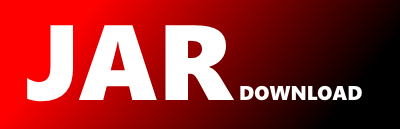
com.mmnaseri.utils.tuples.impl.SixTuple Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tuples4j Show documentation
Show all versions of tuples4j Show documentation
Tiny framework for using tuples as first-class citizens in Java.
package com.mmnaseri.utils.tuples.impl;
import com.mmnaseri.utils.tuples.facade.HasFifth;
import com.mmnaseri.utils.tuples.facade.HasFirst;
import com.mmnaseri.utils.tuples.facade.HasFourth;
import com.mmnaseri.utils.tuples.facade.HasSecond;
import com.mmnaseri.utils.tuples.facade.HasSixth;
import com.mmnaseri.utils.tuples.facade.HasThird;
import java.util.function.Function;
import java.util.function.Supplier;
import static com.mmnaseri.utils.tuples.utils.TupleUtils.checkIndex;
/**
* Class for dealing with a {@link com.mmnaseri.utils.tuples.FixedTuple} with six elements.
*
* @author Milad Naseri ([email protected])
*/
public class SixTuple<
Z, A extends Z, B extends Z, C extends Z, D extends Z, E extends Z, F extends Z>
extends AbstractFixedTuple>
implements HasFirst>,
HasSecond>,
HasThird>,
HasFourth>,
HasFifth>,
HasSixth> {
/** Creates a new instance of this class from the provided values. */
public SixTuple(A first, B second, C third, D fourth, E fifth, F sixth) {
super(first, second, third, fourth, fifth, sixth);
}
/**
* Returns a new tuple by keeping all the values from this tuple and overriding the value at the
* provided index with the value returned from the supplier.
*/
@Override
public SixTuple change(int index, Supplier extends Z> supplier) {
checkIndex(index, size());
return new SixTuple<>(
index == 0 ? supplier.get() : first(),
index == 1 ? supplier.get() : second(),
index == 2 ? supplier.get() : third(),
index == 3 ? supplier.get() : fourth(),
index == 4 ? supplier.get() : fifth(),
index == 5 ? supplier.get() : sixth());
}
/**
* Returns a new tuple by keeping all the values from this tuple and overriding the value at the
* provided index with the value returned from the function.
*/
@Override
public SixTuple change(
int index, Function, ? extends Z> function) {
checkIndex(index, size());
return new SixTuple<>(
index == 0 ? function.apply(this) : first(),
index == 1 ? function.apply(this) : second(),
index == 2 ? function.apply(this) : third(),
index == 3 ? function.apply(this) : fourth(),
index == 4 ? function.apply(this) : fifth(),
index == 5 ? function.apply(this) : sixth());
}
/**
* Returns a new tuple of one size larger by adding the provided value to the end of this tuple.
*/
@Override
public SevenTuple extend(X value) {
return new SevenTuple<>(first(), second(), third(), fourth(), fifth(), sixth(), value);
}
/**
* Returns a new tuple of one size larger by adding the value returned from the supplier to the
* end of this tuple.
*/
@Override
public SevenTuple extend(Supplier supplier) {
return new SevenTuple<>(first(), second(), third(), fourth(), fifth(), sixth(), supplier.get());
}
/**
* Returns a new tuple of one size larger by adding the value returned from the function to the
* end of this tuple.
*/
@Override
public SevenTuple extend(
Function, X> function) {
return new SevenTuple<>(
first(), second(), third(), fourth(), fifth(), sixth(), function.apply(this));
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the first element with the provided value.
*/
@Override
public SixTuple first(X value) {
return new SixTuple<>(value, second(), third(), fourth(), fifth(), sixth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the first element with the value returned from the given supplier.
*/
@Override
public SixTuple first(Supplier supplier) {
return new SixTuple<>(supplier.get(), second(), third(), fourth(), fifth(), sixth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the first element with the value returned by applying the given function to this tuple's first
* element.
*/
@Override
public SixTuple first(Function function) {
return new SixTuple<>(function.apply(first()), second(), third(), fourth(), fifth(), sixth());
}
/**
* Returns a new tuple of one size smaller by keeping all the values from this tuple except the
* first element.
*/
@Override
public FiveTuple dropFirst() {
return new FiveTuple<>(second(), third(), fourth(), fifth(), sixth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the second element with the provided value.
*/
@Override
public SixTuple second(X value) {
return new SixTuple<>(first(), value, third(), fourth(), fifth(), sixth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the second element with the value returned from the given supplier.
*/
@Override
public SixTuple second(Supplier supplier) {
return new SixTuple<>(first(), supplier.get(), third(), fourth(), fifth(), sixth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the second element with the value returned by applying the given function to this tuple's
* second element.
*/
@Override
public SixTuple second(Function function) {
return new SixTuple<>(first(), function.apply(second()), third(), fourth(), fifth(), sixth());
}
/**
* Returns a new tuple of one size smaller by keeping all the values from this tuple except the
* second element.
*/
@Override
public FiveTuple dropSecond() {
return new FiveTuple<>(first(), third(), fourth(), fifth(), sixth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the third element with the provided value.
*/
@Override
public SixTuple third(X value) {
return new SixTuple<>(first(), second(), value, fourth(), fifth(), sixth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the third element with the value returned from the given supplier.
*/
@Override
public SixTuple third(Supplier supplier) {
return new SixTuple<>(first(), second(), supplier.get(), fourth(), fifth(), sixth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the third element with the value returned by applying the given function to this tuple's third
* element.
*/
@Override
public SixTuple third(Function function) {
return new SixTuple<>(first(), second(), function.apply(third()), fourth(), fifth(), sixth());
}
/**
* Returns a new tuple of one size smaller by keeping all the values from this tuple except the
* third element.
*/
@Override
public FiveTuple dropThird() {
return new FiveTuple<>(first(), second(), fourth(), fifth(), sixth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the fourth element with the provided value.
*/
@Override
public SixTuple fourth(X value) {
return new SixTuple<>(first(), second(), third(), value, fifth(), sixth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the fourth element with the value returned from the given supplier.
*/
@Override
public SixTuple fourth(Supplier supplier) {
return new SixTuple<>(first(), second(), third(), supplier.get(), fifth(), sixth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the fourth element with the value returned by applying the given function to this tuple's
* fourth element.
*/
@Override
public SixTuple fourth(Function function) {
return new SixTuple<>(first(), second(), third(), function.apply(fourth()), fifth(), sixth());
}
/**
* Returns a new tuple of one size smaller by keeping all the values from this tuple except the
* fourth element.
*/
@Override
public FiveTuple dropFourth() {
return new FiveTuple<>(first(), second(), third(), fifth(), sixth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the fifth element with the provided value.
*/
@Override
public SixTuple fifth(X value) {
return new SixTuple<>(first(), second(), third(), fourth(), value, sixth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the fifth element with the value returned from the given supplier.
*/
@Override
public SixTuple fifth(Supplier supplier) {
return new SixTuple<>(first(), second(), third(), fourth(), supplier.get(), sixth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the fifth element with the value returned by applying the given function to this tuple's fifth
* element.
*/
@Override
public SixTuple fifth(Function function) {
return new SixTuple<>(first(), second(), third(), fourth(), function.apply(fifth()), sixth());
}
/**
* Returns a new tuple of one size smaller by keeping all the values from this tuple except the
* fifth element.
*/
@Override
public FiveTuple dropFifth() {
return new FiveTuple<>(first(), second(), third(), fourth(), sixth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the sixth element with the provided value.
*/
@Override
public SixTuple sixth(X value) {
return new SixTuple<>(first(), second(), third(), fourth(), fifth(), value);
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the sixth element with the value returned from the given supplier.
*/
@Override
public SixTuple sixth(Supplier supplier) {
return new SixTuple<>(first(), second(), third(), fourth(), fifth(), supplier.get());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the sixth element with the value returned by applying the given function to this tuple's sixth
* element.
*/
@Override
public SixTuple sixth(Function function) {
return new SixTuple<>(first(), second(), third(), fourth(), fifth(), function.apply(sixth()));
}
/**
* Returns a new tuple of one size smaller by keeping all the values from this tuple except the
* sixth element.
*/
@Override
public FiveTuple dropSixth() {
return new FiveTuple<>(first(), second(), third(), fourth(), fifth());
}
/**
* Extends the tuple to which this is applied by adding the provided value to the end.
*
* This is especially useful in functional contexts. For instance:
*
*
* sevenTupleStream = sixTupleStream.map(SixTuple.extendWith(value));
*
*
* @see #extend(Object)
*/
public static <
Z,
A extends Z,
B extends Z,
C extends Z,
D extends Z,
E extends Z,
F extends Z,
X extends Z>
Function, SevenTuple> extendWith(
X value) {
return tuple -> tuple.extend(value);
}
/**
* Extends the tuple to which this is applied by adding the value from the supplier to the end.
*
* This is especially useful in functional contexts. For instance:
*
*
* sevenTupleStream = sixTupleStream.map(SixTuple.extendWith(supplier));
*
*
* @see #extend(Object)
*/
public static <
Z,
A extends Z,
B extends Z,
C extends Z,
D extends Z,
E extends Z,
F extends Z,
X extends Z>
Function, SevenTuple> extendWith(
Supplier supplier) {
return tuple -> tuple.extend(supplier);
}
/**
* Extends the tuple to which this is applied by adding the value from the function to the end.
*
* This is especially useful in functional contexts. For instance:
*
*
* sevenTupleStream = sixTupleStream.map(SixTuple.extendWith(function));
*
*
* @see #extend(Object)
*/
public static <
Z,
A extends Z,
B extends Z,
C extends Z,
D extends Z,
E extends Z,
F extends Z,
X extends Z>
Function, SevenTuple> extendWith(
Function, X> function) {
return tuple -> tuple.extend(function);
}
/** Creates a new instance of this class. */
public static
SixTuple of(A first, B second, C third, D fourth, E fifth, F sixth) {
return new SixTuple<>(first, second, third, fourth, fifth, sixth);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy