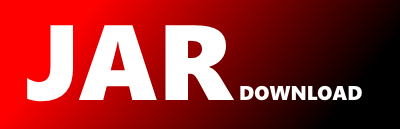
com.microsoft.store.partnercenter.customerusers.CustomerUserOperations Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of partnercenter Show documentation
Show all versions of partnercenter Show documentation
SDK for accessing Microsoft Partner Center API.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT license. See the LICENSE file in the project root for full license information.
package com.microsoft.store.partnercenter.customerusers;
import java.text.MessageFormat;
import com.fasterxml.jackson.core.type.TypeReference;
import com.microsoft.store.partnercenter.BasePartnerComponent;
import com.microsoft.store.partnercenter.IPartner;
import com.microsoft.store.partnercenter.PartnerService;
import com.microsoft.store.partnercenter.models.users.CustomerUser;
import com.microsoft.store.partnercenter.models.utils.Tuple;
import com.microsoft.store.partnercenter.utils.StringHelper;
public class CustomerUserOperations
extends BasePartnerComponent>
implements ICustomerUser {
/**
* The customer user directory role collection operations.
*/
private ICustomerUserRoleCollection customerUserDirectoryRoleCollectionOperations;
/**
* The customer user license collection operations.
*/
private ICustomerUserLicenseCollection customerUserLicenseCollectionOperations;
/**
* The customer user license update operations.
*/
private ICustomerUserLicenseUpdates customerUserLicenseUpdateOperations;
/**
* Initializes a new instance of the CustomerUserOperations class.
*
* @param rootPartnerOperations The root partner operations instance.
* @param customerId The customer identifier.
* @param userId The user identifier.
*/
public CustomerUserOperations(IPartner rootPartnerOperations, String customerId, String userId)
{
super(rootPartnerOperations, new Tuple(customerId, userId));
if (StringHelper.isNullOrWhiteSpace(customerId))
{
throw new IllegalArgumentException("customerId must be set");
}
if (StringHelper.isNullOrWhiteSpace(userId))
{
throw new IllegalArgumentException("userId must be set");
}
}
/**
* Retrieves information of a specific customer user.
*
* @return The customer user object.
*/
@Override
public CustomerUser get()
{
return this.getPartner().getServiceClient().get(
this.getPartner(),
new TypeReference(){},
MessageFormat.format(
PartnerService.getInstance().getConfiguration().getApis().get("GetCustomerUserDetails").getPath(),
this.getContext().getItem1(),
this.getContext().getItem2()));
}
/**
* Deletes the customer user.
*/
@Override
public void delete()
{
this.getPartner().getServiceClient().delete(
this.getPartner(),
new TypeReference(){},
MessageFormat.format(
PartnerService.getInstance().getConfiguration().getApis().get("DeleteCustomerUser").getPath(),
this.getContext().getItem1(),
this.getContext().getItem2()));
}
/**
* Updates the customer user.
*
* @param customerUser The customer to be updated.
* @return The updated user.
*/
@Override
public CustomerUser patch(CustomerUser customerUser)
{
if (customerUser == null)
{
throw new IllegalArgumentException("customerUser cannot be null");
}
return this.getPartner().getServiceClient().patch(
this.getPartner(),
new TypeReference(){},
MessageFormat.format(
PartnerService.getInstance().getConfiguration().getApis().get("UpdateCustomerUser").getPath(),
this.getContext().getItem1(),
this.getContext().getItem2()),
customerUser);
}
/**
* Retrieves the customer user's directory roles.
*
* @return The customer user's directory roles.
*/
@Override
public ICustomerUserRoleCollection getDirectoryRoles()
{
if (customerUserDirectoryRoleCollectionOperations == null)
{
customerUserDirectoryRoleCollectionOperations = new CustomerUserRoleCollectionOperations(this.getPartner(), this.getContext().getItem1(), this.getContext().getItem2());
}
return customerUserDirectoryRoleCollectionOperations;
}
/**
* Gets the current user's license collection operation.
*
* @return The customer user's licenses collection operations.
*/
@Override
public ICustomerUserLicenseCollection getLicenses()
{
if (customerUserLicenseCollectionOperations == null)
{
customerUserLicenseCollectionOperations = new CustomerUserLicenseCollectionOperations(this.getPartner(), this.getContext().getItem1(), this.getContext().getItem2());
}
return customerUserLicenseCollectionOperations;
}
/**
* Gets the current user's license updates operation.
*
* @return The customer user's license updates collection operations.
*/
@Override
public ICustomerUserLicenseUpdates getLicenseUpdates()
{
if (customerUserLicenseUpdateOperations == null)
{
customerUserLicenseUpdateOperations = new CustomerUserLicenseUpdateOperations(this.getPartner(), this.getContext().getItem1(), this.getContext().getItem2());
}
return customerUserLicenseUpdateOperations;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy