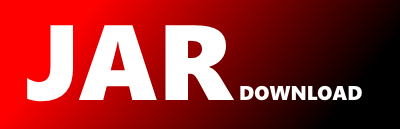
com.microsoft.store.partnercenter.models.ResourceCollectionWithLinks Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of partnercenter Show documentation
Show all versions of partnercenter Show documentation
SDK for accessing Microsoft Partner Center API.
// -----------------------------------------------------------------------
//
// Copyright (c) Microsoft Corporation. All rights reserved.
//
// -----------------------------------------------------------------------
package com.microsoft.store.partnercenter.models;
import java.util.ArrayList;
import java.util.List;
/**
* Contains a collection of resources with JSON properties to represent the output Type of objects in collection
*/
public class ResourceCollectionWithLinks
extends ResourceBaseWithLinks
{
public ResourceCollectionWithLinks()
{
super( "Collection" );
this.items = new ArrayList();
}
/**
* The collection items
*/
private List items;
/**
* Initializes a new instance of the ResourceCollection class.
*
* @param items The items.
*/
public ResourceCollectionWithLinks( List items )
{
super( "Collection" );
this.items = items;
}
/**
* Initializes a new instance of the ResourceCollection class.
*
* @param resourceCollection The resource collection.
*/
protected ResourceCollectionWithLinks( ResourceCollectionWithLinks resourceCollection )
{
super( "Collection" );
if ( resourceCollection == null )
{
throw new IllegalArgumentException( "resourceCollection" );
}
this.items = resourceCollection.items;
}
private int totalCount;
/**
* Gets the total count.
*
* @return The total count.
*/
public int getTotalCount()
{
return this.totalCount;
}
public void setTotalCount( int value )
{
this.totalCount = value;
}
/**
* Gets the collection items.
*
* @return The items in the collection.
*/
public Iterable getItems()
{
return this.items;
}
/**
* Sets the collection items.
*
* @param value The items in the collection.
*/
public void setItems( List value )
{
this.items = value;
}
/**
* Converts this object to a string.
*
* @return A string that represents this object.
*/
@Override
public String toString()
{
StringBuilder collectionDescription = new StringBuilder();
collectionDescription.append( "Count: " + this.getTotalCount() + "\n" );
if ( this.getItems() != null )
{
collectionDescription.append( "Items:\n" );
for ( TResource item : this.getItems() )
{
collectionDescription.append( item.toString() + "\n" );
}
}
return collectionDescription.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy