameters,
@WebParam(name = "MapAccountIdToExternalAccountIdsResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.MapAccountIdToExternalAccountIdsResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "MapAccountIdToExternalAccountIds", action = "MapAccountIdToExternalAccountIds")
@WebResult(name = "MapAccountIdToExternalAccountIdsResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public MapAccountIdToExternalAccountIdsResponse mapAccountIdToExternalAccountIds(
@WebParam(name = "MapAccountIdToExternalAccountIdsRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
MapAccountIdToExternalAccountIdsRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "SearchCustomers", action = "SearchCustomers")
public Response searchCustomersAsync(
@WebParam(name = "SearchCustomersRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
SearchCustomersRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "SearchCustomers", action = "SearchCustomers")
public Future> searchCustomersAsync(
@WebParam(name = "SearchCustomersRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
SearchCustomersRequest parameters,
@WebParam(name = "SearchCustomersResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.SearchCustomersResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "SearchCustomers", action = "SearchCustomers")
@WebResult(name = "SearchCustomersResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public SearchCustomersResponse searchCustomers(
@WebParam(name = "SearchCustomersRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
SearchCustomersRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "AddClientLinks", action = "AddClientLinks")
public Response addClientLinksAsync(
@WebParam(name = "AddClientLinksRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
AddClientLinksRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "AddClientLinks", action = "AddClientLinks")
public Future> addClientLinksAsync(
@WebParam(name = "AddClientLinksRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
AddClientLinksRequest parameters,
@WebParam(name = "AddClientLinksResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.AddClientLinksResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "AddClientLinks", action = "AddClientLinks")
@WebResult(name = "AddClientLinksResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public AddClientLinksResponse addClientLinks(
@WebParam(name = "AddClientLinksRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
AddClientLinksRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "UpdateClientLinks", action = "UpdateClientLinks")
public Response updateClientLinksAsync(
@WebParam(name = "UpdateClientLinksRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
UpdateClientLinksRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "UpdateClientLinks", action = "UpdateClientLinks")
public Future> updateClientLinksAsync(
@WebParam(name = "UpdateClientLinksRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
UpdateClientLinksRequest parameters,
@WebParam(name = "UpdateClientLinksResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.UpdateClientLinksResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "UpdateClientLinks", action = "UpdateClientLinks")
@WebResult(name = "UpdateClientLinksResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public UpdateClientLinksResponse updateClientLinks(
@WebParam(name = "UpdateClientLinksRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
UpdateClientLinksRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "SearchClientLinks", action = "SearchClientLinks")
public Response searchClientLinksAsync(
@WebParam(name = "SearchClientLinksRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
SearchClientLinksRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "SearchClientLinks", action = "SearchClientLinks")
public Future> searchClientLinksAsync(
@WebParam(name = "SearchClientLinksRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
SearchClientLinksRequest parameters,
@WebParam(name = "SearchClientLinksResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.SearchClientLinksResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "SearchClientLinks", action = "SearchClientLinks")
@WebResult(name = "SearchClientLinksResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public SearchClientLinksResponse searchClientLinks(
@WebParam(name = "SearchClientLinksRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
SearchClientLinksRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "SearchAccounts", action = "SearchAccounts")
public Response searchAccountsAsync(
@WebParam(name = "SearchAccountsRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
SearchAccountsRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "SearchAccounts", action = "SearchAccounts")
public Future> searchAccountsAsync(
@WebParam(name = "SearchAccountsRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
SearchAccountsRequest parameters,
@WebParam(name = "SearchAccountsResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.SearchAccountsResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "SearchAccounts", action = "SearchAccounts")
@WebResult(name = "SearchAccountsResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public SearchAccountsResponse searchAccounts(
@WebParam(name = "SearchAccountsRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
SearchAccountsRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "SendUserInvitation", action = "SendUserInvitation")
public Response sendUserInvitationAsync(
@WebParam(name = "SendUserInvitationRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
SendUserInvitationRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "SendUserInvitation", action = "SendUserInvitation")
public Future> sendUserInvitationAsync(
@WebParam(name = "SendUserInvitationRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
SendUserInvitationRequest parameters,
@WebParam(name = "SendUserInvitationResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.SendUserInvitationResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "SendUserInvitation", action = "SendUserInvitation")
@WebResult(name = "SendUserInvitationResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public SendUserInvitationResponse sendUserInvitation(
@WebParam(name = "SendUserInvitationRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
SendUserInvitationRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "SearchUserInvitations", action = "SearchUserInvitations")
public Response searchUserInvitationsAsync(
@WebParam(name = "SearchUserInvitationsRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
SearchUserInvitationsRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "SearchUserInvitations", action = "SearchUserInvitations")
public Future> searchUserInvitationsAsync(
@WebParam(name = "SearchUserInvitationsRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
SearchUserInvitationsRequest parameters,
@WebParam(name = "SearchUserInvitationsResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.SearchUserInvitationsResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "SearchUserInvitations", action = "SearchUserInvitations")
@WebResult(name = "SearchUserInvitationsResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public SearchUserInvitationsResponse searchUserInvitations(
@WebParam(name = "SearchUserInvitationsRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
SearchUserInvitationsRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "ValidateAddress", action = "ValidateAddress")
public Response validateAddressAsync(
@WebParam(name = "ValidateAddressRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
ValidateAddressRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "ValidateAddress", action = "ValidateAddress")
public Future> validateAddressAsync(
@WebParam(name = "ValidateAddressRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
ValidateAddressRequest parameters,
@WebParam(name = "ValidateAddressResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.ValidateAddressResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "ValidateAddress", action = "ValidateAddress")
@WebResult(name = "ValidateAddressResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public ValidateAddressResponse validateAddress(
@WebParam(name = "ValidateAddressRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
ValidateAddressRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetLinkedAccountsAndCustomersInfo", action = "GetLinkedAccountsAndCustomersInfo")
public Response getLinkedAccountsAndCustomersInfoAsync(
@WebParam(name = "GetLinkedAccountsAndCustomersInfoRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetLinkedAccountsAndCustomersInfoRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetLinkedAccountsAndCustomersInfo", action = "GetLinkedAccountsAndCustomersInfo")
public Future> getLinkedAccountsAndCustomersInfoAsync(
@WebParam(name = "GetLinkedAccountsAndCustomersInfoRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetLinkedAccountsAndCustomersInfoRequest parameters,
@WebParam(name = "GetLinkedAccountsAndCustomersInfoResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.GetLinkedAccountsAndCustomersInfoResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "GetLinkedAccountsAndCustomersInfo", action = "GetLinkedAccountsAndCustomersInfo")
@WebResult(name = "GetLinkedAccountsAndCustomersInfoResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public GetLinkedAccountsAndCustomersInfoResponse getLinkedAccountsAndCustomersInfo(
@WebParam(name = "GetLinkedAccountsAndCustomersInfoRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetLinkedAccountsAndCustomersInfoRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetUserMFAStatus", action = "GetUserMFAStatus")
public Response getUserMFAStatusAsync(
@WebParam(name = "GetUserMFAStatusRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetUserMFAStatusRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetUserMFAStatus", action = "GetUserMFAStatus")
public Future> getUserMFAStatusAsync(
@WebParam(name = "GetUserMFAStatusRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetUserMFAStatusRequest parameters,
@WebParam(name = "GetUserMFAStatusResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.GetUserMFAStatusResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "GetUserMFAStatus", action = "GetUserMFAStatus")
@WebResult(name = "GetUserMFAStatusResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public GetUserMFAStatusResponse getUserMFAStatus(
@WebParam(name = "GetUserMFAStatusRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetUserMFAStatusRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "GetNotifications", action = "GetNotifications")
public Response getNotificationsAsync(
@WebParam(name = "GetNotificationsRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetNotificationsRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "GetNotifications", action = "GetNotifications")
public Future> getNotificationsAsync(
@WebParam(name = "GetNotificationsRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetNotificationsRequest parameters,
@WebParam(name = "GetNotificationsResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.GetNotificationsResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "GetNotifications", action = "GetNotifications")
@WebResult(name = "GetNotificationsResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public GetNotificationsResponse getNotifications(
@WebParam(name = "GetNotificationsRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
GetNotificationsRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
/**
*
* @param parameters
* @return
* returns jakarta.xml.ws.Response
*/
@WebMethod(operationName = "DismissNotifications", action = "DismissNotifications")
public Response dismissNotificationsAsync(
@WebParam(name = "DismissNotificationsRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
DismissNotificationsRequest parameters);
/**
*
* @param asyncHandler
* @param parameters
* @return
* returns java.util.concurrent.Future extends java.lang.Object>
*/
@WebMethod(operationName = "DismissNotifications", action = "DismissNotifications")
public Future> dismissNotificationsAsync(
@WebParam(name = "DismissNotificationsRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
DismissNotificationsRequest parameters,
@WebParam(name = "DismissNotificationsResponse", targetNamespace = "", partName = "asyncHandler")
AsyncHandler asyncHandler);
/**
*
* @param parameters
* @return
* returns com.microsoft.bingads.v13.customermanagement.DismissNotificationsResponse
* @throws AdApiFaultDetail_Exception
* @throws ApiFault_Exception
*/
@WebMethod(operationName = "DismissNotifications", action = "DismissNotifications")
@WebResult(name = "DismissNotificationsResponse", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
public DismissNotificationsResponse dismissNotifications(
@WebParam(name = "DismissNotificationsRequest", targetNamespace = "https://bingads.microsoft.com/Customer/v13", partName = "parameters")
DismissNotificationsRequest parameters)
throws AdApiFaultDetail_Exception, ApiFault_Exception
;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy