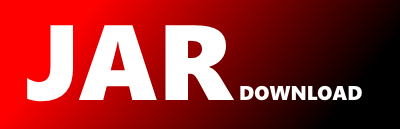
com.microsoft.store.partnercenter.profiles.MpnProfileOperations Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of partnercenter Show documentation
Show all versions of partnercenter Show documentation
SDK for accessing Microsoft Partner Center API.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT license. See the LICENSE file in the project root for full license information.
package com.microsoft.store.partnercenter.profiles;
import java.util.ArrayList;
import java.util.Collection;
import com.fasterxml.jackson.core.type.TypeReference;
import com.microsoft.store.partnercenter.BasePartnerComponentString;
import com.microsoft.store.partnercenter.IPartner;
import com.microsoft.store.partnercenter.PartnerService;
import com.microsoft.store.partnercenter.models.partners.MpnProfile;
import com.microsoft.store.partnercenter.models.utils.KeyValuePair;
/**
* Class which contains operations for Partner Network Profile
*/
public class MpnProfileOperations
extends BasePartnerComponentString
implements IMpnProfile
{
/**
* Initializes a new instance of the MpnProfileOperations class.
*
* @param rootPartnerOperations The root partner operations instance.
*/
public MpnProfileOperations(IPartner rootPartnerOperations)
{
super(rootPartnerOperations);
}
/**
* Retrieves the logged in reseller's MpnProfile.
*
* @return The partner network profile.
*/
@Override
public MpnProfile get()
{
return this.getPartner().getServiceClient().get(
this.getPartner(),
new TypeReference(){},
PartnerService.getInstance().getConfiguration().getApis().get("GetMpnProfile").getPath());
}
/**
* Retrieves a MpnProfile by MPN Id.
*
* @param mpnId The MPN Id.
* @return The partner network profile.
*/
@Override
public MpnProfile get(String mpnId)
{
Collection> parameters = new ArrayList>();
parameters.add
(
new KeyValuePair
(
PartnerService.getInstance().getConfiguration().getApis().get("GetMpnProfile").getParameters().get("MpnId"),
mpnId
)
);
return this.getPartner().getServiceClient().get(
this.getPartner(),
new TypeReference(){},
PartnerService.getInstance().getConfiguration().getApis().get("GetMpnProfile").getPath(),
parameters);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy