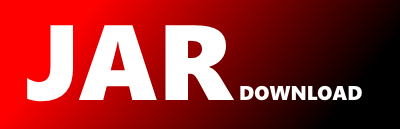
com.mmnaseri.utils.tuples.impl.EmptyTuple Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tuples4j Show documentation
Show all versions of tuples4j Show documentation
Tiny framework for using tuples as first-class citizens in Java.
package com.mmnaseri.utils.tuples.impl;
import java.util.function.Function;
import java.util.function.Supplier;
import static com.mmnaseri.utils.tuples.utils.TupleUtils.checkIndex;
/**
* Class for dealing with a {@link com.mmnaseri.utils.tuples.FixedTuple} with empty elements.
*
* @author Milad Naseri ([email protected])
*/
public class EmptyTuple extends AbstractFixedTuple> {
/**
* Returns a new tuple by keeping all the values from this tuple and overriding the value at the
* provided index with the value returned from the supplier.
*/
@Override
public EmptyTuple change(int index, Supplier extends Z> supplier) {
return checkIndex(index, size());
}
/**
* Returns a new tuple by keeping all the values from this tuple and overriding the value at the
* provided index with the value returned from the function.
*/
@Override
public EmptyTuple change(int index, Function, ? extends Z> function) {
return checkIndex(index, size());
}
/**
* Returns a new tuple of one size larger by adding the provided value to the end of this tuple.
*/
@Override
public OneTuple extend(X value) {
return new OneTuple<>(value);
}
/**
* Returns a new tuple of one size larger by adding the value returned from the supplier to the
* end of this tuple.
*/
@Override
public OneTuple extend(Supplier supplier) {
return new OneTuple<>(supplier.get());
}
/**
* Returns a new tuple of one size larger by adding the value returned from the function to the
* end of this tuple.
*/
@Override
public OneTuple extend(Function, X> function) {
return new OneTuple<>(function.apply(this));
}
/**
* Extends the tuple to which this is applied by adding the provided value to the end.
*
* This is especially useful in functional contexts. For instance:
*
*
* oneTupleStream = emptyTupleStream.map(EmptyTuple.extendWith(value));
*
*
* @see #extend(Object)
*/
public static Function, OneTuple> extendWith(X value) {
return tuple -> tuple.extend(value);
}
/**
* Extends the tuple to which this is applied by adding the value from the supplier to the end.
*
* This is especially useful in functional contexts. For instance:
*
*
* oneTupleStream = emptyTupleStream.map(EmptyTuple.extendWith(supplier));
*
*
* @see #extend(Object)
*/
public static Function, OneTuple> extendWith(
Supplier supplier) {
return tuple -> tuple.extend(supplier);
}
/**
* Extends the tuple to which this is applied by adding the value from the function to the end.
*
* This is especially useful in functional contexts. For instance:
*
*
* oneTupleStream = emptyTupleStream.map(EmptyTuple.extendWith(function));
*
*
* @see #extend(Object)
*/
public static Function, OneTuple> extendWith(
Function, X> function) {
return tuple -> tuple.extend(function);
}
/** Creates a new instance of this class. */
public static EmptyTuple of() {
return new EmptyTuple<>();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy