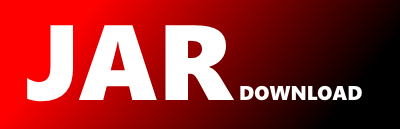
com.mmnaseri.utils.tuples.facade.HasFirst Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tuples4j Show documentation
Show all versions of tuples4j Show documentation
Tiny framework for using tuples as first-class citizens in Java.
package com.mmnaseri.utils.tuples.facade;
import com.mmnaseri.utils.tuples.FixedTuple;
import com.mmnaseri.utils.tuples.Tuple;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.function.Supplier;
/**
* Defines methods that work with the first element of a {@link FixedTuple}.
*
* @param the super-type of the tuple's main data type.
* @param the type of the object at the first position.
* @param the concrete type of the fixed-size tuple.
* @author Milad Naseri ([email protected])
*/
public interface HasFirst> extends FixedTuple {
/** Returns the first element in the current tuple. */
@SuppressWarnings("unchecked")
default A first() {
return (A) get(0);
}
/** Sets the first element of the tuple to the indicated value. */
Tuple first(X value);
/** Sets the first element of the tuple to the supplied value. */
Tuple first(Supplier supplier);
/** Sets the first element of the tuple to the value returned from the function. */
Tuple first(Function function);
/** Drops the first element of the tuple, to return a tuple of one size smaller. */
Tuple dropFirst();
/** Checks to see if the first element of this tuple matches the given predicate. */
default boolean checkFirst(Predicate predicate) {
return predicate.test(first());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy