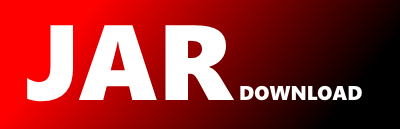
com.microsoft.bot.builder.dialogs.DialogContext Maven / Gradle / Ivy
package com.microsoft.bot.builder.dialogs;
//
// Copyright (c) Microsoft. All rights reserved.
// Licensed under the MIT license.
//
// Microsoft Bot Framework: http://botframework.com
//
// Bot Builder SDK GitHub:
// https://github.com/Microsoft/BotBuilder
//
// Copyright (c) Microsoft Corporation
// All rights reserved.
//
// MIT License:
// Permission is hereby granted, free of charge, to any person obtaining
// a copy of this software and associated documentation files (the
// "Software"), to deal in the Software without restriction, including
// without limitation the rights to use, copy, modify, merge, publish,
// distribute, sublicense, and/or sell copies of the Software, and to
// permit persons to whom the Software is furnished to do so, subject to
// the following conditions:
//
// The above copyright notice and this permission notice shall be
// included in all copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED ""AS IS"", WITHOUT WARRANTY OF ANY KIND,
// EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
// MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
// NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
// LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
// OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
// WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
//
///
/// Encapsulates a method that represents the code to execute after a result is available.
///
///
/// The result is often a message from the user.
///
/// The type of the result.
/// The dialog context.
/// The result.
/// A task that represents the code that will resume after the result is available.
import com.microsoft.bot.schema.models.Attachment;
import com.microsoft.bot.schema.models.AttachmentLayoutTypes;
import com.microsoft.bot.schema.models.Entity;
import com.microsoft.bot.schema.models.TextFormatTypes;
import java.util.ArrayList;
import java.util.List;
/*
public interface ResumeAfter
{
CompletableFuture invoke(DialogContext contenxt, Available)
}
public delegate Task ResumeAfter(IDialogContext context, IAwaitable result);
///
/// Encapsulate a method that represents the code to start a dialog.
///
/// The dialog context.
/// A task that represents the start code for a dialog.
public delegate Task StartAsync(IDialogContext context);
*/
///
/// The context for the execution of a dialog's conversational process.
///
// DAVETA: TODO
// public interface DialogContext extends DialogStack, BotContext
public interface DialogContext extends BotContext
{
}
///
/// Helper methods.
///
/*
public static partial class Extensions
{
///
/// Post a message to be sent to the user, using previous messages to establish a conversation context.
///
///
/// If the locale parameter is not set, locale of the incoming message will be used for reply.
///
/// Communication channel to use.
/// The message text.
/// The locale of the text.
/// The cancellation token.
/// A task that represents the post operation.
public static async Task PostAsync(this IBotToUser botToUser, string text, string locale = null, CancellationToken cancellationToken = default(CancellationToken))
{
var message = botToUser.MakeMessage();
message.Text = text;
if (!string.IsNullOrEmpty(locale))
{
message.Locale = locale;
}
await botToUser.PostAsync(message, cancellationToken);
}
///
/// Post a message and optional SSML to be sent to the user, using previous messages to establish a conversation context.
///
///
/// If the locale parameter is not set, locale of the incoming message will be used for reply.
///
/// Communication channel to use.
/// The message text.
/// The SSML markup for text to speech.
/// The options for the message.
/// The locale of the text.
/// The cancellation token.
/// A task that represents the post operation.
public static async Task SayAsync(this IBotToUser botToUser, string text, string speak = null, MessageOptions options = null, string locale = null, CancellationToken cancellationToken = default(CancellationToken))
{
var message = botToUser.MakeMessage();
message.Text = text;
message.Speak = speak;
if (!string.IsNullOrEmpty(locale))
{
message.Locale = locale;
}
if (options != null)
{
message.InputHint = options.InputHint;
message.TextFormat = options.TextFormat;
message.AttachmentLayout = options.AttachmentLayout;
message.Attachments = options.Attachments;
message.Entities = options.Entities;
}
await botToUser.PostAsync(message, cancellationToken);
}
///
/// Suspend the current dialog until the user has sent a message to the bot.
///
/// The dialog stack.
/// The method to resume when the message has been received.
public static void Wait(this IDialogStack stack, ResumeAfter resume)
{
stack.Wait(resume);
}
///
/// Call a child dialog, add it to the top of the stack and post the message to the child dialog.
///
/// The type of result expected from the child dialog.
/// The dialog stack.
/// The child dialog.
/// The method to resume when the child dialog has completed.
/// The message that will be posted to child dialog.
/// A cancellation token.
/// A task representing the Forward operation.
public static async Task Forward(this IDialogStack stack, IDialog child, ResumeAfter resume, IMessageActivity message, CancellationToken token = default(CancellationToken))
{
await stack.Forward(child, resume, message, token);
}
}
*/
© 2015 - 2025 Weber Informatics LLC | Privacy Policy