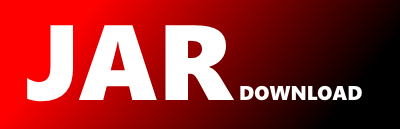
com.mntviews.jreport.JRConnection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mnt-report Show documentation
Show all versions of mnt-report Show documentation
JasperReport server engine
package com.mntviews.jreport;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder;
import com.mntviews.jreport.exception.JRConnectionException;
import com.mntviews.jreport.exception.JRTemplateException;
import lombok.extern.java.Log;
import java.sql.Connection;
import java.sql.SQLException;
import static com.mntviews.jreport.JRContext.checkIfNullThenDefault;
@JsonDeserialize(builder = JRConnection.Builder.class)
@JsonInclude(JsonInclude.Include.NON_NULL)
@Log
public class JRConnection {
@JsonIgnore
private final Connection connection;
@JsonIgnore
private final JRConnectionCreator connectionCreator;
@JsonProperty("url")
private final String url;
@JsonProperty("userName")
private final String userName;
@JsonProperty("password")
private final String password;
@JsonProperty("tag")
private final String tag;
private JRConnection(Builder builder) {
JRProfile jrProfile = JRContext.findProfile();
JRConnection jrConnection = jrProfile.getJrConnection();
if (jrConnection != null) {
JRConnectionCreator connectionCreatorDefault = JRContext.findConnectionCreator();
this.connection = builder.connection;
this.url = checkIfNullThenDefault(builder.url, jrConnection.getUrl());
this.userName = checkIfNullThenDefault(builder.userName, jrConnection.getUserName());
this.password = checkIfNullThenDefault(builder.password, jrConnection.getPassword());
this.connectionCreator = checkIfNullThenDefault(builder.connectionCreator, connectionCreatorDefault);
this.tag = builder.tag;
if (this.url == null && this.tag == null)
throw new JRTemplateException("'url' is not defined");
if (this.userName == null && this.tag == null)
throw new JRTemplateException("'userName' is not defined");
if (this.password == null && this.tag == null)
throw new JRTemplateException("'password' is not defined");
} else { /* Profile initialization */
this.url = builder.url;
this.userName = builder.userName;
this.password = builder.password;
this.tag = builder.tag;
this.connectionCreator = null;
this.connection = null;
}
}
@JsonIgnore
public JRConnectionCreator getConnectionCreator() {
return connectionCreator;
}
public String getUrl() {
return url;
}
public String getUserName() {
return userName;
}
public String getPassword() {
return password;
}
public String getTag() {
return tag;
}
@JsonIgnore
public Connection getConnection() {
if (connection != null)
return connection;
else {
if (connectionCreator != null) {
try {
return connectionCreator.createConnection(url, userName, password);
} catch (SQLException e) {
throw new JRConnectionException(e);
}
} else
throw new JRConnectionException("'connectionCreator' is not defined");
}
}
public static Builder custom() {
return new JRConnection.Builder();
}
public static JRConnection clone(JRConnection jrConnection) {
return new JRConnection.Builder()
.withTag(jrConnection.getTag())
.withUrl(jrConnection.getUrl())
.withUserName(jrConnection.getUserName())
.withPassword(jrConnection.getPassword())
.withConnectionCreator(jrConnection.getConnectionCreator()).build();
}
@JsonPOJOBuilder
public static class Builder {
private Connection connection;
private JRConnectionCreator connectionCreator;
@JsonProperty("url")
private String url;
@JsonProperty("userName")
private String userName;
@JsonProperty("password")
private String password;
@JsonProperty("tag")
private String tag;
public JRConnection build() {
return new JRConnection(this);
}
@JsonIgnore
public Builder withConnection(Connection connection) {
this.connection = connection;
return this;
}
@JsonProperty("url")
public Builder withUrl(String url) {
this.url = url;
return this;
}
@JsonProperty("userName")
public Builder withUserName(String userName) {
this.userName = userName;
return this;
}
@JsonProperty("password")
public Builder withPassword(String password) {
this.password = password;
return this;
}
@JsonProperty("tag")
public Builder withTag(String tag) {
this.tag = tag;
return this;
}
public Builder withConnectionCreator(JRConnectionCreator connectionCreator) {
this.connectionCreator = connectionCreator;
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy