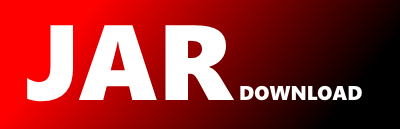
com.microsoft.graph.generated.models.WindowsAutopilotDeploymentProfile Maven / Gradle / Ivy
package com.microsoft.graph.models;
import com.microsoft.kiota.serialization.Parsable;
import com.microsoft.kiota.serialization.ParseNode;
import com.microsoft.kiota.serialization.SerializationWriter;
import java.time.OffsetDateTime;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
/**
* Windows Autopilot Deployment Profile
*/
@jakarta.annotation.Generated("com.microsoft.kiota")
public class WindowsAutopilotDeploymentProfile extends Entity implements Parsable {
/**
* Instantiates a new {@link WindowsAutopilotDeploymentProfile} and sets the default values.
*/
public WindowsAutopilotDeploymentProfile() {
super();
}
/**
* Creates a new instance of the appropriate class based on discriminator value
* @param parseNode The parse node to use to read the discriminator value and create the object
* @return a {@link WindowsAutopilotDeploymentProfile}
*/
@jakarta.annotation.Nonnull
public static WindowsAutopilotDeploymentProfile createFromDiscriminatorValue(@jakarta.annotation.Nonnull final ParseNode parseNode) {
Objects.requireNonNull(parseNode);
return new WindowsAutopilotDeploymentProfile();
}
/**
* Gets the assignedDevices property value. The list of assigned devices for the profile.
* @return a {@link java.util.List}
*/
@jakarta.annotation.Nullable
public java.util.List getAssignedDevices() {
return this.backingStore.get("assignedDevices");
}
/**
* Gets the createdDateTime property value. The date and time of when the deployment profile was created. The value cannot be modified and is automatically populated when the profile was created. The timestamp type represents date and time information using ISO 8601 format and is always in UTC time. For example, midnight UTC on Jan 1, 2014 would look like this: '2014-01-01T00:00:00Z'. Supports: $select, $top, $skip. $Search, $orderBy and $filter are not supported. Read-Only.
* @return a {@link OffsetDateTime}
*/
@jakarta.annotation.Nullable
public OffsetDateTime getCreatedDateTime() {
return this.backingStore.get("createdDateTime");
}
/**
* Gets the description property value. A description of the deployment profile. Max allowed length is 1500 chars. Supports: $select, $top, $skip, $orderBy. $Search and $filter are not supported.
* @return a {@link String}
*/
@jakarta.annotation.Nullable
public String getDescription() {
return this.backingStore.get("description");
}
/**
* Gets the deviceNameTemplate property value. The template used to name the Autopilot device. This can be a custom text and can also contain either the serial number of the device, or a randomly generated number. The total length of the text generated by the template can be no more than 15 characters. Supports: $select, $top, $skip. $Search, $orderBy and $filter are not supported.
* @return a {@link String}
*/
@jakarta.annotation.Nullable
public String getDeviceNameTemplate() {
return this.backingStore.get("deviceNameTemplate");
}
/**
* Gets the deviceType property value. The deviceType property
* @return a {@link WindowsAutopilotDeviceType}
*/
@jakarta.annotation.Nullable
public WindowsAutopilotDeviceType getDeviceType() {
return this.backingStore.get("deviceType");
}
/**
* Gets the displayName property value. The display name of the deployment profile. Max allowed length is 200 chars. Returned by default. Supports: $select, $top, $skip, $orderby. $Search and $filter are not supported.
* @return a {@link String}
*/
@jakarta.annotation.Nullable
public String getDisplayName() {
return this.backingStore.get("displayName");
}
/**
* The deserialization information for the current model
* @return a {@link Map>}
*/
@jakarta.annotation.Nonnull
public Map> getFieldDeserializers() {
final HashMap> deserializerMap = new HashMap>(super.getFieldDeserializers());
deserializerMap.put("assignedDevices", (n) -> { this.setAssignedDevices(n.getCollectionOfObjectValues(WindowsAutopilotDeviceIdentity::createFromDiscriminatorValue)); });
deserializerMap.put("createdDateTime", (n) -> { this.setCreatedDateTime(n.getOffsetDateTimeValue()); });
deserializerMap.put("description", (n) -> { this.setDescription(n.getStringValue()); });
deserializerMap.put("deviceNameTemplate", (n) -> { this.setDeviceNameTemplate(n.getStringValue()); });
deserializerMap.put("deviceType", (n) -> { this.setDeviceType(n.getEnumValue(WindowsAutopilotDeviceType::forValue)); });
deserializerMap.put("displayName", (n) -> { this.setDisplayName(n.getStringValue()); });
deserializerMap.put("hardwareHashExtractionEnabled", (n) -> { this.setHardwareHashExtractionEnabled(n.getBooleanValue()); });
deserializerMap.put("lastModifiedDateTime", (n) -> { this.setLastModifiedDateTime(n.getOffsetDateTimeValue()); });
deserializerMap.put("locale", (n) -> { this.setLocale(n.getStringValue()); });
deserializerMap.put("managementServiceAppId", (n) -> { this.setManagementServiceAppId(n.getStringValue()); });
deserializerMap.put("outOfBoxExperienceSetting", (n) -> { this.setOutOfBoxExperienceSetting(n.getObjectValue(OutOfBoxExperienceSetting::createFromDiscriminatorValue)); });
deserializerMap.put("preprovisioningAllowed", (n) -> { this.setPreprovisioningAllowed(n.getBooleanValue()); });
deserializerMap.put("roleScopeTagIds", (n) -> { this.setRoleScopeTagIds(n.getCollectionOfPrimitiveValues(String.class)); });
return deserializerMap;
}
/**
* Gets the hardwareHashExtractionEnabled property value. Indicates whether the profile supports the extraction of hardware hash values and registration of the device into Windows Autopilot. When TRUE, indicates if hardware extraction and Windows Autopilot registration will happen on the next successful check-in. When FALSE, hardware hash extraction and Windows Autopilot registration will not happen. Default value is FALSE. Supports: $select, $top, $skip. $Search, $orderBy and $filter are not supported.
* @return a {@link Boolean}
*/
@jakarta.annotation.Nullable
public Boolean getHardwareHashExtractionEnabled() {
return this.backingStore.get("hardwareHashExtractionEnabled");
}
/**
* Gets the lastModifiedDateTime property value. The date and time of when the deployment profile was last modified. The value cannot be updated manually and is automatically populated when any changes are made to the profile. The Timestamp type represents date and time information using ISO 8601 format and is always in UTC time. For example, midnight UTC on Jan 1, 2014 would look like this: '2014-01-01T00:00:00Z'. Supports: $select, $top, $skip. $Search, $orderBy and $filter are not supported Read-Only.
* @return a {@link OffsetDateTime}
*/
@jakarta.annotation.Nullable
public OffsetDateTime getLastModifiedDateTime() {
return this.backingStore.get("lastModifiedDateTime");
}
/**
* Gets the locale property value. The locale (language) to be used when configuring the device. E.g. en-US. The default value is os-default. Supports: $select, $top, $skip. $Search, $orderBy and $filter are not supported.
* @return a {@link String}
*/
@jakarta.annotation.Nullable
public String getLocale() {
return this.backingStore.get("locale");
}
/**
* Gets the managementServiceAppId property value. The Entra management service App ID which gets used during client device-based enrollment discovery. Supports: $select, $top, $skip. $Search, $orderBy and $filter are not supported.
* @return a {@link String}
*/
@jakarta.annotation.Nullable
public String getManagementServiceAppId() {
return this.backingStore.get("managementServiceAppId");
}
/**
* Gets the outOfBoxExperienceSetting property value. The Windows Autopilot Deployment Profile settings used by the device for the out-of-box experience. Supports: $select, $top, $skip. $Search, $orderBy and $filter are not supported.
* @return a {@link OutOfBoxExperienceSetting}
*/
@jakarta.annotation.Nullable
public OutOfBoxExperienceSetting getOutOfBoxExperienceSetting() {
return this.backingStore.get("outOfBoxExperienceSetting");
}
/**
* Gets the preprovisioningAllowed property value. Indicates whether the user is allowed to use Windows Autopilot for pre-provisioned deployment mode during Out of Box experience (OOBE). When TRUE, indicates that Windows Autopilot for pre-provisioned deployment mode for OOBE is allowed to be used. When false, Windows Autopilot for pre-provisioned deployment mode for OOBE is not allowed. The default is FALSE.
* @return a {@link Boolean}
*/
@jakarta.annotation.Nullable
public Boolean getPreprovisioningAllowed() {
return this.backingStore.get("preprovisioningAllowed");
}
/**
* Gets the roleScopeTagIds property value. List of role scope tags for the deployment profile.
* @return a {@link java.util.List}
*/
@jakarta.annotation.Nullable
public java.util.List getRoleScopeTagIds() {
return this.backingStore.get("roleScopeTagIds");
}
/**
* Serializes information the current object
* @param writer Serialization writer to use to serialize this model
*/
public void serialize(@jakarta.annotation.Nonnull final SerializationWriter writer) {
Objects.requireNonNull(writer);
super.serialize(writer);
writer.writeCollectionOfObjectValues("assignedDevices", this.getAssignedDevices());
writer.writeOffsetDateTimeValue("createdDateTime", this.getCreatedDateTime());
writer.writeStringValue("description", this.getDescription());
writer.writeStringValue("deviceNameTemplate", this.getDeviceNameTemplate());
writer.writeEnumValue("deviceType", this.getDeviceType());
writer.writeStringValue("displayName", this.getDisplayName());
writer.writeBooleanValue("hardwareHashExtractionEnabled", this.getHardwareHashExtractionEnabled());
writer.writeOffsetDateTimeValue("lastModifiedDateTime", this.getLastModifiedDateTime());
writer.writeStringValue("locale", this.getLocale());
writer.writeStringValue("managementServiceAppId", this.getManagementServiceAppId());
writer.writeObjectValue("outOfBoxExperienceSetting", this.getOutOfBoxExperienceSetting());
writer.writeBooleanValue("preprovisioningAllowed", this.getPreprovisioningAllowed());
writer.writeCollectionOfPrimitiveValues("roleScopeTagIds", this.getRoleScopeTagIds());
}
/**
* Sets the assignedDevices property value. The list of assigned devices for the profile.
* @param value Value to set for the assignedDevices property.
*/
public void setAssignedDevices(@jakarta.annotation.Nullable final java.util.List value) {
this.backingStore.set("assignedDevices", value);
}
/**
* Sets the createdDateTime property value. The date and time of when the deployment profile was created. The value cannot be modified and is automatically populated when the profile was created. The timestamp type represents date and time information using ISO 8601 format and is always in UTC time. For example, midnight UTC on Jan 1, 2014 would look like this: '2014-01-01T00:00:00Z'. Supports: $select, $top, $skip. $Search, $orderBy and $filter are not supported. Read-Only.
* @param value Value to set for the createdDateTime property.
*/
public void setCreatedDateTime(@jakarta.annotation.Nullable final OffsetDateTime value) {
this.backingStore.set("createdDateTime", value);
}
/**
* Sets the description property value. A description of the deployment profile. Max allowed length is 1500 chars. Supports: $select, $top, $skip, $orderBy. $Search and $filter are not supported.
* @param value Value to set for the description property.
*/
public void setDescription(@jakarta.annotation.Nullable final String value) {
this.backingStore.set("description", value);
}
/**
* Sets the deviceNameTemplate property value. The template used to name the Autopilot device. This can be a custom text and can also contain either the serial number of the device, or a randomly generated number. The total length of the text generated by the template can be no more than 15 characters. Supports: $select, $top, $skip. $Search, $orderBy and $filter are not supported.
* @param value Value to set for the deviceNameTemplate property.
*/
public void setDeviceNameTemplate(@jakarta.annotation.Nullable final String value) {
this.backingStore.set("deviceNameTemplate", value);
}
/**
* Sets the deviceType property value. The deviceType property
* @param value Value to set for the deviceType property.
*/
public void setDeviceType(@jakarta.annotation.Nullable final WindowsAutopilotDeviceType value) {
this.backingStore.set("deviceType", value);
}
/**
* Sets the displayName property value. The display name of the deployment profile. Max allowed length is 200 chars. Returned by default. Supports: $select, $top, $skip, $orderby. $Search and $filter are not supported.
* @param value Value to set for the displayName property.
*/
public void setDisplayName(@jakarta.annotation.Nullable final String value) {
this.backingStore.set("displayName", value);
}
/**
* Sets the hardwareHashExtractionEnabled property value. Indicates whether the profile supports the extraction of hardware hash values and registration of the device into Windows Autopilot. When TRUE, indicates if hardware extraction and Windows Autopilot registration will happen on the next successful check-in. When FALSE, hardware hash extraction and Windows Autopilot registration will not happen. Default value is FALSE. Supports: $select, $top, $skip. $Search, $orderBy and $filter are not supported.
* @param value Value to set for the hardwareHashExtractionEnabled property.
*/
public void setHardwareHashExtractionEnabled(@jakarta.annotation.Nullable final Boolean value) {
this.backingStore.set("hardwareHashExtractionEnabled", value);
}
/**
* Sets the lastModifiedDateTime property value. The date and time of when the deployment profile was last modified. The value cannot be updated manually and is automatically populated when any changes are made to the profile. The Timestamp type represents date and time information using ISO 8601 format and is always in UTC time. For example, midnight UTC on Jan 1, 2014 would look like this: '2014-01-01T00:00:00Z'. Supports: $select, $top, $skip. $Search, $orderBy and $filter are not supported Read-Only.
* @param value Value to set for the lastModifiedDateTime property.
*/
public void setLastModifiedDateTime(@jakarta.annotation.Nullable final OffsetDateTime value) {
this.backingStore.set("lastModifiedDateTime", value);
}
/**
* Sets the locale property value. The locale (language) to be used when configuring the device. E.g. en-US. The default value is os-default. Supports: $select, $top, $skip. $Search, $orderBy and $filter are not supported.
* @param value Value to set for the locale property.
*/
public void setLocale(@jakarta.annotation.Nullable final String value) {
this.backingStore.set("locale", value);
}
/**
* Sets the managementServiceAppId property value. The Entra management service App ID which gets used during client device-based enrollment discovery. Supports: $select, $top, $skip. $Search, $orderBy and $filter are not supported.
* @param value Value to set for the managementServiceAppId property.
*/
public void setManagementServiceAppId(@jakarta.annotation.Nullable final String value) {
this.backingStore.set("managementServiceAppId", value);
}
/**
* Sets the outOfBoxExperienceSetting property value. The Windows Autopilot Deployment Profile settings used by the device for the out-of-box experience. Supports: $select, $top, $skip. $Search, $orderBy and $filter are not supported.
* @param value Value to set for the outOfBoxExperienceSetting property.
*/
public void setOutOfBoxExperienceSetting(@jakarta.annotation.Nullable final OutOfBoxExperienceSetting value) {
this.backingStore.set("outOfBoxExperienceSetting", value);
}
/**
* Sets the preprovisioningAllowed property value. Indicates whether the user is allowed to use Windows Autopilot for pre-provisioned deployment mode during Out of Box experience (OOBE). When TRUE, indicates that Windows Autopilot for pre-provisioned deployment mode for OOBE is allowed to be used. When false, Windows Autopilot for pre-provisioned deployment mode for OOBE is not allowed. The default is FALSE.
* @param value Value to set for the preprovisioningAllowed property.
*/
public void setPreprovisioningAllowed(@jakarta.annotation.Nullable final Boolean value) {
this.backingStore.set("preprovisioningAllowed", value);
}
/**
* Sets the roleScopeTagIds property value. List of role scope tags for the deployment profile.
* @param value Value to set for the roleScopeTagIds property.
*/
public void setRoleScopeTagIds(@jakarta.annotation.Nullable final java.util.List value) {
this.backingStore.set("roleScopeTagIds", value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy