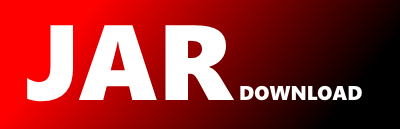
com.microsoft.graph.beta.generated.models.AospDeviceOwnerScepCertificateProfile Maven / Gradle / Ivy
package com.microsoft.graph.beta.models;
import com.microsoft.kiota.serialization.Parsable;
import com.microsoft.kiota.serialization.ParseNode;
import com.microsoft.kiota.serialization.SerializationWriter;
import java.util.EnumSet;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
/**
* AOSP Device Owner SCEP certificate profile
*/
@jakarta.annotation.Generated("com.microsoft.kiota")
public class AospDeviceOwnerScepCertificateProfile extends AospDeviceOwnerCertificateProfileBase implements Parsable {
/**
* Instantiates a new {@link AospDeviceOwnerScepCertificateProfile} and sets the default values.
*/
public AospDeviceOwnerScepCertificateProfile() {
super();
this.setOdataType("#microsoft.graph.aospDeviceOwnerScepCertificateProfile");
}
/**
* Creates a new instance of the appropriate class based on discriminator value
* @param parseNode The parse node to use to read the discriminator value and create the object
* @return a {@link AospDeviceOwnerScepCertificateProfile}
*/
@jakarta.annotation.Nonnull
public static AospDeviceOwnerScepCertificateProfile createFromDiscriminatorValue(@jakarta.annotation.Nonnull final ParseNode parseNode) {
Objects.requireNonNull(parseNode);
return new AospDeviceOwnerScepCertificateProfile();
}
/**
* Gets the certificateStore property value. Target store certificate. This collection can contain a maximum of 500 elements. Possible values are: user, machine.
* @return a {@link CertificateStore}
*/
@jakarta.annotation.Nullable
public CertificateStore getCertificateStore() {
return this.backingStore.get("certificateStore");
}
/**
* Gets the customSubjectAlternativeNames property value. Custom Subject Alternative Name Settings. This collection can contain a maximum of 500 elements.
* @return a {@link java.util.List}
*/
@jakarta.annotation.Nullable
public java.util.List getCustomSubjectAlternativeNames() {
return this.backingStore.get("customSubjectAlternativeNames");
}
/**
* The deserialization information for the current model
* @return a {@link Map>}
*/
@jakarta.annotation.Nonnull
public Map> getFieldDeserializers() {
final HashMap> deserializerMap = new HashMap>(super.getFieldDeserializers());
deserializerMap.put("certificateStore", (n) -> { this.setCertificateStore(n.getEnumValue(CertificateStore::forValue)); });
deserializerMap.put("customSubjectAlternativeNames", (n) -> { this.setCustomSubjectAlternativeNames(n.getCollectionOfObjectValues(CustomSubjectAlternativeName::createFromDiscriminatorValue)); });
deserializerMap.put("hashAlgorithm", (n) -> { this.setHashAlgorithm(n.getEnumSetValue(HashAlgorithms::forValue)); });
deserializerMap.put("keySize", (n) -> { this.setKeySize(n.getEnumValue(KeySize::forValue)); });
deserializerMap.put("keyUsage", (n) -> { this.setKeyUsage(n.getEnumSetValue(KeyUsages::forValue)); });
deserializerMap.put("managedDeviceCertificateStates", (n) -> { this.setManagedDeviceCertificateStates(n.getCollectionOfObjectValues(ManagedDeviceCertificateState::createFromDiscriminatorValue)); });
deserializerMap.put("scepServerUrls", (n) -> { this.setScepServerUrls(n.getCollectionOfPrimitiveValues(String.class)); });
deserializerMap.put("subjectAlternativeNameFormatString", (n) -> { this.setSubjectAlternativeNameFormatString(n.getStringValue()); });
deserializerMap.put("subjectNameFormatString", (n) -> { this.setSubjectNameFormatString(n.getStringValue()); });
return deserializerMap;
}
/**
* Gets the hashAlgorithm property value. Hash Algorithm Options.
* @return a {@link EnumSet}
*/
@jakarta.annotation.Nullable
public EnumSet getHashAlgorithm() {
return this.backingStore.get("hashAlgorithm");
}
/**
* Gets the keySize property value. Key Size Options.
* @return a {@link KeySize}
*/
@jakarta.annotation.Nullable
public KeySize getKeySize() {
return this.backingStore.get("keySize");
}
/**
* Gets the keyUsage property value. Key Usage Options.
* @return a {@link EnumSet}
*/
@jakarta.annotation.Nullable
public EnumSet getKeyUsage() {
return this.backingStore.get("keyUsage");
}
/**
* Gets the managedDeviceCertificateStates property value. Certificate state for devices. This collection can contain a maximum of 2147483647 elements.
* @return a {@link java.util.List}
*/
@jakarta.annotation.Nullable
public java.util.List getManagedDeviceCertificateStates() {
return this.backingStore.get("managedDeviceCertificateStates");
}
/**
* Gets the scepServerUrls property value. SCEP Server Url(s)
* @return a {@link java.util.List}
*/
@jakarta.annotation.Nullable
public java.util.List getScepServerUrls() {
return this.backingStore.get("scepServerUrls");
}
/**
* Gets the subjectAlternativeNameFormatString property value. Custom String that defines the AAD Attribute.
* @return a {@link String}
*/
@jakarta.annotation.Nullable
public String getSubjectAlternativeNameFormatString() {
return this.backingStore.get("subjectAlternativeNameFormatString");
}
/**
* Gets the subjectNameFormatString property value. Custom format to use with SubjectNameFormat = Custom. Example: CN={{EmailAddress}},E={{EmailAddress}},OU=Enterprise Users,O=Contoso Corporation,L=Redmond,ST=WA,C=US
* @return a {@link String}
*/
@jakarta.annotation.Nullable
public String getSubjectNameFormatString() {
return this.backingStore.get("subjectNameFormatString");
}
/**
* Serializes information the current object
* @param writer Serialization writer to use to serialize this model
*/
public void serialize(@jakarta.annotation.Nonnull final SerializationWriter writer) {
Objects.requireNonNull(writer);
super.serialize(writer);
writer.writeEnumValue("certificateStore", this.getCertificateStore());
writer.writeCollectionOfObjectValues("customSubjectAlternativeNames", this.getCustomSubjectAlternativeNames());
writer.writeEnumSetValue("hashAlgorithm", this.getHashAlgorithm());
writer.writeEnumValue("keySize", this.getKeySize());
writer.writeEnumSetValue("keyUsage", this.getKeyUsage());
writer.writeCollectionOfObjectValues("managedDeviceCertificateStates", this.getManagedDeviceCertificateStates());
writer.writeCollectionOfPrimitiveValues("scepServerUrls", this.getScepServerUrls());
writer.writeStringValue("subjectAlternativeNameFormatString", this.getSubjectAlternativeNameFormatString());
writer.writeStringValue("subjectNameFormatString", this.getSubjectNameFormatString());
}
/**
* Sets the certificateStore property value. Target store certificate. This collection can contain a maximum of 500 elements. Possible values are: user, machine.
* @param value Value to set for the certificateStore property.
*/
public void setCertificateStore(@jakarta.annotation.Nullable final CertificateStore value) {
this.backingStore.set("certificateStore", value);
}
/**
* Sets the customSubjectAlternativeNames property value. Custom Subject Alternative Name Settings. This collection can contain a maximum of 500 elements.
* @param value Value to set for the customSubjectAlternativeNames property.
*/
public void setCustomSubjectAlternativeNames(@jakarta.annotation.Nullable final java.util.List value) {
this.backingStore.set("customSubjectAlternativeNames", value);
}
/**
* Sets the hashAlgorithm property value. Hash Algorithm Options.
* @param value Value to set for the hashAlgorithm property.
*/
public void setHashAlgorithm(@jakarta.annotation.Nullable final EnumSet value) {
this.backingStore.set("hashAlgorithm", value);
}
/**
* Sets the keySize property value. Key Size Options.
* @param value Value to set for the keySize property.
*/
public void setKeySize(@jakarta.annotation.Nullable final KeySize value) {
this.backingStore.set("keySize", value);
}
/**
* Sets the keyUsage property value. Key Usage Options.
* @param value Value to set for the keyUsage property.
*/
public void setKeyUsage(@jakarta.annotation.Nullable final EnumSet value) {
this.backingStore.set("keyUsage", value);
}
/**
* Sets the managedDeviceCertificateStates property value. Certificate state for devices. This collection can contain a maximum of 2147483647 elements.
* @param value Value to set for the managedDeviceCertificateStates property.
*/
public void setManagedDeviceCertificateStates(@jakarta.annotation.Nullable final java.util.List value) {
this.backingStore.set("managedDeviceCertificateStates", value);
}
/**
* Sets the scepServerUrls property value. SCEP Server Url(s)
* @param value Value to set for the scepServerUrls property.
*/
public void setScepServerUrls(@jakarta.annotation.Nullable final java.util.List value) {
this.backingStore.set("scepServerUrls", value);
}
/**
* Sets the subjectAlternativeNameFormatString property value. Custom String that defines the AAD Attribute.
* @param value Value to set for the subjectAlternativeNameFormatString property.
*/
public void setSubjectAlternativeNameFormatString(@jakarta.annotation.Nullable final String value) {
this.backingStore.set("subjectAlternativeNameFormatString", value);
}
/**
* Sets the subjectNameFormatString property value. Custom format to use with SubjectNameFormat = Custom. Example: CN={{EmailAddress}},E={{EmailAddress}},OU=Enterprise Users,O=Contoso Corporation,L=Redmond,ST=WA,C=US
* @param value Value to set for the subjectNameFormatString property.
*/
public void setSubjectNameFormatString(@jakarta.annotation.Nullable final String value) {
this.backingStore.set("subjectNameFormatString", value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy