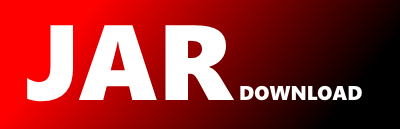
com.microsoft.store.partnercenter.models.partners.LegalBusinessProfile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of partnercenter Show documentation
Show all versions of partnercenter Show documentation
SDK for accessing Microsoft Partner Center API.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT license. See the LICENSE file in the project root for full license information.
package com.microsoft.store.partnercenter.models.partners;
import com.microsoft.store.partnercenter.models.Address;
import com.microsoft.store.partnercenter.models.Contact;
import com.microsoft.store.partnercenter.models.ResourceBaseWithLinks;
import com.microsoft.store.partnercenter.models.StandardResourceLinks;
/**
* The legal business profile.
*/
public class LegalBusinessProfile
extends ResourceBaseWithLinks
{
/**
* Initializes a new instance of the LegalBusinessProfile class.
*/
public LegalBusinessProfile()
{
}
/**
* Gets or sets the legal company name.
*/
private String __CompanyName;
public String getCompanyName()
{
return __CompanyName;
}
public void setCompanyName(String value)
{
__CompanyName = value;
}
/**
* Gets or sets the address.
*/
private Address __Address;
public Address getAddress()
{
return __Address;
}
public void setAddress(Address value)
{
__Address = value;
}
/**
* Gets or sets the primary contact.
*/
private Contact __PrimaryContact;
public Contact getPrimaryContact()
{
return __PrimaryContact;
}
public void setPrimaryContact(Contact value)
{
__PrimaryContact = value;
}
/**
* Gets or sets the company approver address.
*/
private Address __CompanyApproverAddress;
public Address getCompanyApproverAddress()
{
return __CompanyApproverAddress;
}
public void setCompanyApproverAddress(Address value)
{
__CompanyApproverAddress = value;
}
/**
* Gets or sets the company approver email.
*/
private String __CompanyApproverEmail;
public String getCompanyApproverEmail()
{
return __CompanyApproverEmail;
}
public void setCompanyApproverEmail(String value)
{
__CompanyApproverEmail = value;
}
/**
* Gets or sets the vetting status.
*/
private VettingStatus __VettingStatus;
public VettingStatus getVettingStatus()
{
return __VettingStatus;
}
public void setVettingStatus(VettingStatus value)
{
__VettingStatus = value;
}
/**
* Gets or sets the vetting sub status.
*/
private VettingSubStatus __VettingSubStatus;
public VettingSubStatus getVettingSubStatus()
{
return __VettingSubStatus;
}
public void setVettingSubStatus(VettingSubStatus value)
{
__VettingSubStatus = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy