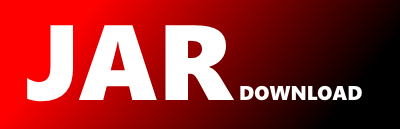
com.mmnaseri.utils.tuples.Tuple Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tuples4j Show documentation
Show all versions of tuples4j Show documentation
Tiny framework for using tuples as first-class citizens in Java.
package com.mmnaseri.utils.tuples;
import com.mmnaseri.utils.tuples.facade.HasEighth;
import com.mmnaseri.utils.tuples.facade.HasEleventh;
import com.mmnaseri.utils.tuples.facade.HasFifth;
import com.mmnaseri.utils.tuples.facade.HasFirst;
import com.mmnaseri.utils.tuples.facade.HasFourth;
import com.mmnaseri.utils.tuples.facade.HasNinth;
import com.mmnaseri.utils.tuples.facade.HasSecond;
import com.mmnaseri.utils.tuples.facade.HasSeventh;
import com.mmnaseri.utils.tuples.facade.HasSixth;
import com.mmnaseri.utils.tuples.facade.HasTenth;
import com.mmnaseri.utils.tuples.facade.HasThird;
import com.mmnaseri.utils.tuples.facade.HasTwelfth;
import com.mmnaseri.utils.tuples.impl.DefaultLabeledTuple;
import com.mmnaseri.utils.tuples.impl.EightTuple;
import com.mmnaseri.utils.tuples.impl.ElevenTuple;
import com.mmnaseri.utils.tuples.impl.EmptyTuple;
import com.mmnaseri.utils.tuples.impl.FiveTuple;
import com.mmnaseri.utils.tuples.impl.FourTuple;
import com.mmnaseri.utils.tuples.impl.NineTuple;
import com.mmnaseri.utils.tuples.impl.OneTuple;
import com.mmnaseri.utils.tuples.impl.SevenTuple;
import com.mmnaseri.utils.tuples.impl.SixTuple;
import com.mmnaseri.utils.tuples.impl.TenTuple;
import com.mmnaseri.utils.tuples.impl.ThirteenOrMoreTuple;
import com.mmnaseri.utils.tuples.impl.ThreeTuple;
import com.mmnaseri.utils.tuples.impl.TwelveTuple;
import com.mmnaseri.utils.tuples.impl.TwoTuple;
import com.mmnaseri.utils.tuples.utils.FluentList;
import java.util.Arrays;
import java.util.List;
import java.util.function.Function;
import java.util.function.IntFunction;
import java.util.function.Predicate;
import java.util.function.Supplier;
import java.util.stream.IntStream;
import java.util.stream.Stream;
import static com.mmnaseri.utils.tuples.utils.TupleUtils.checkSize;
/**
* The base definition for a tuple.
*
* @author Milad Naseri ([email protected])
*/
public interface Tuple {
/** Returns the size of the tuple. */
int size();
/**
* Returns the element at the given position. Index must be between zero and the {@link #size()}
* of this tuple.
*/
Z get(int index);
/** Changes the value of the element at the given index to the new value. */
default Tuple change(int index, Z value) {
return change(index, () -> value);
}
/** Changes the value of the element at the given index to the value provided by the supplier. */
Tuple change(int index, Supplier extends Z> value);
/** Returns a new, empty tuple. This is the same as called {@link #empty()}. */
Tuple clear();
/** Returns a new tuple formed by dropping the element at the indicated index. */
Tuple drop(int index);
/** Returns a stream of all the elements in this tuple. */
default Stream stream() {
return IntStream.range(0, size()).boxed().map(this::get);
}
/** Returns the items in the tuple as an instance of {@link FluentList}. */
FluentList asList();
/**
* Extends this tuple by returning a new tuple that has the provided element added to the end of
* the elements in this tuple.
*/
default Tuple extend(X value) {
return extend((Supplier) () -> value);
}
/**
* Extends this tuple by returning a new tuple that has the element returned from the supplier
* added to the end of the elements in this tuple.
*/
Tuple extend(Supplier value);
/** Checks the indicated element in the tuple against the provided predicate. */
default boolean check(int index, Predicate predicate) {
return predicate.test(get(index));
}
/**
* Returns a new {@link LabeledTuple} by labeling the elements in the tuple with the corresponding
* labels.
*/
default LabeledTuple withLabels(String... labels) {
return withLabels(Arrays.asList(labels));
}
/**
* Returns a new {@link LabeledTuple} by labeling the elements in the tuple with the corresponding
* labels.
*/
default LabeledTuple withLabels(List labels) {
return new DefaultLabeledTuple<>(this, labels);
}
/** Creates an array from the current tuple. */
default Z[] asArray(IntFunction generator) {
return stream().toArray(generator);
}
/** Creates an array from the current tuple. */
default Object[] asArray() {
return stream().toArray();
}
/**
* Returns an instance of {@link EmptyTuple} that gets all of its corresponding values from this
* tuple.
*/
default EmptyTuple