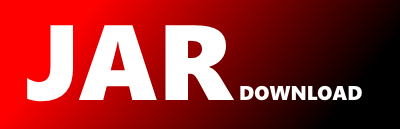
com.microsoft.graph.beta.generated.models.PrintJob Maven / Gradle / Ivy
package com.microsoft.graph.beta.models;
import com.microsoft.kiota.serialization.Parsable;
import com.microsoft.kiota.serialization.ParseNode;
import com.microsoft.kiota.serialization.SerializationWriter;
import java.time.OffsetDateTime;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
@jakarta.annotation.Generated("com.microsoft.kiota")
public class PrintJob extends Entity implements Parsable {
/**
* Instantiates a new {@link PrintJob} and sets the default values.
*/
public PrintJob() {
super();
}
/**
* Creates a new instance of the appropriate class based on discriminator value
* @param parseNode The parse node to use to read the discriminator value and create the object
* @return a {@link PrintJob}
*/
@jakarta.annotation.Nonnull
public static PrintJob createFromDiscriminatorValue(@jakarta.annotation.Nonnull final ParseNode parseNode) {
Objects.requireNonNull(parseNode);
return new PrintJob();
}
/**
* Gets the acknowledgedDateTime property value. The acknowledgedDateTime property
* @return a {@link OffsetDateTime}
*/
@jakarta.annotation.Nullable
public OffsetDateTime getAcknowledgedDateTime() {
return this.backingStore.get("acknowledgedDateTime");
}
/**
* Gets the completedDateTime property value. The completedDateTime property
* @return a {@link OffsetDateTime}
*/
@jakarta.annotation.Nullable
public OffsetDateTime getCompletedDateTime() {
return this.backingStore.get("completedDateTime");
}
/**
* Gets the configuration property value. The configuration property
* @return a {@link PrintJobConfiguration}
*/
@jakarta.annotation.Nullable
public PrintJobConfiguration getConfiguration() {
return this.backingStore.get("configuration");
}
/**
* Gets the createdBy property value. The createdBy property
* @return a {@link UserIdentity}
*/
@jakarta.annotation.Nullable
public UserIdentity getCreatedBy() {
return this.backingStore.get("createdBy");
}
/**
* Gets the createdDateTime property value. The DateTimeOffset when the job was created. Read-only.
* @return a {@link OffsetDateTime}
*/
@jakarta.annotation.Nullable
public OffsetDateTime getCreatedDateTime() {
return this.backingStore.get("createdDateTime");
}
/**
* Gets the displayName property value. The name of the print job.
* @return a {@link String}
*/
@jakarta.annotation.Nullable
public String getDisplayName() {
return this.backingStore.get("displayName");
}
/**
* Gets the documents property value. The documents property
* @return a {@link java.util.List}
*/
@jakarta.annotation.Nullable
public java.util.List getDocuments() {
return this.backingStore.get("documents");
}
/**
* Gets the errorCode property value. The errorCode property
* @return a {@link Integer}
*/
@jakarta.annotation.Nullable
public Integer getErrorCode() {
return this.backingStore.get("errorCode");
}
/**
* The deserialization information for the current model
* @return a {@link Map>}
*/
@jakarta.annotation.Nonnull
public Map> getFieldDeserializers() {
final HashMap> deserializerMap = new HashMap>(super.getFieldDeserializers());
deserializerMap.put("acknowledgedDateTime", (n) -> { this.setAcknowledgedDateTime(n.getOffsetDateTimeValue()); });
deserializerMap.put("completedDateTime", (n) -> { this.setCompletedDateTime(n.getOffsetDateTimeValue()); });
deserializerMap.put("configuration", (n) -> { this.setConfiguration(n.getObjectValue(PrintJobConfiguration::createFromDiscriminatorValue)); });
deserializerMap.put("createdBy", (n) -> { this.setCreatedBy(n.getObjectValue(UserIdentity::createFromDiscriminatorValue)); });
deserializerMap.put("createdDateTime", (n) -> { this.setCreatedDateTime(n.getOffsetDateTimeValue()); });
deserializerMap.put("displayName", (n) -> { this.setDisplayName(n.getStringValue()); });
deserializerMap.put("documents", (n) -> { this.setDocuments(n.getCollectionOfObjectValues(PrintDocument::createFromDiscriminatorValue)); });
deserializerMap.put("errorCode", (n) -> { this.setErrorCode(n.getIntegerValue()); });
deserializerMap.put("isFetchable", (n) -> { this.setIsFetchable(n.getBooleanValue()); });
deserializerMap.put("redirectedFrom", (n) -> { this.setRedirectedFrom(n.getStringValue()); });
deserializerMap.put("redirectedTo", (n) -> { this.setRedirectedTo(n.getStringValue()); });
deserializerMap.put("status", (n) -> { this.setStatus(n.getObjectValue(PrintJobStatus::createFromDiscriminatorValue)); });
deserializerMap.put("tasks", (n) -> { this.setTasks(n.getCollectionOfObjectValues(PrintTask::createFromDiscriminatorValue)); });
return deserializerMap;
}
/**
* Gets the isFetchable property value. If true, document can be fetched by printer.
* @return a {@link Boolean}
*/
@jakarta.annotation.Nullable
public Boolean getIsFetchable() {
return this.backingStore.get("isFetchable");
}
/**
* Gets the redirectedFrom property value. Contains the source job URL, if the job has been redirected from another printer.
* @return a {@link String}
*/
@jakarta.annotation.Nullable
public String getRedirectedFrom() {
return this.backingStore.get("redirectedFrom");
}
/**
* Gets the redirectedTo property value. Contains the destination job URL, if the job has been redirected to another printer.
* @return a {@link String}
*/
@jakarta.annotation.Nullable
public String getRedirectedTo() {
return this.backingStore.get("redirectedTo");
}
/**
* Gets the status property value. The status property
* @return a {@link PrintJobStatus}
*/
@jakarta.annotation.Nullable
public PrintJobStatus getStatus() {
return this.backingStore.get("status");
}
/**
* Gets the tasks property value. A list of printTasks that were triggered by this print job.
* @return a {@link java.util.List}
*/
@jakarta.annotation.Nullable
public java.util.List getTasks() {
return this.backingStore.get("tasks");
}
/**
* Serializes information the current object
* @param writer Serialization writer to use to serialize this model
*/
public void serialize(@jakarta.annotation.Nonnull final SerializationWriter writer) {
Objects.requireNonNull(writer);
super.serialize(writer);
writer.writeOffsetDateTimeValue("acknowledgedDateTime", this.getAcknowledgedDateTime());
writer.writeOffsetDateTimeValue("completedDateTime", this.getCompletedDateTime());
writer.writeObjectValue("configuration", this.getConfiguration());
writer.writeObjectValue("createdBy", this.getCreatedBy());
writer.writeOffsetDateTimeValue("createdDateTime", this.getCreatedDateTime());
writer.writeStringValue("displayName", this.getDisplayName());
writer.writeCollectionOfObjectValues("documents", this.getDocuments());
writer.writeIntegerValue("errorCode", this.getErrorCode());
writer.writeBooleanValue("isFetchable", this.getIsFetchable());
writer.writeStringValue("redirectedFrom", this.getRedirectedFrom());
writer.writeStringValue("redirectedTo", this.getRedirectedTo());
writer.writeObjectValue("status", this.getStatus());
writer.writeCollectionOfObjectValues("tasks", this.getTasks());
}
/**
* Sets the acknowledgedDateTime property value. The acknowledgedDateTime property
* @param value Value to set for the acknowledgedDateTime property.
*/
public void setAcknowledgedDateTime(@jakarta.annotation.Nullable final OffsetDateTime value) {
this.backingStore.set("acknowledgedDateTime", value);
}
/**
* Sets the completedDateTime property value. The completedDateTime property
* @param value Value to set for the completedDateTime property.
*/
public void setCompletedDateTime(@jakarta.annotation.Nullable final OffsetDateTime value) {
this.backingStore.set("completedDateTime", value);
}
/**
* Sets the configuration property value. The configuration property
* @param value Value to set for the configuration property.
*/
public void setConfiguration(@jakarta.annotation.Nullable final PrintJobConfiguration value) {
this.backingStore.set("configuration", value);
}
/**
* Sets the createdBy property value. The createdBy property
* @param value Value to set for the createdBy property.
*/
public void setCreatedBy(@jakarta.annotation.Nullable final UserIdentity value) {
this.backingStore.set("createdBy", value);
}
/**
* Sets the createdDateTime property value. The DateTimeOffset when the job was created. Read-only.
* @param value Value to set for the createdDateTime property.
*/
public void setCreatedDateTime(@jakarta.annotation.Nullable final OffsetDateTime value) {
this.backingStore.set("createdDateTime", value);
}
/**
* Sets the displayName property value. The name of the print job.
* @param value Value to set for the displayName property.
*/
public void setDisplayName(@jakarta.annotation.Nullable final String value) {
this.backingStore.set("displayName", value);
}
/**
* Sets the documents property value. The documents property
* @param value Value to set for the documents property.
*/
public void setDocuments(@jakarta.annotation.Nullable final java.util.List value) {
this.backingStore.set("documents", value);
}
/**
* Sets the errorCode property value. The errorCode property
* @param value Value to set for the errorCode property.
*/
public void setErrorCode(@jakarta.annotation.Nullable final Integer value) {
this.backingStore.set("errorCode", value);
}
/**
* Sets the isFetchable property value. If true, document can be fetched by printer.
* @param value Value to set for the isFetchable property.
*/
public void setIsFetchable(@jakarta.annotation.Nullable final Boolean value) {
this.backingStore.set("isFetchable", value);
}
/**
* Sets the redirectedFrom property value. Contains the source job URL, if the job has been redirected from another printer.
* @param value Value to set for the redirectedFrom property.
*/
public void setRedirectedFrom(@jakarta.annotation.Nullable final String value) {
this.backingStore.set("redirectedFrom", value);
}
/**
* Sets the redirectedTo property value. Contains the destination job URL, if the job has been redirected to another printer.
* @param value Value to set for the redirectedTo property.
*/
public void setRedirectedTo(@jakarta.annotation.Nullable final String value) {
this.backingStore.set("redirectedTo", value);
}
/**
* Sets the status property value. The status property
* @param value Value to set for the status property.
*/
public void setStatus(@jakarta.annotation.Nullable final PrintJobStatus value) {
this.backingStore.set("status", value);
}
/**
* Sets the tasks property value. A list of printTasks that were triggered by this print job.
* @param value Value to set for the tasks property.
*/
public void setTasks(@jakarta.annotation.Nullable final java.util.List value) {
this.backingStore.set("tasks", value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy