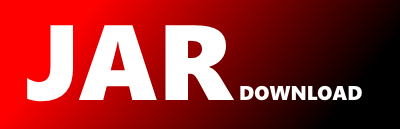
com.microsoft.graph.generated.agreements.item.file.FileRequestBuilder Maven / Gradle / Ivy
package com.microsoft.graph.agreements.item.file;
import com.microsoft.graph.agreements.item.file.localizations.LocalizationsRequestBuilder;
import com.microsoft.graph.models.AgreementFile;
import com.microsoft.graph.models.odataerrors.ODataError;
import com.microsoft.kiota.BaseRequestBuilder;
import com.microsoft.kiota.BaseRequestConfiguration;
import com.microsoft.kiota.HttpMethod;
import com.microsoft.kiota.QueryParameters;
import com.microsoft.kiota.RequestAdapter;
import com.microsoft.kiota.RequestInformation;
import com.microsoft.kiota.RequestOption;
import com.microsoft.kiota.serialization.Parsable;
import com.microsoft.kiota.serialization.ParsableFactory;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
/**
* Provides operations to manage the file property of the microsoft.graph.agreement entity.
*/
@jakarta.annotation.Generated("com.microsoft.kiota")
public class FileRequestBuilder extends BaseRequestBuilder {
/**
* Provides operations to manage the localizations property of the microsoft.graph.agreementFile entity.
* @return a {@link LocalizationsRequestBuilder}
*/
@jakarta.annotation.Nonnull
public LocalizationsRequestBuilder localizations() {
return new LocalizationsRequestBuilder(pathParameters, requestAdapter);
}
/**
* Instantiates a new {@link FileRequestBuilder} and sets the default values.
* @param pathParameters Path parameters for the request
* @param requestAdapter The request adapter to use to execute the requests.
*/
public FileRequestBuilder(@jakarta.annotation.Nonnull final HashMap pathParameters, @jakarta.annotation.Nonnull final RequestAdapter requestAdapter) {
super(requestAdapter, "{+baseurl}/agreements/{agreement%2Did}/file{?%24expand,%24select}", pathParameters);
}
/**
* Instantiates a new {@link FileRequestBuilder} and sets the default values.
* @param rawUrl The raw URL to use for the request builder.
* @param requestAdapter The request adapter to use to execute the requests.
*/
public FileRequestBuilder(@jakarta.annotation.Nonnull final String rawUrl, @jakarta.annotation.Nonnull final RequestAdapter requestAdapter) {
super(requestAdapter, "{+baseurl}/agreements/{agreement%2Did}/file{?%24expand,%24select}", rawUrl);
}
/**
* Delete navigation property file for agreements
* @throws ODataError When receiving a 4XX or 5XX status code
*/
public void delete() {
delete(null);
}
/**
* Delete navigation property file for agreements
* @param requestConfiguration Configuration for the request such as headers, query parameters, and middleware options.
* @throws ODataError When receiving a 4XX or 5XX status code
*/
public void delete(@jakarta.annotation.Nullable final java.util.function.Consumer requestConfiguration) {
final RequestInformation requestInfo = toDeleteRequestInformation(requestConfiguration);
final HashMap> errorMapping = new HashMap>();
errorMapping.put("XXX", ODataError::createFromDiscriminatorValue);
this.requestAdapter.sendPrimitive(requestInfo, errorMapping, Void.class);
}
/**
* Retrieve the details of an agreement file, including the language and version information. The default file can have multiple versions, each with its own language, that can be retrieved by specifying the Accept-Language header.
* @return a {@link AgreementFile}
* @throws ODataError When receiving a 4XX or 5XX status code
* @see Find more info here
*/
@jakarta.annotation.Nullable
public AgreementFile get() {
return get(null);
}
/**
* Retrieve the details of an agreement file, including the language and version information. The default file can have multiple versions, each with its own language, that can be retrieved by specifying the Accept-Language header.
* @param requestConfiguration Configuration for the request such as headers, query parameters, and middleware options.
* @return a {@link AgreementFile}
* @throws ODataError When receiving a 4XX or 5XX status code
* @see Find more info here
*/
@jakarta.annotation.Nullable
public AgreementFile get(@jakarta.annotation.Nullable final java.util.function.Consumer requestConfiguration) {
final RequestInformation requestInfo = toGetRequestInformation(requestConfiguration);
final HashMap> errorMapping = new HashMap>();
errorMapping.put("XXX", ODataError::createFromDiscriminatorValue);
return this.requestAdapter.send(requestInfo, errorMapping, AgreementFile::createFromDiscriminatorValue);
}
/**
* Update the navigation property file in agreements
* @param body The request body
* @return a {@link AgreementFile}
* @throws ODataError When receiving a 4XX or 5XX status code
*/
@jakarta.annotation.Nullable
public AgreementFile patch(@jakarta.annotation.Nonnull final AgreementFile body) {
return patch(body, null);
}
/**
* Update the navigation property file in agreements
* @param body The request body
* @param requestConfiguration Configuration for the request such as headers, query parameters, and middleware options.
* @return a {@link AgreementFile}
* @throws ODataError When receiving a 4XX or 5XX status code
*/
@jakarta.annotation.Nullable
public AgreementFile patch(@jakarta.annotation.Nonnull final AgreementFile body, @jakarta.annotation.Nullable final java.util.function.Consumer requestConfiguration) {
Objects.requireNonNull(body);
final RequestInformation requestInfo = toPatchRequestInformation(body, requestConfiguration);
final HashMap> errorMapping = new HashMap>();
errorMapping.put("XXX", ODataError::createFromDiscriminatorValue);
return this.requestAdapter.send(requestInfo, errorMapping, AgreementFile::createFromDiscriminatorValue);
}
/**
* Delete navigation property file for agreements
* @return a {@link RequestInformation}
*/
@jakarta.annotation.Nonnull
public RequestInformation toDeleteRequestInformation() {
return toDeleteRequestInformation(null);
}
/**
* Delete navigation property file for agreements
* @param requestConfiguration Configuration for the request such as headers, query parameters, and middleware options.
* @return a {@link RequestInformation}
*/
@jakarta.annotation.Nonnull
public RequestInformation toDeleteRequestInformation(@jakarta.annotation.Nullable final java.util.function.Consumer requestConfiguration) {
final RequestInformation requestInfo = new RequestInformation(HttpMethod.DELETE, urlTemplate, pathParameters);
requestInfo.configure(requestConfiguration, DeleteRequestConfiguration::new);
requestInfo.headers.tryAdd("Accept", "application/json");
return requestInfo;
}
/**
* Retrieve the details of an agreement file, including the language and version information. The default file can have multiple versions, each with its own language, that can be retrieved by specifying the Accept-Language header.
* @return a {@link RequestInformation}
*/
@jakarta.annotation.Nonnull
public RequestInformation toGetRequestInformation() {
return toGetRequestInformation(null);
}
/**
* Retrieve the details of an agreement file, including the language and version information. The default file can have multiple versions, each with its own language, that can be retrieved by specifying the Accept-Language header.
* @param requestConfiguration Configuration for the request such as headers, query parameters, and middleware options.
* @return a {@link RequestInformation}
*/
@jakarta.annotation.Nonnull
public RequestInformation toGetRequestInformation(@jakarta.annotation.Nullable final java.util.function.Consumer requestConfiguration) {
final RequestInformation requestInfo = new RequestInformation(HttpMethod.GET, urlTemplate, pathParameters);
requestInfo.configure(requestConfiguration, GetRequestConfiguration::new, x -> x.queryParameters);
requestInfo.headers.tryAdd("Accept", "application/json");
return requestInfo;
}
/**
* Update the navigation property file in agreements
* @param body The request body
* @return a {@link RequestInformation}
*/
@jakarta.annotation.Nonnull
public RequestInformation toPatchRequestInformation(@jakarta.annotation.Nonnull final AgreementFile body) {
return toPatchRequestInformation(body, null);
}
/**
* Update the navigation property file in agreements
* @param body The request body
* @param requestConfiguration Configuration for the request such as headers, query parameters, and middleware options.
* @return a {@link RequestInformation}
*/
@jakarta.annotation.Nonnull
public RequestInformation toPatchRequestInformation(@jakarta.annotation.Nonnull final AgreementFile body, @jakarta.annotation.Nullable final java.util.function.Consumer requestConfiguration) {
Objects.requireNonNull(body);
final RequestInformation requestInfo = new RequestInformation(HttpMethod.PATCH, urlTemplate, pathParameters);
requestInfo.configure(requestConfiguration, PatchRequestConfiguration::new);
requestInfo.headers.tryAdd("Accept", "application/json");
requestInfo.setContentFromParsable(requestAdapter, "application/json", body);
return requestInfo;
}
/**
* Returns a request builder with the provided arbitrary URL. Using this method means any other path or query parameters are ignored.
* @param rawUrl The raw URL to use for the request builder.
* @return a {@link FileRequestBuilder}
*/
@jakarta.annotation.Nonnull
public FileRequestBuilder withUrl(@jakarta.annotation.Nonnull final String rawUrl) {
Objects.requireNonNull(rawUrl);
return new FileRequestBuilder(rawUrl, requestAdapter);
}
/**
* Configuration for the request such as headers, query parameters, and middleware options.
*/
@jakarta.annotation.Generated("com.microsoft.kiota")
public class DeleteRequestConfiguration extends BaseRequestConfiguration {
}
/**
* Retrieve the details of an agreement file, including the language and version information. The default file can have multiple versions, each with its own language, that can be retrieved by specifying the Accept-Language header.
*/
@jakarta.annotation.Generated("com.microsoft.kiota")
public class GetQueryParameters implements QueryParameters {
/**
* Expand related entities
*/
@jakarta.annotation.Nullable
public String[] expand;
/**
* Select properties to be returned
*/
@jakarta.annotation.Nullable
public String[] select;
/**
* Extracts the query parameters into a map for the URI template parsing.
* @return a {@link Map}
*/
@jakarta.annotation.Nonnull
public Map toQueryParameters() {
final Map allQueryParams = new HashMap();
allQueryParams.put("%24expand", expand);
allQueryParams.put("%24select", select);
return allQueryParams;
}
}
/**
* Configuration for the request such as headers, query parameters, and middleware options.
*/
@jakarta.annotation.Generated("com.microsoft.kiota")
public class GetRequestConfiguration extends BaseRequestConfiguration {
/**
* Request query parameters
*/
@jakarta.annotation.Nullable
public GetQueryParameters queryParameters = new GetQueryParameters();
}
/**
* Configuration for the request such as headers, query parameters, and middleware options.
*/
@jakarta.annotation.Generated("com.microsoft.kiota")
public class PatchRequestConfiguration extends BaseRequestConfiguration {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy