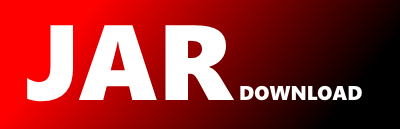
com.microsoft.graph.generated.models.PrivilegedAccessGroup Maven / Gradle / Ivy
package com.microsoft.graph.models;
import com.microsoft.kiota.serialization.Parsable;
import com.microsoft.kiota.serialization.ParseNode;
import com.microsoft.kiota.serialization.SerializationWriter;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
@jakarta.annotation.Generated("com.microsoft.kiota")
public class PrivilegedAccessGroup extends Entity implements Parsable {
/**
* Instantiates a new {@link PrivilegedAccessGroup} and sets the default values.
*/
public PrivilegedAccessGroup() {
super();
}
/**
* Creates a new instance of the appropriate class based on discriminator value
* @param parseNode The parse node to use to read the discriminator value and create the object
* @return a {@link PrivilegedAccessGroup}
*/
@jakarta.annotation.Nonnull
public static PrivilegedAccessGroup createFromDiscriminatorValue(@jakarta.annotation.Nonnull final ParseNode parseNode) {
Objects.requireNonNull(parseNode);
return new PrivilegedAccessGroup();
}
/**
* Gets the assignmentApprovals property value. The assignmentApprovals property
* @return a {@link java.util.List}
*/
@jakarta.annotation.Nullable
public java.util.List getAssignmentApprovals() {
return this.backingStore.get("assignmentApprovals");
}
/**
* Gets the assignmentScheduleInstances property value. The instances of assignment schedules to activate a just-in-time access.
* @return a {@link java.util.List}
*/
@jakarta.annotation.Nullable
public java.util.List getAssignmentScheduleInstances() {
return this.backingStore.get("assignmentScheduleInstances");
}
/**
* Gets the assignmentScheduleRequests property value. The schedule requests for operations to create, update, delete, extend, and renew an assignment.
* @return a {@link java.util.List}
*/
@jakarta.annotation.Nullable
public java.util.List getAssignmentScheduleRequests() {
return this.backingStore.get("assignmentScheduleRequests");
}
/**
* Gets the assignmentSchedules property value. The assignment schedules to activate a just-in-time access.
* @return a {@link java.util.List}
*/
@jakarta.annotation.Nullable
public java.util.List getAssignmentSchedules() {
return this.backingStore.get("assignmentSchedules");
}
/**
* Gets the eligibilityScheduleInstances property value. The instances of eligibility schedules to activate a just-in-time access.
* @return a {@link java.util.List}
*/
@jakarta.annotation.Nullable
public java.util.List getEligibilityScheduleInstances() {
return this.backingStore.get("eligibilityScheduleInstances");
}
/**
* Gets the eligibilityScheduleRequests property value. The schedule requests for operations to create, update, delete, extend, and renew an eligibility.
* @return a {@link java.util.List}
*/
@jakarta.annotation.Nullable
public java.util.List getEligibilityScheduleRequests() {
return this.backingStore.get("eligibilityScheduleRequests");
}
/**
* Gets the eligibilitySchedules property value. The eligibility schedules to activate a just-in-time access.
