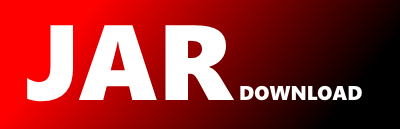
com.mmnaseri.utils.tuples.impl.TwelveTuple Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tuples4j Show documentation
Show all versions of tuples4j Show documentation
Tiny framework for using tuples as first-class citizens in Java.
package com.mmnaseri.utils.tuples.impl;
import com.mmnaseri.utils.tuples.facade.HasEighth;
import com.mmnaseri.utils.tuples.facade.HasEleventh;
import com.mmnaseri.utils.tuples.facade.HasFifth;
import com.mmnaseri.utils.tuples.facade.HasFirst;
import com.mmnaseri.utils.tuples.facade.HasFourth;
import com.mmnaseri.utils.tuples.facade.HasNinth;
import com.mmnaseri.utils.tuples.facade.HasSecond;
import com.mmnaseri.utils.tuples.facade.HasSeventh;
import com.mmnaseri.utils.tuples.facade.HasSixth;
import com.mmnaseri.utils.tuples.facade.HasTenth;
import com.mmnaseri.utils.tuples.facade.HasThird;
import com.mmnaseri.utils.tuples.facade.HasTwelfth;
import java.util.function.Function;
import java.util.function.Supplier;
import static com.mmnaseri.utils.tuples.utils.TupleUtils.checkIndex;
/**
* Class for dealing with a {@link com.mmnaseri.utils.tuples.FixedTuple} with twelve elements.
*
* @author Milad Naseri ([email protected])
*/
public class TwelveTuple<
Z,
A extends Z,
B extends Z,
C extends Z,
D extends Z,
E extends Z,
F extends Z,
G extends Z,
H extends Z,
I extends Z,
J extends Z,
K extends Z,
L extends Z>
extends AbstractFixedTuple>
implements HasFirst>,
HasSecond>,
HasThird>,
HasFourth>,
HasFifth>,
HasSixth>,
HasSeventh>,
HasEighth>,
HasNinth>,
HasTenth>,
HasEleventh>,
HasTwelfth> {
/** Creates a new instance of this class from the provided values. */
public TwelveTuple(
A first,
B second,
C third,
D fourth,
E fifth,
F sixth,
G seventh,
H eighth,
I ninth,
J tenth,
K eleventh,
L twelfth) {
super(
first, second, third, fourth, fifth, sixth, seventh, eighth, ninth, tenth, eleventh,
twelfth);
}
/**
* Returns a new tuple by keeping all the values from this tuple and overriding the value at the
* provided index with the value returned from the supplier.
*/
@Override
public TwelveTuple change(
int index, Supplier extends Z> supplier) {
checkIndex(index, size());
return new TwelveTuple<>(
index == 0 ? supplier.get() : first(),
index == 1 ? supplier.get() : second(),
index == 2 ? supplier.get() : third(),
index == 3 ? supplier.get() : fourth(),
index == 4 ? supplier.get() : fifth(),
index == 5 ? supplier.get() : sixth(),
index == 6 ? supplier.get() : seventh(),
index == 7 ? supplier.get() : eighth(),
index == 8 ? supplier.get() : ninth(),
index == 9 ? supplier.get() : tenth(),
index == 10 ? supplier.get() : eleventh(),
index == 11 ? supplier.get() : twelfth());
}
/**
* Returns a new tuple by keeping all the values from this tuple and overriding the value at the
* provided index with the value returned from the function.
*/
@Override
public TwelveTuple change(
int index,
Function, ? extends Z> function) {
checkIndex(index, size());
return new TwelveTuple<>(
index == 0 ? function.apply(this) : first(),
index == 1 ? function.apply(this) : second(),
index == 2 ? function.apply(this) : third(),
index == 3 ? function.apply(this) : fourth(),
index == 4 ? function.apply(this) : fifth(),
index == 5 ? function.apply(this) : sixth(),
index == 6 ? function.apply(this) : seventh(),
index == 7 ? function.apply(this) : eighth(),
index == 8 ? function.apply(this) : ninth(),
index == 9 ? function.apply(this) : tenth(),
index == 10 ? function.apply(this) : eleventh(),
index == 11 ? function.apply(this) : twelfth());
}
/**
* Returns a new tuple of one size larger by adding the provided value to the end of this tuple.
*/
@Override
public ThirteenOrMoreTuple extend(X value) {
return new ThirteenOrMoreTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth(),
value);
}
/**
* Returns a new tuple of one size larger by adding the value returned from the supplier to the
* end of this tuple.
*/
@Override
public ThirteenOrMoreTuple extend(
Supplier supplier) {
return new ThirteenOrMoreTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth(),
supplier.get());
}
/**
* Returns a new tuple of one size larger by adding the value returned from the function to the
* end of this tuple.
*/
@Override
public ThirteenOrMoreTuple extend(
Function, X> function) {
return new ThirteenOrMoreTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth(),
function.apply(this));
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the first element with the provided value.
*/
@Override
public TwelveTuple first(X value) {
return new TwelveTuple<>(
value,
second(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the first element with the value returned from the given supplier.
*/
@Override
public TwelveTuple first(
Supplier supplier) {
return new TwelveTuple<>(
supplier.get(),
second(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the first element with the value returned by applying the given function to this tuple's first
* element.
*/
@Override
public TwelveTuple first(
Function function) {
return new TwelveTuple<>(
function.apply(first()),
second(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of one size smaller by keeping all the values from this tuple except the
* first element.
*/
@Override
public ElevenTuple dropFirst() {
return new ElevenTuple<>(
second(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the second element with the provided value.
*/
@Override
public TwelveTuple second(X value) {
return new TwelveTuple<>(
first(),
value,
third(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the second element with the value returned from the given supplier.
*/
@Override
public TwelveTuple second(
Supplier supplier) {
return new TwelveTuple<>(
first(),
supplier.get(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the second element with the value returned by applying the given function to this tuple's
* second element.
*/
@Override
public TwelveTuple second(
Function function) {
return new TwelveTuple<>(
first(),
function.apply(second()),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of one size smaller by keeping all the values from this tuple except the
* second element.
*/
@Override
public ElevenTuple dropSecond() {
return new ElevenTuple<>(
first(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the third element with the provided value.
*/
@Override
public TwelveTuple third(X value) {
return new TwelveTuple<>(
first(),
second(),
value,
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the third element with the value returned from the given supplier.
*/
@Override
public TwelveTuple third(
Supplier supplier) {
return new TwelveTuple<>(
first(),
second(),
supplier.get(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the third element with the value returned by applying the given function to this tuple's third
* element.
*/
@Override
public TwelveTuple third(
Function function) {
return new TwelveTuple<>(
first(),
second(),
function.apply(third()),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of one size smaller by keeping all the values from this tuple except the
* third element.
*/
@Override
public ElevenTuple dropThird() {
return new ElevenTuple<>(
first(),
second(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the fourth element with the provided value.
*/
@Override
public TwelveTuple fourth(X value) {
return new TwelveTuple<>(
first(),
second(),
third(),
value,
fifth(),
sixth(),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the fourth element with the value returned from the given supplier.
*/
@Override
public TwelveTuple fourth(
Supplier supplier) {
return new TwelveTuple<>(
first(),
second(),
third(),
supplier.get(),
fifth(),
sixth(),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the fourth element with the value returned by applying the given function to this tuple's
* fourth element.
*/
@Override
public TwelveTuple fourth(
Function function) {
return new TwelveTuple<>(
first(),
second(),
third(),
function.apply(fourth()),
fifth(),
sixth(),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of one size smaller by keeping all the values from this tuple except the
* fourth element.
*/
@Override
public ElevenTuple dropFourth() {
return new ElevenTuple<>(
first(),
second(),
third(),
fifth(),
sixth(),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the fifth element with the provided value.
*/
@Override
public TwelveTuple fifth(X value) {
return new TwelveTuple<>(
first(),
second(),
third(),
fourth(),
value,
sixth(),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the fifth element with the value returned from the given supplier.
*/
@Override
public TwelveTuple fifth(
Supplier supplier) {
return new TwelveTuple<>(
first(),
second(),
third(),
fourth(),
supplier.get(),
sixth(),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the fifth element with the value returned by applying the given function to this tuple's fifth
* element.
*/
@Override
public TwelveTuple fifth(
Function function) {
return new TwelveTuple<>(
first(),
second(),
third(),
fourth(),
function.apply(fifth()),
sixth(),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of one size smaller by keeping all the values from this tuple except the
* fifth element.
*/
@Override
public ElevenTuple dropFifth() {
return new ElevenTuple<>(
first(),
second(),
third(),
fourth(),
sixth(),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the sixth element with the provided value.
*/
@Override
public TwelveTuple sixth(X value) {
return new TwelveTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
value,
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the sixth element with the value returned from the given supplier.
*/
@Override
public TwelveTuple sixth(
Supplier supplier) {
return new TwelveTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
supplier.get(),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the sixth element with the value returned by applying the given function to this tuple's sixth
* element.
*/
@Override
public TwelveTuple sixth(
Function function) {
return new TwelveTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
function.apply(sixth()),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of one size smaller by keeping all the values from this tuple except the
* sixth element.
*/
@Override
public ElevenTuple dropSixth() {
return new ElevenTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the seventh element with the provided value.
*/
@Override
public TwelveTuple seventh(X value) {
return new TwelveTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
sixth(),
value,
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the seventh element with the value returned from the given supplier.
*/
@Override
public TwelveTuple seventh(
Supplier supplier) {
return new TwelveTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
sixth(),
supplier.get(),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the seventh element with the value returned by applying the given function to this tuple's
* seventh element.
*/
@Override
public TwelveTuple seventh(
Function function) {
return new TwelveTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
sixth(),
function.apply(seventh()),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of one size smaller by keeping all the values from this tuple except the
* seventh element.
*/
@Override
public ElevenTuple dropSeventh() {
return new ElevenTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
sixth(),
eighth(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the eighth element with the provided value.
*/
@Override
public TwelveTuple eighth(X value) {
return new TwelveTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
value,
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the eighth element with the value returned from the given supplier.
*/
@Override
public TwelveTuple eighth(
Supplier supplier) {
return new TwelveTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
supplier.get(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the eighth element with the value returned by applying the given function to this tuple's
* eighth element.
*/
@Override
public TwelveTuple eighth(
Function function) {
return new TwelveTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
function.apply(eighth()),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of one size smaller by keeping all the values from this tuple except the
* eighth element.
*/
@Override
public ElevenTuple dropEighth() {
return new ElevenTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
ninth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the ninth element with the provided value.
*/
@Override
public TwelveTuple ninth(X value) {
return new TwelveTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
value,
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the ninth element with the value returned from the given supplier.
*/
@Override
public TwelveTuple ninth(
Supplier supplier) {
return new TwelveTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
supplier.get(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the ninth element with the value returned by applying the given function to this tuple's ninth
* element.
*/
@Override
public TwelveTuple ninth(
Function function) {
return new TwelveTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
function.apply(ninth()),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of one size smaller by keeping all the values from this tuple except the
* ninth element.
*/
@Override
public ElevenTuple dropNinth() {
return new ElevenTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
tenth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the tenth element with the provided value.
*/
@Override
public TwelveTuple tenth(X value) {
return new TwelveTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
ninth(),
value,
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the tenth element with the value returned from the given supplier.
*/
@Override
public TwelveTuple tenth(
Supplier supplier) {
return new TwelveTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
ninth(),
supplier.get(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the tenth element with the value returned by applying the given function to this tuple's tenth
* element.
*/
@Override
public TwelveTuple tenth(
Function function) {
return new TwelveTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
ninth(),
function.apply(tenth()),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of one size smaller by keeping all the values from this tuple except the
* tenth element.
*/
@Override
public ElevenTuple dropTenth() {
return new ElevenTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
ninth(),
eleventh(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the eleventh element with the provided value.
*/
@Override
public TwelveTuple eleventh(X value) {
return new TwelveTuple<>(
first(), second(), third(), fourth(), fifth(), sixth(), seventh(), eighth(), ninth(),
tenth(), value, twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the eleventh element with the value returned from the given supplier.
*/
@Override
public TwelveTuple eleventh(
Supplier supplier) {
return new TwelveTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
ninth(),
tenth(),
supplier.get(),
twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the eleventh element with the value returned by applying the given function to this tuple's
* eleventh element.
*/
@Override
public TwelveTuple eleventh(
Function function) {
return new TwelveTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
ninth(),
tenth(),
function.apply(eleventh()),
twelfth());
}
/**
* Returns a new tuple of one size smaller by keeping all the values from this tuple except the
* eleventh element.
*/
@Override
public ElevenTuple dropEleventh() {
return new ElevenTuple<>(
first(), second(), third(), fourth(), fifth(), sixth(), seventh(), eighth(), ninth(),
tenth(), twelfth());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the twelfth element with the provided value.
*/
@Override
public TwelveTuple twelfth(X value) {
return new TwelveTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
value);
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the twelfth element with the value returned from the given supplier.
*/
@Override
public TwelveTuple twelfth(
Supplier supplier) {
return new TwelveTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
supplier.get());
}
/**
* Returns a new tuple of the same size by keeping all the values from this tuple and overriding
* the twelfth element with the value returned by applying the given function to this tuple's
* twelfth element.
*/
@Override
public TwelveTuple twelfth(
Function function) {
return new TwelveTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh(),
function.apply(twelfth()));
}
/**
* Returns a new tuple of one size smaller by keeping all the values from this tuple except the
* twelfth element.
*/
@Override
public ElevenTuple dropTwelfth() {
return new ElevenTuple<>(
first(),
second(),
third(),
fourth(),
fifth(),
sixth(),
seventh(),
eighth(),
ninth(),
tenth(),
eleventh());
}
/**
* Extends the tuple to which this is applied by adding the provided value to the end.
*
* This is especially useful in functional contexts. For instance:
*
*
* thirteenOrMoreTupleStream = twelveTupleStream.map(TwelveTuple.extendWith(value));
*
*
* @see #extend(Object)
*/
public static <
Z,
A extends Z,
B extends Z,
C extends Z,
D extends Z,
E extends Z,
F extends Z,
G extends Z,
H extends Z,
I extends Z,
J extends Z,
K extends Z,
L extends Z,
X extends Z>
Function<
TwelveTuple,
ThirteenOrMoreTuple>
extendWith(X value) {
return tuple -> tuple.extend(value);
}
/**
* Extends the tuple to which this is applied by adding the value from the supplier to the end.
*
* This is especially useful in functional contexts. For instance:
*
*
* thirteenOrMoreTupleStream = twelveTupleStream.map(TwelveTuple.extendWith(supplier));
*
*
* @see #extend(Object)
*/
public static <
Z,
A extends Z,
B extends Z,
C extends Z,
D extends Z,
E extends Z,
F extends Z,
G extends Z,
H extends Z,
I extends Z,
J extends Z,
K extends Z,
L extends Z,
X extends Z>
Function<
TwelveTuple,
ThirteenOrMoreTuple>
extendWith(Supplier supplier) {
return tuple -> tuple.extend(supplier);
}
/**
* Extends the tuple to which this is applied by adding the value from the function to the end.
*
* This is especially useful in functional contexts. For instance:
*
*
* thirteenOrMoreTupleStream = twelveTupleStream.map(TwelveTuple.extendWith(function));
*
*
* @see #extend(Object)
*/
public static <
Z,
A extends Z,
B extends Z,
C extends Z,
D extends Z,
E extends Z,
F extends Z,
G extends Z,
H extends Z,
I extends Z,
J extends Z,
K extends Z,
L extends Z,
X extends Z>
Function<
TwelveTuple,
ThirteenOrMoreTuple>
extendWith(Function, X> function) {
return tuple -> tuple.extend(function);
}
/** Creates a new instance of this class. */
public static <
Z,
A extends Z,
B extends Z,
C extends Z,
D extends Z,
E extends Z,
F extends Z,
G extends Z,
H extends Z,
I extends Z,
J extends Z,
K extends Z,
L extends Z>
TwelveTuple of(
A first,
B second,
C third,
D fourth,
E fifth,
F sixth,
G seventh,
H eighth,
I ninth,
J tenth,
K eleventh,
L twelfth) {
return new TwelveTuple<>(
first, second, third, fourth, fifth, sixth, seventh, eighth, ninth, tenth, eleventh,
twelfth);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy