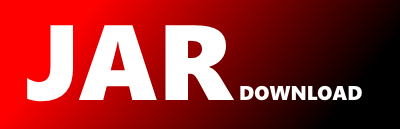
com.microsoft.graph.beta.generated.models.ManagedDeviceWindowsOperatingSystemEdition Maven / Gradle / Ivy
package com.microsoft.graph.beta.models;
import com.microsoft.kiota.serialization.AdditionalDataHolder;
import com.microsoft.kiota.serialization.Parsable;
import com.microsoft.kiota.serialization.ParseNode;
import com.microsoft.kiota.serialization.SerializationWriter;
import com.microsoft.kiota.store.BackedModel;
import com.microsoft.kiota.store.BackingStore;
import com.microsoft.kiota.store.BackingStoreFactorySingleton;
import java.time.LocalDate;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
/**
* Different Windows' versions have Edition specific support timelines. This complex type defines the date until which a particular edition is supported in a Windows' version.
*/
@jakarta.annotation.Generated("com.microsoft.kiota")
public class ManagedDeviceWindowsOperatingSystemEdition implements AdditionalDataHolder, BackedModel, Parsable {
/**
* Stores model information.
*/
@jakarta.annotation.Nonnull
protected BackingStore backingStore;
/**
* Instantiates a new {@link ManagedDeviceWindowsOperatingSystemEdition} and sets the default values.
*/
public ManagedDeviceWindowsOperatingSystemEdition() {
this.backingStore = BackingStoreFactorySingleton.instance.createBackingStore();
this.setAdditionalData(new HashMap<>());
}
/**
* Creates a new instance of the appropriate class based on discriminator value
* @param parseNode The parse node to use to read the discriminator value and create the object
* @return a {@link ManagedDeviceWindowsOperatingSystemEdition}
*/
@jakarta.annotation.Nonnull
public static ManagedDeviceWindowsOperatingSystemEdition createFromDiscriminatorValue(@jakarta.annotation.Nonnull final ParseNode parseNode) {
Objects.requireNonNull(parseNode);
return new ManagedDeviceWindowsOperatingSystemEdition();
}
/**
* Gets the AdditionalData property value. Stores additional data not described in the OpenAPI description found when deserializing. Can be used for serialization as well.
* @return a {@link Map}
*/
@jakarta.annotation.Nonnull
public Map getAdditionalData() {
Map value = this.backingStore.get("additionalData");
if(value == null) {
value = new HashMap<>();
this.setAdditionalData(value);
}
return value;
}
/**
* Gets the backingStore property value. Stores model information.
* @return a {@link BackingStore}
*/
@jakarta.annotation.Nonnull
public BackingStore getBackingStore() {
return this.backingStore;
}
/**
* Gets the editionType property value. Windows Operating System is available in different editions, which have a specific set of features available. This enum type defines the corresponding edition.
* @return a {@link ManagedDeviceWindowsOperatingSystemEditionType}
*/
@jakarta.annotation.Nullable
public ManagedDeviceWindowsOperatingSystemEditionType getEditionType() {
return this.backingStore.get("editionType");
}
/**
* The deserialization information for the current model
* @return a {@link Map>}
*/
@jakarta.annotation.Nonnull
public Map> getFieldDeserializers() {
final HashMap> deserializerMap = new HashMap>(3);
deserializerMap.put("editionType", (n) -> { this.setEditionType(n.getEnumValue(ManagedDeviceWindowsOperatingSystemEditionType::forValue)); });
deserializerMap.put("@odata.type", (n) -> { this.setOdataType(n.getStringValue()); });
deserializerMap.put("supportEndDate", (n) -> { this.setSupportEndDate(n.getLocalDateValue()); });
return deserializerMap;
}
/**
* Gets the @odata.type property value. The OdataType property
* @return a {@link String}
*/
@jakarta.annotation.Nullable
public String getOdataType() {
return this.backingStore.get("odataType");
}
/**
* Gets the supportEndDate property value. Indicates the Date until which this Operating System edition type is officially supported. The Timestamp type represents date and time information using ISO 8601 format and is always in Pacific Time Zone (PT). For example, 2014-01-01 would mean '2014-01-01T07:00:00Z' in UTC time. Returned by default. Read-only.
* @return a {@link LocalDate}
*/
@jakarta.annotation.Nullable
public LocalDate getSupportEndDate() {
return this.backingStore.get("supportEndDate");
}
/**
* Serializes information the current object
* @param writer Serialization writer to use to serialize this model
*/
public void serialize(@jakarta.annotation.Nonnull final SerializationWriter writer) {
Objects.requireNonNull(writer);
writer.writeEnumValue("editionType", this.getEditionType());
writer.writeStringValue("@odata.type", this.getOdataType());
writer.writeLocalDateValue("supportEndDate", this.getSupportEndDate());
writer.writeAdditionalData(this.getAdditionalData());
}
/**
* Sets the AdditionalData property value. Stores additional data not described in the OpenAPI description found when deserializing. Can be used for serialization as well.
* @param value Value to set for the AdditionalData property.
*/
public void setAdditionalData(@jakarta.annotation.Nullable final Map value) {
this.backingStore.set("additionalData", value);
}
/**
* Sets the backingStore property value. Stores model information.
* @param value Value to set for the backingStore property.
*/
public void setBackingStore(@jakarta.annotation.Nonnull final BackingStore value) {
Objects.requireNonNull(value);
this.backingStore = value;
}
/**
* Sets the editionType property value. Windows Operating System is available in different editions, which have a specific set of features available. This enum type defines the corresponding edition.
* @param value Value to set for the editionType property.
*/
public void setEditionType(@jakarta.annotation.Nullable final ManagedDeviceWindowsOperatingSystemEditionType value) {
this.backingStore.set("editionType", value);
}
/**
* Sets the @odata.type property value. The OdataType property
* @param value Value to set for the @odata.type property.
*/
public void setOdataType(@jakarta.annotation.Nullable final String value) {
this.backingStore.set("odataType", value);
}
/**
* Sets the supportEndDate property value. Indicates the Date until which this Operating System edition type is officially supported. The Timestamp type represents date and time information using ISO 8601 format and is always in Pacific Time Zone (PT). For example, 2014-01-01 would mean '2014-01-01T07:00:00Z' in UTC time. Returned by default. Read-only.
* @param value Value to set for the supportEndDate property.
*/
public void setSupportEndDate(@jakarta.annotation.Nullable final LocalDate value) {
this.backingStore.set("supportEndDate", value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy