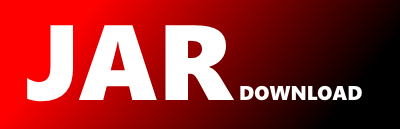
com.microsoft.bot.builder.classic.dialogs.BotDataBag Maven / Gradle / Ivy
//
// Copyright (c) Microsoft. All rights reserved.
// Licensed under the MIT license.
//
// Microsoft Bot Framework: http://botframework.com
//
// Bot Builder SDK GitHub:
// https://github.com/Microsoft/BotBuilder
//
// Copyright (c) Microsoft Corporation
// All rights reserved.
//
// MIT License:
// Permission is hereby granted, free of charge, to any person obtaining
// a copy of this software and associated documentation files (the
// "Software"), to deal in the Software without restriction, including
// without limitation the rights to use, copy, modify, merge, publish,
// distribute, sublicense, and/or sell copies of the Software, and to
// permit persons to whom the Software is furnished to do so, subject to
// the following conditions:
//
// The above copyright notice and this permission notice shall be
// included in all copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED ""AS IS"", WITHOUT WARRANTY OF ANY KIND,
// EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
// MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
// NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
// LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
// OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
// WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
//
package com.microsoft.bot.builder.classic.dialogs;
import com.microsoft.bot.builder.classic.dialogs.BotValue;
///
/// A property bag of bot data.
///
public interface BotDataBag
{
///
/// Gets the number of key/value pairs contained in the .
///
int getCount();
///
/// Checks if data bag contains a value with specified key
///
/// The key.
boolean ContainsKey(String key);
///
/// Gets the value associated with the specified key.
///
/// The type of the value to set.
/// The key of the value to get.
///
/// When this method returns, contains the value associated with the specified key, if the key is found;
/// otherwise, the default value for the type of the value parameter. This parameter is passed uninitialized.
///
/// true if the contains an element with the specified key; otherwise, false.
boolean TryGetValue(String key, BotValue value);
///
/// Adds the specified key and value to the bot data bag.
///
/// The type of the value to get.
/// The key of the element to add.
/// The value of the element to add. The value can be null for reference types.
void SetValue(String key, BotValue value);
///
/// Removes the specified key from the bot data bag.
///
/// They key of the element to remove
/// True if removal of the key is successful; otherwise, false
boolean RemoveValue(String key);
///
/// Removes all of the values from data bag.
///
void Clear();
}
/*
///
/// Helper methods.
///
public static partial class Extensions
{
[System.Obsolete(@"Use GetValue instead", false)]
public static T Get(this IBotDataBag bag, String key)
{
T value;
if (!bag.TryGetValue(key, out value))
{
throw new KeyNotFoundException(key);
}
return value;
}
///
/// Gets the value associated with the specified key.
///
/// The type of the value to get.
/// The bot data bag.
/// The key of the value to get or set.
/// The value associated with the specified key. If the specified key is not found, a get operation throws a KeyNotFoundException.
///
public static T GetValue(this IBotDataBag bag, String key)
{
T value;
if (!bag.TryGetValue(key, out value))
{
throw new KeyNotFoundException(key);
}
return value;
}
///
/// Gets the value associated with the specified key or a default value if not found.
///
/// The type of the value to get.
/// The bot data bag.
/// The key of the value to get or set.
/// The value to return if the key is not present
/// The value associated with the specified key. If the specified key is not found,
/// is returned
public static T GetValueOrDefault(this IBotDataBag bag, String key, T defaultValue = default(T))
{
T value;
if (!bag.TryGetValue(key, out value))
{
value = defaultValue;
}
return value;
}
}
*/
© 2015 - 2025 Weber Informatics LLC | Privacy Policy