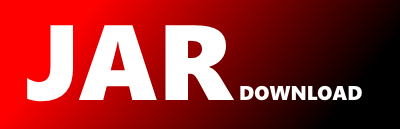
com.microsoft.durabletask.CompositeTaskFailedException Maven / Gradle / Ivy
Show all versions of durabletask-client Show documentation
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
package com.microsoft.durabletask;
import java.util.ArrayList;
import java.util.List;
/**
* Exception that gets thrown when multiple {@link Task}s for an activity or sub-orchestration fails with an
* unhandled exception.
*
* Detailed information associated with each task failure can be retrieved using the {@link #getExceptions()}
* method.
*/
public class CompositeTaskFailedException extends RuntimeException {
private final List exceptions;
CompositeTaskFailedException() {
this.exceptions = new ArrayList<>();
}
CompositeTaskFailedException(List exceptions) {
this.exceptions = exceptions;
}
CompositeTaskFailedException(String message, List exceptions) {
super(message);
this.exceptions = exceptions;
}
CompositeTaskFailedException(String message, Throwable cause, List exceptions) {
super(message, cause);
this.exceptions = exceptions;
}
CompositeTaskFailedException(Throwable cause, List exceptions) {
super(cause);
this.exceptions = exceptions;
}
CompositeTaskFailedException(String message, Throwable cause, boolean enableSuppression, boolean writableStackTrace, List exceptions) {
super(message, cause, enableSuppression, writableStackTrace);
this.exceptions = exceptions;
}
/**
* Gets a list of exceptions that occurred during execution of a group of {@link Task}
* These exceptions include details of the task failure and exception information
*
* @return a list of exceptions
*/
public List getExceptions() {
return new ArrayList<>(this.exceptions);
}
}