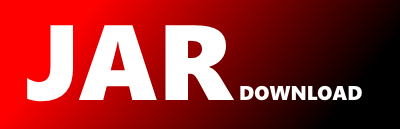
com.mmnaseri.utils.tuples.impl.EightTuple Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tuples4j Show documentation
Show all versions of tuples4j Show documentation
Tiny framework for using tuples as first-class citizens in Java.
package com.mmnaseri.utils.tuples.impl;
import com.mmnaseri.utils.tuples.facade.HasEighth;
import com.mmnaseri.utils.tuples.facade.HasFifth;
import com.mmnaseri.utils.tuples.facade.HasFirst;
import com.mmnaseri.utils.tuples.facade.HasFourth;
import com.mmnaseri.utils.tuples.facade.HasSecond;
import com.mmnaseri.utils.tuples.facade.HasSeventh;
import com.mmnaseri.utils.tuples.facade.HasSixth;
import com.mmnaseri.utils.tuples.facade.HasThird;
import java.util.function.Function;
import java.util.function.Supplier;
import static com.mmnaseri.utils.tuples.utils.TupleUtils.checkIndex;
/**
* Class for dealing with a {@link com.mmnaseri.utils.tuples.FixedTuple} with eight elements.
*
* @author Milad Naseri ([email protected])
*/
public class EightTuple<
Z,
A extends Z,
B extends Z,
C extends Z,
D extends Z,
E extends Z,
F extends Z,
G extends Z,
H extends Z>
extends AbstractFixedTuple>
implements HasFirst>,
HasSecond>,
HasThird>,
HasFourth>,
HasFifth>,
HasSixth>,
HasSeventh>,
HasEighth> {
/** Creates a new instance of this class from the provided values. */
public EightTuple(A first, B second, C third, D fourth, E fifth, F sixth, G seventh, H eighth) {
super(first, second, third, fourth, fifth, sixth, seventh, eighth);
}
/**
* Returns a new tuple by keeping all the values from this tuple and overriding the value at the
* provided index with the value returned from the supplier.
*/
@Override
public EightTuple change(int index, Supplier extends Z> supplier) {
checkIndex(index, size());
return new EightTuple<>(
index == 0 ? supplier.get() : first(),
index == 1 ? supplier.get() : second(),
index == 2 ? supplier.get() : third(),
index == 3 ? supplier.get() : fourth(),
index == 4 ? supplier.get() : fifth(),
index == 5 ? supplier.get() : sixth(),
index == 6 ? supplier.get() : seventh(),
index == 7 ? supplier.get() : eighth());
}
/**
* Returns a new tuple by keeping all the values from this tuple and overriding the value at the
* provided index with the value returned from the function.
*/
@Override
public EightTuple change(
int index, Function, ? extends Z> function) {
checkIndex(index, size());
return new EightTuple<>(
index == 0 ? function.apply(this) : first(),
index == 1 ? function.apply(this) : second(),
index == 2 ? function.apply(this) : third(),
index == 3 ? function.apply(this) : fourth(),
index == 4 ? function.apply(this) : fifth(),
index == 5 ? function.apply(this) : sixth(),
index == 6 ? function.apply(this) : seventh(),
index == 7 ? function.apply(this) : eighth());
}
/**
* Returns a new tuple of one size larger by adding the provided value to the end of this tuple.
*/
@Override
public NineTuple extend(X value) {
return new NineTuple<>(
first(), second(), third(), fourth(), fifth(), sixth(), seventh(), eighth(), value);
}
/**
* Returns a new tuple of one size larger by adding the value returned from the supplier to the
* end of this tuple.
*/
@Override
public NineTuple extend(Supplier supplier) {
return new NineTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
supplier.get());
}
/**
* Returns a new tuple of one size larger by adding the value returned from the function to the
* end of this tuple.
*/
@Override
public NineTuple extend(
Function, X> function) {
return new NineTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
function.apply(this));
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the first element with the provided value.
*/
@Override
public EightTuple first(X value) {
return new EightTuple<>(
value, second(), third(), fourth(), fifth(), sixth(), seventh(), eighth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the first element with the value returned from the given supplier.
*/
@Override
public EightTuple first(Supplier supplier) {
return new EightTuple<>(
supplier.get(), second(), third(), fourth(), fifth(), sixth(), seventh(), eighth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the first element with the value returned by applying the given function to this tuple's first
* element.
*/
@Override
public EightTuple first(Function function) {
return new EightTuple<>(
function.apply(first()),
second(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth());
}
/**
* Returns a new tuple of one size smaller by keeping all the values from this tuple except the
* first element.
*/
@Override
public SevenTuple dropFirst() {
return new SevenTuple<>(second(), third(), fourth(), fifth(), sixth(), seventh(), eighth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the second element with the provided value.
*/
@Override
public EightTuple second(X value) {
return new EightTuple<>(
first(), value, third(), fourth(), fifth(), sixth(), seventh(), eighth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the second element with the value returned from the given supplier.
*/
@Override
public EightTuple second(Supplier supplier) {
return new EightTuple<>(
first(), supplier.get(), third(), fourth(), fifth(), sixth(), seventh(), eighth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the second element with the value returned by applying the given function to this tuple's
* second element.
*/
@Override
public EightTuple second(Function function) {
return new EightTuple<>(
first(),
function.apply(second()),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth());
}
/**
* Returns a new tuple of one size smaller by keeping all the values from this tuple except the
* second element.
*/
@Override
public SevenTuple dropSecond() {
return new SevenTuple<>(first(), third(), fourth(), fifth(), sixth(), seventh(), eighth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the third element with the provided value.
*/
@Override
public EightTuple third(X value) {
return new EightTuple<>(
first(), second(), value, fourth(), fifth(), sixth(), seventh(), eighth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the third element with the value returned from the given supplier.
*/
@Override
public EightTuple third(Supplier supplier) {
return new EightTuple<>(
first(), second(), supplier.get(), fourth(), fifth(), sixth(), seventh(), eighth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the third element with the value returned by applying the given function to this tuple's third
* element.
*/
@Override
public EightTuple third(Function function) {
return new EightTuple<>(
first(),
second(),
function.apply(third()),
fourth(),
fifth(),
sixth(),
seventh(),
eighth());
}
/**
* Returns a new tuple of one size smaller by keeping all the values from this tuple except the
* third element.
*/
@Override
public SevenTuple dropThird() {
return new SevenTuple<>(first(), second(), fourth(), fifth(), sixth(), seventh(), eighth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the fourth element with the provided value.
*/
@Override
public EightTuple fourth(X value) {
return new EightTuple<>(
first(), second(), third(), value, fifth(), sixth(), seventh(), eighth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the fourth element with the value returned from the given supplier.
*/
@Override
public EightTuple fourth(Supplier supplier) {
return new EightTuple<>(
first(), second(), third(), supplier.get(), fifth(), sixth(), seventh(), eighth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the fourth element with the value returned by applying the given function to this tuple's
* fourth element.
*/
@Override
public EightTuple fourth(Function function) {
return new EightTuple<>(
first(),
second(),
third(),
function.apply(fourth()),
fifth(),
sixth(),
seventh(),
eighth());
}
/**
* Returns a new tuple of one size smaller by keeping all the values from this tuple except the
* fourth element.
*/
@Override
public SevenTuple dropFourth() {
return new SevenTuple<>(first(), second(), third(), fifth(), sixth(), seventh(), eighth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the fifth element with the provided value.
*/
@Override
public EightTuple fifth(X value) {
return new EightTuple<>(
first(), second(), third(), fourth(), value, sixth(), seventh(), eighth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the fifth element with the value returned from the given supplier.
*/
@Override
public EightTuple fifth(Supplier supplier) {
return new EightTuple<>(
first(), second(), third(), fourth(), supplier.get(), sixth(), seventh(), eighth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the fifth element with the value returned by applying the given function to this tuple's fifth
* element.
*/
@Override
public EightTuple fifth(Function function) {
return new EightTuple<>(
first(),
second(),
third(),
fourth(),
function.apply(fifth()),
sixth(),
seventh(),
eighth());
}
/**
* Returns a new tuple of one size smaller by keeping all the values from this tuple except the
* fifth element.
*/
@Override
public SevenTuple dropFifth() {
return new SevenTuple<>(first(), second(), third(), fourth(), sixth(), seventh(), eighth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the sixth element with the provided value.
*/
@Override
public EightTuple sixth(X value) {
return new EightTuple<>(
first(), second(), third(), fourth(), fifth(), value, seventh(), eighth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the sixth element with the value returned from the given supplier.
*/
@Override
public EightTuple sixth(Supplier supplier) {
return new EightTuple<>(
first(), second(), third(), fourth(), fifth(), supplier.get(), seventh(), eighth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the sixth element with the value returned by applying the given function to this tuple's sixth
* element.
*/
@Override
public EightTuple sixth(Function function) {
return new EightTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
function.apply(sixth()),
seventh(),
eighth());
}
/**
* Returns a new tuple of one size smaller by keeping all the values from this tuple except the
* sixth element.
*/
@Override
public SevenTuple dropSixth() {
return new SevenTuple<>(first(), second(), third(), fourth(), fifth(), seventh(), eighth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the seventh element with the provided value.
*/
@Override
public EightTuple seventh(X value) {
return new EightTuple<>(
first(), second(), third(), fourth(), fifth(), sixth(), value, eighth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the seventh element with the value returned from the given supplier.
*/
@Override
public EightTuple seventh(Supplier supplier) {
return new EightTuple<>(
first(), second(), third(), fourth(), fifth(), sixth(), supplier.get(), eighth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the seventh element with the value returned by applying the given function to this tuple's
* seventh element.
*/
@Override
public EightTuple seventh(Function function) {
return new EightTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
sixth(),
function.apply(seventh()),
eighth());
}
/**
* Returns a new tuple of one size smaller by keeping all the values from this tuple except the
* seventh element.
*/
@Override
public SevenTuple dropSeventh() {
return new SevenTuple<>(first(), second(), third(), fourth(), fifth(), sixth(), eighth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the eighth element with the provided value.
*/
@Override
public EightTuple eighth(X value) {
return new EightTuple<>(
first(), second(), third(), fourth(), fifth(), sixth(), seventh(), value);
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the eighth element with the value returned from the given supplier.
*/
@Override
public EightTuple eighth(Supplier supplier) {
return new EightTuple<>(
first(), second(), third(), fourth(), fifth(), sixth(), seventh(), supplier.get());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the eighth element with the value returned by applying the given function to this tuple's
* eighth element.
*/
@Override
public EightTuple eighth(Function function) {
return new EightTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
function.apply(eighth()));
}
/**
* Returns a new tuple of one size smaller by keeping all the values from this tuple except the
* eighth element.
*/
@Override
public SevenTuple dropEighth() {
return new SevenTuple<>(first(), second(), third(), fourth(), fifth(), sixth(), seventh());
}
/**
* Extends the tuple to which this is applied by adding the provided value to the end.
*
* This is especially useful in functional contexts. For instance:
*
*
* nineTupleStream = eightTupleStream.map(EightTuple.extendWith(value));
*
*
* @see #extend(Object)
*/
public static <
Z,
A extends Z,
B extends Z,
C extends Z,
D extends Z,
E extends Z,
F extends Z,
G extends Z,
H extends Z,
X extends Z>
Function, NineTuple>
extendWith(X value) {
return tuple -> tuple.extend(value);
}
/**
* Extends the tuple to which this is applied by adding the value from the supplier to the end.
*
* This is especially useful in functional contexts. For instance:
*
*
* nineTupleStream = eightTupleStream.map(EightTuple.extendWith(supplier));
*
*
* @see #extend(Object)
*/
public static <
Z,
A extends Z,
B extends Z,
C extends Z,
D extends Z,
E extends Z,
F extends Z,
G extends Z,
H extends Z,
X extends Z>
Function, NineTuple>
extendWith(Supplier supplier) {
return tuple -> tuple.extend(supplier);
}
/**
* Extends the tuple to which this is applied by adding the value from the function to the end.
*
* This is especially useful in functional contexts. For instance:
*
*
* nineTupleStream = eightTupleStream.map(EightTuple.extendWith(function));
*
*
* @see #extend(Object)
*/
public static <
Z,
A extends Z,
B extends Z,
C extends Z,
D extends Z,
E extends Z,
F extends Z,
G extends Z,
H extends Z,
X extends Z>
Function, NineTuple>
extendWith(Function, X> function) {
return tuple -> tuple.extend(function);
}
/** Creates a new instance of this class. */
public static <
Z,
A extends Z,
B extends Z,
C extends Z,
D extends Z,
E extends Z,
F extends Z,
G extends Z,
H extends Z>
EightTuple of(
A first, B second, C third, D fourth, E fifth, F sixth, G seventh, H eighth) {
return new EightTuple<>(first, second, third, fourth, fifth, sixth, seventh, eighth);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy