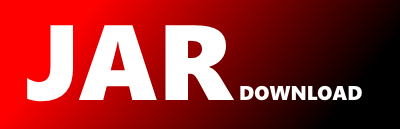
com.mmnaseri.utils.tuples.impl.OneTuple Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tuples4j Show documentation
Show all versions of tuples4j Show documentation
Tiny framework for using tuples as first-class citizens in Java.
package com.mmnaseri.utils.tuples.impl;
import com.mmnaseri.utils.tuples.facade.HasFirst;
import java.util.function.Function;
import java.util.function.Supplier;
import static com.mmnaseri.utils.tuples.utils.TupleUtils.checkIndex;
/**
* Class for dealing with a {@link com.mmnaseri.utils.tuples.FixedTuple} with one elements.
*
* @author Milad Naseri ([email protected])
*/
public class OneTuple extends AbstractFixedTuple>
implements HasFirst> {
/** Creates a new instance of this class from the provided values. */
public OneTuple(A first) {
super(first);
}
/**
* Returns a new tuple by keeping all the values from this tuple and overriding the value at the
* provided index with the value returned from the supplier.
*/
@Override
public OneTuple change(int index, Supplier extends Z> supplier) {
checkIndex(index, size());
return new OneTuple<>(index == 0 ? supplier.get() : first());
}
/**
* Returns a new tuple by keeping all the values from this tuple and overriding the value at the
* provided index with the value returned from the function.
*/
@Override
public OneTuple change(int index, Function, ? extends Z> function) {
checkIndex(index, size());
return new OneTuple<>(index == 0 ? function.apply(this) : first());
}
/**
* Returns a new tuple of one size larger by adding the provided value to the end of this tuple.
*/
@Override
public TwoTuple extend(X value) {
return new TwoTuple<>(first(), value);
}
/**
* Returns a new tuple of one size larger by adding the value returned from the supplier to the
* end of this tuple.
*/
@Override
public TwoTuple extend(Supplier supplier) {
return new TwoTuple<>(first(), supplier.get());
}
/**
* Returns a new tuple of one size larger by adding the value returned from the function to the
* end of this tuple.
*/
@Override
public TwoTuple extend(Function, X> function) {
return new TwoTuple<>(first(), function.apply(this));
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the first element with the provided value.
*/
@Override
public OneTuple first(X value) {
return new OneTuple<>(value);
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the first element with the value returned from the given supplier.
*/
@Override
public OneTuple first(Supplier supplier) {
return new OneTuple<>(supplier.get());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the first element with the value returned by applying the given function to this tuple's first
* element.
*/
@Override
public OneTuple first(Function function) {
return new OneTuple<>(function.apply(first()));
}
/**
* Returns a new tuple of one size smaller by keeping all the values from this tuple except the
* first element.
*/
@Override
public EmptyTuple dropFirst() {
return new EmptyTuple<>();
}
/**
* Extends the tuple to which this is applied by adding the provided value to the end.
*
* This is especially useful in functional contexts. For instance:
*
*
* twoTupleStream = oneTupleStream.map(OneTuple.extendWith(value));
*
*
* @see #extend(Object)
*/
public static
Function, TwoTuple> extendWith(X value) {
return tuple -> tuple.extend(value);
}
/**
* Extends the tuple to which this is applied by adding the value from the supplier to the end.
*
* This is especially useful in functional contexts. For instance:
*
*
* twoTupleStream = oneTupleStream.map(OneTuple.extendWith(supplier));
*
*
* @see #extend(Object)
*/
public static
Function, TwoTuple> extendWith(Supplier supplier) {
return tuple -> tuple.extend(supplier);
}
/**
* Extends the tuple to which this is applied by adding the value from the function to the end.
*
* This is especially useful in functional contexts. For instance:
*
*
* twoTupleStream = oneTupleStream.map(OneTuple.extendWith(function));
*
*
* @see #extend(Object)
*/
public static
Function, TwoTuple> extendWith(Function, X> function) {
return tuple -> tuple.extend(function);
}
/** Creates a new instance of this class. */
public static OneTuple of(A first) {
return new OneTuple<>(first);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy