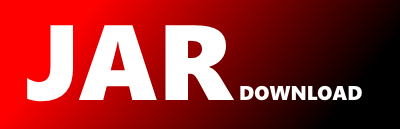
com.microsoft.bot.builder.core.TurnContext Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
package com.microsoft.bot.builder.core;
///
/// A method that can participate in send activity events for the current turn.
///
/// The context object for the turn.
/// The activities to send.
/// The delegate to call to continue event processing.
/// A task that represents the work queued to execute.
/// A handler calls the delegate to pass control to
/// the next registered handler. If a handler doesn’t call the next delegate,
/// the adapter does not call any of the subsequent handlers and does not send the
/// .
///
///
///
///
import com.microsoft.bot.schema.models.Activity;
import com.microsoft.bot.schema.models.ConversationReference;
import com.microsoft.bot.schema.models.ResourceResponse;
import java.util.concurrent.CompletableFuture;
//public delegate Task DeleteActivityHandler(ITurnContext context, ConversationReference reference, Func next);
///
/// Provides context for a turn of a bot.
///
/// Context provides information needed to process an incoming activity.
/// The context object is created by a and persists for the
/// length of the turn.
///
///
public interface TurnContext
{
///
/// Gets the bot adapter that created this context object.
///
// TODO: daveta
//BotAdapter getAdapter();
///
/// Gets the services registered on this context object.
///
// TODO: daveta
//ITurnContextServiceCollection getServices();
///
/// Incoming request
///
Activity getActivity();
///
/// Indicates whether at least one response was sent for the current turn.
///
/// true if at least one response was sent for the current turn.
boolean getResponded();
void setResponded(boolean responded);
///
/// Sends a message activity to the sender of the incoming activity.
///
/// The text of the message to send.
/// Optional, text to be spoken by your bot on a speech-enabled
/// channel.
/// Optional, indicates whether your bot is accepting,
/// expecting, or ignoring user input after the message is delivered to the client.
/// One of: "acceptingInput", "ignoringInput", or "expectingInput".
/// Default is "acceptingInput".
/// A task that represents the work queued to execute.
/// If the activity is successfully sent, the task result contains
/// a object containing the ID that the receiving
/// channel assigned to the activity.
/// See the channel's documentation for limits imposed upon the contents of
/// .
/// To control various characteristics of your bot's speech such as voice,
/// rate, volume, pronunciation, and pitch, specify in
/// Speech Synthesis Markup Language (SSML) format.
///
CompletableFuture SendActivity(String textReplyToSend);
CompletableFuture SendActivity(String textReplyToSend, String speak);
//CompletableFuture SendActivity(String textReplyToSend, String speak = null, String inputHint = InputHints.AcceptingInput);
CompletableFuture SendActivity(String textReplyToSend, String speak, String inputHint);
///
/// Sends an activity to the sender of the incoming activity.
///
/// The activity to send.
/// A task that represents the work queued to execute.
/// If the activity is successfully sent, the task result contains
/// a object containing the ID that the receiving
/// channel assigned to the activity.
CompletableFuture SendActivity(Activity activity);
///
/// Sends a set of activities to the sender of the incoming activity.
///
/// The activities to send.
/// A task that represents the work queued to execute.
/// If the activities are successfully sent, the task result contains
/// an array of objects containing the IDs that
/// the receiving channel assigned to the activities.
CompletableFuture SendActivities(Activity[] activities);
///
/// Replaces an existing activity.
///
/// New replacement activity.
/// A task that represents the work queued to execute.
/// If the activity is successfully sent, the task result contains
/// a object containing the ID that the receiving
/// channel assigned to the activity.
/// Before calling this, set the ID of the replacement activity to the ID
/// of the activity to replace.
CompletableFuture UpdateActivity(Activity activity);
///
/// Deletes an existing activity.
///
/// The ID of the activity to delete.
/// A task that represents the work queued to execute.
CompletableFuture DeleteActivity(String activityId);
///
/// Deletes an existing activity.
///
/// The conversation containing the activity to delete.
/// A task that represents the work queued to execute.
/// The conversation reference's
/// indicates the activity in the conversation to delete.
CompletableFuture DeleteActivity(ConversationReference conversationReference);
///
/// Adds a response handler for send activity operations.
///
/// The handler to add to the context object.
/// The updated context object.
/// When the context's
/// or methods are called,
/// the adapter calls the registered handlers in the order in which they were
/// added to the context object.
///
TurnContext OnSendActivities(SendActivitiesHandler handler);
///
/// Adds a response handler for update activity operations.
///
/// The handler to add to the context object.
/// The updated context object.
/// When the context's is called,
/// the adapter calls the registered handlers in the order in which they were
/// added to the context object.
///
TurnContext OnUpdateActivity(UpdateActivityHandler handler);
///
/// Adds a response handler for delete activity operations.
///
/// The handler to add to the context object.
/// The updated context object.
/// is null .
/// When the context's is called,
/// the adapter calls the registered handlers in the order in which they were
/// added to the context object.
///
TurnContext OnDeleteActivity(DeleteActivityHandler handler);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy