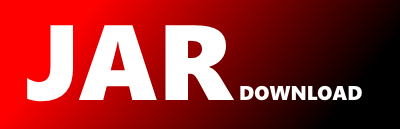
com.microsoft.store.partnercenter.offers.OfferCollectionOperations Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of partnercenter Show documentation
Show all versions of partnercenter Show documentation
SDK for accessing Microsoft Partner Center API.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT license. See the LICENSE file in the project root for full license information.
package com.microsoft.store.partnercenter.offers;
import java.util.ArrayList;
import java.util.Collection;
import com.fasterxml.jackson.core.type.TypeReference;
import com.microsoft.store.partnercenter.BasePartnerComponentString;
import com.microsoft.store.partnercenter.IPartner;
import com.microsoft.store.partnercenter.PartnerService;
import com.microsoft.store.partnercenter.models.ResourceCollection;
import com.microsoft.store.partnercenter.models.offers.Offer;
import com.microsoft.store.partnercenter.models.utils.KeyValuePair;
import com.microsoft.store.partnercenter.utils.ParameterValidator;
/**
* Offer Collection operations implementation.
*/
public class OfferCollectionOperations
extends BasePartnerComponentString
implements IOfferCollection
{
/**
* Initializes a new instance of the OfferCollectionOperations class.
*
* @param rootPartnerOperations The root partner operations instance.
* @param country The country on which to base the offers
*/
public OfferCollectionOperations(IPartner rootPartnerOperations, String country)
{
super(rootPartnerOperations, country);
ParameterValidator.isValidCountryCode(country);
}
/**
* Retrieves the operations tied with a specific offer.
*
* @param offerId The offer id.
* @return The offer operations.
*/
@Override
public IOffer byId(String offerId)
{
return new OfferOperations(this.getPartner(), offerId, this.getContext());
}
/**
* Retrieves all the offers for the provided country.
*
* @return All offers for the provided country.
*/
@Override
public ResourceCollection get()
{
Collection> parameters = new ArrayList>();
parameters.add
(
new KeyValuePair
(
PartnerService.getInstance().getConfiguration().getApis().get("GetOffers").getParameters().get("Country"),
this.getContext()
)
);
return this.getPartner().getServiceClient().get(
this.getPartner(),
new TypeReference>(){},
PartnerService.getInstance().getConfiguration().getApis().get("GetOffers").getPath(),
parameters);
}
/**
* Retrieves all the offers for the provided country.
*
* @param offset The starting index.
* @param size The maximum number of offers to return.
* @return All offers for the provided country.
*/
@Override
public ResourceCollection get(int offset, int size)
{
Collection> parameters = new ArrayList>();
parameters.add
(
new KeyValuePair
(
PartnerService.getInstance().getConfiguration().getApis().get("GetOffers").getParameters().get("Country"),
this.getContext()
)
);
parameters.add
(
new KeyValuePair
(
PartnerService.getInstance().getConfiguration().getApis().get("GetOffers").getParameters().get("Offset"),
String.valueOf(offset)
)
);
parameters.add
(
new KeyValuePair
(
PartnerService.getInstance().getConfiguration().getApis().get("GetOffers").getParameters().get("Size"),
String.valueOf(size)
)
);
return this.getPartner().getServiceClient().get(
this.getPartner(),
new TypeReference>(){},
PartnerService.getInstance().getConfiguration().getApis().get("GetOffers").getPath(),
parameters);
}
/**
* Retrieves the operations that can be applied on offers the belong to an offer category.
* @param categoryId The offer category Id.
* @return The category offers operations.
*/
public ICategoryOffersCollection byCategory(String categoryId)
{
return new CategoryOffersCollectionOperations(this.getPartner(), categoryId, this.getContext());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy