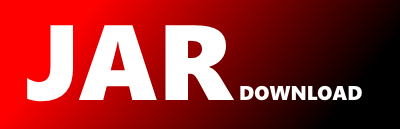
com.microsoft.thrifty.schema.antlr.AntlrThriftParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of thrifty-schema Show documentation
Show all versions of thrifty-schema Show documentation
A simple Thrift IDL parser and validator
// Generated from com/microsoft/thrifty/schema/antlr/AntlrThrift.g4 by ANTLR 4.7.1
package com.microsoft.thrifty.schema.antlr;
import org.antlr.v4.runtime.atn.*;
import org.antlr.v4.runtime.dfa.DFA;
import org.antlr.v4.runtime.*;
import org.antlr.v4.runtime.misc.*;
import org.antlr.v4.runtime.tree.*;
import java.util.List;
import java.util.Iterator;
import java.util.ArrayList;
@SuppressWarnings({"all", "warnings", "unchecked", "unused", "cast"})
public class AntlrThriftParser extends Parser {
static { RuntimeMetaData.checkVersion("4.7.1", RuntimeMetaData.VERSION); }
protected static final DFA[] _decisionToDFA;
protected static final PredictionContextCache _sharedContextCache =
new PredictionContextCache();
public static final int
T__0=1, T__1=2, T__2=3, T__3=4, T__4=5, T__5=6, T__6=7, T__7=8, T__8=9,
T__9=10, T__10=11, T__11=12, T__12=13, T__13=14, T__14=15, T__15=16, T__16=17,
T__17=18, T__18=19, T__19=20, T__20=21, T__21=22, T__22=23, T__23=24,
T__24=25, T__25=26, T__26=27, T__27=28, T__28=29, T__29=30, T__30=31,
T__31=32, T__32=33, T__33=34, T__34=35, T__35=36, T__36=37, T__37=38,
T__38=39, T__39=40, T__40=41, T__41=42, LITERAL=43, VOID=44, ONEWAY=45,
COMMA=46, SEMICOLON=47, IDENTIFIER=48, NS_SCOPE=49, INTEGER=50, DOUBLE=51,
SLASH_SLASH_COMMENT=52, HASH_COMMENT=53, MULTILINE_COMMENT=54, NEWLINE=55,
WHITESPACE=56;
public static final int
RULE_document = 0, RULE_header = 1, RULE_include = 2, RULE_cppInclude = 3,
RULE_namespace = 4, RULE_standardNamespace = 5, RULE_namespaceScope = 6,
RULE_phpNamespace = 7, RULE_xsdNamespace = 8, RULE_definition = 9, RULE_constDef = 10,
RULE_constValue = 11, RULE_constList = 12, RULE_constMap = 13, RULE_constMapEntry = 14,
RULE_typedef = 15, RULE_enumDef = 16, RULE_enumMember = 17, RULE_senum = 18,
RULE_structDef = 19, RULE_unionDef = 20, RULE_exceptionDef = 21, RULE_serviceDef = 22,
RULE_function = 23, RULE_fieldList = 24, RULE_field = 25, RULE_requiredness = 26,
RULE_throwsList = 27, RULE_fieldType = 28, RULE_baseType = 29, RULE_containerType = 30,
RULE_mapType = 31, RULE_listType = 32, RULE_setType = 33, RULE_cppType = 34,
RULE_annotationList = 35, RULE_annotation = 36, RULE_separator = 37;
public static final String[] ruleNames = {
"document", "header", "include", "cppInclude", "namespace", "standardNamespace",
"namespaceScope", "phpNamespace", "xsdNamespace", "definition", "constDef",
"constValue", "constList", "constMap", "constMapEntry", "typedef", "enumDef",
"enumMember", "senum", "structDef", "unionDef", "exceptionDef", "serviceDef",
"function", "fieldList", "field", "requiredness", "throwsList", "fieldType",
"baseType", "containerType", "mapType", "listType", "setType", "cppType",
"annotationList", "annotation", "separator"
};
private static final String[] _LITERAL_NAMES = {
null, "'include'", "'cpp_include'", "'namespace'", "'*'", "'php_namespace'",
"'xsd_namespace'", "'const'", "'='", "'['", "']'", "'{'", "'}'", "':'",
"'typedef'", "'enum'", "'senum'", "'struct'", "'union'", "'exception'",
"'service'", "'extends'", "'('", "')'", "'optional'", "'required'", "'throws'",
"'bool'", "'byte'", "'i8'", "'i16'", "'i32'", "'i64'", "'double'", "'string'",
"'binary'", "'slist'", "'map'", "'<'", "'>'", "'list'", "'set'", "'cpp_type'",
null, "'void'", "'oneway'", "','", "';'"
};
private static final String[] _SYMBOLIC_NAMES = {
null, null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, null, null, "LITERAL", "VOID", "ONEWAY",
"COMMA", "SEMICOLON", "IDENTIFIER", "NS_SCOPE", "INTEGER", "DOUBLE", "SLASH_SLASH_COMMENT",
"HASH_COMMENT", "MULTILINE_COMMENT", "NEWLINE", "WHITESPACE"
};
public static final Vocabulary VOCABULARY = new VocabularyImpl(_LITERAL_NAMES, _SYMBOLIC_NAMES);
/**
* @deprecated Use {@link #VOCABULARY} instead.
*/
@Deprecated
public static final String[] tokenNames;
static {
tokenNames = new String[_SYMBOLIC_NAMES.length];
for (int i = 0; i < tokenNames.length; i++) {
tokenNames[i] = VOCABULARY.getLiteralName(i);
if (tokenNames[i] == null) {
tokenNames[i] = VOCABULARY.getSymbolicName(i);
}
if (tokenNames[i] == null) {
tokenNames[i] = "";
}
}
}
@Override
@Deprecated
public String[] getTokenNames() {
return tokenNames;
}
@Override
public Vocabulary getVocabulary() {
return VOCABULARY;
}
@Override
public String getGrammarFileName() { return "AntlrThrift.g4"; }
@Override
public String[] getRuleNames() { return ruleNames; }
@Override
public String getSerializedATN() { return _serializedATN; }
@Override
public ATN getATN() { return _ATN; }
public AntlrThriftParser(TokenStream input) {
super(input);
_interp = new ParserATNSimulator(this,_ATN,_decisionToDFA,_sharedContextCache);
}
public static class DocumentContext extends ParserRuleContext {
public List header() {
return getRuleContexts(HeaderContext.class);
}
public HeaderContext header(int i) {
return getRuleContext(HeaderContext.class,i);
}
public List definition() {
return getRuleContexts(DefinitionContext.class);
}
public DefinitionContext definition(int i) {
return getRuleContext(DefinitionContext.class,i);
}
public DocumentContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_document; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterDocument(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitDocument(this);
}
}
public final DocumentContext document() throws RecognitionException {
DocumentContext _localctx = new DocumentContext(_ctx, getState());
enterRule(_localctx, 0, RULE_document);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(79);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << T__0) | (1L << T__1) | (1L << T__2) | (1L << T__4) | (1L << T__5))) != 0)) {
{
{
setState(76);
header();
}
}
setState(81);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(85);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << T__6) | (1L << T__13) | (1L << T__14) | (1L << T__15) | (1L << T__16) | (1L << T__17) | (1L << T__18) | (1L << T__19))) != 0)) {
{
{
setState(82);
definition();
}
}
setState(87);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class HeaderContext extends ParserRuleContext {
public IncludeContext include() {
return getRuleContext(IncludeContext.class,0);
}
public CppIncludeContext cppInclude() {
return getRuleContext(CppIncludeContext.class,0);
}
public NamespaceContext namespace() {
return getRuleContext(NamespaceContext.class,0);
}
public HeaderContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_header; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterHeader(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitHeader(this);
}
}
public final HeaderContext header() throws RecognitionException {
HeaderContext _localctx = new HeaderContext(_ctx, getState());
enterRule(_localctx, 2, RULE_header);
try {
setState(91);
_errHandler.sync(this);
switch (_input.LA(1)) {
case T__0:
enterOuterAlt(_localctx, 1);
{
setState(88);
include();
}
break;
case T__1:
enterOuterAlt(_localctx, 2);
{
setState(89);
cppInclude();
}
break;
case T__2:
case T__4:
case T__5:
enterOuterAlt(_localctx, 3);
{
setState(90);
namespace();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class IncludeContext extends ParserRuleContext {
public TerminalNode LITERAL() { return getToken(AntlrThriftParser.LITERAL, 0); }
public SeparatorContext separator() {
return getRuleContext(SeparatorContext.class,0);
}
public IncludeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_include; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterInclude(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitInclude(this);
}
}
public final IncludeContext include() throws RecognitionException {
IncludeContext _localctx = new IncludeContext(_ctx, getState());
enterRule(_localctx, 4, RULE_include);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(93);
match(T__0);
setState(94);
match(LITERAL);
setState(96);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COMMA || _la==SEMICOLON) {
{
setState(95);
separator();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class CppIncludeContext extends ParserRuleContext {
public TerminalNode LITERAL() { return getToken(AntlrThriftParser.LITERAL, 0); }
public SeparatorContext separator() {
return getRuleContext(SeparatorContext.class,0);
}
public CppIncludeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_cppInclude; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterCppInclude(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitCppInclude(this);
}
}
public final CppIncludeContext cppInclude() throws RecognitionException {
CppIncludeContext _localctx = new CppIncludeContext(_ctx, getState());
enterRule(_localctx, 6, RULE_cppInclude);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(98);
match(T__1);
setState(99);
match(LITERAL);
setState(101);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COMMA || _la==SEMICOLON) {
{
setState(100);
separator();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class NamespaceContext extends ParserRuleContext {
public StandardNamespaceContext standardNamespace() {
return getRuleContext(StandardNamespaceContext.class,0);
}
public PhpNamespaceContext phpNamespace() {
return getRuleContext(PhpNamespaceContext.class,0);
}
public XsdNamespaceContext xsdNamespace() {
return getRuleContext(XsdNamespaceContext.class,0);
}
public NamespaceContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_namespace; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterNamespace(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitNamespace(this);
}
}
public final NamespaceContext namespace() throws RecognitionException {
NamespaceContext _localctx = new NamespaceContext(_ctx, getState());
enterRule(_localctx, 8, RULE_namespace);
try {
setState(106);
_errHandler.sync(this);
switch (_input.LA(1)) {
case T__2:
enterOuterAlt(_localctx, 1);
{
setState(103);
standardNamespace();
}
break;
case T__4:
enterOuterAlt(_localctx, 2);
{
setState(104);
phpNamespace();
}
break;
case T__5:
enterOuterAlt(_localctx, 3);
{
setState(105);
xsdNamespace();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class StandardNamespaceContext extends ParserRuleContext {
public Token ns;
public NamespaceScopeContext namespaceScope() {
return getRuleContext(NamespaceScopeContext.class,0);
}
public TerminalNode IDENTIFIER() { return getToken(AntlrThriftParser.IDENTIFIER, 0); }
public AnnotationListContext annotationList() {
return getRuleContext(AnnotationListContext.class,0);
}
public SeparatorContext separator() {
return getRuleContext(SeparatorContext.class,0);
}
public StandardNamespaceContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_standardNamespace; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterStandardNamespace(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitStandardNamespace(this);
}
}
public final StandardNamespaceContext standardNamespace() throws RecognitionException {
StandardNamespaceContext _localctx = new StandardNamespaceContext(_ctx, getState());
enterRule(_localctx, 10, RULE_standardNamespace);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(108);
match(T__2);
setState(109);
namespaceScope();
setState(110);
((StandardNamespaceContext)_localctx).ns = match(IDENTIFIER);
setState(112);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==T__21) {
{
setState(111);
annotationList();
}
}
setState(115);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COMMA || _la==SEMICOLON) {
{
setState(114);
separator();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class NamespaceScopeContext extends ParserRuleContext {
public TerminalNode IDENTIFIER() { return getToken(AntlrThriftParser.IDENTIFIER, 0); }
public NamespaceScopeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_namespaceScope; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterNamespaceScope(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitNamespaceScope(this);
}
}
public final NamespaceScopeContext namespaceScope() throws RecognitionException {
NamespaceScopeContext _localctx = new NamespaceScopeContext(_ctx, getState());
enterRule(_localctx, 12, RULE_namespaceScope);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(117);
_la = _input.LA(1);
if ( !(_la==T__3 || _la==IDENTIFIER) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class PhpNamespaceContext extends ParserRuleContext {
public Token ns;
public TerminalNode LITERAL() { return getToken(AntlrThriftParser.LITERAL, 0); }
public AnnotationListContext annotationList() {
return getRuleContext(AnnotationListContext.class,0);
}
public SeparatorContext separator() {
return getRuleContext(SeparatorContext.class,0);
}
public PhpNamespaceContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_phpNamespace; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterPhpNamespace(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitPhpNamespace(this);
}
}
public final PhpNamespaceContext phpNamespace() throws RecognitionException {
PhpNamespaceContext _localctx = new PhpNamespaceContext(_ctx, getState());
enterRule(_localctx, 14, RULE_phpNamespace);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(119);
match(T__4);
setState(120);
((PhpNamespaceContext)_localctx).ns = match(LITERAL);
setState(122);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==T__21) {
{
setState(121);
annotationList();
}
}
setState(125);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COMMA || _la==SEMICOLON) {
{
setState(124);
separator();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class XsdNamespaceContext extends ParserRuleContext {
public Token ns;
public TerminalNode LITERAL() { return getToken(AntlrThriftParser.LITERAL, 0); }
public AnnotationListContext annotationList() {
return getRuleContext(AnnotationListContext.class,0);
}
public SeparatorContext separator() {
return getRuleContext(SeparatorContext.class,0);
}
public XsdNamespaceContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_xsdNamespace; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterXsdNamespace(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitXsdNamespace(this);
}
}
public final XsdNamespaceContext xsdNamespace() throws RecognitionException {
XsdNamespaceContext _localctx = new XsdNamespaceContext(_ctx, getState());
enterRule(_localctx, 16, RULE_xsdNamespace);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(127);
match(T__5);
setState(128);
((XsdNamespaceContext)_localctx).ns = match(LITERAL);
setState(130);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==T__21) {
{
setState(129);
annotationList();
}
}
setState(133);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COMMA || _la==SEMICOLON) {
{
setState(132);
separator();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class DefinitionContext extends ParserRuleContext {
public ConstDefContext constDef() {
return getRuleContext(ConstDefContext.class,0);
}
public TypedefContext typedef() {
return getRuleContext(TypedefContext.class,0);
}
public EnumDefContext enumDef() {
return getRuleContext(EnumDefContext.class,0);
}
public SenumContext senum() {
return getRuleContext(SenumContext.class,0);
}
public StructDefContext structDef() {
return getRuleContext(StructDefContext.class,0);
}
public UnionDefContext unionDef() {
return getRuleContext(UnionDefContext.class,0);
}
public ExceptionDefContext exceptionDef() {
return getRuleContext(ExceptionDefContext.class,0);
}
public ServiceDefContext serviceDef() {
return getRuleContext(ServiceDefContext.class,0);
}
public DefinitionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_definition; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterDefinition(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitDefinition(this);
}
}
public final DefinitionContext definition() throws RecognitionException {
DefinitionContext _localctx = new DefinitionContext(_ctx, getState());
enterRule(_localctx, 18, RULE_definition);
try {
setState(143);
_errHandler.sync(this);
switch (_input.LA(1)) {
case T__6:
enterOuterAlt(_localctx, 1);
{
setState(135);
constDef();
}
break;
case T__13:
enterOuterAlt(_localctx, 2);
{
setState(136);
typedef();
}
break;
case T__14:
enterOuterAlt(_localctx, 3);
{
setState(137);
enumDef();
}
break;
case T__15:
enterOuterAlt(_localctx, 4);
{
setState(138);
senum();
}
break;
case T__16:
enterOuterAlt(_localctx, 5);
{
setState(139);
structDef();
}
break;
case T__17:
enterOuterAlt(_localctx, 6);
{
setState(140);
unionDef();
}
break;
case T__18:
enterOuterAlt(_localctx, 7);
{
setState(141);
exceptionDef();
}
break;
case T__19:
enterOuterAlt(_localctx, 8);
{
setState(142);
serviceDef();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ConstDefContext extends ParserRuleContext {
public FieldTypeContext fieldType() {
return getRuleContext(FieldTypeContext.class,0);
}
public TerminalNode IDENTIFIER() { return getToken(AntlrThriftParser.IDENTIFIER, 0); }
public ConstValueContext constValue() {
return getRuleContext(ConstValueContext.class,0);
}
public SeparatorContext separator() {
return getRuleContext(SeparatorContext.class,0);
}
public ConstDefContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_constDef; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterConstDef(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitConstDef(this);
}
}
public final ConstDefContext constDef() throws RecognitionException {
ConstDefContext _localctx = new ConstDefContext(_ctx, getState());
enterRule(_localctx, 20, RULE_constDef);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(145);
match(T__6);
setState(146);
fieldType();
setState(147);
match(IDENTIFIER);
setState(148);
match(T__7);
setState(149);
constValue();
setState(151);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COMMA || _la==SEMICOLON) {
{
setState(150);
separator();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ConstValueContext extends ParserRuleContext {
public TerminalNode INTEGER() { return getToken(AntlrThriftParser.INTEGER, 0); }
public TerminalNode DOUBLE() { return getToken(AntlrThriftParser.DOUBLE, 0); }
public TerminalNode LITERAL() { return getToken(AntlrThriftParser.LITERAL, 0); }
public TerminalNode IDENTIFIER() { return getToken(AntlrThriftParser.IDENTIFIER, 0); }
public ConstListContext constList() {
return getRuleContext(ConstListContext.class,0);
}
public ConstMapContext constMap() {
return getRuleContext(ConstMapContext.class,0);
}
public ConstValueContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_constValue; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterConstValue(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitConstValue(this);
}
}
public final ConstValueContext constValue() throws RecognitionException {
ConstValueContext _localctx = new ConstValueContext(_ctx, getState());
enterRule(_localctx, 22, RULE_constValue);
try {
setState(159);
_errHandler.sync(this);
switch (_input.LA(1)) {
case INTEGER:
enterOuterAlt(_localctx, 1);
{
setState(153);
match(INTEGER);
}
break;
case DOUBLE:
enterOuterAlt(_localctx, 2);
{
setState(154);
match(DOUBLE);
}
break;
case LITERAL:
enterOuterAlt(_localctx, 3);
{
setState(155);
match(LITERAL);
}
break;
case IDENTIFIER:
enterOuterAlt(_localctx, 4);
{
setState(156);
match(IDENTIFIER);
}
break;
case T__8:
enterOuterAlt(_localctx, 5);
{
setState(157);
constList();
}
break;
case T__10:
enterOuterAlt(_localctx, 6);
{
setState(158);
constMap();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ConstListContext extends ParserRuleContext {
public List constValue() {
return getRuleContexts(ConstValueContext.class);
}
public ConstValueContext constValue(int i) {
return getRuleContext(ConstValueContext.class,i);
}
public List separator() {
return getRuleContexts(SeparatorContext.class);
}
public SeparatorContext separator(int i) {
return getRuleContext(SeparatorContext.class,i);
}
public ConstListContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_constList; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterConstList(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitConstList(this);
}
}
public final ConstListContext constList() throws RecognitionException {
ConstListContext _localctx = new ConstListContext(_ctx, getState());
enterRule(_localctx, 24, RULE_constList);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(161);
match(T__8);
setState(168);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << T__8) | (1L << T__10) | (1L << LITERAL) | (1L << IDENTIFIER) | (1L << INTEGER) | (1L << DOUBLE))) != 0)) {
{
{
setState(162);
constValue();
setState(164);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COMMA || _la==SEMICOLON) {
{
setState(163);
separator();
}
}
}
}
setState(170);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(171);
match(T__9);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ConstMapContext extends ParserRuleContext {
public List constMapEntry() {
return getRuleContexts(ConstMapEntryContext.class);
}
public ConstMapEntryContext constMapEntry(int i) {
return getRuleContext(ConstMapEntryContext.class,i);
}
public List separator() {
return getRuleContexts(SeparatorContext.class);
}
public SeparatorContext separator(int i) {
return getRuleContext(SeparatorContext.class,i);
}
public ConstMapContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_constMap; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterConstMap(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitConstMap(this);
}
}
public final ConstMapContext constMap() throws RecognitionException {
ConstMapContext _localctx = new ConstMapContext(_ctx, getState());
enterRule(_localctx, 26, RULE_constMap);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(173);
match(T__10);
setState(180);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << T__8) | (1L << T__10) | (1L << LITERAL) | (1L << IDENTIFIER) | (1L << INTEGER) | (1L << DOUBLE))) != 0)) {
{
{
setState(174);
constMapEntry();
setState(176);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COMMA || _la==SEMICOLON) {
{
setState(175);
separator();
}
}
}
}
setState(182);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(183);
match(T__11);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ConstMapEntryContext extends ParserRuleContext {
public ConstValueContext key;
public ConstValueContext value;
public List constValue() {
return getRuleContexts(ConstValueContext.class);
}
public ConstValueContext constValue(int i) {
return getRuleContext(ConstValueContext.class,i);
}
public ConstMapEntryContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_constMapEntry; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterConstMapEntry(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitConstMapEntry(this);
}
}
public final ConstMapEntryContext constMapEntry() throws RecognitionException {
ConstMapEntryContext _localctx = new ConstMapEntryContext(_ctx, getState());
enterRule(_localctx, 28, RULE_constMapEntry);
try {
enterOuterAlt(_localctx, 1);
{
setState(185);
((ConstMapEntryContext)_localctx).key = constValue();
setState(186);
match(T__12);
setState(187);
((ConstMapEntryContext)_localctx).value = constValue();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class TypedefContext extends ParserRuleContext {
public FieldTypeContext fieldType() {
return getRuleContext(FieldTypeContext.class,0);
}
public TerminalNode IDENTIFIER() { return getToken(AntlrThriftParser.IDENTIFIER, 0); }
public AnnotationListContext annotationList() {
return getRuleContext(AnnotationListContext.class,0);
}
public SeparatorContext separator() {
return getRuleContext(SeparatorContext.class,0);
}
public TypedefContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_typedef; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterTypedef(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitTypedef(this);
}
}
public final TypedefContext typedef() throws RecognitionException {
TypedefContext _localctx = new TypedefContext(_ctx, getState());
enterRule(_localctx, 30, RULE_typedef);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(189);
match(T__13);
setState(190);
fieldType();
setState(191);
match(IDENTIFIER);
setState(193);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==T__21) {
{
setState(192);
annotationList();
}
}
setState(196);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COMMA || _la==SEMICOLON) {
{
setState(195);
separator();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class EnumDefContext extends ParserRuleContext {
public TerminalNode IDENTIFIER() { return getToken(AntlrThriftParser.IDENTIFIER, 0); }
public List enumMember() {
return getRuleContexts(EnumMemberContext.class);
}
public EnumMemberContext enumMember(int i) {
return getRuleContext(EnumMemberContext.class,i);
}
public AnnotationListContext annotationList() {
return getRuleContext(AnnotationListContext.class,0);
}
public EnumDefContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_enumDef; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterEnumDef(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitEnumDef(this);
}
}
public final EnumDefContext enumDef() throws RecognitionException {
EnumDefContext _localctx = new EnumDefContext(_ctx, getState());
enterRule(_localctx, 32, RULE_enumDef);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(198);
match(T__14);
setState(199);
match(IDENTIFIER);
setState(200);
match(T__10);
setState(204);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==IDENTIFIER) {
{
{
setState(201);
enumMember();
}
}
setState(206);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(207);
match(T__11);
setState(209);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==T__21) {
{
setState(208);
annotationList();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class EnumMemberContext extends ParserRuleContext {
public TerminalNode IDENTIFIER() { return getToken(AntlrThriftParser.IDENTIFIER, 0); }
public TerminalNode INTEGER() { return getToken(AntlrThriftParser.INTEGER, 0); }
public AnnotationListContext annotationList() {
return getRuleContext(AnnotationListContext.class,0);
}
public SeparatorContext separator() {
return getRuleContext(SeparatorContext.class,0);
}
public EnumMemberContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_enumMember; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterEnumMember(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitEnumMember(this);
}
}
public final EnumMemberContext enumMember() throws RecognitionException {
EnumMemberContext _localctx = new EnumMemberContext(_ctx, getState());
enterRule(_localctx, 34, RULE_enumMember);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(211);
match(IDENTIFIER);
setState(214);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==T__7) {
{
setState(212);
match(T__7);
setState(213);
match(INTEGER);
}
}
setState(217);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==T__21) {
{
setState(216);
annotationList();
}
}
setState(220);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COMMA || _la==SEMICOLON) {
{
setState(219);
separator();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SenumContext extends ParserRuleContext {
public TerminalNode IDENTIFIER() { return getToken(AntlrThriftParser.IDENTIFIER, 0); }
public List enumMember() {
return getRuleContexts(EnumMemberContext.class);
}
public EnumMemberContext enumMember(int i) {
return getRuleContext(EnumMemberContext.class,i);
}
public SenumContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_senum; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterSenum(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitSenum(this);
}
}
public final SenumContext senum() throws RecognitionException {
SenumContext _localctx = new SenumContext(_ctx, getState());
enterRule(_localctx, 36, RULE_senum);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(222);
match(T__15);
setState(223);
match(IDENTIFIER);
setState(224);
match(T__10);
setState(228);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==IDENTIFIER) {
{
{
setState(225);
enumMember();
}
}
setState(230);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(231);
match(T__11);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class StructDefContext extends ParserRuleContext {
public TerminalNode IDENTIFIER() { return getToken(AntlrThriftParser.IDENTIFIER, 0); }
public List field() {
return getRuleContexts(FieldContext.class);
}
public FieldContext field(int i) {
return getRuleContext(FieldContext.class,i);
}
public AnnotationListContext annotationList() {
return getRuleContext(AnnotationListContext.class,0);
}
public StructDefContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_structDef; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterStructDef(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitStructDef(this);
}
}
public final StructDefContext structDef() throws RecognitionException {
StructDefContext _localctx = new StructDefContext(_ctx, getState());
enterRule(_localctx, 38, RULE_structDef);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(233);
match(T__16);
setState(234);
match(IDENTIFIER);
setState(235);
match(T__10);
setState(239);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << T__23) | (1L << T__24) | (1L << T__26) | (1L << T__27) | (1L << T__28) | (1L << T__29) | (1L << T__30) | (1L << T__31) | (1L << T__32) | (1L << T__33) | (1L << T__34) | (1L << T__35) | (1L << T__36) | (1L << T__39) | (1L << T__40) | (1L << IDENTIFIER) | (1L << INTEGER))) != 0)) {
{
{
setState(236);
field();
}
}
setState(241);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(242);
match(T__11);
setState(244);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==T__21) {
{
setState(243);
annotationList();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class UnionDefContext extends ParserRuleContext {
public TerminalNode IDENTIFIER() { return getToken(AntlrThriftParser.IDENTIFIER, 0); }
public List field() {
return getRuleContexts(FieldContext.class);
}
public FieldContext field(int i) {
return getRuleContext(FieldContext.class,i);
}
public AnnotationListContext annotationList() {
return getRuleContext(AnnotationListContext.class,0);
}
public UnionDefContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_unionDef; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterUnionDef(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitUnionDef(this);
}
}
public final UnionDefContext unionDef() throws RecognitionException {
UnionDefContext _localctx = new UnionDefContext(_ctx, getState());
enterRule(_localctx, 40, RULE_unionDef);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(246);
match(T__17);
setState(247);
match(IDENTIFIER);
setState(248);
match(T__10);
setState(252);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << T__23) | (1L << T__24) | (1L << T__26) | (1L << T__27) | (1L << T__28) | (1L << T__29) | (1L << T__30) | (1L << T__31) | (1L << T__32) | (1L << T__33) | (1L << T__34) | (1L << T__35) | (1L << T__36) | (1L << T__39) | (1L << T__40) | (1L << IDENTIFIER) | (1L << INTEGER))) != 0)) {
{
{
setState(249);
field();
}
}
setState(254);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(255);
match(T__11);
setState(257);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==T__21) {
{
setState(256);
annotationList();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ExceptionDefContext extends ParserRuleContext {
public TerminalNode IDENTIFIER() { return getToken(AntlrThriftParser.IDENTIFIER, 0); }
public List field() {
return getRuleContexts(FieldContext.class);
}
public FieldContext field(int i) {
return getRuleContext(FieldContext.class,i);
}
public AnnotationListContext annotationList() {
return getRuleContext(AnnotationListContext.class,0);
}
public ExceptionDefContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_exceptionDef; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterExceptionDef(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitExceptionDef(this);
}
}
public final ExceptionDefContext exceptionDef() throws RecognitionException {
ExceptionDefContext _localctx = new ExceptionDefContext(_ctx, getState());
enterRule(_localctx, 42, RULE_exceptionDef);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(259);
match(T__18);
setState(260);
match(IDENTIFIER);
setState(261);
match(T__10);
setState(265);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << T__23) | (1L << T__24) | (1L << T__26) | (1L << T__27) | (1L << T__28) | (1L << T__29) | (1L << T__30) | (1L << T__31) | (1L << T__32) | (1L << T__33) | (1L << T__34) | (1L << T__35) | (1L << T__36) | (1L << T__39) | (1L << T__40) | (1L << IDENTIFIER) | (1L << INTEGER))) != 0)) {
{
{
setState(262);
field();
}
}
setState(267);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(268);
match(T__11);
setState(270);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==T__21) {
{
setState(269);
annotationList();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ServiceDefContext extends ParserRuleContext {
public Token name;
public FieldTypeContext superType;
public TerminalNode IDENTIFIER() { return getToken(AntlrThriftParser.IDENTIFIER, 0); }
public List function() {
return getRuleContexts(FunctionContext.class);
}
public FunctionContext function(int i) {
return getRuleContext(FunctionContext.class,i);
}
public AnnotationListContext annotationList() {
return getRuleContext(AnnotationListContext.class,0);
}
public FieldTypeContext fieldType() {
return getRuleContext(FieldTypeContext.class,0);
}
public ServiceDefContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_serviceDef; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterServiceDef(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitServiceDef(this);
}
}
public final ServiceDefContext serviceDef() throws RecognitionException {
ServiceDefContext _localctx = new ServiceDefContext(_ctx, getState());
enterRule(_localctx, 44, RULE_serviceDef);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(272);
match(T__19);
setState(273);
((ServiceDefContext)_localctx).name = match(IDENTIFIER);
setState(276);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==T__20) {
{
setState(274);
match(T__20);
setState(275);
((ServiceDefContext)_localctx).superType = fieldType();
}
}
setState(278);
match(T__10);
setState(282);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << T__26) | (1L << T__27) | (1L << T__28) | (1L << T__29) | (1L << T__30) | (1L << T__31) | (1L << T__32) | (1L << T__33) | (1L << T__34) | (1L << T__35) | (1L << T__36) | (1L << T__39) | (1L << T__40) | (1L << VOID) | (1L << ONEWAY) | (1L << IDENTIFIER))) != 0)) {
{
{
setState(279);
function();
}
}
setState(284);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(285);
match(T__11);
setState(287);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==T__21) {
{
setState(286);
annotationList();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class FunctionContext extends ParserRuleContext {
public TerminalNode IDENTIFIER() { return getToken(AntlrThriftParser.IDENTIFIER, 0); }
public FieldListContext fieldList() {
return getRuleContext(FieldListContext.class,0);
}
public TerminalNode VOID() { return getToken(AntlrThriftParser.VOID, 0); }
public FieldTypeContext fieldType() {
return getRuleContext(FieldTypeContext.class,0);
}
public TerminalNode ONEWAY() { return getToken(AntlrThriftParser.ONEWAY, 0); }
public ThrowsListContext throwsList() {
return getRuleContext(ThrowsListContext.class,0);
}
public AnnotationListContext annotationList() {
return getRuleContext(AnnotationListContext.class,0);
}
public SeparatorContext separator() {
return getRuleContext(SeparatorContext.class,0);
}
public FunctionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_function; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterFunction(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitFunction(this);
}
}
public final FunctionContext function() throws RecognitionException {
FunctionContext _localctx = new FunctionContext(_ctx, getState());
enterRule(_localctx, 46, RULE_function);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(290);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==ONEWAY) {
{
setState(289);
match(ONEWAY);
}
}
setState(294);
_errHandler.sync(this);
switch (_input.LA(1)) {
case VOID:
{
setState(292);
match(VOID);
}
break;
case T__26:
case T__27:
case T__28:
case T__29:
case T__30:
case T__31:
case T__32:
case T__33:
case T__34:
case T__35:
case T__36:
case T__39:
case T__40:
case IDENTIFIER:
{
setState(293);
fieldType();
}
break;
default:
throw new NoViableAltException(this);
}
setState(296);
match(IDENTIFIER);
setState(297);
fieldList();
setState(299);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==T__25) {
{
setState(298);
throwsList();
}
}
setState(302);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==T__21) {
{
setState(301);
annotationList();
}
}
setState(305);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COMMA || _la==SEMICOLON) {
{
setState(304);
separator();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class FieldListContext extends ParserRuleContext {
public List field() {
return getRuleContexts(FieldContext.class);
}
public FieldContext field(int i) {
return getRuleContext(FieldContext.class,i);
}
public FieldListContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_fieldList; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterFieldList(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitFieldList(this);
}
}
public final FieldListContext fieldList() throws RecognitionException {
FieldListContext _localctx = new FieldListContext(_ctx, getState());
enterRule(_localctx, 48, RULE_fieldList);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(307);
match(T__21);
setState(311);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << T__23) | (1L << T__24) | (1L << T__26) | (1L << T__27) | (1L << T__28) | (1L << T__29) | (1L << T__30) | (1L << T__31) | (1L << T__32) | (1L << T__33) | (1L << T__34) | (1L << T__35) | (1L << T__36) | (1L << T__39) | (1L << T__40) | (1L << IDENTIFIER) | (1L << INTEGER))) != 0)) {
{
{
setState(308);
field();
}
}
setState(313);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(314);
match(T__22);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class FieldContext extends ParserRuleContext {
public FieldTypeContext fieldType() {
return getRuleContext(FieldTypeContext.class,0);
}
public TerminalNode IDENTIFIER() { return getToken(AntlrThriftParser.IDENTIFIER, 0); }
public TerminalNode INTEGER() { return getToken(AntlrThriftParser.INTEGER, 0); }
public RequirednessContext requiredness() {
return getRuleContext(RequirednessContext.class,0);
}
public ConstValueContext constValue() {
return getRuleContext(ConstValueContext.class,0);
}
public AnnotationListContext annotationList() {
return getRuleContext(AnnotationListContext.class,0);
}
public SeparatorContext separator() {
return getRuleContext(SeparatorContext.class,0);
}
public FieldContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_field; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterField(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitField(this);
}
}
public final FieldContext field() throws RecognitionException {
FieldContext _localctx = new FieldContext(_ctx, getState());
enterRule(_localctx, 50, RULE_field);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(318);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==INTEGER) {
{
setState(316);
match(INTEGER);
setState(317);
match(T__12);
}
}
setState(321);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==T__23 || _la==T__24) {
{
setState(320);
requiredness();
}
}
setState(323);
fieldType();
setState(324);
match(IDENTIFIER);
setState(327);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==T__7) {
{
setState(325);
match(T__7);
setState(326);
constValue();
}
}
setState(330);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==T__21) {
{
setState(329);
annotationList();
}
}
setState(333);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COMMA || _la==SEMICOLON) {
{
setState(332);
separator();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class RequirednessContext extends ParserRuleContext {
public RequirednessContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_requiredness; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterRequiredness(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitRequiredness(this);
}
}
public final RequirednessContext requiredness() throws RecognitionException {
RequirednessContext _localctx = new RequirednessContext(_ctx, getState());
enterRule(_localctx, 52, RULE_requiredness);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(335);
_la = _input.LA(1);
if ( !(_la==T__23 || _la==T__24) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ThrowsListContext extends ParserRuleContext {
public FieldListContext fieldList() {
return getRuleContext(FieldListContext.class,0);
}
public ThrowsListContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_throwsList; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterThrowsList(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitThrowsList(this);
}
}
public final ThrowsListContext throwsList() throws RecognitionException {
ThrowsListContext _localctx = new ThrowsListContext(_ctx, getState());
enterRule(_localctx, 54, RULE_throwsList);
try {
enterOuterAlt(_localctx, 1);
{
setState(337);
match(T__25);
setState(338);
fieldList();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class FieldTypeContext extends ParserRuleContext {
public BaseTypeContext baseType() {
return getRuleContext(BaseTypeContext.class,0);
}
public AnnotationListContext annotationList() {
return getRuleContext(AnnotationListContext.class,0);
}
public ContainerTypeContext containerType() {
return getRuleContext(ContainerTypeContext.class,0);
}
public TerminalNode IDENTIFIER() { return getToken(AntlrThriftParser.IDENTIFIER, 0); }
public FieldTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_fieldType; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterFieldType(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitFieldType(this);
}
}
public final FieldTypeContext fieldType() throws RecognitionException {
FieldTypeContext _localctx = new FieldTypeContext(_ctx, getState());
enterRule(_localctx, 56, RULE_fieldType);
int _la;
try {
setState(352);
_errHandler.sync(this);
switch (_input.LA(1)) {
case T__26:
case T__27:
case T__28:
case T__29:
case T__30:
case T__31:
case T__32:
case T__33:
case T__34:
case T__35:
enterOuterAlt(_localctx, 1);
{
setState(340);
baseType();
setState(342);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==T__21) {
{
setState(341);
annotationList();
}
}
}
break;
case T__36:
case T__39:
case T__40:
enterOuterAlt(_localctx, 2);
{
setState(344);
containerType();
setState(346);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==T__21) {
{
setState(345);
annotationList();
}
}
}
break;
case IDENTIFIER:
enterOuterAlt(_localctx, 3);
{
setState(348);
match(IDENTIFIER);
setState(350);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==T__21) {
{
setState(349);
annotationList();
}
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class BaseTypeContext extends ParserRuleContext {
public BaseTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_baseType; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterBaseType(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitBaseType(this);
}
}
public final BaseTypeContext baseType() throws RecognitionException {
BaseTypeContext _localctx = new BaseTypeContext(_ctx, getState());
enterRule(_localctx, 58, RULE_baseType);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(354);
_la = _input.LA(1);
if ( !((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << T__26) | (1L << T__27) | (1L << T__28) | (1L << T__29) | (1L << T__30) | (1L << T__31) | (1L << T__32) | (1L << T__33) | (1L << T__34) | (1L << T__35))) != 0)) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ContainerTypeContext extends ParserRuleContext {
public MapTypeContext mapType() {
return getRuleContext(MapTypeContext.class,0);
}
public SetTypeContext setType() {
return getRuleContext(SetTypeContext.class,0);
}
public ListTypeContext listType() {
return getRuleContext(ListTypeContext.class,0);
}
public ContainerTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_containerType; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterContainerType(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitContainerType(this);
}
}
public final ContainerTypeContext containerType() throws RecognitionException {
ContainerTypeContext _localctx = new ContainerTypeContext(_ctx, getState());
enterRule(_localctx, 60, RULE_containerType);
try {
setState(359);
_errHandler.sync(this);
switch (_input.LA(1)) {
case T__36:
enterOuterAlt(_localctx, 1);
{
setState(356);
mapType();
}
break;
case T__40:
enterOuterAlt(_localctx, 2);
{
setState(357);
setType();
}
break;
case T__39:
enterOuterAlt(_localctx, 3);
{
setState(358);
listType();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class MapTypeContext extends ParserRuleContext {
public FieldTypeContext key;
public FieldTypeContext value;
public TerminalNode COMMA() { return getToken(AntlrThriftParser.COMMA, 0); }
public List fieldType() {
return getRuleContexts(FieldTypeContext.class);
}
public FieldTypeContext fieldType(int i) {
return getRuleContext(FieldTypeContext.class,i);
}
public CppTypeContext cppType() {
return getRuleContext(CppTypeContext.class,0);
}
public MapTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_mapType; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterMapType(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitMapType(this);
}
}
public final MapTypeContext mapType() throws RecognitionException {
MapTypeContext _localctx = new MapTypeContext(_ctx, getState());
enterRule(_localctx, 62, RULE_mapType);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(361);
match(T__36);
setState(363);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==T__41) {
{
setState(362);
cppType();
}
}
setState(365);
match(T__37);
setState(366);
((MapTypeContext)_localctx).key = fieldType();
setState(367);
match(COMMA);
setState(368);
((MapTypeContext)_localctx).value = fieldType();
setState(369);
match(T__38);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ListTypeContext extends ParserRuleContext {
public FieldTypeContext fieldType() {
return getRuleContext(FieldTypeContext.class,0);
}
public CppTypeContext cppType() {
return getRuleContext(CppTypeContext.class,0);
}
public ListTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_listType; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterListType(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitListType(this);
}
}
public final ListTypeContext listType() throws RecognitionException {
ListTypeContext _localctx = new ListTypeContext(_ctx, getState());
enterRule(_localctx, 64, RULE_listType);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(371);
match(T__39);
setState(372);
match(T__37);
setState(373);
fieldType();
setState(374);
match(T__38);
setState(376);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==T__41) {
{
setState(375);
cppType();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SetTypeContext extends ParserRuleContext {
public FieldTypeContext fieldType() {
return getRuleContext(FieldTypeContext.class,0);
}
public CppTypeContext cppType() {
return getRuleContext(CppTypeContext.class,0);
}
public SetTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_setType; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterSetType(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitSetType(this);
}
}
public final SetTypeContext setType() throws RecognitionException {
SetTypeContext _localctx = new SetTypeContext(_ctx, getState());
enterRule(_localctx, 66, RULE_setType);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(378);
match(T__40);
setState(380);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==T__41) {
{
setState(379);
cppType();
}
}
setState(382);
match(T__37);
setState(383);
fieldType();
setState(384);
match(T__38);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class CppTypeContext extends ParserRuleContext {
public TerminalNode LITERAL() { return getToken(AntlrThriftParser.LITERAL, 0); }
public CppTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_cppType; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterCppType(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitCppType(this);
}
}
public final CppTypeContext cppType() throws RecognitionException {
CppTypeContext _localctx = new CppTypeContext(_ctx, getState());
enterRule(_localctx, 68, RULE_cppType);
try {
enterOuterAlt(_localctx, 1);
{
setState(386);
match(T__41);
setState(387);
match(LITERAL);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class AnnotationListContext extends ParserRuleContext {
public List annotation() {
return getRuleContexts(AnnotationContext.class);
}
public AnnotationContext annotation(int i) {
return getRuleContext(AnnotationContext.class,i);
}
public AnnotationListContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_annotationList; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterAnnotationList(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitAnnotationList(this);
}
}
public final AnnotationListContext annotationList() throws RecognitionException {
AnnotationListContext _localctx = new AnnotationListContext(_ctx, getState());
enterRule(_localctx, 70, RULE_annotationList);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(389);
match(T__21);
setState(393);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==IDENTIFIER) {
{
{
setState(390);
annotation();
}
}
setState(395);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(396);
match(T__22);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class AnnotationContext extends ParserRuleContext {
public TerminalNode IDENTIFIER() { return getToken(AntlrThriftParser.IDENTIFIER, 0); }
public TerminalNode LITERAL() { return getToken(AntlrThriftParser.LITERAL, 0); }
public SeparatorContext separator() {
return getRuleContext(SeparatorContext.class,0);
}
public AnnotationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_annotation; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterAnnotation(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitAnnotation(this);
}
}
public final AnnotationContext annotation() throws RecognitionException {
AnnotationContext _localctx = new AnnotationContext(_ctx, getState());
enterRule(_localctx, 72, RULE_annotation);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(398);
match(IDENTIFIER);
setState(401);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==T__7) {
{
setState(399);
match(T__7);
setState(400);
match(LITERAL);
}
}
setState(404);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COMMA || _la==SEMICOLON) {
{
setState(403);
separator();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SeparatorContext extends ParserRuleContext {
public TerminalNode COMMA() { return getToken(AntlrThriftParser.COMMA, 0); }
public TerminalNode SEMICOLON() { return getToken(AntlrThriftParser.SEMICOLON, 0); }
public SeparatorContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_separator; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).enterSeparator(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof AntlrThriftListener ) ((AntlrThriftListener)listener).exitSeparator(this);
}
}
public final SeparatorContext separator() throws RecognitionException {
SeparatorContext _localctx = new SeparatorContext(_ctx, getState());
enterRule(_localctx, 74, RULE_separator);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(406);
_la = _input.LA(1);
if ( !(_la==COMMA || _la==SEMICOLON) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static final String _serializedATN =
"\3\u608b\ua72a\u8133\ub9ed\u417c\u3be7\u7786\u5964\3:\u019b\4\2\t\2\4"+
"\3\t\3\4\4\t\4\4\5\t\5\4\6\t\6\4\7\t\7\4\b\t\b\4\t\t\t\4\n\t\n\4\13\t"+
"\13\4\f\t\f\4\r\t\r\4\16\t\16\4\17\t\17\4\20\t\20\4\21\t\21\4\22\t\22"+
"\4\23\t\23\4\24\t\24\4\25\t\25\4\26\t\26\4\27\t\27\4\30\t\30\4\31\t\31"+
"\4\32\t\32\4\33\t\33\4\34\t\34\4\35\t\35\4\36\t\36\4\37\t\37\4 \t \4!"+
"\t!\4\"\t\"\4#\t#\4$\t$\4%\t%\4&\t&\4\'\t\'\3\2\7\2P\n\2\f\2\16\2S\13"+
"\2\3\2\7\2V\n\2\f\2\16\2Y\13\2\3\3\3\3\3\3\5\3^\n\3\3\4\3\4\3\4\5\4c\n"+
"\4\3\5\3\5\3\5\5\5h\n\5\3\6\3\6\3\6\5\6m\n\6\3\7\3\7\3\7\3\7\5\7s\n\7"+
"\3\7\5\7v\n\7\3\b\3\b\3\t\3\t\3\t\5\t}\n\t\3\t\5\t\u0080\n\t\3\n\3\n\3"+
"\n\5\n\u0085\n\n\3\n\5\n\u0088\n\n\3\13\3\13\3\13\3\13\3\13\3\13\3\13"+
"\3\13\5\13\u0092\n\13\3\f\3\f\3\f\3\f\3\f\3\f\5\f\u009a\n\f\3\r\3\r\3"+
"\r\3\r\3\r\3\r\5\r\u00a2\n\r\3\16\3\16\3\16\5\16\u00a7\n\16\7\16\u00a9"+
"\n\16\f\16\16\16\u00ac\13\16\3\16\3\16\3\17\3\17\3\17\5\17\u00b3\n\17"+
"\7\17\u00b5\n\17\f\17\16\17\u00b8\13\17\3\17\3\17\3\20\3\20\3\20\3\20"+
"\3\21\3\21\3\21\3\21\5\21\u00c4\n\21\3\21\5\21\u00c7\n\21\3\22\3\22\3"+
"\22\3\22\7\22\u00cd\n\22\f\22\16\22\u00d0\13\22\3\22\3\22\5\22\u00d4\n"+
"\22\3\23\3\23\3\23\5\23\u00d9\n\23\3\23\5\23\u00dc\n\23\3\23\5\23\u00df"+
"\n\23\3\24\3\24\3\24\3\24\7\24\u00e5\n\24\f\24\16\24\u00e8\13\24\3\24"+
"\3\24\3\25\3\25\3\25\3\25\7\25\u00f0\n\25\f\25\16\25\u00f3\13\25\3\25"+
"\3\25\5\25\u00f7\n\25\3\26\3\26\3\26\3\26\7\26\u00fd\n\26\f\26\16\26\u0100"+
"\13\26\3\26\3\26\5\26\u0104\n\26\3\27\3\27\3\27\3\27\7\27\u010a\n\27\f"+
"\27\16\27\u010d\13\27\3\27\3\27\5\27\u0111\n\27\3\30\3\30\3\30\3\30\5"+
"\30\u0117\n\30\3\30\3\30\7\30\u011b\n\30\f\30\16\30\u011e\13\30\3\30\3"+
"\30\5\30\u0122\n\30\3\31\5\31\u0125\n\31\3\31\3\31\5\31\u0129\n\31\3\31"+
"\3\31\3\31\5\31\u012e\n\31\3\31\5\31\u0131\n\31\3\31\5\31\u0134\n\31\3"+
"\32\3\32\7\32\u0138\n\32\f\32\16\32\u013b\13\32\3\32\3\32\3\33\3\33\5"+
"\33\u0141\n\33\3\33\5\33\u0144\n\33\3\33\3\33\3\33\3\33\5\33\u014a\n\33"+
"\3\33\5\33\u014d\n\33\3\33\5\33\u0150\n\33\3\34\3\34\3\35\3\35\3\35\3"+
"\36\3\36\5\36\u0159\n\36\3\36\3\36\5\36\u015d\n\36\3\36\3\36\5\36\u0161"+
"\n\36\5\36\u0163\n\36\3\37\3\37\3 \3 \3 \5 \u016a\n \3!\3!\5!\u016e\n"+
"!\3!\3!\3!\3!\3!\3!\3\"\3\"\3\"\3\"\3\"\5\"\u017b\n\"\3#\3#\5#\u017f\n"+
"#\3#\3#\3#\3#\3$\3$\3$\3%\3%\7%\u018a\n%\f%\16%\u018d\13%\3%\3%\3&\3&"+
"\3&\5&\u0194\n&\3&\5&\u0197\n&\3\'\3\'\3\'\2\2(\2\4\6\b\n\f\16\20\22\24"+
"\26\30\32\34\36 \"$&(*,.\60\62\64\668:<>@BDFHJL\2\6\4\2\6\6\62\62\3\2"+
"\32\33\3\2\35&\3\2\60\61\2\u01bc\2Q\3\2\2\2\4]\3\2\2\2\6_\3\2\2\2\bd\3"+
"\2\2\2\nl\3\2\2\2\fn\3\2\2\2\16w\3\2\2\2\20y\3\2\2\2\22\u0081\3\2\2\2"+
"\24\u0091\3\2\2\2\26\u0093\3\2\2\2\30\u00a1\3\2\2\2\32\u00a3\3\2\2\2\34"+
"\u00af\3\2\2\2\36\u00bb\3\2\2\2 \u00bf\3\2\2\2\"\u00c8\3\2\2\2$\u00d5"+
"\3\2\2\2&\u00e0\3\2\2\2(\u00eb\3\2\2\2*\u00f8\3\2\2\2,\u0105\3\2\2\2."+
"\u0112\3\2\2\2\60\u0124\3\2\2\2\62\u0135\3\2\2\2\64\u0140\3\2\2\2\66\u0151"+
"\3\2\2\28\u0153\3\2\2\2:\u0162\3\2\2\2<\u0164\3\2\2\2>\u0169\3\2\2\2@"+
"\u016b\3\2\2\2B\u0175\3\2\2\2D\u017c\3\2\2\2F\u0184\3\2\2\2H\u0187\3\2"+
"\2\2J\u0190\3\2\2\2L\u0198\3\2\2\2NP\5\4\3\2ON\3\2\2\2PS\3\2\2\2QO\3\2"+
"\2\2QR\3\2\2\2RW\3\2\2\2SQ\3\2\2\2TV\5\24\13\2UT\3\2\2\2VY\3\2\2\2WU\3"+
"\2\2\2WX\3\2\2\2X\3\3\2\2\2YW\3\2\2\2Z^\5\6\4\2[^\5\b\5\2\\^\5\n\6\2]"+
"Z\3\2\2\2][\3\2\2\2]\\\3\2\2\2^\5\3\2\2\2_`\7\3\2\2`b\7-\2\2ac\5L\'\2"+
"ba\3\2\2\2bc\3\2\2\2c\7\3\2\2\2de\7\4\2\2eg\7-\2\2fh\5L\'\2gf\3\2\2\2"+
"gh\3\2\2\2h\t\3\2\2\2im\5\f\7\2jm\5\20\t\2km\5\22\n\2li\3\2\2\2lj\3\2"+
"\2\2lk\3\2\2\2m\13\3\2\2\2no\7\5\2\2op\5\16\b\2pr\7\62\2\2qs\5H%\2rq\3"+
"\2\2\2rs\3\2\2\2su\3\2\2\2tv\5L\'\2ut\3\2\2\2uv\3\2\2\2v\r\3\2\2\2wx\t"+
"\2\2\2x\17\3\2\2\2yz\7\7\2\2z|\7-\2\2{}\5H%\2|{\3\2\2\2|}\3\2\2\2}\177"+
"\3\2\2\2~\u0080\5L\'\2\177~\3\2\2\2\177\u0080\3\2\2\2\u0080\21\3\2\2\2"+
"\u0081\u0082\7\b\2\2\u0082\u0084\7-\2\2\u0083\u0085\5H%\2\u0084\u0083"+
"\3\2\2\2\u0084\u0085\3\2\2\2\u0085\u0087\3\2\2\2\u0086\u0088\5L\'\2\u0087"+
"\u0086\3\2\2\2\u0087\u0088\3\2\2\2\u0088\23\3\2\2\2\u0089\u0092\5\26\f"+
"\2\u008a\u0092\5 \21\2\u008b\u0092\5\"\22\2\u008c\u0092\5&\24\2\u008d"+
"\u0092\5(\25\2\u008e\u0092\5*\26\2\u008f\u0092\5,\27\2\u0090\u0092\5."+
"\30\2\u0091\u0089\3\2\2\2\u0091\u008a\3\2\2\2\u0091\u008b\3\2\2\2\u0091"+
"\u008c\3\2\2\2\u0091\u008d\3\2\2\2\u0091\u008e\3\2\2\2\u0091\u008f\3\2"+
"\2\2\u0091\u0090\3\2\2\2\u0092\25\3\2\2\2\u0093\u0094\7\t\2\2\u0094\u0095"+
"\5:\36\2\u0095\u0096\7\62\2\2\u0096\u0097\7\n\2\2\u0097\u0099\5\30\r\2"+
"\u0098\u009a\5L\'\2\u0099\u0098\3\2\2\2\u0099\u009a\3\2\2\2\u009a\27\3"+
"\2\2\2\u009b\u00a2\7\64\2\2\u009c\u00a2\7\65\2\2\u009d\u00a2\7-\2\2\u009e"+
"\u00a2\7\62\2\2\u009f\u00a2\5\32\16\2\u00a0\u00a2\5\34\17\2\u00a1\u009b"+
"\3\2\2\2\u00a1\u009c\3\2\2\2\u00a1\u009d\3\2\2\2\u00a1\u009e\3\2\2\2\u00a1"+
"\u009f\3\2\2\2\u00a1\u00a0\3\2\2\2\u00a2\31\3\2\2\2\u00a3\u00aa\7\13\2"+
"\2\u00a4\u00a6\5\30\r\2\u00a5\u00a7\5L\'\2\u00a6\u00a5\3\2\2\2\u00a6\u00a7"+
"\3\2\2\2\u00a7\u00a9\3\2\2\2\u00a8\u00a4\3\2\2\2\u00a9\u00ac\3\2\2\2\u00aa"+
"\u00a8\3\2\2\2\u00aa\u00ab\3\2\2\2\u00ab\u00ad\3\2\2\2\u00ac\u00aa\3\2"+
"\2\2\u00ad\u00ae\7\f\2\2\u00ae\33\3\2\2\2\u00af\u00b6\7\r\2\2\u00b0\u00b2"+
"\5\36\20\2\u00b1\u00b3\5L\'\2\u00b2\u00b1\3\2\2\2\u00b2\u00b3\3\2\2\2"+
"\u00b3\u00b5\3\2\2\2\u00b4\u00b0\3\2\2\2\u00b5\u00b8\3\2\2\2\u00b6\u00b4"+
"\3\2\2\2\u00b6\u00b7\3\2\2\2\u00b7\u00b9\3\2\2\2\u00b8\u00b6\3\2\2\2\u00b9"+
"\u00ba\7\16\2\2\u00ba\35\3\2\2\2\u00bb\u00bc\5\30\r\2\u00bc\u00bd\7\17"+
"\2\2\u00bd\u00be\5\30\r\2\u00be\37\3\2\2\2\u00bf\u00c0\7\20\2\2\u00c0"+
"\u00c1\5:\36\2\u00c1\u00c3\7\62\2\2\u00c2\u00c4\5H%\2\u00c3\u00c2\3\2"+
"\2\2\u00c3\u00c4\3\2\2\2\u00c4\u00c6\3\2\2\2\u00c5\u00c7\5L\'\2\u00c6"+
"\u00c5\3\2\2\2\u00c6\u00c7\3\2\2\2\u00c7!\3\2\2\2\u00c8\u00c9\7\21\2\2"+
"\u00c9\u00ca\7\62\2\2\u00ca\u00ce\7\r\2\2\u00cb\u00cd\5$\23\2\u00cc\u00cb"+
"\3\2\2\2\u00cd\u00d0\3\2\2\2\u00ce\u00cc\3\2\2\2\u00ce\u00cf\3\2\2\2\u00cf"+
"\u00d1\3\2\2\2\u00d0\u00ce\3\2\2\2\u00d1\u00d3\7\16\2\2\u00d2\u00d4\5"+
"H%\2\u00d3\u00d2\3\2\2\2\u00d3\u00d4\3\2\2\2\u00d4#\3\2\2\2\u00d5\u00d8"+
"\7\62\2\2\u00d6\u00d7\7\n\2\2\u00d7\u00d9\7\64\2\2\u00d8\u00d6\3\2\2\2"+
"\u00d8\u00d9\3\2\2\2\u00d9\u00db\3\2\2\2\u00da\u00dc\5H%\2\u00db\u00da"+
"\3\2\2\2\u00db\u00dc\3\2\2\2\u00dc\u00de\3\2\2\2\u00dd\u00df\5L\'\2\u00de"+
"\u00dd\3\2\2\2\u00de\u00df\3\2\2\2\u00df%\3\2\2\2\u00e0\u00e1\7\22\2\2"+
"\u00e1\u00e2\7\62\2\2\u00e2\u00e6\7\r\2\2\u00e3\u00e5\5$\23\2\u00e4\u00e3"+
"\3\2\2\2\u00e5\u00e8\3\2\2\2\u00e6\u00e4\3\2\2\2\u00e6\u00e7\3\2\2\2\u00e7"+
"\u00e9\3\2\2\2\u00e8\u00e6\3\2\2\2\u00e9\u00ea\7\16\2\2\u00ea\'\3\2\2"+
"\2\u00eb\u00ec\7\23\2\2\u00ec\u00ed\7\62\2\2\u00ed\u00f1\7\r\2\2\u00ee"+
"\u00f0\5\64\33\2\u00ef\u00ee\3\2\2\2\u00f0\u00f3\3\2\2\2\u00f1\u00ef\3"+
"\2\2\2\u00f1\u00f2\3\2\2\2\u00f2\u00f4\3\2\2\2\u00f3\u00f1\3\2\2\2\u00f4"+
"\u00f6\7\16\2\2\u00f5\u00f7\5H%\2\u00f6\u00f5\3\2\2\2\u00f6\u00f7\3\2"+
"\2\2\u00f7)\3\2\2\2\u00f8\u00f9\7\24\2\2\u00f9\u00fa\7\62\2\2\u00fa\u00fe"+
"\7\r\2\2\u00fb\u00fd\5\64\33\2\u00fc\u00fb\3\2\2\2\u00fd\u0100\3\2\2\2"+
"\u00fe\u00fc\3\2\2\2\u00fe\u00ff\3\2\2\2\u00ff\u0101\3\2\2\2\u0100\u00fe"+
"\3\2\2\2\u0101\u0103\7\16\2\2\u0102\u0104\5H%\2\u0103\u0102\3\2\2\2\u0103"+
"\u0104\3\2\2\2\u0104+\3\2\2\2\u0105\u0106\7\25\2\2\u0106\u0107\7\62\2"+
"\2\u0107\u010b\7\r\2\2\u0108\u010a\5\64\33\2\u0109\u0108\3\2\2\2\u010a"+
"\u010d\3\2\2\2\u010b\u0109\3\2\2\2\u010b\u010c\3\2\2\2\u010c\u010e\3\2"+
"\2\2\u010d\u010b\3\2\2\2\u010e\u0110\7\16\2\2\u010f\u0111\5H%\2\u0110"+
"\u010f\3\2\2\2\u0110\u0111\3\2\2\2\u0111-\3\2\2\2\u0112\u0113\7\26\2\2"+
"\u0113\u0116\7\62\2\2\u0114\u0115\7\27\2\2\u0115\u0117\5:\36\2\u0116\u0114"+
"\3\2\2\2\u0116\u0117\3\2\2\2\u0117\u0118\3\2\2\2\u0118\u011c\7\r\2\2\u0119"+
"\u011b\5\60\31\2\u011a\u0119\3\2\2\2\u011b\u011e\3\2\2\2\u011c\u011a\3"+
"\2\2\2\u011c\u011d\3\2\2\2\u011d\u011f\3\2\2\2\u011e\u011c\3\2\2\2\u011f"+
"\u0121\7\16\2\2\u0120\u0122\5H%\2\u0121\u0120\3\2\2\2\u0121\u0122\3\2"+
"\2\2\u0122/\3\2\2\2\u0123\u0125\7/\2\2\u0124\u0123\3\2\2\2\u0124\u0125"+
"\3\2\2\2\u0125\u0128\3\2\2\2\u0126\u0129\7.\2\2\u0127\u0129\5:\36\2\u0128"+
"\u0126\3\2\2\2\u0128\u0127\3\2\2\2\u0129\u012a\3\2\2\2\u012a\u012b\7\62"+
"\2\2\u012b\u012d\5\62\32\2\u012c\u012e\58\35\2\u012d\u012c\3\2\2\2\u012d"+
"\u012e\3\2\2\2\u012e\u0130\3\2\2\2\u012f\u0131\5H%\2\u0130\u012f\3\2\2"+
"\2\u0130\u0131\3\2\2\2\u0131\u0133\3\2\2\2\u0132\u0134\5L\'\2\u0133\u0132"+
"\3\2\2\2\u0133\u0134\3\2\2\2\u0134\61\3\2\2\2\u0135\u0139\7\30\2\2\u0136"+
"\u0138\5\64\33\2\u0137\u0136\3\2\2\2\u0138\u013b\3\2\2\2\u0139\u0137\3"+
"\2\2\2\u0139\u013a\3\2\2\2\u013a\u013c\3\2\2\2\u013b\u0139\3\2\2\2\u013c"+
"\u013d\7\31\2\2\u013d\63\3\2\2\2\u013e\u013f\7\64\2\2\u013f\u0141\7\17"+
"\2\2\u0140\u013e\3\2\2\2\u0140\u0141\3\2\2\2\u0141\u0143\3\2\2\2\u0142"+
"\u0144\5\66\34\2\u0143\u0142\3\2\2\2\u0143\u0144\3\2\2\2\u0144\u0145\3"+
"\2\2\2\u0145\u0146\5:\36\2\u0146\u0149\7\62\2\2\u0147\u0148\7\n\2\2\u0148"+
"\u014a\5\30\r\2\u0149\u0147\3\2\2\2\u0149\u014a\3\2\2\2\u014a\u014c\3"+
"\2\2\2\u014b\u014d\5H%\2\u014c\u014b\3\2\2\2\u014c\u014d\3\2\2\2\u014d"+
"\u014f\3\2\2\2\u014e\u0150\5L\'\2\u014f\u014e\3\2\2\2\u014f\u0150\3\2"+
"\2\2\u0150\65\3\2\2\2\u0151\u0152\t\3\2\2\u0152\67\3\2\2\2\u0153\u0154"+
"\7\34\2\2\u0154\u0155\5\62\32\2\u01559\3\2\2\2\u0156\u0158\5<\37\2\u0157"+
"\u0159\5H%\2\u0158\u0157\3\2\2\2\u0158\u0159\3\2\2\2\u0159\u0163\3\2\2"+
"\2\u015a\u015c\5> \2\u015b\u015d\5H%\2\u015c\u015b\3\2\2\2\u015c\u015d"+
"\3\2\2\2\u015d\u0163\3\2\2\2\u015e\u0160\7\62\2\2\u015f\u0161\5H%\2\u0160"+
"\u015f\3\2\2\2\u0160\u0161\3\2\2\2\u0161\u0163\3\2\2\2\u0162\u0156\3\2"+
"\2\2\u0162\u015a\3\2\2\2\u0162\u015e\3\2\2\2\u0163;\3\2\2\2\u0164\u0165"+
"\t\4\2\2\u0165=\3\2\2\2\u0166\u016a\5@!\2\u0167\u016a\5D#\2\u0168\u016a"+
"\5B\"\2\u0169\u0166\3\2\2\2\u0169\u0167\3\2\2\2\u0169\u0168\3\2\2\2\u016a"+
"?\3\2\2\2\u016b\u016d\7\'\2\2\u016c\u016e\5F$\2\u016d\u016c\3\2\2\2\u016d"+
"\u016e\3\2\2\2\u016e\u016f\3\2\2\2\u016f\u0170\7(\2\2\u0170\u0171\5:\36"+
"\2\u0171\u0172\7\60\2\2\u0172\u0173\5:\36\2\u0173\u0174\7)\2\2\u0174A"+
"\3\2\2\2\u0175\u0176\7*\2\2\u0176\u0177\7(\2\2\u0177\u0178\5:\36\2\u0178"+
"\u017a\7)\2\2\u0179\u017b\5F$\2\u017a\u0179\3\2\2\2\u017a\u017b\3\2\2"+
"\2\u017bC\3\2\2\2\u017c\u017e\7+\2\2\u017d\u017f\5F$\2\u017e\u017d\3\2"+
"\2\2\u017e\u017f\3\2\2\2\u017f\u0180\3\2\2\2\u0180\u0181\7(\2\2\u0181"+
"\u0182\5:\36\2\u0182\u0183\7)\2\2\u0183E\3\2\2\2\u0184\u0185\7,\2\2\u0185"+
"\u0186\7-\2\2\u0186G\3\2\2\2\u0187\u018b\7\30\2\2\u0188\u018a\5J&\2\u0189"+
"\u0188\3\2\2\2\u018a\u018d\3\2\2\2\u018b\u0189\3\2\2\2\u018b\u018c\3\2"+
"\2\2\u018c\u018e\3\2\2\2\u018d\u018b\3\2\2\2\u018e\u018f\7\31\2\2\u018f"+
"I\3\2\2\2\u0190\u0193\7\62\2\2\u0191\u0192\7\n\2\2\u0192\u0194\7-\2\2"+
"\u0193\u0191\3\2\2\2\u0193\u0194\3\2\2\2\u0194\u0196\3\2\2\2\u0195\u0197"+
"\5L\'\2\u0196\u0195\3\2\2\2\u0196\u0197\3\2\2\2\u0197K\3\2\2\2\u0198\u0199"+
"\t\5\2\2\u0199M\3\2\2\2
© 2015 - 2025 Weber Informatics LLC | Privacy Policy