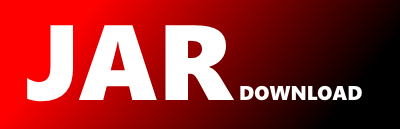
com.microsoft.windowsazure.storage.StorageCredentialsSharedAccessSignature Maven / Gradle / Ivy
/**
* Copyright Microsoft Corporation
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.microsoft.windowsazure.storage;
import java.net.URI;
import java.net.URISyntaxException;
import com.microsoft.windowsazure.storage.core.PathUtility;
/**
* Represents storage credentials for delegated access to Blob service resources via a shared access signature.
*/
public final class StorageCredentialsSharedAccessSignature extends StorageCredentials {
/**
* Stores the shared access signature token.
*/
private final String token;
/**
* Creates an instance of the StorageCredentialsSharedAccessSignature
class using the specified shared
* access signature token.
*
* @param token
* A String
that represents shared access signature token.
*/
public StorageCredentialsSharedAccessSignature(final String token) {
this.token = token;
}
//
// RESERVED, for internal use only. Gets a value indicating whether the
// ComputeHmac
method will return a valid HMAC-encoded
// signature string when called using the specified credentials.
//
// @return False
//
/** Reserved. */
@Override
public boolean canCredentialsComputeHmac() {
return false;
}
//
// RESERVED, for internal use only. Gets a value indicating whether a
// request can be signed under the Shared Key authentication scheme using
// the specified credentials.
//
// @return False
//
/** Reserved. */
@Override
public boolean canCredentialsSignRequest() {
return false;
}
//
// RESERVED, for internal use only. Gets a value indicating whether a
// request can be signed under the Shared Key Lite authentication scheme
// using the specified credentials.
//
// @return False
//
/** Reserved. */
@Override
public boolean canCredentialsSignRequestLite() {
return false;
}
/**
* Computes a signature for the specified string using the HMAC-SHA256 algorithm. This is not a valid operation for
* objects of type StorageCredentialsSharedAccessSignature
so the method merely returns
* null
.
*
* @param value
* The UTF-8-encoded string to sign.
*
* @return null
for objects of type StorageCredentialsSharedAccessSignature
.
*/
@Override
public String computeHmac256(final String value) {
return null;
}
/**
* Computes a signature for the specified string using the HMAC-SHA256 algorithm with the specified operation
* context. This is not a valid operation for objects of type StorageCredentialsSharedAccessSignature
* so the method merely returns null
.
*
* @param value
* The UTF-8-encoded string to sign.
* @param opContext
* An {@link OperationContext} object that represents the context for the current operation. This object
* is used to track requests to the storage service, and to provide additional runtime information about
* the operation.
*
* @return null
for objects of type StorageCredentialsSharedAccessSignature
.
*/
@Override
public String computeHmac256(final String value, final OperationContext opContext) {
return null;
}
/**
* Computes a signature for the specified string using the HMAC-SHA512 algorithm. This is not a valid operation for
* objects of type StorageCredentialsSharedAccessSignature
so the method merely returns
* null
.
*
* @param value
* The UTF-8-encoded string to sign.
*
* @return null
for objects of type StorageCredentialsSharedAccessSignature
.
*/
@Override
public String computeHmac512(final String value) {
return null;
}
/**
* Computes a signature for the specified string using the HMAC-SHA512 algorithm with the specified operation
* context. This is not a valid operation for objects of type StorageCredentialsSharedAccessSignature
* so the method merely returns null
.
*
* @param value
* The UTF-8-encoded string to sign.
*
* @param opContext
* An {@link OperationContext} object that represents the context for the current operation. This object
* is used to track requests to the storage service, and to provide additional runtime information about
* the operation.
*
* @return null
for objects of type StorageCredentialsSharedAccessSignature
.
*/
@Override
public String computeHmac512(final String value, final OperationContext opContext) {
return null;
}
//
// RESERVED, for internal use only. Gets a value indicating whether the
// TransformUri
method should be called to transform a resource
// URI to a URI that includes a token for a shared access signature.
//
// @return True
.
//
/** Reserved. */
@Override
public boolean doCredentialsNeedTransformUri() {
return true;
}
/**
* Returns the associated account name for the credentials. This is not a valid operation for objects of type
* StorageCredentialsSharedAccessSignature
so the method merely returns null
.
*
* @return null
for objects of type StorageCredentialsSharedAccessSignature
.
*/
@Override
public String getAccountName() {
return null;
}
/**
* Returns the shared access signature token.
*
* @return A String
that contains the token.
*/
public String getToken() {
return this.token;
}
/**
* Signs a request using the specified credentials under the Shared Key authentication scheme. This is not a valid
* operation for objects of type StorageCredentialsAnonymous
so the method performs a no-op.
*
* @deprecated This method has been deprecated. Please use either {@link signBlobAndQueueRequest} or
* {@link signBlobAndQueueRequestLite}, depending on your desired shared key authentication scheme.
*
* @param connection
* the request, as an HttpURLConnection
object, to sign
* @param contentLength
* the length of the content written to the output stream. If unknown, specify -1.
*/
@Override
@Deprecated
public void signRequest(final java.net.HttpURLConnection connection, final long contentLength) {
// No op
}
/**
* Signs a request using the specified credentials and operation context under the Shared Key authentication scheme.
* This is not a valid operation for objects of type StorageCredentialsAnonymous
so the method performs
* a no-op.
*
* @deprecated This method has been deprecated. Please use either {@link signBlobAndQueueRequest} or
* {@link signBlobAndQueueRequestLite}, depending on your desired shared key authentication scheme.
*
* @param request
* the request, as an HttpURLConnection
object, to sign
* @param contentLength
* the length of the content written to the output stream. If unknown, specify -1.
* @param opContext
* an operation context, as a {@link com.microsoft.windowsazure.storage.OperationContext} object,
* that represents the current operation
*/
@Override
@Deprecated
public void signRequest(final java.net.HttpURLConnection request, final long contentLength,
final OperationContext opContext) {
// No op
}
/**
* Signs a request using the specified credentials under the Shared Key Lite authentication scheme. This is not a
* valid operation for objects of type StorageCredentialsAnonymous
so the method performs a no-op.
*
* @deprecated This method has been deprecated. Please use either {@link signTableRequest} or
* {@link signTableRequestLite}, depending on your desired shared key authentication scheme.
*
* @param connection
* the request, as an HttpURLConnection
object, to sign
*/
@Override
@Deprecated
public void signRequestLite(final java.net.HttpURLConnection connection, final long contentLength) {
// No op
}
/**
* Signs a request using the specified credentials under the Shared Key Lite authentication scheme. This is not a
* valid operation for objects of type StorageCredentialsSharedAccessSignature
so the method performs a
* no-op.
*
* @deprecated This method has been deprecated. Please use either {@link signTableRequest} or
* {@link signTableRequestLite}, depending on your desired shared key authentication scheme.
*
* @param request
* the request, as an HttpURLConnection
object, to sign
* @param opContext
* an operation context, as a {@link com.microsoft.windowsazure.storage.OperationContext} object,
* that represents the current operation
*/
@Override
@Deprecated
public void signRequestLite(final java.net.HttpURLConnection request, final long contentLength,
final OperationContext opContext) {
// No op
}
/**
* Signs a request under the Shared Key authentication scheme. This is not a valid operation for objects of type
* StorageCredentialsSharedAccessSignature
so the method performs a no-op.
*
* @param request
* An HttpURLConnection
object that represents the request to sign.
* @param contentLength
* The length of the content written to the output stream. If unknown, specify -1.
*/
@Override
public void signBlobAndQueueRequest(final java.net.HttpURLConnection request, final long contentLength) {
// No op
}
/**
* Signs a request using the specified operation context under the Shared Key authentication scheme. This is not a
* valid operation for objects of type StorageCredentialsSharedAccessSignature
so the method performs a
* no-op.
*
* @param request
* An HttpURLConnection
object that represents the request to sign.
* @param contentLength
* The length of the content written to the output stream. If unknown, specify -1.
* @param opContext
* An {@link OperationContext} object that represents the context for the current operation. This object
* is used to track requests to the storage service, and to provide additional runtime information about
* the operation.
*/
@Override
public void signBlobAndQueueRequest(final java.net.HttpURLConnection request, final long contentLength,
final OperationContext opContext) {
// No op
}
/**
* Signs a request under the Shared Key Lite authentication scheme. This is not a valid operation for objects of
* type StorageCredentialsSharedAccessSignature
so the method performs a no-op.
*
* @param request
* An HttpURLConnection
object that represents the request to sign.
* @param contentLength
* The length of the content written to the output stream. If unknown, specify -1.
*/
@Override
public void signBlobAndQueueRequestLite(final java.net.HttpURLConnection request, final long contentLength) {
// No op
}
/**
* Signs a request using the specified operation context under the Shared Key Lite authentication scheme. This is
* not a valid operation for objects of type StorageCredentialsSharedAccessSignature
so the method
* performs a no-op.
*
* @param request
* An HttpURLConnection
object that represents the request to sign.
* @param contentLength
* The length of the content written to the output stream. If unknown, specify -1.
* @param opContext
* An {@link OperationContext} object that represents the context for the current operation. This object
* is used to track requests to the storage service, and to provide additional runtime information about
* the operation.
*/
@Override
public void signBlobAndQueueRequestLite(final java.net.HttpURLConnection request, final long contentLength,
final OperationContext opContext) {
// No op
}
/**
* Signs a request under the Shared Key authentication scheme. This is not a valid operation for objects of type
* StorageCredentialsSharedAccessSignature
so the method performs a no-op.
*
* @param request
* An HttpURLConnection
object that represents the request to sign.
* @param contentLength
* The length of the content written to the output stream. If unknown, specify -1.
*/
@Override
public void signTableRequest(final java.net.HttpURLConnection request, final long contentLength) {
// No op
}
/**
* Signs a request using the specified operation context under the Shared Key authentication scheme. This is not a
* valid operation for objects of type StorageCredentialsSharedAccessSignature
so the method performs a
* no-op.
*
* @param request
* An HttpURLConnection
object that represents the request to sign.
* @param contentLength
* The length of the content written to the output stream. If unknown, specify -1.
* @param opContext
* An {@link OperationContext} object that represents the context for the current operation. This object
* is used to track requests to the storage service, and to provide additional runtime information about
* the operation.
*/
@Override
public void signTableRequest(final java.net.HttpURLConnection request, final long contentLength,
final OperationContext opContext) {
// No op
}
/**
* Signs a request under the Shared Key Lite authentication scheme. This is not a valid operation for objects of
* type StorageCredentialsSharedAccessSignature
so the method performs a no-op.
*
* @param request
* An HttpURLConnection
object that represents the request to sign.
* @param contentLength
* The length of the content written to the output stream. If unknown, specify -1.
*/
@Override
public void signTableRequestLite(final java.net.HttpURLConnection request, final long contentLength) {
// No op
}
/**
* Signs a request using the specified operation context under the Shared Key Lite authentication scheme. This is
* not a valid operation for objects of type StorageCredentialsSharedAccessSignature
so the method
* performs a no-op.
*
* @param request
* An HttpURLConnection
object that represents the request to sign.
* @param contentLength
* The length of the content written to the output stream. If unknown, specify -1.
* @param opContext
* An {@link OperationContext} object that represents the context for the current operation. This object
* is used to track requests to the storage service, and to provide additional runtime information about
* the operation.
*/
@Override
public void signTableRequestLite(final java.net.HttpURLConnection request, final long contentLength,
final OperationContext opContext) {
// No op
}
/**
* Returns a String
that represents this instance, optionally including sensitive data.
*
* @param exportSecrets
* true
to include sensitive data in the return string; otherwise, false
.
* @return A String
that represents this object, optionally including sensitive data.
*/
@Override
public String toString(final boolean exportSecrets) {
return String.format("%s=%s", CloudStorageAccount.SHARED_ACCESS_SIGNATURE_NAME, exportSecrets ? this.token
: "[signature hidden]");
}
/**
* Transforms a resource URI into a shared access signature URI, by appending a shared access token.
*
* @param resourceUri
* A java.net.URI
object that represents the resource URI to be transformed.
*
* @return A java.net.URI
object that represents the signature, including the resource URI and the
* shared access token.
*
* @throws IllegalArgumentException
* If a parameter is invalid.
* @throws StorageException
* If a storage service error occurred.
* @throws URISyntaxException
* If the resource URI is not properly formatted.
*/
@Override
public URI transformUri(final URI resourceUri) throws URISyntaxException, StorageException {
return this.transformUri(resourceUri, null);
}
/**
* Transforms a resource URI into a shared access signature URI, by appending a shared access token and using the
* specified operation context.
*
* @param resourceUri
* A java.net.URI
object that represents the resource URI to be transformed.
* @param opContext
* An {@link OperationContext} object that represents the context for the current operation. This object
* is used to track requests to the storage service, and to provide additional runtime information about
* the operation.
*
* @return A java.net.URI
object that represents the signature, including the resource URI and the
* shared access token.
*
* @throws StorageException
* If a storage service error occurred.
* @throws URISyntaxException
* If the resource URI is not properly formatted.
*/
@Override
public URI transformUri(final URI resourceUri, final OperationContext opContext) throws URISyntaxException,
StorageException {
if (resourceUri == null) {
return null;
}
return PathUtility.addToQuery(resourceUri, this.token);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy