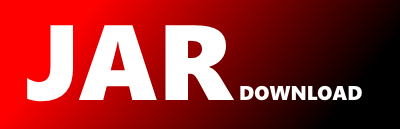
com.mntviews.jreport.JRContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mnt-report Show documentation
Show all versions of mnt-report Show documentation
JasperReport server engine
package com.mntviews.jreport;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.mntviews.jreport.exception.JRDefaultContextException;
import lombok.extern.slf4j.Slf4j;
import java.io.*;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
/**
* Represents default context loaded on the initialization. Each object can be configured by corresponding json file
* for each part of the config
*/
@Slf4j
public class JRContext {
public final static String version = "1.0.7";
private JRContext() {
}
public static final String DEFAULT_PROFILE_TAG = "DEFAULT";
public static final String CONFIG_FILE_PATH = "config/";
public static final String DIRECTORY_PREFIX = "jr-";
public static final String DEFAULT_FILE_PATH = CONFIG_FILE_PATH + "jr-default/";
public static final String DEFAULT_OUTPUT_MAIL = "jr-output-mail-default.json";
public static final String DEFAULT_OUTPUT_TELEGRAM = "jr-output-telegram-default.json";
public static final String DEFAULT_CONNECTION = "jr-connection-default.json";
public static final String DEFAULT_TEMPLATE_SOURCE_DB = "jr-template-source-db-default.json";
public static final String DEFAULT_TEMPLATE_SOURCE_FILE = "jr-template-source-file-default.json";
public static final String DEFAULT_REPORT = "jr-report-default.json";
public static final String DEFAULT_FILE = "jr-file-default.json";
public static final String DEFAULT_PARAM_LIST = "jr-param-list-default.json";
public static final String DEFAULT_INPUT_SOURCE_DB = "jr-input-source-db-default.json";
public static final String DEFAULT_PACKAGE = "jr-package-default.json";
public static final String DEFAULT_CONNECTION_TAG = "dafault";
private static final Map jrProfileMap = new HashMap<>();
static T checkIfNullThenDefault(T obj, T defaultObj) {
return obj != null ? obj : defaultObj;
}
private static final ThreadLocal currentProfileTag = new ThreadLocal<>();
public static String getProfileTag() {
return currentProfileTag.get();
}
public static void setProfileTag(String profile) {
if (profile == null)
throw new JRDefaultContextException("Profile tag is null");
currentProfileTag.set(profile);
}
public static InputStream findInputStream(String filePath, String fileName) {
try {
if (Files.exists(Paths.get(filePath + fileName)))
return new BufferedInputStream(new FileInputStream(filePath + fileName));
else {
if (Files.exists(Paths.get(DEFAULT_FILE_PATH + fileName)))
return new BufferedInputStream(new FileInputStream(DEFAULT_FILE_PATH + fileName));
else {
InputStream inputStream = JRContext.class.getClassLoader().getResourceAsStream(DEFAULT_FILE_PATH + fileName);
if (inputStream == null)
throw new JRDefaultContextException(DEFAULT_FILE_PATH + fileName + " not found");
return inputStream;
}
}
} catch (FileNotFoundException e) {
throw new JRDefaultContextException(e);
}
}
static {
log.info("mnt-report version=" + version);
setProfileTag(DEFAULT_PROFILE_TAG);
ObjectMapper objectMapper = new ObjectMapper();
try {
Path configPath = Paths.get(CONFIG_FILE_PATH);
List profilePaths;
if (Files.exists(configPath)) {
profilePaths = Files.walk(configPath, 1)
.filter(Files::isDirectory)
.filter(p -> p.getFileName().toString().startsWith(DIRECTORY_PREFIX))
.filter((p) -> !p.getFileName().toString().equalsIgnoreCase(DIRECTORY_PREFIX + DEFAULT_PROFILE_TAG))
.collect(Collectors.toList());
} else
profilePaths = new ArrayList<>();
profilePaths.add(0, Paths.get(CONFIG_FILE_PATH + DIRECTORY_PREFIX + DEFAULT_PROFILE_TAG.toLowerCase()));
log.debug("Report context initialization");
for (Path path : profilePaths) {
String profileTag = path.getFileName().toString().toUpperCase().replaceFirst(DIRECTORY_PREFIX.toUpperCase(), "");
JRProfile jrProfile = new JRProfile();
setProfileTag(profileTag);
String pathName = CONFIG_FILE_PATH + path.getFileName() + "/";
jrProfileMap.put(profileTag, jrProfile);
log.debug("read config path " + pathName + " for profile tag " + profileTag);
jrProfile.setJrOutputMail(objectMapper.readValue(findInputStream(pathName, DEFAULT_OUTPUT_MAIL), JROutputMail.class));
log.debug(String.format("jrOutputMail=%s", objectMapper.writeValueAsString(jrProfile.getJrOutputMail())));
jrProfile.setJrOutputTelegram(objectMapper.readValue(findInputStream(pathName, DEFAULT_OUTPUT_TELEGRAM), JROutputTelegram.class));
log.debug(String.format("jrOutputTelegram=%s", objectMapper.writeValueAsString(jrProfile.getJrOutputTelegram())));
jrProfile.setJrConnection(objectMapper.readValue(findInputStream(pathName, DEFAULT_CONNECTION), JRConnection.class));
log.debug(String.format("jrConnection=%s", objectMapper.writeValueAsString(jrProfile.getJrConnection())));
jrProfile.setJrTemplateSourceDB(objectMapper.readValue(findInputStream(pathName, DEFAULT_TEMPLATE_SOURCE_DB), JRTemplateSourceDB.class));
log.debug(String.format("jrTemplateSourceDB=%s", objectMapper.writeValueAsString(jrProfile.getJrTemplateSourceDB())));
jrProfile.setJrTemplateSourceFile(objectMapper.readValue(findInputStream(pathName, DEFAULT_TEMPLATE_SOURCE_FILE), JRTemplateSourceFile.class));
log.debug(String.format("jrTemplateSourceFile=%s", objectMapper.writeValueAsString(jrProfile.getJrTemplateSourceFile())));
jrProfile.setJrInputSourceDB(objectMapper.readValue(findInputStream(pathName, DEFAULT_INPUT_SOURCE_DB), JRInputSourceDB.class));
log.debug(String.format("jrInputSourceDB=%s", objectMapper.writeValueAsString(jrProfile.getJrInputSourceDB())));
jrProfile.setJrParamList(objectMapper.readValue(findInputStream(pathName, DEFAULT_PARAM_LIST), new TypeReference>() {
}));
log.debug(String.format("jrParamList=%s", objectMapper.writeValueAsString(jrProfile.getJrParamList())));
jrProfile.setJrReport(objectMapper.readValue(findInputStream(pathName, DEFAULT_REPORT), JRReport.class));
log.debug(String.format("jrReport=%s", objectMapper.writeValueAsString(jrProfile.getJrReport())));
jrProfile.setJrFile(objectMapper.readValue(findInputStream(pathName, DEFAULT_FILE), JRFile.class));
log.debug(String.format("jrFile=%s", objectMapper.writeValueAsString(jrProfile.getJrFile())));
jrProfile.setJrPackage(objectMapper.readValue(findInputStream(pathName, DEFAULT_PACKAGE), JRPackage.class));
log.debug(String.format("jrPackage=%s", objectMapper.writeValueAsString(jrProfile.getJrPackage())));
}
setProfileTag(DEFAULT_PROFILE_TAG);
} catch (Exception e) {
log.error(e.getMessage());
throw new JRDefaultContextException(e);
}
}
static JRProfile findProfileByTag(String profileTag) {
JRProfile jrProfile;
if (profileTag != null)
jrProfile = jrProfileMap.get(profileTag.toUpperCase());
else jrProfile = jrProfileMap.get(DEFAULT_PROFILE_TAG);
if (jrProfile == null)
throw new JRDefaultContextException(String.format("Profile not found {profileTag=%s}", profileTag));
return jrProfile;
}
public static JRProfile findProfile() {
return findProfileByTag(getProfileTag());
}
public static void setDefaultTemplateDBExtractor(JRTemplateDBExtractor jrTemplateDBExtractor) {
findProfileByTag(DEFAULT_PROFILE_TAG).setJrTemplateDBExtractor(jrTemplateDBExtractor);
}
public static void setDefaultConnectionCreator(JRConnectionCreator jrConnectionCreator) {
findProfileByTag(DEFAULT_PROFILE_TAG).setJrConnectionCreator(jrConnectionCreator);
}
public static JRTemplateDBExtractor findTemplateDBExtractor() {
JRTemplateDBExtractor templateDBExtractor = findProfile().getJrTemplateDBExtractor();
if (templateDBExtractor == null)
return findProfileByTag(DEFAULT_PROFILE_TAG).getJrTemplateDBExtractor();
else return templateDBExtractor;
}
public static JRConnectionCreator findConnectionCreator() {
JRConnectionCreator jrConnectionCreator = findProfile().getJrConnectionCreator();
if (jrConnectionCreator == null)
return findProfileByTag(DEFAULT_PROFILE_TAG).getJrConnectionCreator();
else return jrConnectionCreator;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy