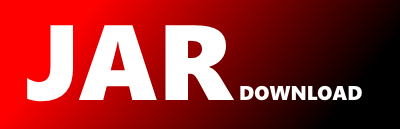
com.microsoft.gctoolkit.parser.unified.UnifiedLoggingTokens Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation.
// Licensed under the MIT License.
package com.microsoft.gctoolkit.parser.unified;
import com.microsoft.gctoolkit.parser.GenericTokens;
import java.util.regex.Pattern;
public interface UnifiedLoggingTokens extends GenericTokens {
/*
JEP 158 Decorators
time -- Current time and date in ISO-8601 format
uptime -- Time since the start of the JVM in seconds and milliseconds (e.g., 6.567s)
timemillis -- The same value as generated by System.currentTimeMillis()
uptimemillis -- Milliseconds since the JVM started
timenanos -- The same value as generated by System.nanoTime()
uptimenanos -- Nanoseconds since the JVM started
pid -- The process identifier
tid -- The thread identifier
level -- The level associated with the log message
tags -- The tag-set associated with the log message
[2018-04-04T09:10:00.586-0100][0.018s][1522825800586ms][18ms][10026341461044ns][17738937ns][1375][7427][info][gc] Using G1
*/
String DATE_STAMP = "\\[" + DATE + "\\]";
String UPTIME = "\\[" + INTEGER + DECIMAL_POINT + "\\d{3}s\\]";
String TIME_MILLIS = "\\[\\d+ms\\]";
String TIME_NANOS = "\\[\\d+ns\\]";
String PID_TID = "\\[\\d+\\]";
String UNIFIED_LOG_LEVEL_BLOCK = "\\[(?:error|warning|info|debug|trace|develop) *\\]";
Pattern DECORATORS = Pattern.compile("(" + DATE_STAMP + ")?(" + UPTIME + ")?(" + TIME_MILLIS + ")?(" + TIME_MILLIS + ")?(" + TIME_NANOS + ")?(" + TIME_NANOS + ")?(" + PID_TID + ")?(" + PID_TID + ")?(" + UNIFIED_LOG_LEVEL_BLOCK + ")?");
//Using zero-width negative lookbehind to miss capturing records formatted like [0x1f03].
//[0.081s][trace][safepoint] Thread: 0x00007fd0d2006800 [0x1f03] State: _at_safepoint _has_called_back 0 _at_poll_safepoint 0
Pattern TAGS = Pattern.compile(".*(?<=^|\\])\\[([a-z0-9,. ]+)\\]");
String UNIFIED_META_RECORD = "Metaspace: " + BEFORE_AFTER_CONFIGURED;
String WORKER_SUMMARY_REAL = "Min:\\s+" + TIME + ", Avg:\\s+" + TIME + ", Max:\\s+" + TIME + ", Diff:\\s+" + TIME + ", Sum:\\s+" + TIME + ", Workers:\\s+" + COUNTER;
String WORKER_SUMMARY_INT = "Min:\\s+" + COUNTER + ", Avg:\\s+" + TIME + ", Max:\\s+" + COUNTER + ", Diff:\\s+" + COUNTER + ", Sum:\\s+" + COUNTER + ", Workers:\\s+" + COUNTER;
String POOL_SUMMARY = "used " + COUNTER + "K, capacity " + COUNTER + "K, committed " + COUNTER + "K, reserved " + COUNTER + "K";
String REGION_MEMORY_BLOCK = COUNTER + "->" + COUNTER + "(?:\\(" + COUNTER + "\\))?";
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy