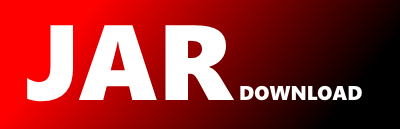
com.microsoft.windowsazure.services.servicebus.models.SubscriptionInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of microsoft-azure-api-servicebus Show documentation
Show all versions of microsoft-azure-api-servicebus Show documentation
Microsoft Azure Service Bus SDK Clients
The newest version!
/**
* Copyright Microsoft Corporation
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.microsoft.windowsazure.services.servicebus.models;
import java.util.Calendar;
import javax.ws.rs.core.MediaType;
import javax.xml.datatype.Duration;
import com.microsoft.windowsazure.services.servicebus.implementation.Content;
import com.microsoft.windowsazure.services.servicebus.implementation.EntityAvailabilityStatus;
import com.microsoft.windowsazure.services.servicebus.implementation.EntityStatus;
import com.microsoft.windowsazure.services.servicebus.implementation.Entry;
import com.microsoft.windowsazure.services.servicebus.implementation.EntryModel;
import com.microsoft.windowsazure.services.servicebus.implementation.MessageCountDetails;
import com.microsoft.windowsazure.services.servicebus.implementation.RuleDescription;
import com.microsoft.windowsazure.services.servicebus.implementation.SubscriptionDescription;
/**
* Represents a subscription.
*/
public class SubscriptionInfo extends EntryModel {
/**
* Creates an instance of the SubscriptionInfo
class.
*/
public SubscriptionInfo() {
super(new Entry(), new SubscriptionDescription());
getEntry().setContent(new Content());
getEntry().getContent().setType(MediaType.APPLICATION_XML);
getEntry().getContent().setSubscriptionDescription(getModel());
}
/**
* Creates an instance of the SubscriptionInfo
class using the
* specified entry.
*
* @param entry
* An Entry
object.
*/
public SubscriptionInfo(Entry entry) {
super(entry, entry.getContent().getSubscriptionDescription());
}
/**
* Creates an instance of the SubscriptionInfo
class using the
* specified name.
*
* @param name
* A String
object that represents the name of the
* subscription.
*/
public SubscriptionInfo(String name) {
this();
setName(name);
}
/**
* Returns the name of the subscription.
*
* @return A String
object that represents the name of the
* subscription.
*/
public String getName() {
return getEntry().getTitle();
}
/**
* Sets the name of the subscription.
*
* @param value
* A String
that represents the name of the
* subscription.
*
* @return A SubscriptionInfo
object that represents the
* updated subscription.
*/
public SubscriptionInfo setName(String value) {
getEntry().setTitle(value);
return this;
}
/**
* Returns the duration of the lock.
*
* @return A Duration
object that represents the duration of
* the lock.
*/
public Duration getLockDuration() {
return getModel().getLockDuration();
}
/**
* Sets the duration of the lock.
*
* @param value
* The duration, in seconds, of the lock.
*
* @return A SubscriptionInfo
object that represents the
* updated subscription.
*/
public SubscriptionInfo setLockDuration(Duration value) {
getModel().setLockDuration(value);
return this;
}
/**
* Indicates whether the subscription is session-aware.
*
* @return true
if the subscription is session aware;
* otherwise, false
.
*/
public Boolean isRequiresSession() {
return getModel().isRequiresSession();
}
/**
* Specifies whether the subscription is session-aware.
*
* @param value
* true
if the subscription is session aware;
* otherwise, false
.
*
* @return A SubscriptionInfo
object that represents the
* updated subscription.
*/
public SubscriptionInfo setRequiresSession(Boolean value) {
getModel().setRequiresSession(value);
return this;
}
/**
* Returns the default message time-to-live (TTL). This applies when dead
* lettering is in effect.
*
* @return A Duration
object that represents the default
* message TTL.
*/
public Duration getDefaultMessageTimeToLive() {
return getModel().getDefaultMessageTimeToLive();
}
/**
* Sets the default message time-to-live (TTL). This applies when dead
* lettering is in effect.
*
* @param value
* A Duration
object that represents the default
* message TTL.
*
* @return A SubscriptionInfo
object that represents the
* updated subscription.
*/
public SubscriptionInfo setDefaultMessageTimeToLive(Duration value) {
getModel().setDefaultMessageTimeToLive(value);
return this;
}
/**
* Indicates whether dead lettering is in effect upon message expiration.
*
* @return true
if dead lettering is in effect; otherwise,
* false
.
*/
public Boolean isDeadLetteringOnMessageExpiration() {
return getModel().isDeadLetteringOnMessageExpiration();
}
/**
* Specifies whether dead lettering is in effect upon message expiration.
*
* @param value
* true
if dead lettering is in effect; otherwise,
* false
.
*
* @return A SubscriptionInfo
object that represents the
* updated subscription.
*/
public SubscriptionInfo setDeadLetteringOnMessageExpiration(Boolean value) {
getModel().setDeadLetteringOnMessageExpiration(value);
return this;
}
/**
* Indicates whether dead lettering is in effect when filter evaluation
* exceptions are encountered.
*
* @return true
if dead lettering is in effect; otherwise,
* false
.
*/
public Boolean isDeadLetteringOnFilterEvaluationExceptions() {
return getModel().isDeadLetteringOnFilterEvaluationExceptions();
}
/**
* Specifies whether dead lettering is in effect when filter evaluation
* exceptions are encountered.
*
* @param value
* true
if dead lettering is in effect; otherwise,
* false
.
*
* @return A SubscriptionInfo
object that represents the
* updated subscription.
*/
public SubscriptionInfo setDeadLetteringOnFilterEvaluationExceptions(
Boolean value) {
getModel().setDeadLetteringOnFilterEvaluationExceptions(value);
return this;
}
/**
* Returns the description of the default rule.
*
* @return A RuleDescription
object that represents the default
* rule description.
*/
public RuleDescription getDefaultRuleDescription() {
return getModel().getDefaultRuleDescription();
}
/**
* Specifies the description for the default rule.
*
* @param value
* A RuleDescription
object that represents the
* default rule description.
*
* @return A SubscriptionInfo
object that represents the
* updated subscription.
*/
public SubscriptionInfo setDefaultRuleDescription(RuleDescription value) {
getModel().setDefaultRuleDescription(value);
return this;
}
/**
* Returns the number of messages in the subscription.
*
* @return A Long
object represents the count of the message.
*/
public Long getMessageCount() {
return getModel().getMessageCount();
}
/**
* Returns the maximum delivery count for the subscription.
*
* @return A Integer
represents the maximum delivery count.
*/
public Integer getMaxDeliveryCount() {
return getModel().getMaxDeliveryCount();
}
/**
* Sets the maximum delivery count for the subscription.
*
* @param value
* A value
represents the maximum delivery count for
* the subscription.
*
* @return A SubscriptionInfo
object that represents the
* updated subscription.
*/
public SubscriptionInfo setMaxDeliveryCount(Integer value) {
getModel().setMaxDeliveryCount(value);
return this;
}
/**
* Indicates whether batch operations are enabled.
*
* @return true
if batch operations are enabled; otherwise,
* false
.
*/
public Boolean isEnableBatchedOperations() {
return getModel().isEnableBatchedOperations();
}
/**
* Specifies whether batch operations are enabled.
*
* @param value
* true
if batch operations are enabled; otherwise,
* false
.
*
* @return A SubscriptionInfo
object that represents the
* updated subscription.
*/
public SubscriptionInfo setEnableBatchedOperations(Boolean value) {
getModel().setEnableBatchedOperations(value);
return this;
}
/**
* Sets the message count.
*
* @param messageCount
* A Long
object represents the message count.
* @return A SubscriptionInfo
object that represents the
* updated subscription.
*/
public SubscriptionInfo setMessageCount(Long messageCount) {
getModel().setMessageCount(messageCount);
return this;
}
/**
* Sets the status.
*
* @param entityStatus
* A EntityStatus
object represents the status of
* the entity.
* @return A SubscriptionInfo
object that represents the
* updated subscription.
*/
public SubscriptionInfo setStatus(EntityStatus entityStatus) {
getModel().setStatus(entityStatus);
return this;
}
/**
* Gets the status.
*
* @return A EntityStatus
object represents the status of the
* entity.
*/
public EntityStatus getStatus() {
return getModel().getStatus();
}
/**
* Sets the created at.
*
* @param createdAt
* the created at
* @return A SubscriptionInfo
object that represents the
* updated subscription.
*/
public SubscriptionInfo setCreatedAt(Calendar createdAt) {
getModel().setCreatedAt(createdAt);
return this;
}
/**
* Gets the created at.
*
* @return the created at
*/
public Calendar getCreatedAt() {
return getModel().getCreatedAt();
}
/**
* Sets the updated at.
*
* @param updatedAt
* the updated at
* @return A SubscriptionInfo
object that represents the
* updated subscription.
*/
public SubscriptionInfo setUpdatedAt(Calendar updatedAt) {
getModel().setUpdatedAt(updatedAt);
return this;
}
/**
* Gets the updated at.
*
* @return the updated at
*/
public Calendar getUpdatedAt() {
return getModel().getUpdatedAt();
}
/**
* Sets the accessed at.
*
* @param accessedAt
* the accessed at
* @return A SubscriptionInfo
object that represents the
* updated subscription.
*/
public SubscriptionInfo setAccessedAt(Calendar accessedAt) {
getModel().setAccessedAt(accessedAt);
return this;
}
/**
* Gets the accessed at.
*
* @return the accessed at
*/
public Calendar getAccessedAt() {
return getModel().getAccessedAt();
}
/**
* Sets the user metadata.
*
* @param userMetadata
* the user metadata
* @return A SubscriptionInfo
object that represents the
* updated subscription.
*/
public SubscriptionInfo setUserMetadata(String userMetadata) {
getModel().setUserMetadata(userMetadata);
return this;
}
/**
* Gets the user metadata.
*
* @return the user metadata
*/
public String getUserMetadata() {
return getModel().getUserMetadata();
}
/**
* Sets the auto delete on idle.
*
* @param autoDeleteOnIdle
* A Duration
object represents the auto delete on
* idle.
* @return A SubscriptionInfo
object that represents the
* updated subscription.
*/
public SubscriptionInfo setAutoDeleteOnIdle(Duration autoDeleteOnIdle) {
getModel().setAutoDeleteOnIdle(autoDeleteOnIdle);
return this;
}
/**
* Gets the auto delete on idle.
*
* @return A Duration
object represents the auto delete on
* idle.
*/
public Duration getAutoDeleteOnIdle() {
return getModel().getAutoDeleteOnIdle();
}
/**
* Sets the entity availability status.
*
* @param entityAvailabilityStatus
* An EntityAvailabilityStatus
instance representing
* the entity availiability status.
* @return A SubscriptionInfo
object that represents the
* updated subscription.
*/
public SubscriptionInfo setEntityAvailabilityStatus(
EntityAvailabilityStatus entityAvailabilityStatus) {
getModel().setEntityAvailabilityStatus(entityAvailabilityStatus);
return this;
}
/**
* Gets the entity availability status.
*
* @return An EntityAvailabilityStatus
instance representing
* the entity availiability status.
*/
public EntityAvailabilityStatus getEntityAvailabilityStatus() {
return getModel().getEntityAvailabilityStatus();
}
/**
* Gets the message count details.
*
* @return A MessageCountDetails
instance representing the
* details of the message count.
*/
public MessageCountDetails getCountDetails() {
return getModel().getCountDetails();
}
/**
* Sets the forward to.
*
* @param forwardTo
* A String
representing the string to forward to.
* @return the subscription info
*/
public SubscriptionInfo setForwardTo(String forwardTo) {
getModel().setForwardTo(forwardTo);
return this;
}
/**
* Gets a String
representing the URI of the entity to forward
* to.
*
* @return A String
representing the URI of the entity to
* forward to.
*/
public String getForwardTo() {
return getModel().getForwardTo();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy