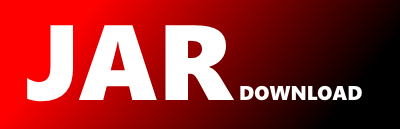
com.microsoft.store.partnercenter.models.entitlements.Entitlement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of partnercenter Show documentation
Show all versions of partnercenter Show documentation
SDK for accessing Microsoft Partner Center API.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT license. See the LICENSE file in the project root for full license information.
package com.microsoft.store.partnercenter.models.entitlements;
import com.microsoft.store.partnercenter.models.ResourceBaseWithLinks;
import com.microsoft.store.partnercenter.models.StandardResourceLinks;
import org.joda.time.DateTime;
/**
* Class that represents an entitlement.
*/
public class Entitlement
extends ResourceBaseWithLinks
{
private Iterable entitledArtifacts;
private String entitlementType;
/**
* The entitlement expiry date for the entitlement.
*/
private DateTime expiryDate;
/**
* The fulfillment state for the entitlement.
*/
private String fulfillmentState;
private Iterable includedEntitlements;
private String productId;
private Integer quantity;
private Iterable quantityDetails;
private ReferenceOrder referenceOrder;
private String skuId;
/**
* Gets the collection of entitled artifacts.
*
* @return The collection of entitled artifacts.
*/
public Iterable getEntitledArtifacts()
{
return entitledArtifacts;
}
/**
* Sets the collection of entitled artifacts.
*
* @param value The collection of entitled artifacts.
*/
public void setEntitledArtifacts(Iterable value)
{
entitledArtifacts = value;
}
/**
* Gets the entitlement type.
*
* @return The entitlement type.
*/
public String getEntitlementType()
{
return entitlementType;
}
/**
* Sets the entitlement type.
*
* @param value The entitlement type.
*/
public void setEntitlementType(String value)
{
entitlementType = value;
}
/**
* Gets the expiry date for the entitlement.
*
* @return The expiry date for the entitlement.
*/
public DateTime getExpiryDate()
{
return expiryDate;
}
/**
* Sets the expiry date for the entitlement.
*
* @param value The expiry date for the entitlement.
*/
public void setExpiryDate(DateTime value)
{
expiryDate = value;
}
/**
* Gets the fulfillment state for the entitlement.
*
* @return The fulfillment state for the entitlement.
*/
public String getFulfillmentState()
{
return fulfillmentState;
}
/**
* Sets the fulfillment state for the entitlement.
*
* @param value The the fulfillment state for the entitlement.
*/
public void setFulfillmentState(String value)
{
fulfillmentState = value;
}
/**
* Gets the included entitlements.
*
* @return The included entitlements.
*/
public Iterable getIncludedEntitlements()
{
return includedEntitlements;
}
/**
* Sets the included entitlements.
*
* @param value The included entitlements.
*/
public void setIncludedEntitlements(Iterable value)
{
includedEntitlements = value;
}
/**
* Gets the product identifier.
*
* @return The product identifier.
*/
public String getProductId()
{
return productId;
}
/**
* Sets the product identifier.
*
* @param value The product identifier.
*/
public void setProductId(String value)
{
productId = value;
}
/**
* Gets the quantity.
*
* @return The quantity.
*/
public Integer getQuantity()
{
return quantity;
}
/**
* Sets the quantity.
*
* @param value The quantity.
*/
public void setQuantity(Integer value)
{
quantity = value;
}
/**
* Gets the quantity details (quantity - state).
*
* @return The quantity details (quantity - state).
*/
public Iterable getQuantityDetails()
{
return quantityDetails;
}
/**
* Sets the quantity details (quantity - state).
*
* @param value The quantity details (quantity - state).
*/
public void setQuantityDetails(Iterable value)
{
quantityDetails = value;
}
/**
* Gets the reference order related to the entitlement.
*
* @return The reference order related to the entitlement.
*/
public ReferenceOrder getReferenceOrder()
{
return referenceOrder;
}
/**
* Sets the reference order related to the entitlement.
*
* @param value The reference order related to the entitlement.
*/
public void setReferenceOrder(ReferenceOrder value)
{
referenceOrder = value;
}
/**
* Gets the SKU identifier.
*
* @return The SKU identifier.
*/
public String getSkuId()
{
return skuId;
}
/**
* Sets the SKU identifier.
*
* @param value The SKU identifier.
*/
public void setSkuId(String value)
{
skuId = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy