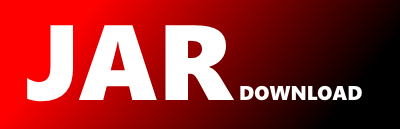
com.microsoft.graph.generated.teams.item.schedule.ScheduleRequestBuilder Maven / Gradle / Ivy
package com.microsoft.graph.teams.item.schedule;
import com.microsoft.graph.models.odataerrors.ODataError;
import com.microsoft.graph.models.Schedule;
import com.microsoft.graph.teams.item.schedule.offershiftrequests.OfferShiftRequestsRequestBuilder;
import com.microsoft.graph.teams.item.schedule.openshiftchangerequests.OpenShiftChangeRequestsRequestBuilder;
import com.microsoft.graph.teams.item.schedule.openshifts.OpenShiftsRequestBuilder;
import com.microsoft.graph.teams.item.schedule.schedulinggroups.SchedulingGroupsRequestBuilder;
import com.microsoft.graph.teams.item.schedule.share.ShareRequestBuilder;
import com.microsoft.graph.teams.item.schedule.shifts.ShiftsRequestBuilder;
import com.microsoft.graph.teams.item.schedule.swapshiftschangerequests.SwapShiftsChangeRequestsRequestBuilder;
import com.microsoft.graph.teams.item.schedule.timeoffreasons.TimeOffReasonsRequestBuilder;
import com.microsoft.graph.teams.item.schedule.timeoffrequests.TimeOffRequestsRequestBuilder;
import com.microsoft.graph.teams.item.schedule.timesoff.TimesOffRequestBuilder;
import com.microsoft.kiota.BaseRequestBuilder;
import com.microsoft.kiota.BaseRequestConfiguration;
import com.microsoft.kiota.HttpMethod;
import com.microsoft.kiota.QueryParameters;
import com.microsoft.kiota.RequestAdapter;
import com.microsoft.kiota.RequestInformation;
import com.microsoft.kiota.RequestOption;
import com.microsoft.kiota.serialization.Parsable;
import com.microsoft.kiota.serialization.ParsableFactory;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
/**
* Provides operations to manage the schedule property of the microsoft.graph.team entity.
*/
@jakarta.annotation.Generated("com.microsoft.kiota")
public class ScheduleRequestBuilder extends BaseRequestBuilder {
/**
* Provides operations to manage the offerShiftRequests property of the microsoft.graph.schedule entity.
* @return a {@link OfferShiftRequestsRequestBuilder}
*/
@jakarta.annotation.Nonnull
public OfferShiftRequestsRequestBuilder offerShiftRequests() {
return new OfferShiftRequestsRequestBuilder(pathParameters, requestAdapter);
}
/**
* Provides operations to manage the openShiftChangeRequests property of the microsoft.graph.schedule entity.
* @return a {@link OpenShiftChangeRequestsRequestBuilder}
*/
@jakarta.annotation.Nonnull
public OpenShiftChangeRequestsRequestBuilder openShiftChangeRequests() {
return new OpenShiftChangeRequestsRequestBuilder(pathParameters, requestAdapter);
}
/**
* Provides operations to manage the openShifts property of the microsoft.graph.schedule entity.
* @return a {@link OpenShiftsRequestBuilder}
*/
@jakarta.annotation.Nonnull
public OpenShiftsRequestBuilder openShifts() {
return new OpenShiftsRequestBuilder(pathParameters, requestAdapter);
}
/**
* Provides operations to manage the schedulingGroups property of the microsoft.graph.schedule entity.
* @return a {@link SchedulingGroupsRequestBuilder}
*/
@jakarta.annotation.Nonnull
public SchedulingGroupsRequestBuilder schedulingGroups() {
return new SchedulingGroupsRequestBuilder(pathParameters, requestAdapter);
}
/**
* Provides operations to call the share method.
* @return a {@link ShareRequestBuilder}
*/
@jakarta.annotation.Nonnull
public ShareRequestBuilder share() {
return new ShareRequestBuilder(pathParameters, requestAdapter);
}
/**
* Provides operations to manage the shifts property of the microsoft.graph.schedule entity.
* @return a {@link ShiftsRequestBuilder}
*/
@jakarta.annotation.Nonnull
public ShiftsRequestBuilder shifts() {
return new ShiftsRequestBuilder(pathParameters, requestAdapter);
}
/**
* Provides operations to manage the swapShiftsChangeRequests property of the microsoft.graph.schedule entity.
* @return a {@link SwapShiftsChangeRequestsRequestBuilder}
*/
@jakarta.annotation.Nonnull
public SwapShiftsChangeRequestsRequestBuilder swapShiftsChangeRequests() {
return new SwapShiftsChangeRequestsRequestBuilder(pathParameters, requestAdapter);
}
/**
* Provides operations to manage the timeOffReasons property of the microsoft.graph.schedule entity.
* @return a {@link TimeOffReasonsRequestBuilder}
*/
@jakarta.annotation.Nonnull
public TimeOffReasonsRequestBuilder timeOffReasons() {
return new TimeOffReasonsRequestBuilder(pathParameters, requestAdapter);
}
/**
* Provides operations to manage the timeOffRequests property of the microsoft.graph.schedule entity.
* @return a {@link TimeOffRequestsRequestBuilder}
*/
@jakarta.annotation.Nonnull
public TimeOffRequestsRequestBuilder timeOffRequests() {
return new TimeOffRequestsRequestBuilder(pathParameters, requestAdapter);
}
/**
* Provides operations to manage the timesOff property of the microsoft.graph.schedule entity.
* @return a {@link TimesOffRequestBuilder}
*/
@jakarta.annotation.Nonnull
public TimesOffRequestBuilder timesOff() {
return new TimesOffRequestBuilder(pathParameters, requestAdapter);
}
/**
* Instantiates a new {@link ScheduleRequestBuilder} and sets the default values.
* @param pathParameters Path parameters for the request
* @param requestAdapter The request adapter to use to execute the requests.
*/
public ScheduleRequestBuilder(@jakarta.annotation.Nonnull final HashMap pathParameters, @jakarta.annotation.Nonnull final RequestAdapter requestAdapter) {
super(requestAdapter, "{+baseurl}/teams/{team%2Did}/schedule{?%24expand,%24select}", pathParameters);
}
/**
* Instantiates a new {@link ScheduleRequestBuilder} and sets the default values.
* @param rawUrl The raw URL to use for the request builder.
* @param requestAdapter The request adapter to use to execute the requests.
*/
public ScheduleRequestBuilder(@jakarta.annotation.Nonnull final String rawUrl, @jakarta.annotation.Nonnull final RequestAdapter requestAdapter) {
super(requestAdapter, "{+baseurl}/teams/{team%2Did}/schedule{?%24expand,%24select}", rawUrl);
}
/**
* Delete navigation property schedule for teams
* @throws ODataError When receiving a 4XX or 5XX status code
*/
public void delete() {
delete(null);
}
/**
* Delete navigation property schedule for teams
* @param requestConfiguration Configuration for the request such as headers, query parameters, and middleware options.
* @throws ODataError When receiving a 4XX or 5XX status code
*/
public void delete(@jakarta.annotation.Nullable final java.util.function.Consumer requestConfiguration) {
final RequestInformation requestInfo = toDeleteRequestInformation(requestConfiguration);
final HashMap> errorMapping = new HashMap>();
errorMapping.put("XXX", ODataError::createFromDiscriminatorValue);
this.requestAdapter.sendPrimitive(requestInfo, errorMapping, Void.class);
}
/**
* Retrieve the properties and relationships of a schedule object. The schedule creation process conforms to the One API guideline for resource based long running operations (RELO).When clients use the PUT method, if the schedule is provisioned, the operation updates the schedule; otherwise, the operation starts the schedule provisioning process in the background. During schedule provisioning, clients can use the GET method to get the schedule and look at the provisionStatus property for the current state of the provisioning. If the provisioning failed, clients can get additional information from the provisionStatusCode property. Clients can also inspect the configuration of the schedule.
* @return a {@link Schedule}
* @throws ODataError When receiving a 4XX or 5XX status code
* @see Find more info here
*/
@jakarta.annotation.Nullable
public Schedule get() {
return get(null);
}
/**
* Retrieve the properties and relationships of a schedule object. The schedule creation process conforms to the One API guideline for resource based long running operations (RELO).When clients use the PUT method, if the schedule is provisioned, the operation updates the schedule; otherwise, the operation starts the schedule provisioning process in the background. During schedule provisioning, clients can use the GET method to get the schedule and look at the provisionStatus property for the current state of the provisioning. If the provisioning failed, clients can get additional information from the provisionStatusCode property. Clients can also inspect the configuration of the schedule.
* @param requestConfiguration Configuration for the request such as headers, query parameters, and middleware options.
* @return a {@link Schedule}
* @throws ODataError When receiving a 4XX or 5XX status code
* @see Find more info here
*/
@jakarta.annotation.Nullable
public Schedule get(@jakarta.annotation.Nullable final java.util.function.Consumer requestConfiguration) {
final RequestInformation requestInfo = toGetRequestInformation(requestConfiguration);
final HashMap> errorMapping = new HashMap>();
errorMapping.put("XXX", ODataError::createFromDiscriminatorValue);
return this.requestAdapter.send(requestInfo, errorMapping, Schedule::createFromDiscriminatorValue);
}
/**
* Create or replace a schedule object. The schedule creation process conforms to the One API guideline for resource based long running operations (RELO).When clients use the PUT method, if the schedule is provisioned, the operation replaces the schedule; otherwise, the operation starts the schedule provisioning process in the background. During schedule provisioning, clients can use the GET method to get the schedule and look at the provisionStatus property for the current state of the provisioning. If the provisioning failed, clients can get additional information from the provisionStatusCode property. Clients can also inspect the configuration of the schedule.
* @param body The request body
* @return a {@link Schedule}
* @throws ODataError When receiving a 4XX or 5XX status code
* @see Find more info here
*/
@jakarta.annotation.Nullable
public Schedule put(@jakarta.annotation.Nonnull final Schedule body) {
return put(body, null);
}
/**
* Create or replace a schedule object. The schedule creation process conforms to the One API guideline for resource based long running operations (RELO).When clients use the PUT method, if the schedule is provisioned, the operation replaces the schedule; otherwise, the operation starts the schedule provisioning process in the background. During schedule provisioning, clients can use the GET method to get the schedule and look at the provisionStatus property for the current state of the provisioning. If the provisioning failed, clients can get additional information from the provisionStatusCode property. Clients can also inspect the configuration of the schedule.
* @param body The request body
* @param requestConfiguration Configuration for the request such as headers, query parameters, and middleware options.
* @return a {@link Schedule}
* @throws ODataError When receiving a 4XX or 5XX status code
* @see Find more info here
*/
@jakarta.annotation.Nullable
public Schedule put(@jakarta.annotation.Nonnull final Schedule body, @jakarta.annotation.Nullable final java.util.function.Consumer requestConfiguration) {
Objects.requireNonNull(body);
final RequestInformation requestInfo = toPutRequestInformation(body, requestConfiguration);
final HashMap> errorMapping = new HashMap>();
errorMapping.put("XXX", ODataError::createFromDiscriminatorValue);
return this.requestAdapter.send(requestInfo, errorMapping, Schedule::createFromDiscriminatorValue);
}
/**
* Delete navigation property schedule for teams
* @return a {@link RequestInformation}
*/
@jakarta.annotation.Nonnull
public RequestInformation toDeleteRequestInformation() {
return toDeleteRequestInformation(null);
}
/**
* Delete navigation property schedule for teams
* @param requestConfiguration Configuration for the request such as headers, query parameters, and middleware options.
* @return a {@link RequestInformation}
*/
@jakarta.annotation.Nonnull
public RequestInformation toDeleteRequestInformation(@jakarta.annotation.Nullable final java.util.function.Consumer requestConfiguration) {
final RequestInformation requestInfo = new RequestInformation(HttpMethod.DELETE, urlTemplate, pathParameters);
requestInfo.configure(requestConfiguration, DeleteRequestConfiguration::new);
requestInfo.headers.tryAdd("Accept", "application/json");
return requestInfo;
}
/**
* Retrieve the properties and relationships of a schedule object. The schedule creation process conforms to the One API guideline for resource based long running operations (RELO).When clients use the PUT method, if the schedule is provisioned, the operation updates the schedule; otherwise, the operation starts the schedule provisioning process in the background. During schedule provisioning, clients can use the GET method to get the schedule and look at the provisionStatus property for the current state of the provisioning. If the provisioning failed, clients can get additional information from the provisionStatusCode property. Clients can also inspect the configuration of the schedule.
* @return a {@link RequestInformation}
*/
@jakarta.annotation.Nonnull
public RequestInformation toGetRequestInformation() {
return toGetRequestInformation(null);
}
/**
* Retrieve the properties and relationships of a schedule object. The schedule creation process conforms to the One API guideline for resource based long running operations (RELO).When clients use the PUT method, if the schedule is provisioned, the operation updates the schedule; otherwise, the operation starts the schedule provisioning process in the background. During schedule provisioning, clients can use the GET method to get the schedule and look at the provisionStatus property for the current state of the provisioning. If the provisioning failed, clients can get additional information from the provisionStatusCode property. Clients can also inspect the configuration of the schedule.
* @param requestConfiguration Configuration for the request such as headers, query parameters, and middleware options.
* @return a {@link RequestInformation}
*/
@jakarta.annotation.Nonnull
public RequestInformation toGetRequestInformation(@jakarta.annotation.Nullable final java.util.function.Consumer requestConfiguration) {
final RequestInformation requestInfo = new RequestInformation(HttpMethod.GET, urlTemplate, pathParameters);
requestInfo.configure(requestConfiguration, GetRequestConfiguration::new, x -> x.queryParameters);
requestInfo.headers.tryAdd("Accept", "application/json");
return requestInfo;
}
/**
* Create or replace a schedule object. The schedule creation process conforms to the One API guideline for resource based long running operations (RELO).When clients use the PUT method, if the schedule is provisioned, the operation replaces the schedule; otherwise, the operation starts the schedule provisioning process in the background. During schedule provisioning, clients can use the GET method to get the schedule and look at the provisionStatus property for the current state of the provisioning. If the provisioning failed, clients can get additional information from the provisionStatusCode property. Clients can also inspect the configuration of the schedule.
* @param body The request body
* @return a {@link RequestInformation}
*/
@jakarta.annotation.Nonnull
public RequestInformation toPutRequestInformation(@jakarta.annotation.Nonnull final Schedule body) {
return toPutRequestInformation(body, null);
}
/**
* Create or replace a schedule object. The schedule creation process conforms to the One API guideline for resource based long running operations (RELO).When clients use the PUT method, if the schedule is provisioned, the operation replaces the schedule; otherwise, the operation starts the schedule provisioning process in the background. During schedule provisioning, clients can use the GET method to get the schedule and look at the provisionStatus property for the current state of the provisioning. If the provisioning failed, clients can get additional information from the provisionStatusCode property. Clients can also inspect the configuration of the schedule.
* @param body The request body
* @param requestConfiguration Configuration for the request such as headers, query parameters, and middleware options.
* @return a {@link RequestInformation}
*/
@jakarta.annotation.Nonnull
public RequestInformation toPutRequestInformation(@jakarta.annotation.Nonnull final Schedule body, @jakarta.annotation.Nullable final java.util.function.Consumer requestConfiguration) {
Objects.requireNonNull(body);
final RequestInformation requestInfo = new RequestInformation(HttpMethod.PUT, urlTemplate, pathParameters);
requestInfo.configure(requestConfiguration, PutRequestConfiguration::new);
requestInfo.headers.tryAdd("Accept", "application/json");
requestInfo.setContentFromParsable(requestAdapter, "application/json", body);
return requestInfo;
}
/**
* Returns a request builder with the provided arbitrary URL. Using this method means any other path or query parameters are ignored.
* @param rawUrl The raw URL to use for the request builder.
* @return a {@link ScheduleRequestBuilder}
*/
@jakarta.annotation.Nonnull
public ScheduleRequestBuilder withUrl(@jakarta.annotation.Nonnull final String rawUrl) {
Objects.requireNonNull(rawUrl);
return new ScheduleRequestBuilder(rawUrl, requestAdapter);
}
/**
* Configuration for the request such as headers, query parameters, and middleware options.
*/
@jakarta.annotation.Generated("com.microsoft.kiota")
public class DeleteRequestConfiguration extends BaseRequestConfiguration {
}
/**
* Retrieve the properties and relationships of a schedule object. The schedule creation process conforms to the One API guideline for resource based long running operations (RELO).When clients use the PUT method, if the schedule is provisioned, the operation updates the schedule; otherwise, the operation starts the schedule provisioning process in the background. During schedule provisioning, clients can use the GET method to get the schedule and look at the provisionStatus property for the current state of the provisioning. If the provisioning failed, clients can get additional information from the provisionStatusCode property. Clients can also inspect the configuration of the schedule.
*/
@jakarta.annotation.Generated("com.microsoft.kiota")
public class GetQueryParameters implements QueryParameters {
/**
* Expand related entities
*/
@jakarta.annotation.Nullable
public String[] expand;
/**
* Select properties to be returned
*/
@jakarta.annotation.Nullable
public String[] select;
/**
* Extracts the query parameters into a map for the URI template parsing.
* @return a {@link Map}
*/
@jakarta.annotation.Nonnull
public Map toQueryParameters() {
final Map allQueryParams = new HashMap();
allQueryParams.put("%24expand", expand);
allQueryParams.put("%24select", select);
return allQueryParams;
}
}
/**
* Configuration for the request such as headers, query parameters, and middleware options.
*/
@jakarta.annotation.Generated("com.microsoft.kiota")
public class GetRequestConfiguration extends BaseRequestConfiguration {
/**
* Request query parameters
*/
@jakarta.annotation.Nullable
public GetQueryParameters queryParameters = new GetQueryParameters();
}
/**
* Configuration for the request such as headers, query parameters, and middleware options.
*/
@jakarta.annotation.Generated("com.microsoft.kiota")
public class PutRequestConfiguration extends BaseRequestConfiguration {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy