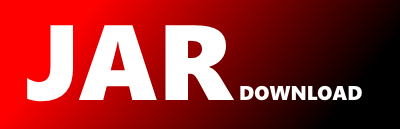
com.microsoft.graph.beta.generated.models.DefaultUserRolePermissions Maven / Gradle / Ivy
package com.microsoft.graph.beta.models;
import com.microsoft.kiota.serialization.AdditionalDataHolder;
import com.microsoft.kiota.serialization.Parsable;
import com.microsoft.kiota.serialization.ParseNode;
import com.microsoft.kiota.serialization.SerializationWriter;
import com.microsoft.kiota.store.BackedModel;
import com.microsoft.kiota.store.BackingStore;
import com.microsoft.kiota.store.BackingStoreFactorySingleton;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
@jakarta.annotation.Generated("com.microsoft.kiota")
public class DefaultUserRolePermissions implements AdditionalDataHolder, BackedModel, Parsable {
/**
* Stores model information.
*/
@jakarta.annotation.Nonnull
protected BackingStore backingStore;
/**
* Instantiates a new {@link DefaultUserRolePermissions} and sets the default values.
*/
public DefaultUserRolePermissions() {
this.backingStore = BackingStoreFactorySingleton.instance.createBackingStore();
this.setAdditionalData(new HashMap<>());
}
/**
* Creates a new instance of the appropriate class based on discriminator value
* @param parseNode The parse node to use to read the discriminator value and create the object
* @return a {@link DefaultUserRolePermissions}
*/
@jakarta.annotation.Nonnull
public static DefaultUserRolePermissions createFromDiscriminatorValue(@jakarta.annotation.Nonnull final ParseNode parseNode) {
Objects.requireNonNull(parseNode);
return new DefaultUserRolePermissions();
}
/**
* Gets the AdditionalData property value. Stores additional data not described in the OpenAPI description found when deserializing. Can be used for serialization as well.
* @return a {@link Map}
*/
@jakarta.annotation.Nonnull
public Map getAdditionalData() {
Map value = this.backingStore.get("additionalData");
if(value == null) {
value = new HashMap<>();
this.setAdditionalData(value);
}
return value;
}
/**
* Gets the allowedToCreateApps property value. Indicates whether the default user role can create applications. This setting corresponds to the Users can register applications setting in the User settings menu in the Microsoft Entra admin center.
* @return a {@link Boolean}
*/
@jakarta.annotation.Nullable
public Boolean getAllowedToCreateApps() {
return this.backingStore.get("allowedToCreateApps");
}
/**
* Gets the allowedToCreateSecurityGroups property value. Indicates whether the default user role can create security groups. This setting corresponds to the following menus in the Microsoft Entra admin center: The Users can create security groups in Microsoft Entra admin centers, API or PowerShell setting in the Group settings menu. Users can create security groups setting in the User settings menu.
* @return a {@link Boolean}
*/
@jakarta.annotation.Nullable
public Boolean getAllowedToCreateSecurityGroups() {
return this.backingStore.get("allowedToCreateSecurityGroups");
}
/**
* Gets the allowedToCreateTenants property value. Indicates whether the default user role can create tenants. This setting corresponds to the Restrict non-admin users from creating tenants setting in the User settings menu in the Microsoft Entra admin center. When this setting is false, users assigned the Tenant Creator role can still create tenants.
* @return a {@link Boolean}
*/
@jakarta.annotation.Nullable
public Boolean getAllowedToCreateTenants() {
return this.backingStore.get("allowedToCreateTenants");
}
/**
* Gets the allowedToReadBitlockerKeysForOwnedDevice property value. Indicates whether the registered owners of a device can read their own BitLocker recovery keys with default user role.
* @return a {@link Boolean}
*/
@jakarta.annotation.Nullable
public Boolean getAllowedToReadBitlockerKeysForOwnedDevice() {
return this.backingStore.get("allowedToReadBitlockerKeysForOwnedDevice");
}
/**
* Gets the allowedToReadOtherUsers property value. Indicates whether the default user role can read other users. DO NOT SET THIS VALUE TO false.
* @return a {@link Boolean}
*/
@jakarta.annotation.Nullable
public Boolean getAllowedToReadOtherUsers() {
return this.backingStore.get("allowedToReadOtherUsers");
}
/**
* Gets the backingStore property value. Stores model information.
* @return a {@link BackingStore}
*/
@jakarta.annotation.Nonnull
public BackingStore getBackingStore() {
return this.backingStore;
}
/**
* The deserialization information for the current model
* @return a {@link Map>}
*/
@jakarta.annotation.Nonnull
public Map> getFieldDeserializers() {
final HashMap> deserializerMap = new HashMap>(6);
deserializerMap.put("allowedToCreateApps", (n) -> { this.setAllowedToCreateApps(n.getBooleanValue()); });
deserializerMap.put("allowedToCreateSecurityGroups", (n) -> { this.setAllowedToCreateSecurityGroups(n.getBooleanValue()); });
deserializerMap.put("allowedToCreateTenants", (n) -> { this.setAllowedToCreateTenants(n.getBooleanValue()); });
deserializerMap.put("allowedToReadBitlockerKeysForOwnedDevice", (n) -> { this.setAllowedToReadBitlockerKeysForOwnedDevice(n.getBooleanValue()); });
deserializerMap.put("allowedToReadOtherUsers", (n) -> { this.setAllowedToReadOtherUsers(n.getBooleanValue()); });
deserializerMap.put("@odata.type", (n) -> { this.setOdataType(n.getStringValue()); });
return deserializerMap;
}
/**
* Gets the @odata.type property value. The OdataType property
* @return a {@link String}
*/
@jakarta.annotation.Nullable
public String getOdataType() {
return this.backingStore.get("odataType");
}
/**
* Serializes information the current object
* @param writer Serialization writer to use to serialize this model
*/
public void serialize(@jakarta.annotation.Nonnull final SerializationWriter writer) {
Objects.requireNonNull(writer);
writer.writeBooleanValue("allowedToCreateApps", this.getAllowedToCreateApps());
writer.writeBooleanValue("allowedToCreateSecurityGroups", this.getAllowedToCreateSecurityGroups());
writer.writeBooleanValue("allowedToCreateTenants", this.getAllowedToCreateTenants());
writer.writeBooleanValue("allowedToReadBitlockerKeysForOwnedDevice", this.getAllowedToReadBitlockerKeysForOwnedDevice());
writer.writeBooleanValue("allowedToReadOtherUsers", this.getAllowedToReadOtherUsers());
writer.writeStringValue("@odata.type", this.getOdataType());
writer.writeAdditionalData(this.getAdditionalData());
}
/**
* Sets the AdditionalData property value. Stores additional data not described in the OpenAPI description found when deserializing. Can be used for serialization as well.
* @param value Value to set for the AdditionalData property.
*/
public void setAdditionalData(@jakarta.annotation.Nullable final Map value) {
this.backingStore.set("additionalData", value);
}
/**
* Sets the allowedToCreateApps property value. Indicates whether the default user role can create applications. This setting corresponds to the Users can register applications setting in the User settings menu in the Microsoft Entra admin center.
* @param value Value to set for the allowedToCreateApps property.
*/
public void setAllowedToCreateApps(@jakarta.annotation.Nullable final Boolean value) {
this.backingStore.set("allowedToCreateApps", value);
}
/**
* Sets the allowedToCreateSecurityGroups property value. Indicates whether the default user role can create security groups. This setting corresponds to the following menus in the Microsoft Entra admin center: The Users can create security groups in Microsoft Entra admin centers, API or PowerShell setting in the Group settings menu. Users can create security groups setting in the User settings menu.
* @param value Value to set for the allowedToCreateSecurityGroups property.
*/
public void setAllowedToCreateSecurityGroups(@jakarta.annotation.Nullable final Boolean value) {
this.backingStore.set("allowedToCreateSecurityGroups", value);
}
/**
* Sets the allowedToCreateTenants property value. Indicates whether the default user role can create tenants. This setting corresponds to the Restrict non-admin users from creating tenants setting in the User settings menu in the Microsoft Entra admin center. When this setting is false, users assigned the Tenant Creator role can still create tenants.
* @param value Value to set for the allowedToCreateTenants property.
*/
public void setAllowedToCreateTenants(@jakarta.annotation.Nullable final Boolean value) {
this.backingStore.set("allowedToCreateTenants", value);
}
/**
* Sets the allowedToReadBitlockerKeysForOwnedDevice property value. Indicates whether the registered owners of a device can read their own BitLocker recovery keys with default user role.
* @param value Value to set for the allowedToReadBitlockerKeysForOwnedDevice property.
*/
public void setAllowedToReadBitlockerKeysForOwnedDevice(@jakarta.annotation.Nullable final Boolean value) {
this.backingStore.set("allowedToReadBitlockerKeysForOwnedDevice", value);
}
/**
* Sets the allowedToReadOtherUsers property value. Indicates whether the default user role can read other users. DO NOT SET THIS VALUE TO false.
* @param value Value to set for the allowedToReadOtherUsers property.
*/
public void setAllowedToReadOtherUsers(@jakarta.annotation.Nullable final Boolean value) {
this.backingStore.set("allowedToReadOtherUsers", value);
}
/**
* Sets the backingStore property value. Stores model information.
* @param value Value to set for the backingStore property.
*/
public void setBackingStore(@jakarta.annotation.Nonnull final BackingStore value) {
Objects.requireNonNull(value);
this.backingStore = value;
}
/**
* Sets the @odata.type property value. The OdataType property
* @param value Value to set for the @odata.type property.
*/
public void setOdataType(@jakarta.annotation.Nullable final String value) {
this.backingStore.set("odataType", value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy