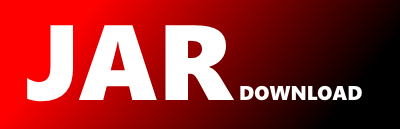
com.mizhousoft.redis.lettuce.command.LettuceZSetCommand Maven / Gradle / Ivy
package com.mizhousoft.redis.lettuce.command;
import java.util.ArrayList;
import java.util.List;
import com.mizhousoft.redis.ScoreValue;
import com.mizhousoft.redis.codec.Codec;
import com.mizhousoft.redis.command.ZSetCommand;
import com.mizhousoft.redis.lettuce.client.LettuceRedisClient;
import io.lettuce.core.ScoredValue;
import io.lettuce.core.api.sync.RedisCommands;
/**
* Sorted Set Command
*
* @version
* @param
*/
public class LettuceZSetCommand extends AbstractRedisCommand implements ZSetCommand
{
/**
* 构造函数
*
* @param redisClient
*/
public LettuceZSetCommand(LettuceRedisClient redisClient)
{
super(redisClient);
}
/**
* {@inheritDoc}
*/
@Override
public Long zadd(String key, double score, T member, Codec codec)
{
String value = codec.encode(member);
RedisCommands redisCommands = redisClient.getRedisCommands();
return redisCommands.zadd(key, score, value);
}
/**
* {@inheritDoc}
*/
@Override
public Double zaddincr(String key, double score, T member, Codec codec)
{
String value = codec.encode(member);
RedisCommands redisCommands = redisClient.getRedisCommands();
return redisCommands.zaddincr(key, score, value);
}
/**
* {@inheritDoc}
*/
@Override
public Long zcard(String key)
{
RedisCommands redisCommands = redisClient.getRedisCommands();
return redisCommands.zcard(key);
}
/**
* {@inheritDoc}
*/
@Override
public List zrange(String key, long start, long stop, Codec codec)
{
RedisCommands redisCommands = redisClient.getRedisCommands();
List values = redisCommands.zrange(key, start, stop);
List results = new ArrayList<>(values.size());
for (String value : values)
{
T t = codec.decode(value);
results.add(t);
}
return results;
}
/**
* {@inheritDoc}
*/
@Override
public List> zrangeWithScores(String key, long start, long stop, Codec codec)
{
RedisCommands redisCommands = redisClient.getRedisCommands();
List> values = redisCommands.zrangeWithScores(key, start, stop);
List> results = new ArrayList<>(values.size());
for (ScoredValue value : values)
{
T t = codec.decode(value.getValue());
ScoreValue v = new ScoreValue(t, value.getScore());
results.add(v);
}
return results;
}
/**
* {@inheritDoc}
*/
@Override
public List zrevrange(String key, long start, long stop, Codec codec)
{
RedisCommands redisCommands = redisClient.getRedisCommands();
List values = redisCommands.zrevrange(key, start, stop);
List results = new ArrayList<>(values.size());
for (String value : values)
{
T t = codec.decode(value);
results.add(t);
}
return results;
}
/**
* {@inheritDoc}
*/
@Override
public List> zrevrangeWithScores(String key, long start, long stop, Codec codec)
{
RedisCommands redisCommands = redisClient.getRedisCommands();
List> values = redisCommands.zrevrangeWithScores(key, start, stop);
List> results = new ArrayList<>(values.size());
for (ScoredValue value : values)
{
T t = codec.decode(value.getValue());
ScoreValue v = new ScoreValue(t, value.getScore());
results.add(v);
}
return results;
}
/**
* {@inheritDoc}
*/
@Override
public T zpopmin(String key, Codec codec)
{
RedisCommands redisCommands = redisClient.getRedisCommands();
ScoredValue value = redisCommands.zpopmin(key);
return codec.decode(value.getValue());
}
/**
* {@inheritDoc}
*/
@Override
public List zpopmin(String key, long count, Codec codec)
{
RedisCommands redisCommands = redisClient.getRedisCommands();
List> list = redisCommands.zpopmin(key, count);
List results = new ArrayList<>(list.size());
for (ScoredValue value : list)
{
T t = codec.decode(value.getValue());
results.add(t);
}
return results;
}
/**
* {@inheritDoc}
*/
@Override
public Long zrem(String key, T[] members, Codec codec)
{
RedisCommands redisCommands = redisClient.getRedisCommands();
List values = new ArrayList<>(members.length);
for (int i = 0; i < members.length; ++i)
{
String value = codec.encode(members[i]);
values.add(value);
}
String[] ms = values.toArray(new String[values.size()]);
return redisCommands.zrem(key, ms);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy