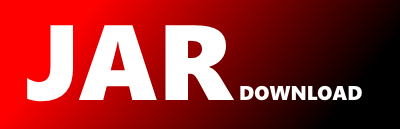
com.microsoft.graph.beta.generated.models.AppVulnerabilityTask Maven / Gradle / Ivy
package com.microsoft.graph.beta.models;
import com.microsoft.kiota.serialization.Parsable;
import com.microsoft.kiota.serialization.ParseNode;
import com.microsoft.kiota.serialization.SerializationWriter;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
/**
* An app vulnerability task.
*/
@jakarta.annotation.Generated("com.microsoft.kiota")
public class AppVulnerabilityTask extends DeviceAppManagementTask implements Parsable {
/**
* Instantiates a new {@link AppVulnerabilityTask} and sets the default values.
*/
public AppVulnerabilityTask() {
super();
this.setOdataType("#microsoft.graph.appVulnerabilityTask");
}
/**
* Creates a new instance of the appropriate class based on discriminator value
* @param parseNode The parse node to use to read the discriminator value and create the object
* @return a {@link AppVulnerabilityTask}
*/
@jakarta.annotation.Nonnull
public static AppVulnerabilityTask createFromDiscriminatorValue(@jakarta.annotation.Nonnull final ParseNode parseNode) {
Objects.requireNonNull(parseNode);
return new AppVulnerabilityTask();
}
/**
* Gets the appName property value. The app name.
* @return a {@link String}
*/
@jakarta.annotation.Nullable
public String getAppName() {
return this.backingStore.get("appName");
}
/**
* Gets the appPublisher property value. The app publisher.
* @return a {@link String}
*/
@jakarta.annotation.Nullable
public String getAppPublisher() {
return this.backingStore.get("appPublisher");
}
/**
* Gets the appVersion property value. The app version.
* @return a {@link String}
*/
@jakarta.annotation.Nullable
public String getAppVersion() {
return this.backingStore.get("appVersion");
}
/**
* The deserialization information for the current model
* @return a {@link Map>}
*/
@jakarta.annotation.Nonnull
public Map> getFieldDeserializers() {
final HashMap> deserializerMap = new HashMap>(super.getFieldDeserializers());
deserializerMap.put("appName", (n) -> { this.setAppName(n.getStringValue()); });
deserializerMap.put("appPublisher", (n) -> { this.setAppPublisher(n.getStringValue()); });
deserializerMap.put("appVersion", (n) -> { this.setAppVersion(n.getStringValue()); });
deserializerMap.put("insights", (n) -> { this.setInsights(n.getStringValue()); });
deserializerMap.put("managedDeviceCount", (n) -> { this.setManagedDeviceCount(n.getIntegerValue()); });
deserializerMap.put("managedDevices", (n) -> { this.setManagedDevices(n.getCollectionOfObjectValues(AppVulnerabilityManagedDevice::createFromDiscriminatorValue)); });
deserializerMap.put("mitigationType", (n) -> { this.setMitigationType(n.getEnumValue(AppVulnerabilityTaskMitigationType::forValue)); });
deserializerMap.put("mobileAppCount", (n) -> { this.setMobileAppCount(n.getIntegerValue()); });
deserializerMap.put("mobileApps", (n) -> { this.setMobileApps(n.getCollectionOfObjectValues(AppVulnerabilityMobileApp::createFromDiscriminatorValue)); });
deserializerMap.put("remediation", (n) -> { this.setRemediation(n.getStringValue()); });
return deserializerMap;
}
/**
* Gets the insights property value. Information about the mitigation.
* @return a {@link String}
*/
@jakarta.annotation.Nullable
public String getInsights() {
return this.backingStore.get("insights");
}
/**
* Gets the managedDeviceCount property value. The number of vulnerable devices.
* @return a {@link Integer}
*/
@jakarta.annotation.Nullable
public Integer getManagedDeviceCount() {
return this.backingStore.get("managedDeviceCount");
}
/**
* Gets the managedDevices property value. The vulnerable managed devices.
* @return a {@link java.util.List}
*/
@jakarta.annotation.Nullable
public java.util.List getManagedDevices() {
return this.backingStore.get("managedDevices");
}
/**
* Gets the mitigationType property value. Device app management task mitigation type.
* @return a {@link AppVulnerabilityTaskMitigationType}
*/
@jakarta.annotation.Nullable
public AppVulnerabilityTaskMitigationType getMitigationType() {
return this.backingStore.get("mitigationType");
}
/**
* Gets the mobileAppCount property value. The number of vulnerable mobile apps.
* @return a {@link Integer}
*/
@jakarta.annotation.Nullable
public Integer getMobileAppCount() {
return this.backingStore.get("mobileAppCount");
}
/**
* Gets the mobileApps property value. The vulnerable mobile apps.
* @return a {@link java.util.List}
*/
@jakarta.annotation.Nullable
public java.util.List getMobileApps() {
return this.backingStore.get("mobileApps");
}
/**
* Gets the remediation property value. The remediation steps.
* @return a {@link String}
*/
@jakarta.annotation.Nullable
public String getRemediation() {
return this.backingStore.get("remediation");
}
/**
* Serializes information the current object
* @param writer Serialization writer to use to serialize this model
*/
public void serialize(@jakarta.annotation.Nonnull final SerializationWriter writer) {
Objects.requireNonNull(writer);
super.serialize(writer);
writer.writeStringValue("appName", this.getAppName());
writer.writeStringValue("appPublisher", this.getAppPublisher());
writer.writeStringValue("appVersion", this.getAppVersion());
writer.writeStringValue("insights", this.getInsights());
writer.writeIntegerValue("managedDeviceCount", this.getManagedDeviceCount());
writer.writeCollectionOfObjectValues("managedDevices", this.getManagedDevices());
writer.writeEnumValue("mitigationType", this.getMitigationType());
writer.writeIntegerValue("mobileAppCount", this.getMobileAppCount());
writer.writeCollectionOfObjectValues("mobileApps", this.getMobileApps());
writer.writeStringValue("remediation", this.getRemediation());
}
/**
* Sets the appName property value. The app name.
* @param value Value to set for the appName property.
*/
public void setAppName(@jakarta.annotation.Nullable final String value) {
this.backingStore.set("appName", value);
}
/**
* Sets the appPublisher property value. The app publisher.
* @param value Value to set for the appPublisher property.
*/
public void setAppPublisher(@jakarta.annotation.Nullable final String value) {
this.backingStore.set("appPublisher", value);
}
/**
* Sets the appVersion property value. The app version.
* @param value Value to set for the appVersion property.
*/
public void setAppVersion(@jakarta.annotation.Nullable final String value) {
this.backingStore.set("appVersion", value);
}
/**
* Sets the insights property value. Information about the mitigation.
* @param value Value to set for the insights property.
*/
public void setInsights(@jakarta.annotation.Nullable final String value) {
this.backingStore.set("insights", value);
}
/**
* Sets the managedDeviceCount property value. The number of vulnerable devices.
* @param value Value to set for the managedDeviceCount property.
*/
public void setManagedDeviceCount(@jakarta.annotation.Nullable final Integer value) {
this.backingStore.set("managedDeviceCount", value);
}
/**
* Sets the managedDevices property value. The vulnerable managed devices.
* @param value Value to set for the managedDevices property.
*/
public void setManagedDevices(@jakarta.annotation.Nullable final java.util.List value) {
this.backingStore.set("managedDevices", value);
}
/**
* Sets the mitigationType property value. Device app management task mitigation type.
* @param value Value to set for the mitigationType property.
*/
public void setMitigationType(@jakarta.annotation.Nullable final AppVulnerabilityTaskMitigationType value) {
this.backingStore.set("mitigationType", value);
}
/**
* Sets the mobileAppCount property value. The number of vulnerable mobile apps.
* @param value Value to set for the mobileAppCount property.
*/
public void setMobileAppCount(@jakarta.annotation.Nullable final Integer value) {
this.backingStore.set("mobileAppCount", value);
}
/**
* Sets the mobileApps property value. The vulnerable mobile apps.
* @param value Value to set for the mobileApps property.
*/
public void setMobileApps(@jakarta.annotation.Nullable final java.util.List value) {
this.backingStore.set("mobileApps", value);
}
/**
* Sets the remediation property value. The remediation steps.
* @param value Value to set for the remediation property.
*/
public void setRemediation(@jakarta.annotation.Nullable final String value) {
this.backingStore.set("remediation", value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy