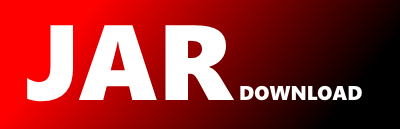
com.mindoo.domino.jna.formula.FormulaExecution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of domino-jna Show documentation
Show all versions of domino-jna Show documentation
Java project to access the HCL Domino C API using Java Native Access (JNA)
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy