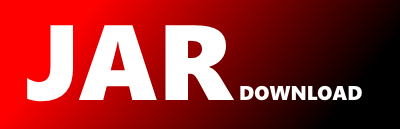
com.microsoft.rest.v2.DateTimeRfc1123 Maven / Gradle / Ivy
/**
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for
* license information.
*/
package com.microsoft.rest.v2;
import java.time.OffsetDateTime;
import java.time.ZoneId;
import java.time.format.DateTimeFormatter;
import java.util.Locale;
/**
* Simple wrapper over java.time.OffsetDateTime used for specifying RFC1123 format during serialization/deserialization.
*/
public final class DateTimeRfc1123 {
/**
* The pattern of the datetime used for RFC1123 datetime format.
*/
private static final DateTimeFormatter RFC1123_DATE_TIME_FORMATTER =
DateTimeFormatter.ofPattern("EEE, dd MMM yyyy HH:mm:ss 'GMT'").withZone(ZoneId.of("UTC")).withLocale(Locale.US);
/**
* The actual datetime object.
*/
private final OffsetDateTime dateTime;
/**
* Creates a new DateTimeRfc1123 object with the specified DateTime.
* @param dateTime The DateTime object to wrap.
*/
public DateTimeRfc1123(OffsetDateTime dateTime) {
this.dateTime = dateTime;
}
/**
* Creates a new DateTimeRfc1123 object with the specified DateTime.
* @param formattedString The datetime string in RFC1123 format
*/
public DateTimeRfc1123(String formattedString) {
this.dateTime = OffsetDateTime.parse(formattedString, DateTimeFormatter.RFC_1123_DATE_TIME);
}
/**
* Returns the underlying DateTime.
* @return The underlying DateTime.
*/
public OffsetDateTime dateTime() {
if (this.dateTime == null) {
return null;
}
return this.dateTime;
}
@Override
public String toString() {
return RFC1123_DATE_TIME_FORMATTER.format(this.dateTime);
}
@Override
public int hashCode() {
return this.dateTime.hashCode();
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (!(obj instanceof DateTimeRfc1123)) {
return false;
}
DateTimeRfc1123 rhs = (DateTimeRfc1123) obj;
return this.dateTime.equals(rhs.dateTime());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy