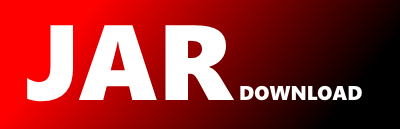
com.microsoft.graph.beta.generated.models.security.EdiscoverySearchExportOperation Maven / Gradle / Ivy
package com.microsoft.graph.beta.models.security;
import com.microsoft.kiota.serialization.Parsable;
import com.microsoft.kiota.serialization.ParseNode;
import com.microsoft.kiota.serialization.SerializationWriter;
import java.util.EnumSet;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
@jakarta.annotation.Generated("com.microsoft.kiota")
public class EdiscoverySearchExportOperation extends CaseOperation implements Parsable {
/**
* Instantiates a new {@link EdiscoverySearchExportOperation} and sets the default values.
*/
public EdiscoverySearchExportOperation() {
super();
}
/**
* Creates a new instance of the appropriate class based on discriminator value
* @param parseNode The parse node to use to read the discriminator value and create the object
* @return a {@link EdiscoverySearchExportOperation}
*/
@jakarta.annotation.Nonnull
public static EdiscoverySearchExportOperation createFromDiscriminatorValue(@jakarta.annotation.Nonnull final ParseNode parseNode) {
Objects.requireNonNull(parseNode);
return new EdiscoverySearchExportOperation();
}
/**
* Gets the additionalOptions property value. The additional items to include in the export. The possible values are: none, teamsAndYammerConversations, cloudAttachments, allDocumentVersions, subfolderContents, listAttachments, unknownFutureValue.
* @return a {@link EnumSet}
*/
@jakarta.annotation.Nullable
public EnumSet getAdditionalOptions() {
return this.backingStore.get("additionalOptions");
}
/**
* Gets the description property value. The description of the export by the user.
* @return a {@link String}
*/
@jakarta.annotation.Nullable
public String getDescription() {
return this.backingStore.get("description");
}
/**
* Gets the displayName property value. The name of export provided by the user.
* @return a {@link String}
*/
@jakarta.annotation.Nullable
public String getDisplayName() {
return this.backingStore.get("displayName");
}
/**
* Gets the exportCriteria property value. Items to be included in the export. The possible values are: searchHits, partiallyIndexed, unknownFutureValue.
* @return a {@link EnumSet}
*/
@jakarta.annotation.Nullable
public EnumSet getExportCriteria() {
return this.backingStore.get("exportCriteria");
}
/**
* Gets the exportFileMetadata property value. Contains the properties for an export file metadata, including downloadUrl, fileName, and size.
* @return a {@link java.util.List}
*/
@jakarta.annotation.Nullable
public java.util.List getExportFileMetadata() {
return this.backingStore.get("exportFileMetadata");
}
/**
* Gets the exportFormat property value. Format of the emails of the export. The possible values are: pst, msg, eml, unknownFutureValue.
* @return a {@link ExportFormat}
*/
@jakarta.annotation.Nullable
public ExportFormat getExportFormat() {
return this.backingStore.get("exportFormat");
}
/**
* Gets the exportLocation property value. Location scope for partially indexed items. You can choose to include partially indexed items only in responsive locations with search hits or in all targeted locations. The possible values are: responsiveLocations, nonresponsiveLocations, unknownFutureValue.
* @return a {@link EnumSet}
*/
@jakarta.annotation.Nullable
public EnumSet getExportLocation() {
return this.backingStore.get("exportLocation");
}
/**
* Gets the exportSingleItems property value. Indicates whether to export single items.
* @return a {@link Boolean}
*/
@jakarta.annotation.Nullable
public Boolean getExportSingleItems() {
return this.backingStore.get("exportSingleItems");
}
/**
* The deserialization information for the current model
* @return a {@link Map>}
*/
@jakarta.annotation.Nonnull
public Map> getFieldDeserializers() {
final HashMap> deserializerMap = new HashMap>(super.getFieldDeserializers());
deserializerMap.put("additionalOptions", (n) -> { this.setAdditionalOptions(n.getEnumSetValue(AdditionalOptions::forValue)); });
deserializerMap.put("description", (n) -> { this.setDescription(n.getStringValue()); });
deserializerMap.put("displayName", (n) -> { this.setDisplayName(n.getStringValue()); });
deserializerMap.put("exportCriteria", (n) -> { this.setExportCriteria(n.getEnumSetValue(ExportCriteria::forValue)); });
deserializerMap.put("exportFileMetadata", (n) -> { this.setExportFileMetadata(n.getCollectionOfObjectValues(ExportFileMetadata::createFromDiscriminatorValue)); });
deserializerMap.put("exportFormat", (n) -> { this.setExportFormat(n.getEnumValue(ExportFormat::forValue)); });
deserializerMap.put("exportLocation", (n) -> { this.setExportLocation(n.getEnumSetValue(ExportLocation::forValue)); });
deserializerMap.put("exportSingleItems", (n) -> { this.setExportSingleItems(n.getBooleanValue()); });
deserializerMap.put("search", (n) -> { this.setSearch(n.getObjectValue(EdiscoverySearch::createFromDiscriminatorValue)); });
return deserializerMap;
}
/**
* Gets the search property value. The eDiscovery searches under each case.
* @return a {@link EdiscoverySearch}
*/
@jakarta.annotation.Nullable
public EdiscoverySearch getSearch() {
return this.backingStore.get("search");
}
/**
* Serializes information the current object
* @param writer Serialization writer to use to serialize this model
*/
public void serialize(@jakarta.annotation.Nonnull final SerializationWriter writer) {
Objects.requireNonNull(writer);
super.serialize(writer);
writer.writeEnumSetValue("additionalOptions", this.getAdditionalOptions());
writer.writeStringValue("description", this.getDescription());
writer.writeStringValue("displayName", this.getDisplayName());
writer.writeEnumSetValue("exportCriteria", this.getExportCriteria());
writer.writeCollectionOfObjectValues("exportFileMetadata", this.getExportFileMetadata());
writer.writeEnumValue("exportFormat", this.getExportFormat());
writer.writeEnumSetValue("exportLocation", this.getExportLocation());
writer.writeBooleanValue("exportSingleItems", this.getExportSingleItems());
writer.writeObjectValue("search", this.getSearch());
}
/**
* Sets the additionalOptions property value. The additional items to include in the export. The possible values are: none, teamsAndYammerConversations, cloudAttachments, allDocumentVersions, subfolderContents, listAttachments, unknownFutureValue.
* @param value Value to set for the additionalOptions property.
*/
public void setAdditionalOptions(@jakarta.annotation.Nullable final EnumSet value) {
this.backingStore.set("additionalOptions", value);
}
/**
* Sets the description property value. The description of the export by the user.
* @param value Value to set for the description property.
*/
public void setDescription(@jakarta.annotation.Nullable final String value) {
this.backingStore.set("description", value);
}
/**
* Sets the displayName property value. The name of export provided by the user.
* @param value Value to set for the displayName property.
*/
public void setDisplayName(@jakarta.annotation.Nullable final String value) {
this.backingStore.set("displayName", value);
}
/**
* Sets the exportCriteria property value. Items to be included in the export. The possible values are: searchHits, partiallyIndexed, unknownFutureValue.
* @param value Value to set for the exportCriteria property.
*/
public void setExportCriteria(@jakarta.annotation.Nullable final EnumSet value) {
this.backingStore.set("exportCriteria", value);
}
/**
* Sets the exportFileMetadata property value. Contains the properties for an export file metadata, including downloadUrl, fileName, and size.
* @param value Value to set for the exportFileMetadata property.
*/
public void setExportFileMetadata(@jakarta.annotation.Nullable final java.util.List value) {
this.backingStore.set("exportFileMetadata", value);
}
/**
* Sets the exportFormat property value. Format of the emails of the export. The possible values are: pst, msg, eml, unknownFutureValue.
* @param value Value to set for the exportFormat property.
*/
public void setExportFormat(@jakarta.annotation.Nullable final ExportFormat value) {
this.backingStore.set("exportFormat", value);
}
/**
* Sets the exportLocation property value. Location scope for partially indexed items. You can choose to include partially indexed items only in responsive locations with search hits or in all targeted locations. The possible values are: responsiveLocations, nonresponsiveLocations, unknownFutureValue.
* @param value Value to set for the exportLocation property.
*/
public void setExportLocation(@jakarta.annotation.Nullable final EnumSet value) {
this.backingStore.set("exportLocation", value);
}
/**
* Sets the exportSingleItems property value. Indicates whether to export single items.
* @param value Value to set for the exportSingleItems property.
*/
public void setExportSingleItems(@jakarta.annotation.Nullable final Boolean value) {
this.backingStore.set("exportSingleItems", value);
}
/**
* Sets the search property value. The eDiscovery searches under each case.
* @param value Value to set for the search property.
*/
public void setSearch(@jakarta.annotation.Nullable final EdiscoverySearch value) {
this.backingStore.set("search", value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy