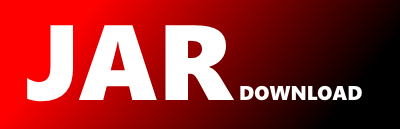
com.microsoft.store.partnercenter.subscriptions.SubscriptionCollectionOperations Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of partnercenter Show documentation
Show all versions of partnercenter Show documentation
SDK for accessing Microsoft Partner Center API.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT license. See the LICENSE file in the project root for full license information.
package com.microsoft.store.partnercenter.subscriptions;
import java.text.MessageFormat;
import com.fasterxml.jackson.core.type.TypeReference;
import com.microsoft.store.partnercenter.BasePartnerComponentString;
import com.microsoft.store.partnercenter.IPartner;
import com.microsoft.store.partnercenter.PartnerService;
import com.microsoft.store.partnercenter.genericoperations.IEntireEntityCollectionRetrievalOperations;
import com.microsoft.store.partnercenter.models.ResourceCollection;
import com.microsoft.store.partnercenter.models.subscriptions.Subscription;
import com.microsoft.store.partnercenter.usage.ISubscriptionMonthlyUsageRecordCollection;
import com.microsoft.store.partnercenter.usage.SubscriptionMonthlyUsageRecordCollectionOperations;
import com.microsoft.store.partnercenter.utils.ParameterValidator;
import com.microsoft.store.partnercenter.utils.StringHelper;
/**
* The customer subscriptions implementation.
*/
public class SubscriptionCollectionOperations
extends BasePartnerComponentString
implements ISubscriptionCollection
{
/**
* A lazy reference to the current customer's subscription usage records operations.
*/
private ISubscriptionMonthlyUsageRecordCollection usageRecords;
/**
* Initializes a new instance of the SubscriptionCollectionOperations class.
*
* @param rootPartnerOperations The root partner operations instance.
* @param customerId The customer Id to whom the subscriptions belong.
*/
public SubscriptionCollectionOperations(IPartner rootPartnerOperations, String customerId)
{
super(rootPartnerOperations, customerId);
if (StringHelper.isNullOrWhiteSpace(customerId))
{
throw new IllegalArgumentException("customerId must be set");
}
usageRecords = new SubscriptionMonthlyUsageRecordCollectionOperations(this.getPartner(), this.getContext());
}
/**
* Obtains the subscription usage records behavior for the customer.
*
* @return The customer subscription usage records.
*/
@Override
public ISubscriptionMonthlyUsageRecordCollection getUsageRecords()
{
return this.usageRecords;
}
/**
* Groups customer subscriptions by an order.
*
* @param orderId The order id.
* @return The order subscriptions operations.
*/
@Override
public IEntireEntityCollectionRetrievalOperations> byOrder(String orderId)
{
ParameterValidator.isValidUriQueryValue(orderId, "orderId is an invalid query value");
return new OrderSubscriptionCollectionOperations(this.getPartner(), this.getContext(), orderId);
}
/**
* Groups customer subscriptions by a partner.
*
* @param partnerId The partner id.
* @return The partner subscriptions operations.
*/
@Override
public IEntireEntityCollectionRetrievalOperations> byPartner(String partnerId)
{
ParameterValidator.isValidUriQueryValue(partnerId, "partnerId is an invalid query value");
return new PartnerSubscriptionCollectionOperations(this.getPartner(), this.getContext(), partnerId);
}
/**
* Retrieves a specific customer subscription behavior.
*
* @param subscriptionId The subscription identifier
* @return The customer subscription.
*/
@Override
public ISubscription byId(String subscriptionId)
{
return new SubscriptionOperations(this.getPartner(), this.getContext(), subscriptionId);
}
//
/**
* Retrieves all subscriptions.
*
* @return The subscriptions.
*/
@Override
public ResourceCollection get()
{
return this.getPartner().getServiceClient().get(
this.getPartner(),
new TypeReference>(){},
MessageFormat.format(
PartnerService.getInstance().getConfiguration().getApis().get("GetCustomerSubscriptions").getPath(),
this.getContext()));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy