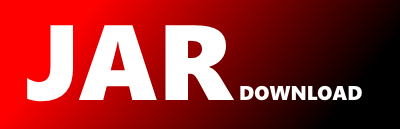
com.minlia.cloud.utils.AccessLogUtils Maven / Gradle / Ivy
///**
// * Copyright © 2012-2016 JeeSite All rights reserved.
// */
//package com.minlia.cloud.utils;
//
//import com.google.common.collect.Lists;
//import com.google.common.collect.Maps;
//import com.minlia.cloud.dao.LogDao;
//import com.minlia.cloud.dao.MenuDao;
//import com.minlia.cloud.exception.Exceptions;
//import com.minlia.cloud.holder.SpringContextHolder;
//import com.minlia.cloud.persistence.domain.Log;
//import com.minlia.cloud.persistence.domain.Menu;
//import jdk.nashorn.internal.objects.Global;
//import org.springframework.web.method.HandlerMethod;
//
//import javax.servlet.http.HttpServletRequest;
//import java.lang.reflect.Method;
//import java.util.List;
//import java.util.Map;
//
///**
// * 字典工具类
// * @author ThinkGem
// * @version 2014-11-7
// */
//public class AccessLogUtils {
//
// public static final String CACHE_MENU_NAME_PATH_MAP = "menuNamePathMap";
//
// private static LogDao logDao = SpringContextHolder.getBean(LogDao.class);
// private static MenuDao menuDao = SpringContextHolder.getBean(MenuDao.class);
//
// /**
// * 保存日志
// */
// public static void saveLog(HttpServletRequest request, String title){
// saveLog(request, null, null, title);
// }
//
// /**
// * 保存日志
// */
// public static void saveLog(HttpServletRequest request, Object handler, Exception ex, String title){
// User user = UserUtils.getUser();
// if (user != null && user.getId() != null){
// Log log = new Log();
// log.setTitle(title);
// log.setType(ex == null ? Log.TYPE_ACCESS : Log.TYPE_EXCEPTION);
// log.setRemoteAddr(StringUtils.getRemoteAddr(request));
// log.setUserAgent(request.getHeader("user-agent"));
// log.setRequestUri(request.getRequestURI());
// log.setParams(request.getParameterMap());
// log.setMethod(request.getMethod());
// // 异步保存日志
// new SaveLogThread(log, handler, ex).start();
// }
// }
//
// /**
// * 保存日志线程
// */
// public static class SaveLogThread extends Thread{
//
// private Log log;
// private Object handler;
// private Exception ex;
//
// public SaveLogThread(Log log, Object handler, Exception ex){
// super(SaveLogThread.class.getSimpleName());
// this.log = log;
// this.handler = handler;
// this.ex = ex;
// }
//
// @Override
// public void run() {
// // 获取日志标题
// if (StringUtils.isBlank(log.getTitle())){
// String permission = "";
// if (handler instanceof HandlerMethod){
// Method m = ((HandlerMethod)handler).getMethod();
// RequiresPermissions rp = m.getAnnotation(RequiresPermissions.class);
// permission = (rp != null ? StringUtils.join(rp.value(), ",") : "");
// }
// log.setTitle(getMenuNamePath(log.getRequestUri(), permission));
// }
// // 如果有异常,设置异常信息
// log.setException(Exceptions.getStackTraceAsString(ex));
// // 如果无标题并无异常日志,则不保存信息
// if (StringUtils.isBlank(log.getTitle()) && StringUtils.isBlank(log.getException())){
// return;
// }
// // 保存日志信息
// log.preInsert();
// logDao.insert(log);
// }
// }
//
// /**
// * 获取菜单名称路径(如:系统设置-机构用户-用户管理-编辑)
// */
// public static String getMenuNamePath(String requestUri, String permission){
// String href = StringUtils.substringAfter(requestUri, Global.getAdminPath());
// @SuppressWarnings("unchecked")
// Map menuMap = (Map)CacheUtils.get(CACHE_MENU_NAME_PATH_MAP);
// if (menuMap == null){
// menuMap = Maps.newHashMap();
// List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy