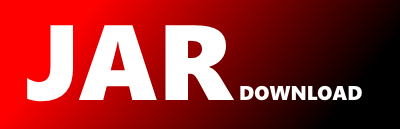
com.microsoft.windowsazure.services.table.models.Property Maven / Gradle / Ivy
/**
* Copyright Microsoft Corporation
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.microsoft.windowsazure.services.table.models;
/**
* Represents a table entity property value as a data-type and value pair.
*
* The following table shows the supported property data types in Windows Azure storage and the corresponding Java types
* when deserialized.
*
*
* Storage Type
* EdmType Value
* Java Type
* Description
*
*
* Edm.Binary
* {@link EdmType#BINARY}
* byte[], Byte[]
* An array of bytes up to 64 KB in size.
*
*
* Edm.Boolean
* {@link EdmType#BOOLEAN}
* boolean, Boolean
* A Boolean value.
*
*
* Edm.DateTime
* {@link EdmType#DATETIME}
* Date
* A 64-bit value expressed as Coordinated Universal Time (UTC). The supported range begins from 12:00 midnight,
* January 1, 1601 A.D. (C.E.), UTC. The range ends at December 31, 9999.
*
*
* Edm.Double
* {@link EdmType#DOUBLE}
* double, Double
* A 64-bit double-precision floating point value.
*
*
* Edm.Guid
* {@link EdmType#GUID}
* UUID
* A 128-bit globally unique identifier.
*
*
* Edm.Int32
* {@link EdmType#INT32}
* int, Integer
* A 32-bit integer value.
*
*
* Edm.Int64
* {@link EdmType#INT64}
* long, Long
* A 64-bit integer value.
*
*
* Edm.String
* {@link EdmType#STRING}
* String
* A UTF-16-encoded value. String values may be up to 64 KB in size.
*
*
*
* See the MSDN topic Understanding the
* Table Service Data Model for an overview of tables, entities, and properties as used in the Windows Azure Storage
* service.
*
* For an overview of the available EDM primitive data types and names, see the Primitive Data Types section of the
* OData Protocol Overview.
*/
public class Property {
private String edmType;
private Object value;
/**
* Gets the EDM data type of the property.
*
* @return
* A String
containing the EDM data type of the property.
*/
public String getEdmType() {
return edmType;
}
/**
* Sets the EDM data type of the property. The edmType parameter must be set to one of the string constants
* defined in the {@link EdmType} class.
*
* @param edmType
* A {@link String} containing the EDM data type to associate with the property value. This must be one
* of the supported EDM types, defined as string constants in the {@link EdmType} class.
* @return
* A reference to this {@link Property} instance.
*/
public Property setEdmType(String edmType) {
this.edmType = edmType;
return this;
}
/**
* Gets the data value of the property.
*
* @return
* An {@link Object} containing the data value of the property.
*/
public Object getValue() {
return value;
}
/**
* Sets the data value of the property. The value parameter must contain an {@link Object} serializable as
* the associated EDM data type of the property.
*
* @param value
* An {@link Object} containing the data value of the property, serializable as the associated EDM data
* type of the property.
* @return
* A reference to this {@link Property} instance.
*/
public Property setValue(Object value) {
this.value = value;
return this;
}
}