* @return a {@link java.util.List}
*/
@jakarta.annotation.Nullable
public java.util.List getEligibilitySchedules() {
return this.backingStore.get("eligibilitySchedules");
}
/**
* The deserialization information for the current model
* @return a {@link Map>}
*/
@jakarta.annotation.Nonnull
public Map> getFieldDeserializers() {
final HashMap> deserializerMap = new HashMap>(super.getFieldDeserializers());
deserializerMap.put("assignmentApprovals", (n) -> { this.setAssignmentApprovals(n.getCollectionOfObjectValues(Approval::createFromDiscriminatorValue)); });
deserializerMap.put("assignmentScheduleInstances", (n) -> { this.setAssignmentScheduleInstances(n.getCollectionOfObjectValues(PrivilegedAccessGroupAssignmentScheduleInstance::createFromDiscriminatorValue)); });
deserializerMap.put("assignmentScheduleRequests", (n) -> { this.setAssignmentScheduleRequests(n.getCollectionOfObjectValues(PrivilegedAccessGroupAssignmentScheduleRequest::createFromDiscriminatorValue)); });
deserializerMap.put("assignmentSchedules", (n) -> { this.setAssignmentSchedules(n.getCollectionOfObjectValues(PrivilegedAccessGroupAssignmentSchedule::createFromDiscriminatorValue)); });
deserializerMap.put("eligibilityScheduleInstances", (n) -> { this.setEligibilityScheduleInstances(n.getCollectionOfObjectValues(PrivilegedAccessGroupEligibilityScheduleInstance::createFromDiscriminatorValue)); });
deserializerMap.put("eligibilityScheduleRequests", (n) -> { this.setEligibilityScheduleRequests(n.getCollectionOfObjectValues(PrivilegedAccessGroupEligibilityScheduleRequest::createFromDiscriminatorValue)); });
deserializerMap.put("eligibilitySchedules", (n) -> { this.setEligibilitySchedules(n.getCollectionOfObjectValues(PrivilegedAccessGroupEligibilitySchedule::createFromDiscriminatorValue)); });
return deserializerMap;
}
/**
* Serializes information the current object
* @param writer Serialization writer to use to serialize this model
*/
public void serialize(@jakarta.annotation.Nonnull final SerializationWriter writer) {
Objects.requireNonNull(writer);
super.serialize(writer);
writer.writeCollectionOfObjectValues("assignmentApprovals", this.getAssignmentApprovals());
writer.writeCollectionOfObjectValues("assignmentScheduleInstances", this.getAssignmentScheduleInstances());
writer.writeCollectionOfObjectValues("assignmentScheduleRequests", this.getAssignmentScheduleRequests());
writer.writeCollectionOfObjectValues("assignmentSchedules", this.getAssignmentSchedules());
writer.writeCollectionOfObjectValues("eligibilityScheduleInstances", this.getEligibilityScheduleInstances());
writer.writeCollectionOfObjectValues("eligibilityScheduleRequests", this.getEligibilityScheduleRequests());
writer.writeCollectionOfObjectValues("eligibilitySchedules", this.getEligibilitySchedules());
}
/**
* Sets the assignmentApprovals property value. The assignmentApprovals property
* @param value Value to set for the assignmentApprovals property.
*/
public void setAssignmentApprovals(@jakarta.annotation.Nullable final java.util.List value) {
this.backingStore.set("assignmentApprovals", value);
}
/**
* Sets the assignmentScheduleInstances property value. The instances of assignment schedules to activate a just-in-time access.
* @param value Value to set for the assignmentScheduleInstances property.
*/
public void setAssignmentScheduleInstances(@jakarta.annotation.Nullable final java.util.List value) {
this.backingStore.set("assignmentScheduleInstances", value);
}
/**
* Sets the assignmentScheduleRequests property value. The schedule requests for operations to create, update, delete, extend, and renew an assignment.
* @param value Value to set for the assignmentScheduleRequests property.
*/
public void setAssignmentScheduleRequests(@jakarta.annotation.Nullable final java.util.List value) {
this.backingStore.set("assignmentScheduleRequests", value);
}
/**
* Sets the assignmentSchedules property value. The assignment schedules to activate a just-in-time access.
* @param value Value to set for the assignmentSchedules property.
*/
public void setAssignmentSchedules(@jakarta.annotation.Nullable final java.util.List value) {
this.backingStore.set("assignmentSchedules", value);
}
/**
* Sets the eligibilityScheduleInstances property value. The instances of eligibility schedules to activate a just-in-time access.
* @param value Value to set for the eligibilityScheduleInstances property.
*/
public void setEligibilityScheduleInstances(@jakarta.annotation.Nullable final java.util.List value) {
this.backingStore.set("eligibilityScheduleInstances", value);
}
/**
* Sets the eligibilityScheduleRequests property value. The schedule requests for operations to create, update, delete, extend, and renew an eligibility.
* @param value Value to set for the eligibilityScheduleRequests property.
*/
public void setEligibilityScheduleRequests(@jakarta.annotation.Nullable final java.util.List value) {
this.backingStore.set("eligibilityScheduleRequests", value);
}
/**
* Sets the eligibilitySchedules property value. The eligibility schedules to activate a just-in-time access.
* @param value Value to set for the eligibilitySchedules property.
*/
public void setEligibilitySchedules(@jakarta.annotation.Nullable final java.util.List value) {
this.backingStore.set("eligibilitySchedules", value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy