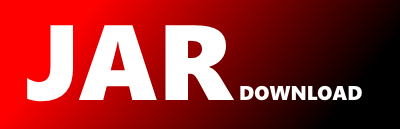
com.mizhousoft.redis.lettuce.command.LettuceSetCommand Maven / Gradle / Ivy
package com.mizhousoft.redis.lettuce.command;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import com.mizhousoft.redis.codec.Codec;
import com.mizhousoft.redis.command.SetCommand;
import com.mizhousoft.redis.lettuce.client.LettuceRedisClient;
import io.lettuce.core.api.sync.RedisCommands;
/**
* SetCommand
*
* @version
*/
public class LettuceSetCommand extends AbstractRedisCommand implements SetCommand
{
/**
* 构造函数
*
* @param redisClient
*/
public LettuceSetCommand(LettuceRedisClient redisClient)
{
super(redisClient);
}
/**
* {@inheritDoc}
*/
@Override
public Set smembers(String key, Codec codec)
{
RedisCommands redisCommands = redisClient.getRedisCommands();
Set values = redisCommands.smembers(key);
Set results = new HashSet<>(values.size());
for (String value : values)
{
T t = codec.decode(value);
results.add(t);
}
return results;
}
/**
* {@inheritDoc}
*/
@Override
public long scard(String key)
{
RedisCommands redisCommands = redisClient.getRedisCommands();
return redisCommands.scard(key);
}
/**
* {@inheritDoc}
*/
@Override
public boolean sismember(String key, T o, Codec codec)
{
RedisCommands redisCommands = redisClient.getRedisCommands();
return redisCommands.sismember(key, codec.encode(o));
}
/**
* {@inheritDoc}
*/
@Override
public long sadd(String key, T[] values, Codec codec)
{
RedisCommands redisCommands = redisClient.getRedisCommands();
List list = new ArrayList<>(values.length);
for (int i = 0; i < values.length; ++i)
{
String value = codec.encode(values[i]);
list.add(value);
}
String[] ms = list.toArray(new String[list.size()]);
return redisCommands.sadd(key, ms);
}
/**
* {@inheritDoc}
*/
@Override
public long srem(String key, T[] values, Codec codec)
{
RedisCommands redisCommands = redisClient.getRedisCommands();
List list = new ArrayList<>(values.length);
for (int i = 0; i < values.length; ++i)
{
String value = codec.encode(values[i]);
list.add(value);
}
String[] ms = list.toArray(new String[list.size()]);
return redisCommands.srem(key, ms);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy