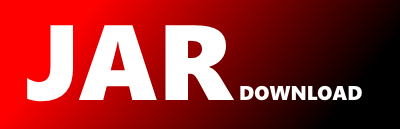
com.microsoft.graph.beta.generated.models.AccessReviewInstance Maven / Gradle / Ivy
package com.microsoft.graph.beta.models;
import com.microsoft.kiota.serialization.Parsable;
import com.microsoft.kiota.serialization.ParseNode;
import com.microsoft.kiota.serialization.SerializationWriter;
import java.time.OffsetDateTime;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
@jakarta.annotation.Generated("com.microsoft.kiota")
public class AccessReviewInstance extends Entity implements Parsable {
/**
* Instantiates a new {@link AccessReviewInstance} and sets the default values.
*/
public AccessReviewInstance() {
super();
}
/**
* Creates a new instance of the appropriate class based on discriminator value
* @param parseNode The parse node to use to read the discriminator value and create the object
* @return a {@link AccessReviewInstance}
*/
@jakarta.annotation.Nonnull
public static AccessReviewInstance createFromDiscriminatorValue(@jakarta.annotation.Nonnull final ParseNode parseNode) {
Objects.requireNonNull(parseNode);
return new AccessReviewInstance();
}
/**
* Gets the contactedReviewers property value. Returns the collection of reviewers who were contacted to complete this review. While the reviewers and fallbackReviewers properties of the accessReviewScheduleDefinition might specify group owners or managers as reviewers, contactedReviewers returns their individual identities. Supports $select. Read-only.
* @return a {@link java.util.List}
*/
@jakarta.annotation.Nullable
public java.util.List getContactedReviewers() {
return this.backingStore.get("contactedReviewers");
}
/**
* Gets the decisions property value. Each user reviewed in an accessReviewInstance has a decision item representing if they were approved, denied, or not yet reviewed.
* @return a {@link java.util.List}
*/
@jakarta.annotation.Nullable
public java.util.List getDecisions() {
return this.backingStore.get("decisions");
}
/**
* Gets the definition property value. There's exactly one accessReviewScheduleDefinition associated with each instance. It's the parent schedule for the instance, where instances are created for each recurrence of a review definition and each group selected to review by the definition.
* @return a {@link AccessReviewScheduleDefinition}
*/
@jakarta.annotation.Nullable
public AccessReviewScheduleDefinition getDefinition() {
return this.backingStore.get("definition");
}
/**
* Gets the endDateTime property value. DateTime when review instance is scheduled to end. The DatetimeOffset type represents date and time information using ISO 8601 format and is always in UTC time. For example, midnight UTC on Jan 1, 2014 is 2014-01-01T00:00:00Z. Supports $select. Read-only.
* @return a {@link OffsetDateTime}
*/
@jakarta.annotation.Nullable
public OffsetDateTime getEndDateTime() {
return this.backingStore.get("endDateTime");
}
/**
* Gets the errors property value. Collection of errors in an access review instance lifecycle. Read-only.
* @return a {@link java.util.List}
*/
@jakarta.annotation.Nullable
public java.util.List getErrors() {
return this.backingStore.get("errors");
}
/**
* Gets the fallbackReviewers property value. This collection of reviewer scopes is used to define the list of fallback reviewers. These fallback reviewers are notified to take action if no users are found from the list of reviewers specified. This could occur when either the group owner is specified as the reviewer but the group owner doesn't exist, or manager is specified as reviewer but a user's manager doesn't exist. Supports $select.
* @return a {@link java.util.List}
*/
@jakarta.annotation.Nullable
public java.util.List getFallbackReviewers() {
return this.backingStore.get("fallbackReviewers");
}
/**
* The deserialization information for the current model
* @return a {@link Map>}
*/
@jakarta.annotation.Nonnull
public Map> getFieldDeserializers() {
final HashMap> deserializerMap = new HashMap>(super.getFieldDeserializers());
deserializerMap.put("contactedReviewers", (n) -> { this.setContactedReviewers(n.getCollectionOfObjectValues(AccessReviewReviewer::createFromDiscriminatorValue)); });
deserializerMap.put("decisions", (n) -> { this.setDecisions(n.getCollectionOfObjectValues(AccessReviewInstanceDecisionItem::createFromDiscriminatorValue)); });
deserializerMap.put("definition", (n) -> { this.setDefinition(n.getObjectValue(AccessReviewScheduleDefinition::createFromDiscriminatorValue)); });
deserializerMap.put("endDateTime", (n) -> { this.setEndDateTime(n.getOffsetDateTimeValue()); });
deserializerMap.put("errors", (n) -> { this.setErrors(n.getCollectionOfObjectValues(AccessReviewError::createFromDiscriminatorValue)); });
deserializerMap.put("fallbackReviewers", (n) -> { this.setFallbackReviewers(n.getCollectionOfObjectValues(AccessReviewReviewerScope::createFromDiscriminatorValue)); });
deserializerMap.put("reviewers", (n) -> { this.setReviewers(n.getCollectionOfObjectValues(AccessReviewReviewerScope::createFromDiscriminatorValue)); });
deserializerMap.put("scope", (n) -> { this.setScope(n.getObjectValue(AccessReviewScope::createFromDiscriminatorValue)); });
deserializerMap.put("stages", (n) -> { this.setStages(n.getCollectionOfObjectValues(AccessReviewStage::createFromDiscriminatorValue)); });
deserializerMap.put("startDateTime", (n) -> { this.setStartDateTime(n.getOffsetDateTimeValue()); });
deserializerMap.put("status", (n) -> { this.setStatus(n.getStringValue()); });
return deserializerMap;
}
/**
* Gets the reviewers property value. This collection of access review scopes is used to define who the reviewers are. Supports $select. For examples of options for assigning reviewers, see Assign reviewers to your access review definition using the Microsoft Graph API.
* @return a {@link java.util.List}
*/
@jakarta.annotation.Nullable
public java.util.List getReviewers() {
return this.backingStore.get("reviewers");
}
/**
* Gets the scope property value. Created based on scope and instanceEnumerationScope at the accessReviewScheduleDefinition level. Defines the scope of users reviewed in a group. Supports $select and $filter (contains only). Read-only.
* @return a {@link AccessReviewScope}
*/
@jakarta.annotation.Nullable
public AccessReviewScope getScope() {
return this.backingStore.get("scope");
}
/**
* Gets the stages property value. If the instance has multiple stages, this returns the collection of stages. A new stage will only be created when the previous stage ends. The existence, number, and settings of stages on a review instance are created based on the accessReviewStageSettings on the parent accessReviewScheduleDefinition.
* @return a {@link java.util.List}
*/
@jakarta.annotation.Nullable
public java.util.List getStages() {
return this.backingStore.get("stages");
}
/**
* Gets the startDateTime property value. DateTime when review instance is scheduled to start. May be in the future. The DateTimeOffset type represents date and time information using ISO 8601 format and is always in UTC time. For example, midnight UTC on Jan 1, 2014 is 2014-01-01T00:00:00Z. Supports $select. Read-only.
* @return a {@link OffsetDateTime}
*/
@jakarta.annotation.Nullable
public OffsetDateTime getStartDateTime() {
return this.backingStore.get("startDateTime");
}
/**
* Gets the status property value. Specifies the status of an accessReview. Possible values: Initializing, NotStarted, Starting, InProgress, Completing, Completed, AutoReviewing, and AutoReviewed. Supports $select, $orderby, and $filter (eq only). Read-only.
* @return a {@link String}
*/
@jakarta.annotation.Nullable
public String getStatus() {
return this.backingStore.get("status");
}
/**
* Serializes information the current object
* @param writer Serialization writer to use to serialize this model
*/
public void serialize(@jakarta.annotation.Nonnull final SerializationWriter writer) {
Objects.requireNonNull(writer);
super.serialize(writer);
writer.writeCollectionOfObjectValues("contactedReviewers", this.getContactedReviewers());
writer.writeCollectionOfObjectValues("decisions", this.getDecisions());
writer.writeObjectValue("definition", this.getDefinition());
writer.writeOffsetDateTimeValue("endDateTime", this.getEndDateTime());
writer.writeCollectionOfObjectValues("errors", this.getErrors());
writer.writeCollectionOfObjectValues("fallbackReviewers", this.getFallbackReviewers());
writer.writeCollectionOfObjectValues("reviewers", this.getReviewers());
writer.writeObjectValue("scope", this.getScope());
writer.writeCollectionOfObjectValues("stages", this.getStages());
writer.writeOffsetDateTimeValue("startDateTime", this.getStartDateTime());
writer.writeStringValue("status", this.getStatus());
}
/**
* Sets the contactedReviewers property value. Returns the collection of reviewers who were contacted to complete this review. While the reviewers and fallbackReviewers properties of the accessReviewScheduleDefinition might specify group owners or managers as reviewers, contactedReviewers returns their individual identities. Supports $select. Read-only.
* @param value Value to set for the contactedReviewers property.
*/
public void setContactedReviewers(@jakarta.annotation.Nullable final java.util.List value) {
this.backingStore.set("contactedReviewers", value);
}
/**
* Sets the decisions property value. Each user reviewed in an accessReviewInstance has a decision item representing if they were approved, denied, or not yet reviewed.
* @param value Value to set for the decisions property.
*/
public void setDecisions(@jakarta.annotation.Nullable final java.util.List value) {
this.backingStore.set("decisions", value);
}
/**
* Sets the definition property value. There's exactly one accessReviewScheduleDefinition associated with each instance. It's the parent schedule for the instance, where instances are created for each recurrence of a review definition and each group selected to review by the definition.
* @param value Value to set for the definition property.
*/
public void setDefinition(@jakarta.annotation.Nullable final AccessReviewScheduleDefinition value) {
this.backingStore.set("definition", value);
}
/**
* Sets the endDateTime property value. DateTime when review instance is scheduled to end. The DatetimeOffset type represents date and time information using ISO 8601 format and is always in UTC time. For example, midnight UTC on Jan 1, 2014 is 2014-01-01T00:00:00Z. Supports $select. Read-only.
* @param value Value to set for the endDateTime property.
*/
public void setEndDateTime(@jakarta.annotation.Nullable final OffsetDateTime value) {
this.backingStore.set("endDateTime", value);
}
/**
* Sets the errors property value. Collection of errors in an access review instance lifecycle. Read-only.
* @param value Value to set for the errors property.
*/
public void setErrors(@jakarta.annotation.Nullable final java.util.List value) {
this.backingStore.set("errors", value);
}
/**
* Sets the fallbackReviewers property value. This collection of reviewer scopes is used to define the list of fallback reviewers. These fallback reviewers are notified to take action if no users are found from the list of reviewers specified. This could occur when either the group owner is specified as the reviewer but the group owner doesn't exist, or manager is specified as reviewer but a user's manager doesn't exist. Supports $select.
* @param value Value to set for the fallbackReviewers property.
*/
public void setFallbackReviewers(@jakarta.annotation.Nullable final java.util.List value) {
this.backingStore.set("fallbackReviewers", value);
}
/**
* Sets the reviewers property value. This collection of access review scopes is used to define who the reviewers are. Supports $select. For examples of options for assigning reviewers, see Assign reviewers to your access review definition using the Microsoft Graph API.
* @param value Value to set for the reviewers property.
*/
public void setReviewers(@jakarta.annotation.Nullable final java.util.List value) {
this.backingStore.set("reviewers", value);
}
/**
* Sets the scope property value. Created based on scope and instanceEnumerationScope at the accessReviewScheduleDefinition level. Defines the scope of users reviewed in a group. Supports $select and $filter (contains only). Read-only.
* @param value Value to set for the scope property.
*/
public void setScope(@jakarta.annotation.Nullable final AccessReviewScope value) {
this.backingStore.set("scope", value);
}
/**
* Sets the stages property value. If the instance has multiple stages, this returns the collection of stages. A new stage will only be created when the previous stage ends. The existence, number, and settings of stages on a review instance are created based on the accessReviewStageSettings on the parent accessReviewScheduleDefinition.
* @param value Value to set for the stages property.
*/
public void setStages(@jakarta.annotation.Nullable final java.util.List value) {
this.backingStore.set("stages", value);
}
/**
* Sets the startDateTime property value. DateTime when review instance is scheduled to start. May be in the future. The DateTimeOffset type represents date and time information using ISO 8601 format and is always in UTC time. For example, midnight UTC on Jan 1, 2014 is 2014-01-01T00:00:00Z. Supports $select. Read-only.
* @param value Value to set for the startDateTime property.
*/
public void setStartDateTime(@jakarta.annotation.Nullable final OffsetDateTime value) {
this.backingStore.set("startDateTime", value);
}
/**
* Sets the status property value. Specifies the status of an accessReview. Possible values: Initializing, NotStarted, Starting, InProgress, Completing, Completed, AutoReviewing, and AutoReviewed. Supports $select, $orderby, and $filter (eq only). Read-only.
* @param value Value to set for the status property.
*/
public void setStatus(@jakarta.annotation.Nullable final String value) {
this.backingStore.set("status", value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy