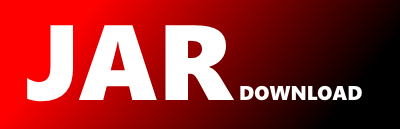
com.microsoft.store.partnercenter.servicerequests.CustomerServiceRequestCollectionOperations Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of partnercenter Show documentation
Show all versions of partnercenter Show documentation
SDK for accessing Microsoft Partner Center API.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT license. See the LICENSE file in the project root for full license information.
package com.microsoft.store.partnercenter.servicerequests;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.text.MessageFormat;
import java.util.ArrayList;
import java.util.Collection;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.microsoft.store.partnercenter.BasePartnerComponentString;
import com.microsoft.store.partnercenter.IPartner;
import com.microsoft.store.partnercenter.PartnerService;
import com.microsoft.store.partnercenter.exception.PartnerErrorCategory;
import com.microsoft.store.partnercenter.exception.PartnerException;
import com.microsoft.store.partnercenter.models.ResourceCollection;
import com.microsoft.store.partnercenter.models.SeekBasedResourceCollection;
import com.microsoft.store.partnercenter.models.query.IQuery;
import com.microsoft.store.partnercenter.models.query.QueryType;
import com.microsoft.store.partnercenter.models.servicerequests.ServiceRequest;
import com.microsoft.store.partnercenter.models.utils.KeyValuePair;
import com.microsoft.store.partnercenter.utils.ParameterValidator;
import com.microsoft.store.partnercenter.utils.StringHelper;
/**
* The customer's service requests operations implementation.
*/
public class CustomerServiceRequestCollectionOperations
extends BasePartnerComponentString
implements IServiceRequestCollection
{
/**
* The minimum allowed page size for the collection.
*/
private static final int MIN_PAGE_SIZE = 1;
/**
* The maximum allowed page size for the collection.
*/
private static final int MAX_PAGE_SIZE = 1000;
/**
* Initializes a new instance of the CustomerServiceRequestCollectionOperations class.
*
* @param rootPartnerOperations The root partner operations instance.
* @param customerId The customer identifier.
*/
public CustomerServiceRequestCollectionOperations(IPartner rootPartnerOperations, String customerId)
{
super(rootPartnerOperations, customerId);
if (StringHelper.isNullOrWhiteSpace(customerId))
{
throw new IllegalArgumentException("customerId can't be null");
}
}
/**
* Retrieves a Service Request specified by Id
*
* @param serviceRequestId Service Request Id
* @return Service Request Operations
*/
@Override
public IServiceRequest byId(String serviceRequestId)
{
return new CustomerServiceRequestOperations(this.getPartner(), this.getContext(), serviceRequestId);
}
/**
* Queries for service requests associated with a customer
*
* @param serviceRequestsQuery The query with search parameters
* @return A collection of service requests matching the criteria
*/
@Override
public SeekBasedResourceCollection query(IQuery serviceRequestsQuery)
{
if (serviceRequestsQuery == null)
{
throw new IllegalArgumentException("serviceRequestsQuery can't be null");
}
if (serviceRequestsQuery.getType() != QueryType.INDEXED && serviceRequestsQuery.getType() != QueryType.SIMPLE)
{
throw new IllegalArgumentException("Specified query type is not supported.");
}
Collection> parameters = new ArrayList>();
if (serviceRequestsQuery.getType() == QueryType.INDEXED)
{
// if the query specifies a page size, validate it and add it to the request
ParameterValidator.isIntInclusive(MIN_PAGE_SIZE, MAX_PAGE_SIZE, serviceRequestsQuery.getPageSize(),
MessageFormat.format("Allowed page size values are from {0}-{1}",
MIN_PAGE_SIZE, MAX_PAGE_SIZE));
parameters.add(
new KeyValuePair(
PartnerService.getInstance().getConfiguration().getApis().get("SearchCustomerServiceRequests").getParameters().get("Size"),
String.valueOf(serviceRequestsQuery.getPageSize())));
parameters.add(
new KeyValuePair(
PartnerService.getInstance().getConfiguration().getApis().get("SearchCustomerServiceRequests").getParameters().get("Offset"),
String.valueOf(serviceRequestsQuery.getIndex())));
}
if (serviceRequestsQuery.getFilter() != null)
{
// add the filter to the request if specified
ObjectMapper mapper = new ObjectMapper();
try
{
parameters.add(
new KeyValuePair(
PartnerService.getInstance().getConfiguration().getApis().get("SearchCustomerServiceRequests").getParameters().get("Filter"),
URLEncoder.encode(mapper.writeValueAsString(serviceRequestsQuery.getFilter()),
"UTF-8")));
}
catch (JsonProcessingException e)
{
throw new PartnerException("", null, PartnerErrorCategory.REQUEST_PARSING, e);
}
catch (UnsupportedEncodingException e)
{
throw new PartnerException("", null, PartnerErrorCategory.REQUEST_PARSING, e);
}
}
return this.getPartner().getServiceClient().get(
this.getPartner(),
new TypeReference>(){},
MessageFormat.format(
PartnerService.getInstance().getConfiguration().getApis().get("SearchCustomerServiceRequests").getPath(),
this.getContext()),
parameters);
}
/**
* Retrieves Service Requests associated to the customer.
*
* @return A collection of service requests
*/
@Override
public ResourceCollection get()
{
return this.getPartner().getServiceClient().get(
this.getPartner(),
new TypeReference>(){},
MessageFormat.format(
PartnerService.getInstance().getConfiguration().getApis().get("GetAllServiceRequestsCustomer").getPath(),
this.getContext()));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy